mirror of
https://code.videolan.org/videolan/vlc
synced 2024-07-25 09:41:30 +02:00
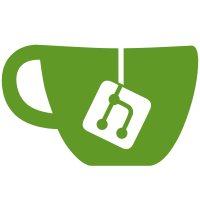
Any LibVLC users could request a timer from the player. This Media Player timer has its own event API since: - It is only used to receive time update points: - The timer is not locked by the player lock. Indeed the player lock can be too "slow" (it can be recursive, it is used by the playlist, and is it held when sending all events). So it's not a good idea to hold this lock for every frame/sample updates. - The minimum delay between each updates can be configured: it avoids to flood the UI when playing a media file with very high fps or very low audio sample size. The libvlc_media_player_time_point struct is used by timer update callbacks. This public struct hold all the informations to interpolate a time at a given date. It can be done with the libvlc_media_player_time_point_interpolate() helper. That way, it is now possible to get the last player time without holding any locks. There is only one type of timer (for now): libvlc_media_player_watch_time(): update are sent only when a frame or a sample is outputted. Users of this timer should take into account that the delay between each updates is not regular and can be up to 1seconds (depending of the input). In that case, they should use their own timer (from their mainloop) and use libvlc_media_player_time_point_interpolate() to get the last time.
75 lines
2.4 KiB
C
75 lines
2.4 KiB
C
/*****************************************************************************
|
|
* media_player_internal.h : Definition of opaque structures for libvlc exported API
|
|
* Also contains some internal utility functions
|
|
*****************************************************************************
|
|
* Copyright (C) 2005-2009 VLC authors and VideoLAN
|
|
*
|
|
* Authors: Clément Stenac <zorglub@videolan.org>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU Lesser General Public License as published by
|
|
* the Free Software Foundation; either version 2.1 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public License
|
|
* along with this program; if not, write to the Free Software Foundation,
|
|
* Inc., 51 Franklin Street, Fifth Floor, Boston MA 02110-1301, USA.
|
|
*****************************************************************************/
|
|
|
|
#ifndef _LIBVLC_MEDIA_PLAYER_INTERNAL_H
|
|
#define _LIBVLC_MEDIA_PLAYER_INTERNAL_H 1
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
# include "config.h"
|
|
#endif
|
|
|
|
#include <vlc/vlc.h>
|
|
#include <vlc/libvlc_media.h>
|
|
#include <vlc_input.h>
|
|
#include <vlc_player.h>
|
|
#include <vlc_viewpoint.h>
|
|
#include "media_internal.h"
|
|
|
|
#include "../modules/audio_filter/equalizer_presets.h"
|
|
|
|
struct libvlc_media_player_t
|
|
{
|
|
struct vlc_object_t obj;
|
|
vlc_atomic_rc_t rc;
|
|
|
|
vlc_player_t *player;
|
|
vlc_player_listener_id *listener;
|
|
vlc_player_aout_listener_id *aout_listener;
|
|
|
|
struct libvlc_instance_t * p_libvlc_instance; /* Parent instance */
|
|
libvlc_media_t * p_md; /* current media descriptor */
|
|
libvlc_event_manager_t event_manager;
|
|
|
|
struct {
|
|
vlc_player_timer_id *id;
|
|
libvlc_media_player_watch_time_on_update on_update;
|
|
libvlc_media_player_watch_time_on_discontinuity on_discontinuity;
|
|
void *cbs_data;
|
|
} timer;
|
|
};
|
|
|
|
libvlc_track_description_t * libvlc_get_track_description(
|
|
libvlc_media_player_t *p_mi,
|
|
enum es_format_category_e cat );
|
|
|
|
/**
|
|
* Internal equalizer structure.
|
|
*/
|
|
struct libvlc_equalizer_t
|
|
{
|
|
float f_preamp;
|
|
float f_amp[EQZ_BANDS_MAX];
|
|
};
|
|
|
|
#endif
|