mirror of
https://code.videolan.org/videolan/vlc
synced 2024-09-04 09:11:33 +02:00
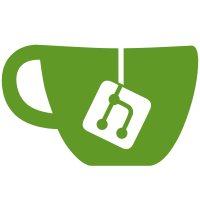
* Removed duplicate function checks from configure.in. * Added extra magic to Makefile.modules so that the module Makefiles are now ridiculously simple. And I mean *simple*. Check it! This will make a possible switch to full autoconf/automake a lot easier. * Added the vlc version name to the plugin symbols, to be sure we only load plugins with the same version number. A nasty consequence is that you need to rebuild your tree after midnight if you are using a CVS tree :-) * Got rid of modules_export.h by #defining exported functions in the same header as their prototype. * Added modules_inner.h and other commonly used .h files to common.h so there are less and less files to include, and renamed common.h to <videolan/vlc.h>. * First modifications to the module handling system towards my ultimate goal to get rid of the *_Probe functions. Got rid of TestMethod and TestCPU, as well as src/misc/tests.c. * Wrote the chroma plugin handling functions. No YUV functions have been ported yet because it'ls a lot of work, but the core system works, I tried it with a naive yv12->rgb16 plugin (which will disappear when the real functions are ready). * Made a lot of functions in dvd_summary.c one-liners to avoid wasting too many output lines. * Fixed a segfault in input_dvd.c:DVDInit. * Added a fixfiles.sh script in plugins/gtk to be run after Glade has generated its C files. * Did some work on the KDE interface to make it suck a bit less. It still segfaults, but at least it runs and it looks less ugly. * RGB SDL rendering works again, though in 16bpp only. * Made plugins/vcd/linux_cdrom_tools.c independent of any vlc structure so that it'll be easily put in a library. Maybe libdvdcss? * Fixed VCD date display. * Merged vout_xvideo.c, vout_x11.c and vout_common.c into xcommon.c. * Wrote non-Shm XVideo output. * Made X11 output work again. Still pretty unstable, only works for 16bpp. * Additional french translation in po/fr.po. Any taker for the rest? * Fixed a segfault in video_output.c when the allocated pictures were not direct buffers. * If $DISPLAY isn't set, don't try to run the Gtk+ interface. * Replaced 48x48 .xpm images with 32x32 ones to conform to Debian policy (Closes Debian bug #126939). * Removed the automatic ./configure launch when running `make all' for the first time. Stuff currently more broken than it ought to be: * The wall filter. Being fixed. * x11 and sdl plugins for depth != 16bpp. * Software YUV. * gvlc, gnome-vlc, kvlc shortcuts. Use --intf instead for the moment.
93 lines
4.4 KiB
C
93 lines
4.4 KiB
C
/*****************************************************************************
|
|
* intf_playlist.h : Playlist functions
|
|
*****************************************************************************
|
|
* Copyright (C) 1999, 2000 VideoLAN
|
|
* $Id: intf_playlist.h,v 1.5 2001/12/30 07:09:54 sam Exp $
|
|
*
|
|
* Authors: Samuel Hocevar <sam@zoy.org>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111, USA.
|
|
*****************************************************************************/
|
|
|
|
/*****************************************************************************
|
|
* playlist_item_t: playlist item
|
|
*****************************************************************************/
|
|
typedef struct playlist_item_s
|
|
{
|
|
char* psz_name;
|
|
int i_type; /* unused yet */
|
|
int i_status; /* unused yet */
|
|
} playlist_item_t;
|
|
|
|
/*****************************************************************************
|
|
* playlist_t: playlist structure
|
|
*****************************************************************************
|
|
* The structure contains information about the size and browsing mode of
|
|
* the playlist, a change lock, a dynamic array of playlist items, and a
|
|
* current item which is an exact copy of one of the array members.
|
|
*****************************************************************************/
|
|
typedef struct playlist_s
|
|
{
|
|
int i_index; /* current index */
|
|
int i_size; /* total size */
|
|
|
|
int i_mode; /* parse mode (random, forward, backward) */
|
|
int i_seed; /* seed used for random mode */
|
|
boolean_t b_stopped;
|
|
|
|
vlc_mutex_t change_lock;
|
|
|
|
playlist_item_t current;
|
|
playlist_item_t* p_item;
|
|
} playlist_t;
|
|
|
|
/* Used by intf_PlaylistAdd */
|
|
#define PLAYLIST_START 0
|
|
#define PLAYLIST_END -1
|
|
|
|
/* Playlist parsing mode */
|
|
#define PLAYLIST_REPEAT_CURRENT 0 /* Keep playing current item */
|
|
#define PLAYLIST_FORWARD 1 /* Parse playlist until end */
|
|
#define PLAYLIST_BACKWARD -1 /* Parse backwards */
|
|
#define PLAYLIST_FORWARD_LOOP 2 /* Parse playlist and loop */
|
|
#define PLAYLIST_BACKWARD_LOOP -2 /* Parse backwards and loop */
|
|
#define PLAYLIST_RANDOM 3 /* Shuffle play */
|
|
#define PLAYLIST_REVERSE_RANDOM -3 /* Reverse shuffle play */
|
|
|
|
/*****************************************************************************
|
|
* Prototypes
|
|
*****************************************************************************/
|
|
#ifndef PLUGIN
|
|
playlist_t * intf_PlaylistCreate ( void );
|
|
void intf_PlaylistInit ( playlist_t * p_playlist );
|
|
int intf_PlaylistAdd ( playlist_t * p_playlist,
|
|
int i_pos, const char * psz_item );
|
|
int intf_PlaylistDelete ( playlist_t * p_playlist, int i_pos );
|
|
void intf_PlaylistNext ( playlist_t * p_playlist );
|
|
void intf_PlaylistPrev ( playlist_t * p_playlist );
|
|
void intf_PlaylistDestroy ( playlist_t * p_playlist );
|
|
void intf_PlaylistJumpto ( playlist_t * p_playlist , int i_pos);
|
|
void intf_UrlDecode ( char * );
|
|
#else
|
|
# define intf_PlaylistAdd p_symbols->intf_PlaylistAdd
|
|
# define intf_PlaylistDelete p_symbols->intf_PlaylistDelete
|
|
# define intf_PlaylistNext p_symbols->intf_PlaylistNext
|
|
# define intf_PlaylistPrev p_symbols->intf_PlaylistPrev
|
|
# define intf_PlaylistDestroy p_symbols->intf_PlaylistDestroy
|
|
# define intf_PlaylistJumpto p_symbols->intf_PlaylistJumpto
|
|
# define intf_UrlDecode p_symbols->intf_UrlDecode
|
|
#endif
|
|
|