mirror of
https://git.videolan.org/git/ffmpeg.git
synced 2024-09-16 11:54:09 +02:00
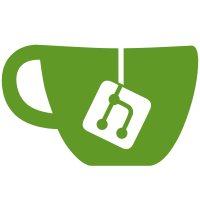
This filter, when used in the "pad" mode, currently makes the distinction between limited and full range solely by testing for YUVJ pixel formats at link setup time. This is deprecated and should be improved to perform the detection based on the per-frame metadata. In order to make this distinction based on color range metadata, which is only known at the time of filtering frames, for simplicity, we simply allocate two copies of the "black" frame - one for limited range and the other for full range metadata. This could be done more dynamically (e.g. as-needed or simply by blitting the appropriate pixel value directly), but this change is relatively simple and preserves the structure of the existing code. This commit actually fixes a bug in FATE - the new output is correct for the first time. The previous md5 ref was of a frame that incorrectly combined full-range pixel data with limited-range black fields. The corresponding result has been updated. Signed-off-by: Niklas Haas <git@haasn.dev>
85 lines
2.5 KiB
C
85 lines
2.5 KiB
C
/*
|
|
* Copyright (c) 2011 Stefano Sabatini
|
|
* Copyright (c) 2010 Baptiste Coudurier
|
|
* Copyright (c) 2003 Michael Zucchi <notzed@ximian.com>
|
|
*
|
|
* This file is part of FFmpeg.
|
|
*
|
|
* FFmpeg is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* FFmpeg is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with FFmpeg; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
/**
|
|
* @file
|
|
* temporal field interlace filter, ported from MPlayer/libmpcodecs
|
|
*/
|
|
#ifndef AVFILTER_TINTERLACE_H
|
|
#define AVFILTER_TINTERLACE_H
|
|
|
|
#include "libavutil/bswap.h"
|
|
#include "libavutil/opt.h"
|
|
#include "libavutil/pixdesc.h"
|
|
#include "drawutils.h"
|
|
#include "avfilter.h"
|
|
|
|
#define TINTERLACE_FLAG_VLPF 01
|
|
#define TINTERLACE_FLAG_CVLPF 2
|
|
#define TINTERLACE_FLAG_EXACT_TB 4
|
|
#define TINTERLACE_FLAG_BYPASS_IL 8
|
|
|
|
enum VLPFilter {
|
|
VLPF_OFF = 0,
|
|
VLPF_LIN = 1,
|
|
VLPF_CMP = 2,
|
|
};
|
|
|
|
enum TInterlaceMode {
|
|
MODE_MERGE = 0,
|
|
MODE_DROP_EVEN,
|
|
MODE_DROP_ODD,
|
|
MODE_PAD,
|
|
MODE_INTERLEAVE_TOP,
|
|
MODE_INTERLEAVE_BOTTOM,
|
|
MODE_INTERLACEX2,
|
|
MODE_MERGEX2,
|
|
MODE_NB,
|
|
};
|
|
|
|
enum InterlaceScanMode {
|
|
MODE_TFF = 0,
|
|
MODE_BFF,
|
|
};
|
|
|
|
typedef struct TInterlaceContext {
|
|
const AVClass *class;
|
|
int mode; ///< TInterlaceMode, interlace mode selected
|
|
AVRational preout_time_base;
|
|
int flags; ///< flags affecting interlacing algorithm
|
|
int lowpass; ///< legacy interlace filter lowpass mode
|
|
int vsub; ///< chroma vertical subsampling
|
|
AVFrame *cur;
|
|
AVFrame *next;
|
|
uint8_t *black_data[2][4]; ///< buffer used to fill padded lines (limited/full)
|
|
int black_linesize[4];
|
|
FFDrawContext draw;
|
|
FFDrawColor color;
|
|
const AVPixFmtDescriptor *csp;
|
|
void (*lowpass_line)(uint8_t *dstp, ptrdiff_t width, const uint8_t *srcp,
|
|
ptrdiff_t mref, ptrdiff_t pref, int clip_max);
|
|
} TInterlaceContext;
|
|
|
|
void ff_tinterlace_init_x86(TInterlaceContext *interlace);
|
|
|
|
#endif /* AVFILTER_TINTERLACE_H */
|