mirror of
https://github.com/mpv-player/mpv
synced 2024-09-28 17:52:52 +02:00
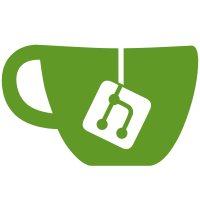
The completion function itself now parses --list-options on the first tab press and caches the results. This does mean a slight delay on that first tab press, but it will only do this if the argument being completed looks like an option (i.e. starts with "-"), so there is never a delay when just completing a file name. I've also put some effort into making it reasonably fast; on my machine it's consistently under 100 ms, more than half of which is mpv itself. Installation of zsh completion is now done unconditionally because it's nothing more than copying a file. If you really don't want it installed, set zshdir to empty: `./waf configure --zshdir= ...` Improvements in functionality compared to the old script: * Produces the right results for mpv binaries other than the one it was installed with (like a dev build for testing changes). * Does not require running mpv at build time, so it won't cause problems with cross compilation. * Handles aliases. * Slightly nicer handling of options that take comma-separated values and/or sub-options: A space is now inserted at the end instead of a comma, allowing you to immediately start typing the next argument, but typing a comma will still remove the automatically added space, and = and : will now do that too, so you can immediately add a sub-option. * More general/flexible handling of values for options that print their possible values with --option=help. The code as is could handle quite a few more options (*scale, demuxers, decoders, ...), but nobody wants to maintain that list here so we'll just stick with what the old completion script already did.
96 lines
3.2 KiB
Python
96 lines
3.2 KiB
Python
from waflib.Build import BuildContext
|
|
from waflib import TaskGen, Utils
|
|
from io import StringIO
|
|
from TOOLS.matroska import generate_C_header, generate_C_definitions
|
|
from TOOLS.file2string import file2string
|
|
import os
|
|
|
|
def __wayland_scanner_cmd__(ctx, mode, dir, src, vendored_file):
|
|
return "${{WAYSCAN}} {0} < {1} > ${{TGT}}".format(
|
|
mode,
|
|
"${SRC}" if vendored_file else "{}/{}".format(dir, src)
|
|
)
|
|
|
|
def __file2string__(ctx, **kwargs):
|
|
ctx(
|
|
rule = __file2string_cmd__(ctx),
|
|
before = ("c",),
|
|
name = os.path.basename(kwargs['target']),
|
|
**kwargs
|
|
)
|
|
|
|
def execf(self, fn):
|
|
setattr(self, 'before', ['c'])
|
|
setattr(self, 'rule', ' ') # waf doesn't print the task with no rule
|
|
target = getattr(self, 'target', None)
|
|
out = self.path.find_or_declare(target)
|
|
tmp = StringIO()
|
|
fn(tmp)
|
|
out.write(tmp.getvalue())
|
|
tmp.close()
|
|
|
|
@TaskGen.feature('file2string')
|
|
def f2s(self):
|
|
def fn(out):
|
|
source = getattr(self, 'source', None)
|
|
src = self.path.find_resource(source)
|
|
file2string(source, iter(src.read().splitlines(True)), out)
|
|
execf(self, fn)
|
|
|
|
@TaskGen.feature('ebml_header')
|
|
def ebml_header(self):
|
|
execf(self, generate_C_header)
|
|
|
|
@TaskGen.feature('ebml_definitions')
|
|
def ebml_definitions(self):
|
|
execf(self, generate_C_definitions)
|
|
|
|
def __wayland_protocol_code__(ctx, **kwargs):
|
|
protocol_is_vendored = kwargs.get("vendored_protocol", False)
|
|
file_name = kwargs['protocol'] + '.xml'
|
|
|
|
if protocol_is_vendored:
|
|
del kwargs['vendored_protocol']
|
|
kwargs['source'] = '{}/{}'.format(kwargs['proto_dir'], file_name)
|
|
|
|
ctx(
|
|
rule = __wayland_scanner_cmd__(ctx, 'private-code', kwargs['proto_dir'],
|
|
file_name,
|
|
protocol_is_vendored),
|
|
name = os.path.basename(kwargs['target']),
|
|
**kwargs
|
|
)
|
|
|
|
def __wayland_protocol_header__(ctx, **kwargs):
|
|
protocol_is_vendored = kwargs.get("vendored_protocol", False)
|
|
file_name = kwargs['protocol'] + '.xml'
|
|
|
|
if protocol_is_vendored:
|
|
del kwargs['vendored_protocol']
|
|
kwargs['source'] = '{}/{}'.format(kwargs['proto_dir'], file_name)
|
|
|
|
ctx(
|
|
rule = __wayland_scanner_cmd__(ctx, 'client-header', kwargs['proto_dir'],
|
|
file_name,
|
|
protocol_is_vendored),
|
|
before = ('c',),
|
|
name = os.path.basename(kwargs['target']),
|
|
**kwargs
|
|
)
|
|
|
|
@TaskGen.feature('cprogram')
|
|
@TaskGen.feature('cshlib')
|
|
@TaskGen.feature('cstlib')
|
|
@TaskGen.feature('apply_link')
|
|
@TaskGen.after_method('process_source', 'process_use', 'apply_link', 'process_uselib_local', 'propagate_uselib_vars', 'do_the_symbol_stuff')
|
|
def handle_add_object(tgen):
|
|
if getattr(tgen, 'add_objects', None):
|
|
for input in tgen.add_objects:
|
|
input_node = tgen.path.find_resource(input)
|
|
if input_node is not None:
|
|
tgen.link_task.inputs.append(input_node)
|
|
|
|
BuildContext.file2string = __file2string__
|
|
BuildContext.wayland_protocol_code = __wayland_protocol_code__
|
|
BuildContext.wayland_protocol_header = __wayland_protocol_header__
|