mirror of
https://github.com/mpv-player/mpv
synced 2024-11-03 03:19:24 +01:00
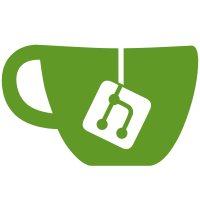
Libav 10 was released, so we can enable testing the stable Libav version again. FFmpeg 2.2 was also released, but since we still support 2.1.4, we stick with the older version. This is better for testing.
101 lines
2.0 KiB
Ruby
Executable File
101 lines
2.0 KiB
Ruby
Executable File
#!/usr/bin/ruby
|
|
|
|
class TravisDepsBuilder
|
|
def self.make(name)
|
|
info = build_map[name]
|
|
raise "unrecognized dependency identifier '#{name}'" unless info
|
|
instance = new(name, info[:url], info[:action])
|
|
instance.deps
|
|
instance.build
|
|
end
|
|
|
|
attr_reader :name, :url, :action
|
|
|
|
def initialize(name, url=nil, action=nil)
|
|
@name, @url, @action = name, url, action
|
|
end
|
|
|
|
def build
|
|
send(@action)
|
|
end
|
|
|
|
def deps; end
|
|
|
|
private
|
|
def git
|
|
sh "git clone --depth=1 #{url} #{name}"
|
|
compile name
|
|
end
|
|
|
|
def stable
|
|
filename = File.basename(url)
|
|
sh "wget #{url}"
|
|
sh "tar -xzvf #{filename}"
|
|
dirname = File.basename(url, ".tar.gz" )
|
|
compile dirname
|
|
end
|
|
|
|
def compile(dirname)
|
|
sh "cd #{dirname} && #{configure} && make && sudo make install"
|
|
sh "cd $TRAVIS_BUILD_DIR"
|
|
end
|
|
|
|
def configure
|
|
"./configure"
|
|
end
|
|
|
|
def sh(command)
|
|
`#{command}`
|
|
end
|
|
end
|
|
|
|
class Libav < TravisDepsBuilder
|
|
def self.build_map
|
|
{
|
|
"libav-stable" => {
|
|
:action => :stable,
|
|
:url => 'http://libav.org/releases/libav-10.tar.gz'
|
|
},
|
|
"libav-git" => {
|
|
:action => :git,
|
|
:url => "git://git.libav.org/libav.git"
|
|
},
|
|
"ffmpeg-stable" => {
|
|
:action => :stable,
|
|
:url => 'http://www.ffmpeg.org/releases/ffmpeg-2.1.4.tar.gz'
|
|
},
|
|
"ffmpeg-git" => {
|
|
:action => :git,
|
|
:url => "git://github.com/FFmpeg/FFmpeg.git"
|
|
}
|
|
}
|
|
end
|
|
|
|
def configure
|
|
[super, "--cc=#{ENV['CC']}"].join(" ")
|
|
end
|
|
end
|
|
|
|
class Libass < TravisDepsBuilder
|
|
def self.build_map
|
|
{
|
|
"libass-stable" => {
|
|
:action => :stable,
|
|
:url => 'http://libass.googlecode.com/files/libass-0.10.1.tar.gz'
|
|
}
|
|
}
|
|
end
|
|
end
|
|
|
|
class Dependencies < TravisDepsBuilder
|
|
def deps
|
|
sh "sudo apt-get update -y"
|
|
sh "sudo apt-get install pkg-config fontconfig libfribidi-dev yasm -y"
|
|
end
|
|
end
|
|
|
|
Dependencies.new(:deps).deps
|
|
|
|
Libass.make(ARGV[0])
|
|
Libav.make(ARGV[1])
|