mirror of
https://git.videolan.org/git/ffmpeg.git
synced 2024-08-07 10:04:15 +02:00
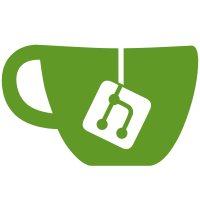
This commit removes the badly duplicated code between the encoder and the muxer. That may sound surprising, but the encoder is now responsible from the encoding of the picture when muxing to a .gif file. It also does not require anymore a manual user intervention such as a -pix_fmt rgb24 to work properly. To summarize, output gif are now easier to generate, code is saner and simpler, and files are smaller (thanks to the lzw encoding which was unused so far with the default .gif output). We can certainly make things even better, but this is the first step. FATE is updated because of the output being produced by the encoder and not the muxer (no lzw in the muxer), and in the seek test only the size mismatches. Fixes Ticket #2262
103 lines
3.5 KiB
C
103 lines
3.5 KiB
C
/*
|
|
* Image format
|
|
* Copyright (c) 2000, 2001, 2002 Fabrice Bellard
|
|
* Copyright (c) 2004 Michael Niedermayer
|
|
*
|
|
* This file is part of FFmpeg.
|
|
*
|
|
* FFmpeg is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* FFmpeg is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with FFmpeg; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
#include "libavutil/avstring.h"
|
|
#include "internal.h"
|
|
|
|
typedef struct {
|
|
enum AVCodecID id;
|
|
const char *str;
|
|
} IdStrMap;
|
|
|
|
static const IdStrMap img_tags[] = {
|
|
{ AV_CODEC_ID_MJPEG, "jpeg" },
|
|
{ AV_CODEC_ID_MJPEG, "jpg" },
|
|
{ AV_CODEC_ID_MJPEG, "jps" },
|
|
{ AV_CODEC_ID_LJPEG, "ljpg" },
|
|
{ AV_CODEC_ID_JPEGLS, "jls" },
|
|
{ AV_CODEC_ID_PNG, "png" },
|
|
{ AV_CODEC_ID_PNG, "pns" },
|
|
{ AV_CODEC_ID_PNG, "mng" },
|
|
{ AV_CODEC_ID_PPM, "ppm" },
|
|
{ AV_CODEC_ID_PPM, "pnm" },
|
|
{ AV_CODEC_ID_PGM, "pgm" },
|
|
{ AV_CODEC_ID_PGMYUV, "pgmyuv" },
|
|
{ AV_CODEC_ID_PBM, "pbm" },
|
|
{ AV_CODEC_ID_PAM, "pam" },
|
|
{ AV_CODEC_ID_MPEG1VIDEO, "mpg1-img" },
|
|
{ AV_CODEC_ID_MPEG2VIDEO, "mpg2-img" },
|
|
{ AV_CODEC_ID_MPEG4, "mpg4-img" },
|
|
{ AV_CODEC_ID_FFV1, "ffv1-img" },
|
|
{ AV_CODEC_ID_RAWVIDEO, "y" },
|
|
{ AV_CODEC_ID_RAWVIDEO, "raw" },
|
|
{ AV_CODEC_ID_BMP, "bmp" },
|
|
{ AV_CODEC_ID_TARGA, "tga" },
|
|
{ AV_CODEC_ID_TIFF, "tiff" },
|
|
{ AV_CODEC_ID_TIFF, "tif" },
|
|
{ AV_CODEC_ID_SGI, "sgi" },
|
|
{ AV_CODEC_ID_PTX, "ptx" },
|
|
{ AV_CODEC_ID_PCX, "pcx" },
|
|
{ AV_CODEC_ID_BRENDER_PIX, "pix" },
|
|
{ AV_CODEC_ID_SUNRAST, "sun" },
|
|
{ AV_CODEC_ID_SUNRAST, "ras" },
|
|
{ AV_CODEC_ID_SUNRAST, "rs" },
|
|
{ AV_CODEC_ID_SUNRAST, "im1" },
|
|
{ AV_CODEC_ID_SUNRAST, "im8" },
|
|
{ AV_CODEC_ID_SUNRAST, "im24" },
|
|
{ AV_CODEC_ID_SUNRAST, "im32" },
|
|
{ AV_CODEC_ID_SUNRAST, "sunras" },
|
|
{ AV_CODEC_ID_JPEG2000, "j2c" },
|
|
{ AV_CODEC_ID_JPEG2000, "jp2" },
|
|
{ AV_CODEC_ID_JPEG2000, "jpc" },
|
|
{ AV_CODEC_ID_JPEG2000, "j2k" },
|
|
{ AV_CODEC_ID_DPX, "dpx" },
|
|
{ AV_CODEC_ID_EXR, "exr" },
|
|
{ AV_CODEC_ID_PICTOR, "pic" },
|
|
{ AV_CODEC_ID_V210X, "yuv10" },
|
|
{ AV_CODEC_ID_WEBP, "webp" },
|
|
{ AV_CODEC_ID_XBM, "xbm" },
|
|
{ AV_CODEC_ID_XFACE, "xface" },
|
|
{ AV_CODEC_ID_XWD, "xwd" },
|
|
{ AV_CODEC_ID_NONE, NULL }
|
|
};
|
|
|
|
static enum AVCodecID av_str2id(const IdStrMap *tags, const char *str)
|
|
{
|
|
str = strrchr(str, '.');
|
|
if (!str)
|
|
return AV_CODEC_ID_NONE;
|
|
str++;
|
|
|
|
while (tags->id) {
|
|
if (!av_strcasecmp(str, tags->str))
|
|
return tags->id;
|
|
|
|
tags++;
|
|
}
|
|
return AV_CODEC_ID_NONE;
|
|
}
|
|
|
|
enum AVCodecID ff_guess_image2_codec(const char *filename)
|
|
{
|
|
return av_str2id(img_tags, filename);
|
|
}
|