mirror of
https://git.videolan.org/git/ffmpeg.git
synced 2024-07-22 20:21:30 +02:00
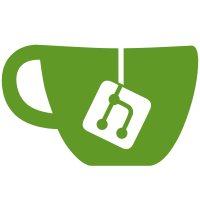
The query_formats function of the showpalette filter tries to allocate two lists of formats which on success are attached to more permanent objects (AVFilterLinks) for storage afterwards. If attaching a list to an AVFilterLink succeeds, the link becomes one (in this case the only one) of the owners of the list. Yet if attaching the first list to its link succeeds and attaching the second list fails, both lists were manually freed, which means that the first link's pointer to the first list becomes dangling and there will be a double-free when the first link is cleaned up automatically. This commit fixes this by removing the custom free code; this will temporarily add a leaking codepath (if attaching a list to a link fails, the list will leak), but this will be fixed shortly by making sure that an AVFilterFormats without owner will be automatically freed when attaching it to an AVFilterLink fails. Notice at most one list leaks because as of this commit a new list is only allocated after the old list has been successfully attached to a link. Reviewed-by: Nicolas George <george@nsup.org> Signed-off-by: Andreas Rheinhardt <andreas.rheinhardt@gmail.com>
125 lines
3.7 KiB
C
125 lines
3.7 KiB
C
/*
|
|
* This file is part of FFmpeg.
|
|
*
|
|
* FFmpeg is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* FFmpeg is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with FFmpeg; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
/**
|
|
* @file
|
|
* Display frame palette (AV_PIX_FMT_PAL8)
|
|
*/
|
|
|
|
#include "libavutil/avassert.h"
|
|
#include "libavutil/opt.h"
|
|
#include "avfilter.h"
|
|
#include "formats.h"
|
|
#include "internal.h"
|
|
#include "video.h"
|
|
|
|
typedef struct ShowPaletteContext {
|
|
const AVClass *class;
|
|
int size;
|
|
} ShowPaletteContext;
|
|
|
|
#define OFFSET(x) offsetof(ShowPaletteContext, x)
|
|
#define FLAGS AV_OPT_FLAG_FILTERING_PARAM|AV_OPT_FLAG_VIDEO_PARAM
|
|
static const AVOption showpalette_options[] = {
|
|
{ "s", "set pixel box size", OFFSET(size), AV_OPT_TYPE_INT, {.i64=30}, 1, 100, FLAGS },
|
|
{ NULL }
|
|
};
|
|
|
|
AVFILTER_DEFINE_CLASS(showpalette);
|
|
|
|
static int query_formats(AVFilterContext *ctx)
|
|
{
|
|
static const enum AVPixelFormat in_fmts[] = {AV_PIX_FMT_PAL8, AV_PIX_FMT_NONE};
|
|
static const enum AVPixelFormat out_fmts[] = {AV_PIX_FMT_RGB32, AV_PIX_FMT_NONE};
|
|
int ret = ff_formats_ref(ff_make_format_list(in_fmts),
|
|
&ctx->inputs[0]->out_formats);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
return ff_formats_ref(ff_make_format_list(out_fmts),
|
|
&ctx->outputs[0]->in_formats);
|
|
}
|
|
|
|
static int config_output(AVFilterLink *outlink)
|
|
{
|
|
AVFilterContext *ctx = outlink->src;
|
|
const ShowPaletteContext *s = ctx->priv;
|
|
outlink->w = outlink->h = 16 * s->size;
|
|
return 0;
|
|
}
|
|
|
|
static void disp_palette(AVFrame *out, const AVFrame *in, int size)
|
|
{
|
|
int x, y, i, j;
|
|
uint32_t *dst = (uint32_t *)out->data[0];
|
|
const int dst_linesize = out->linesize[0] >> 2;
|
|
const uint32_t *pal = (uint32_t *)in->data[1];
|
|
|
|
for (y = 0; y < 16; y++)
|
|
for (x = 0; x < 16; x++)
|
|
for (j = 0; j < size; j++)
|
|
for (i = 0; i < size; i++)
|
|
dst[(y*dst_linesize + x) * size + j*dst_linesize + i] = pal[y*16 + x];
|
|
}
|
|
|
|
static int filter_frame(AVFilterLink *inlink, AVFrame *in)
|
|
{
|
|
AVFrame *out;
|
|
AVFilterContext *ctx = inlink->dst;
|
|
const ShowPaletteContext *s = ctx->priv;
|
|
AVFilterLink *outlink = ctx->outputs[0];
|
|
|
|
out = ff_get_video_buffer(outlink, outlink->w, outlink->h);
|
|
if (!out) {
|
|
av_frame_free(&in);
|
|
return AVERROR(ENOMEM);
|
|
}
|
|
av_frame_copy_props(out, in);
|
|
disp_palette(out, in, s->size);
|
|
av_frame_free(&in);
|
|
return ff_filter_frame(outlink, out);
|
|
}
|
|
|
|
static const AVFilterPad showpalette_inputs[] = {
|
|
{
|
|
.name = "default",
|
|
.type = AVMEDIA_TYPE_VIDEO,
|
|
.filter_frame = filter_frame,
|
|
},
|
|
{ NULL }
|
|
};
|
|
|
|
static const AVFilterPad showpalette_outputs[] = {
|
|
{
|
|
.name = "default",
|
|
.type = AVMEDIA_TYPE_VIDEO,
|
|
.config_props = config_output,
|
|
},
|
|
{ NULL }
|
|
};
|
|
|
|
AVFilter ff_vf_showpalette = {
|
|
.name = "showpalette",
|
|
.description = NULL_IF_CONFIG_SMALL("Display frame palette."),
|
|
.priv_size = sizeof(ShowPaletteContext),
|
|
.query_formats = query_formats,
|
|
.inputs = showpalette_inputs,
|
|
.outputs = showpalette_outputs,
|
|
.priv_class = &showpalette_class,
|
|
};
|