mirror of
https://code.videolan.org/videolan/vlc
synced 2024-08-27 04:21:53 +02:00
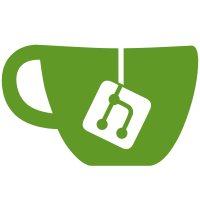
* Fixes to aout_darwin.c by Colin Delacroix <colin@zoy.org>. * Fixes to configure.in, Makefile.in and main.c (Altivec detection) by Eugenio Jarosiewicz <ej0@cise.ufl.edu>. * Added Colin and Eugenio to the AUTHORS file.
112 lines
4.5 KiB
C
112 lines
4.5 KiB
C
/*****************************************************************************
|
|
* ts.c : Transport Stream input module for vlc
|
|
*****************************************************************************
|
|
* Copyright (C) 2000 VideoLAN
|
|
* $Id: ts.c,v 1.3 2001/03/21 13:42:34 sam Exp $
|
|
*
|
|
* Authors: Henri Fallon <henri@via.ecp.fr>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111, USA.
|
|
*****************************************************************************/
|
|
|
|
#define MODULE_NAME ts
|
|
#include "modules_inner.h"
|
|
|
|
/*****************************************************************************
|
|
* Preamble
|
|
*****************************************************************************/
|
|
#include "defs.h"
|
|
|
|
#include <stdlib.h> /* malloc(), free() */
|
|
#include <string.h> /* strdup() */
|
|
|
|
#include "config.h"
|
|
#include "common.h" /* boolean_t, byte_t */
|
|
#include "threads.h"
|
|
#include "mtime.h"
|
|
|
|
#include "modules.h"
|
|
|
|
/*****************************************************************************
|
|
* Build configuration tree.
|
|
*****************************************************************************/
|
|
MODULE_CONFIG_START
|
|
ADD_WINDOW( "Configuration for TS module" )
|
|
ADD_COMMENT( "foobar !" )
|
|
MODULE_CONFIG_END
|
|
|
|
/*****************************************************************************
|
|
* Capabilities defined in the other files.
|
|
*****************************************************************************/
|
|
void _M( input_getfunctions )( function_list_t * p_function_list );
|
|
|
|
/*****************************************************************************
|
|
* InitModule: get the module structure and configuration.
|
|
*****************************************************************************
|
|
* We have to fill psz_name, psz_longname and psz_version. These variables
|
|
* will be strdup()ed later by the main application because the module can
|
|
* be unloaded later to save memory, and we want to be able to access this
|
|
* data even after the module has been unloaded.
|
|
*****************************************************************************/
|
|
MODULE_INIT
|
|
{
|
|
p_module->psz_name = MODULE_STRING;
|
|
p_module->psz_longname = "ISO 13818-1 MPEG Transport Stream input module";
|
|
p_module->psz_version = VERSION;
|
|
|
|
p_module->i_capabilities = MODULE_CAPABILITY_NULL
|
|
| MODULE_CAPABILITY_INPUT;
|
|
|
|
return( 0 );
|
|
}
|
|
|
|
/*****************************************************************************
|
|
* ActivateModule: set the module to an usable state.
|
|
*****************************************************************************
|
|
* This function fills the capability functions and the configuration
|
|
* structure. Once ActivateModule() has been called, the i_usage can
|
|
* be set to 0 and calls to NeedModule() be made to increment it. To unload
|
|
* the module, one has to wait until i_usage == 0 and call DeactivateModule().
|
|
*****************************************************************************/
|
|
MODULE_ACTIVATE
|
|
{
|
|
p_module->p_functions = malloc( sizeof( module_functions_t ) );
|
|
if( p_module->p_functions == NULL )
|
|
{
|
|
return( -1 );
|
|
}
|
|
|
|
_M( input_getfunctions )( &p_module->p_functions->input );
|
|
|
|
p_module->p_config = p_config;
|
|
|
|
return( 0 );
|
|
}
|
|
|
|
/*****************************************************************************
|
|
* DeactivateModule: make sure the module can be unloaded.
|
|
*****************************************************************************
|
|
* This function must only be called when i_usage == 0. If it successfully
|
|
* returns, i_usage can be set to -1 and the module unloaded. Be careful to
|
|
* lock usage_lock during the whole process.
|
|
*****************************************************************************/
|
|
MODULE_DEACTIVATE
|
|
{
|
|
free( p_module->p_functions );
|
|
|
|
return( 0 );
|
|
}
|
|
|