mirror of
https://github.com/mpv-player/mpv
synced 2024-10-30 04:46:41 +01:00
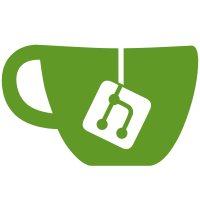
git-svn-id: svn://svn.mplayerhq.hu/mplayer/trunk@15822 b3059339-0415-0410-9bf9-f77b7e298cf2
7842 lines
202 KiB
Bash
Executable File
7842 lines
202 KiB
Bash
Executable File
#! /bin/sh
|
|
#
|
|
# Original version (C) 2000 Pontscho/fresh!mindworkz
|
|
# pontscho@makacs.poliod.hu
|
|
#
|
|
# History / Contributors: check the cvs log !
|
|
#
|
|
# Cleanups all over the place (c) 2001 pl
|
|
#
|
|
#
|
|
# Guidelines:
|
|
# If the option is named 'opt':
|
|
# _opt : should have a value in yes/no/auto
|
|
# _def_opt : '#define ... 1' or '#undef ...' that is: some C code
|
|
# _ld_opt : ' -L/path/dir -lopt ' that is: some GCC option
|
|
# _inc_opt : ' -I/path/dir/include '
|
|
#
|
|
# In this file, a tab is 8 chars and indentation shift is 2 characters
|
|
#
|
|
# GOTCHAS:
|
|
# - config files are currently:
|
|
# config.h config.mak libvo/config.mak libao2/config.mak Gui/config.mak
|
|
#
|
|
#############################################################################
|
|
|
|
# Prevent locale nonsense from breaking basic text processing utils
|
|
LC_ALL=C
|
|
export LC_ALL
|
|
|
|
# Prefer these macros to full length text !
|
|
# These macros only return an error code - NO display is done
|
|
cc_check() {
|
|
echo >> "$TMPLOG"
|
|
cat "$TMPC" >> "$TMPLOG"
|
|
echo >> "$TMPLOG"
|
|
echo "$_cc $CFLAGS $_inc_extra $_ld_static $_ld_extra $TMPC -o $TMPO $@" >> "$TMPLOG"
|
|
rm -f "$TMPO"
|
|
( $_cc $CFLAGS $_inc_extra $_ld_static $_ld_extra "$TMPC" -o "$TMPO" "$@" ) >> "$TMPLOG" 2>&1
|
|
TMP="$?"
|
|
echo >> "$TMPLOG"
|
|
echo "ldd $TMPO" >> "$TMPLOG"
|
|
( $_ldd "$TMPO" ) >> "$TMPLOG" 2>&1
|
|
echo >> "$TMPLOG"
|
|
return "$TMP"
|
|
}
|
|
|
|
# Display error message, flushes tempfile, exit
|
|
die () {
|
|
echo
|
|
echo "Error: $@" >&2
|
|
echo >&2
|
|
rm -f "$TMPO" "$TMPC" "$TMPS" "$TMPCPP"
|
|
echo "Check \"$TMPLOG\" if you do not understand why it failed."
|
|
exit 1
|
|
}
|
|
|
|
# OS test booleans functions
|
|
issystem() {
|
|
test "`echo $system_name | tr A-Z a-z`" = "`echo $1 | tr A-Z a-z`"
|
|
}
|
|
linux() { issystem "Linux" ; return "$?" ; }
|
|
sunos() { issystem "SunOS" ; return "$?" ; }
|
|
hpux() { issystem "HP-UX" ; return "$?" ; }
|
|
irix() { issystem "IRIX" ; return "$?" ; }
|
|
cygwin() { issystem "CYGWIN" ; return "$?" ; }
|
|
freebsd() { issystem "FreeBSD" ; return "$?" ; }
|
|
netbsd() { issystem "NetBSD" ; return "$?" ; }
|
|
bsdos() { issystem "BSD/OS" ; return "$?" ; }
|
|
openbsd() { issystem "OpenBSD" ; return "$?" ; }
|
|
bsd() { freebsd || netbsd || bsdos || openbsd ; return "$?" ; }
|
|
qnx() { issystem "QNX" ; return "$?" ; }
|
|
darwin() { issystem "Darwin" ; return "$?" ; }
|
|
gnu() { issystem "GNU" ; return "$?" ; }
|
|
mingw32() { issystem "MINGW32" ; return "$?" ; }
|
|
morphos() { issystem "MorphOS" ; return "$?" ; }
|
|
win32() { cygwin || mingw32 ; return "$?" ; }
|
|
beos() { issystem "BEOS" ; return "$?" ; }
|
|
|
|
# arch test boolean functions
|
|
# x86/x86pc is used by QNX
|
|
x86() {
|
|
case "$host_arch" in
|
|
i[3-9]86|x86|x86pc|k5|k6|k6-2|k6-3|pentium*|athlon*|i586-i686) return 0 ;;
|
|
*) return 1 ;;
|
|
esac
|
|
}
|
|
|
|
ppc() {
|
|
case "$host_arch" in
|
|
ppc) return 0;;
|
|
*) return 1;;
|
|
esac
|
|
}
|
|
|
|
alpha() {
|
|
case "$host_arch" in
|
|
alpha) return 0;;
|
|
*) return 1;;
|
|
esac
|
|
}
|
|
|
|
# not boolean test: implement the posix shell "!" operator for a
|
|
# non-posix /bin/sh.
|
|
# usage: not {command}
|
|
# returns exit status "success" when the execution of "command"
|
|
# fails.
|
|
not() {
|
|
eval "$@"
|
|
test $? -ne 0
|
|
}
|
|
|
|
# Use this before starting a check
|
|
echocheck() {
|
|
echo "============ Checking for $@ ============" >> "$TMPLOG"
|
|
echo ${_echo_n} "Checking for $@ ... ${_echo_c}"
|
|
}
|
|
|
|
# Use this to echo the results of a check
|
|
echores() {
|
|
echo "Result is: $@" >> "$TMPLOG"
|
|
echo "##########################################" >> "$TMPLOG"
|
|
echo "" >> "$TMPLOG"
|
|
echo "$@"
|
|
}
|
|
#############################################################################
|
|
|
|
# Check how echo works in this /bin/sh
|
|
case `echo -n` in
|
|
-n) _echo_n= _echo_c='\c' ;; # SysV echo
|
|
*) _echo_n='-n ' _echo_c= ;; # BSD echo
|
|
esac
|
|
|
|
LANGUAGES=`echo help/help_mp-??.h help/help_mp-??_??.h | sed "s:help/help_mp-\(..\).h:\1:g" | sed "s:help/help_mp-\(.....\).h:\1:g"`
|
|
|
|
for parm in "$@" ; do
|
|
if test "$parm" = "--help" || test "$parm" = "-help" || test "$parm" = "-h" ; then
|
|
cat << EOF
|
|
|
|
Usage: $0 [OPTIONS]...
|
|
|
|
Configuration:
|
|
-h, --help display this help and exit
|
|
|
|
Installation directories:
|
|
--prefix=DIR use this prefix for installing mplayer [/usr/local]
|
|
--bindir=DIR use this prefix for installing mplayer binary
|
|
[PREFIX/bin]
|
|
--datadir=DIR use this prefix for installing machine independent
|
|
data files (fonts, skins) [PREFIX/share/mplayer]
|
|
--mandir=DIR use this prefix for installing manpages [PREFIX/man]
|
|
--confdir=DIR use this prefix for installing configuration files
|
|
[PREFIX/etc/mplayer]
|
|
--libdir=DIR use this prefix for object code libraries [PREFIX/lib]
|
|
|
|
Optional features:
|
|
--disable-mencoder disable mencoder (a/v encoder) compilation [enable]
|
|
--enable-gui enable gmplayer compilation (GTK 1.2 GUI) [disable]
|
|
--enable-largefiles enable support for files > 2 GBytes [disable]
|
|
--enable-linux-devfs set default devices to devfs ones [disable]
|
|
--enable-termcap use termcap database for key codes [autodetect]
|
|
--enable-termios use termios database for key codes [autodetect]
|
|
--disable-iconv do not use iconv(3) function [autodetect]
|
|
--disable-setlocale disable setlocale using in mplayer [autodetect]
|
|
--disable-langinfo do not use langinfo [autodetect]
|
|
--enable-lirc enable LIRC (remote control) support [autodetect]
|
|
--enable-lircc enable LIRCCD (LIRC client daemon) input [autodetect]
|
|
--enable-joystick enable joystick support [disable]
|
|
--disable-vm disable support X video mode extensions [autodetect]
|
|
--disable-xf86keysym disable support for 'multimedia' keys [autodetect]
|
|
--disable-tv disable TV Interface (tv/dvb grabbers) [enable]
|
|
--disable-tv-v4l disable Video4Linux TV Interface support [autodetect]
|
|
--disable-tv-v4l2 disable Video4Linux2 TV Interface support [autodetect]
|
|
--disable-tv-bsdbt848 disable BSD BT848 Interface support [autodetect]
|
|
--disable-edl disable EDL (edit decision list) support [enable]
|
|
--disable-rtc disable RTC (/dev/rtc) on Linux [autodetect]
|
|
--disable-network disable network support (for: http/mms/rtp) [enable]
|
|
--enable-winsock2 enable winsock2 usage [autodetect]
|
|
--enable-smb enable Samba (SMB) input support [autodetect]
|
|
--enable-live enable LIVE.COM Streaming Media support [autodetect]
|
|
--disable-dvdread Disable libdvdread support [autodetect]
|
|
--disable-mpdvdkit Disable mpdvdkit/mpdvdkit2 support [autodetect]
|
|
--disable-cdparanoia Disable cdparanoia support [autodetect]
|
|
--disable-freetype Disable freetype2 font rendering support [autodetect]
|
|
--disable-fontconfig Disable fontconfig font lookup support [autodetect]
|
|
--disable-unrarlib Disable Unique RAR File Library [enabled]
|
|
--enable-menu Enable OSD menu support (NOT DVD MENU) [disabled]
|
|
--disable-sortsub Disable subtitles sorting [enabled]
|
|
--enable-fribidi Enable using the FriBiDi libs [disabled]
|
|
--disable-enca Disable using ENCA charset oracle library [autodetect]
|
|
--disable-macosx Disable Mac OS X specific features [autodetect]
|
|
--enable-macosx-finder-support Enable Mac OS X Finder invocation parameter parsing [disabled]
|
|
--enable-macosx-bundle Enable Mac OS X bundle file locations [autodetect]
|
|
--disable-inet6 Disable IPv6 support [autodetect]
|
|
--disable-gethostbyname2 gethostbyname() function is not provided by the C
|
|
library [autodetect]
|
|
--disable-ftp Disable ftp support [enabled]
|
|
--disable-vstream Disable tivo vstream client support [autodetect]
|
|
--disable-pthreads Disable Posix threads support [autodetect]
|
|
|
|
Codecs:
|
|
--enable-gif enable gif support [autodetect]
|
|
--enable-png enable png input/output support [autodetect]
|
|
--enable-jpeg enable jpeg input/output support [autodetect]
|
|
--enable-liblzo enable external liblzo support [autodetect]
|
|
--disable-win32 disable Win32 DLL support [autodetect]
|
|
--disable-dshow disable Win32/DirectShow support [autodetect]
|
|
--disable-qtx disable Quicktime codecs [autodetect]
|
|
--disable-xanim disable XAnim DLL support [autodetect]
|
|
--disable-real disable RealPlayer DLL support [autodetect]
|
|
--disable-xvid disable XviD codec [autodetect]
|
|
--disable-x264 disable H.264 encoder [autodetect]
|
|
--disable-divx4linux disable DivX4linux/Divx5linux codec [autodetect]
|
|
--enable-opendivx enable _old_ OpenDivx codec [disable]
|
|
--disable-libavcodec disable libavcodec [autodetect]
|
|
--disable-libavformat disable libavformat [autodetect]
|
|
--enable-libfame enable libfame realtime encoder [autodetect]
|
|
--disable-internal-tremor do not build internal OggVorbis support [enabled]
|
|
--enable-tremor-low build with lower accuracy internal tremor [disabled]
|
|
--enable-external-tremor build with external tremor [disabled]
|
|
--disable-vorbis disable OggVorbis support entirely [autodetect]
|
|
--enable-theora build with OggTheora support [autodetect]
|
|
--disable-internal-matroska disable internal Matroska support [enabled]
|
|
--enable-external-faad build with external FAAD2 (AAC) support [autodetect]
|
|
--disable-internal-faad disable internal FAAD2 (AAC) support [autodetect]
|
|
--disable-faac disable support for FAAC (AAC encoder) [autodetect]
|
|
--disable-ladspa disable LADSPA plugin support [autodetect]
|
|
--disable-libdv disable libdv 0.9.5 en/decoding support [autodetect]
|
|
--disable-mad disable libmad (MPEG audio) support [autodetect]
|
|
--disable-toolame disable Toolame (MPEG layer 2 audio) support in mencoder [autodetect]
|
|
--disable-twolame disable Twolame (MPEG layer 2 audio) support in mencoder [autodetect]
|
|
--enable-xmms build with XMMS inputplugin support [disabled]
|
|
--disable-mp3lib disable builtin mp3lib [enabled]
|
|
--disable-liba52 disable builtin liba52 [enabled]
|
|
--enable-libdts enable libdts support [autodetect]
|
|
--disable-libmpeg2 disable builtin libmpeg2 [enabled]
|
|
--disable-amr_nb disable amr narrowband, floating point [autodetect]
|
|
--disable-amr_nb-fixed disable amr narrowband, fixed point [autodetect]
|
|
--disable-amr_wb disable amr wideband, floating point [autodetect]
|
|
--disable-codec=CODEC disable specified codec
|
|
--enable-codec=CODEC dnable specified codec
|
|
|
|
Video output:
|
|
--disable-vidix disable VIDIX [enable on x86 *nix]
|
|
--enable-gl build with OpenGL render support [autodetect]
|
|
--enable-dga[=n] build with DGA [n in {1, 2} ] support [autodetect]
|
|
--enable-vesa build with VESA support [autodetect]
|
|
--enable-svga build with SVGAlib support [autodetect]
|
|
--enable-sdl build with SDL render support [autodetect]
|
|
--enable-aa build with AAlib render support [autodetect]
|
|
--enable-caca build with CACA render support [autodetect]
|
|
--enable-ggi build with GGI render support [autodetect]
|
|
--enable-ggiwmh build with GGI libggiwmh extension [autodetect]
|
|
--enable-directx build with DirectX support [autodetect]
|
|
--enable-dxr2 build with DXR2 render support [autodetect]
|
|
--enable-dxr3 build with DXR3/H+ render support [autodetect]
|
|
--enable-dvb build with support for output via DVB-Card [autodetect]
|
|
--enable-dvbhead build with DVB support (HEAD version) [autodetect]
|
|
--enable-mga build with mga_vid (for Matrox G200/G4x0/G550) support
|
|
(check for /dev/mga_vid) [autodetect]
|
|
--enable-xmga build with mga_vid X Window support
|
|
(check for X & /dev/mga_vid) [autodetect]
|
|
--enable-xv build with Xv render support for X 4.x [autodetect]
|
|
--enable-xvmc build with XvMC acceleration for X 4.x [disable]
|
|
--enable-vm build with XF86VidMode support for X11 [autodetect]
|
|
--enable-xinerama build with Xinerama support for X11 [autodetect]
|
|
--enable-x11 build with X11 render support [autodetect]
|
|
--enable-fbdev build with FBDev render support [autodetect]
|
|
--enable-mlib build with MLIB support (Solaris only) [autodetect]
|
|
--enable-3dfx build with obsolete /dev/3dfx support [disable]
|
|
--enable-tdfxfb build with tdfxfb (Voodoo 3/banshee) support [disable]
|
|
--enable-directfb build with DirectFB support [autodetect]
|
|
--enable-zr build with ZR360[56]7/ZR36060 support [autodetect]
|
|
--enable-bl build with Blinkenlights support [disable]
|
|
--enable-tdfxvid build with tdfx_vid support [disable]
|
|
--disable-tga disable targa output support [enable]
|
|
--disable-pnm disable pnm output support [enable]
|
|
--disable-md5sum disable md5sum output support [enable]
|
|
|
|
Audio output:
|
|
--disable-alsa disable ALSA sound support [autodetect]
|
|
--disable-ossaudio disable OSS sound support [autodetect]
|
|
--disable-arts disable aRts sound support [autodetect]
|
|
--disable-esd disable esd sound support [autodetect]
|
|
--disable-polyp disable Polypaudio sound support [autodetect]
|
|
--disable-jack disable JACK sound support [autodetect]
|
|
--disable-nas disable NAS sound support [autodetect]
|
|
--disable-sgiaudio disable SGI sound support [autodetect]
|
|
--disable-sunaudio disable Sun sound support [autodetect]
|
|
--disable-win32waveout disable Windows waveout sound support [autodetect]
|
|
--disable-select disable using select() on audio device [enable]
|
|
|
|
Miscellaneous options:
|
|
--enable-runtime-cpudetection Enable runtime CPU detection [disable]
|
|
--cc=COMPILER use this C compiler to build MPlayer [gcc]
|
|
--host-cc=COMPILER use this C compiler to build apps needed for the build process [gcc]
|
|
--as=ASSEMBLER use this assembler to build MPlayer [as]
|
|
--target=PLATFORM target platform (i386-linux, arm-linux, etc)
|
|
--enable-static build a statically linked binary. Set further linking
|
|
options with --enable-static="-lslang -lncurses"
|
|
--charset convert the help messages to this charset
|
|
--language=list a white space or comma separated list of languages
|
|
for translated man pages, the first language is the
|
|
primary and therefore used for translated messages
|
|
and GUI (also the environment variable \$LINGUAS is
|
|
honored) [en]
|
|
(Available: $LANGUAGES all)
|
|
--enable-shared-pp install & use shared postprocessing lib
|
|
--with-install=PATH use a custom install program (useful if your OS uses
|
|
a GNU-incompatible install utility by default and
|
|
you want to use GNU version)
|
|
--install-path=PATH the path to a custom install program
|
|
this option is obsolete and will be removed soon,
|
|
use --with-install instead.
|
|
|
|
Advanced options:
|
|
--enable-mmx build with MMX support [autodetect]
|
|
--enable-mmx2 build with MMX2 support (PIII, Athlon) [autodetect]
|
|
--enable-3dnow build with 3DNow! support [autodetect]
|
|
--enable-3dnowex build with extended 3DNow! support [autodetect]
|
|
--enable-sse build with SSE support [autodetect]
|
|
--enable-sse2 build with SSE2 support [autodetect]
|
|
--enable-shm build with shm support [autodetect]
|
|
--enable-altivec build with Altivec support (PowerPC) [autodetect]
|
|
--disable-fastmemcpy disable 3DNow!/SSE/MMX optimized memcpy() [enable]
|
|
--enable-big-endian Force byte order to big-endian [autodetect]
|
|
--enable-debug[=1-3] compile debugging information into mplayer [disable]
|
|
--enable-profile compile profiling information into mplayer [disable]
|
|
--disable-sighandler disable sighandler for crashes [enable]
|
|
--enable-crash-debug enable automatic gdb attach on crash [disable]
|
|
--enable-i18n _experimental_ gnu gettext() support [autodetect]
|
|
--enable-dynamic-plugins Enable support for dynamic a/v plugins [disable]
|
|
|
|
Hazardous options a.k.a. "DO NOT REPORT ANY BUGS!"
|
|
--disable-gcc-checking disable gcc version checking [enable]
|
|
|
|
Use these options if autodetection fails (Options marked with (*) accept
|
|
multiple paths separated by ':'):
|
|
--with-extraincdir=DIR extra headers (png, mad, sdl, ...) in DIR (*)
|
|
--with-extralibdir=DIR extra library files (png, mad, sdl, ...) in DIR (*)
|
|
--with-x11incdir=DIR X headers in DIR (*)
|
|
--with-x11libdir=DIR X library files in DIR (*)
|
|
--with-dxr2incdir=DIR DXR2 headers in DIR (*)
|
|
--with-dvbincdir=DIR DVB headers in DIR (*)
|
|
--with-madlibdir=DIR libmad (libmad shared library) in DIR (*)
|
|
--with-mlibdir=DIR libmlib (MLIB support) in DIR (Solaris only)
|
|
--with-codecsdir=DIR Binary codec files in DIR
|
|
--with-win32libdir=DIR W*ndows DLL files in DIR
|
|
--with-xanimlibdir=DIR XAnim DLL files in DIR
|
|
--with-reallibdir=DIR RealPlayer DLL files in DIR
|
|
--with-xvidlibdir=DIR libxvidcore (XviD) in DIR (*)
|
|
--with-xvidincdir=DIR XviD header in DIR (*)
|
|
--with-x264libdir=DIR libx264 in DIR
|
|
--with-x264incdir=DIR x264 header in DIR
|
|
--with-dtslibdir=DIR libdts library in DIR (*)
|
|
--with-dtsincdir=DIR libdts header in DIR (*)
|
|
--with-livelibdir=DIR LIVE.COM Streaming Media libraries in DIR
|
|
--with-toolamedir=DIR path to Toolame library and include file
|
|
--with-xmmsplugindir=DIR XMMS plugins in DIR
|
|
--with-xmmslibdir=DIR libxmms.so.1 in DIR
|
|
--with-cdparanoiaincdir=DIR cdparanoia headers in DIR (*)
|
|
--with-cdparanoialibdir=DIR cdparanoia libraries (libcdda_*) in DIR (*)
|
|
--with-xvmclib=NAME name of adapter-specific library (e.g. XvMCNVIDIA)
|
|
--with-termcaplib=NAME name of library with termcap functionality
|
|
name should be given without leading "lib"
|
|
checks for "termcap" and "tinfo"
|
|
|
|
--with-freetype-config=PATH path to freetype-config
|
|
(e.g. /opt/bin/freetype-config)
|
|
--with-fribidi-config=PATH path to fribidi-config
|
|
(e.g. /opt/bin/fribidi-config)
|
|
--with-glib-config=PATH path to glib*-config (e.g. /opt/bin/glib-config)
|
|
--with-gtk-config=PATH path to gtk*-config (e.g. /opt/bin/gtk-config)
|
|
--with-sdl-config=PATH path to sdl*-config (e.g. /opt/bin/sdl-config)
|
|
|
|
This configure script is NOT autoconf-based, even though its output is similar.
|
|
It will try to autodetect all configuration options. If you --enable an option
|
|
it will be forcefully turned on, skipping autodetection. This can break
|
|
compilation, so you need to know what you are doing.
|
|
EOF
|
|
exit 0
|
|
fi
|
|
done # for parm in ...
|
|
|
|
|
|
# 1st pass checking for vital options
|
|
_install=install
|
|
_ranlib=ranlib
|
|
_cc=cc
|
|
test "$CC" && _cc="$CC"
|
|
_as=auto
|
|
_runtime_cpudetection=no
|
|
for ac_option do
|
|
case "$ac_option" in
|
|
--target=*)
|
|
_target=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--cc=*)
|
|
_cc=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--host-cc=*)
|
|
_host_cc=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--as=*)
|
|
_as=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--enable-gcc-checking)
|
|
_skip_cc_check=no
|
|
;;
|
|
--disable-gcc-checking)
|
|
_skip_cc_check=yes
|
|
;;
|
|
--enable-static)
|
|
_ld_static='-static'
|
|
;;
|
|
--disable-static)
|
|
_ld_static=''
|
|
;;
|
|
--enable-static=*)
|
|
_ld_static="-static `echo $ac_option | cut -d '=' -f 2`"
|
|
;;
|
|
--with-extraincdir=*)
|
|
_inc_extra=-I`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -I,g'`
|
|
;;
|
|
--with-extralibdir=*)
|
|
_ld_extra=-L`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -L,g'`
|
|
;;
|
|
--enable-runtime-cpudetection)
|
|
_runtime_cpudetection=yes
|
|
;;
|
|
--disable-runtime-cpudetection)
|
|
_runtime_cpudetection=no
|
|
;;
|
|
--install-path=*)
|
|
_install=`echo $ac_option | cut -d '=' -f 2 | sed 's/\/$//'`"/install"
|
|
;;
|
|
--with-install=*)
|
|
_install=`echo $ac_option | cut -d '=' -f 2 `
|
|
;;
|
|
esac
|
|
done
|
|
|
|
# Determine our OS name and CPU architecture
|
|
if test -z "$_target" ; then
|
|
# OS name
|
|
system_name=`( uname -s ) 2>&1`
|
|
case "$system_name" in
|
|
Linux|FreeBSD|NetBSD|BSD/OS|OpenBSD|SunOS|QNX|Darwin|GNU|BeOS)
|
|
;;
|
|
IRIX*)
|
|
system_name=IRIX
|
|
;;
|
|
HP-UX*)
|
|
system_name=HP-UX
|
|
;;
|
|
[cC][yY][gG][wW][iI][nN]*)
|
|
system_name=CYGWIN
|
|
;;
|
|
MINGW32*)
|
|
system_name=MINGW32
|
|
;;
|
|
MorphOS)
|
|
system_name=MorphOS
|
|
;;
|
|
*)
|
|
system_name="$system_name-UNKNOWN"
|
|
;;
|
|
esac
|
|
|
|
|
|
# host's CPU/instruction set
|
|
host_arch=`( uname -p ) 2>&1`
|
|
case "$host_arch" in
|
|
i386|sparc|ppc|alpha|arm|mips|vax)
|
|
;;
|
|
powerpc) # Darwin returns 'powerpc'
|
|
host_arch=ppc
|
|
;;
|
|
*) # uname -p on Linux returns 'unknown' for the processor type,
|
|
# OpenBSD returns 'Intel Pentium/MMX ("Genuine Intel" 586-class)'
|
|
|
|
# Maybe uname -m (machine hardware name) returns something we
|
|
# recognize.
|
|
|
|
# x86/x86pc is used by QNX
|
|
case "`( uname -m ) 2>&1`" in
|
|
i[3-9]86*|x86|x86pc|k5|k6|k6_2|k6_3|k6-2|k6-3|pentium*|athlon*|i586_i686|i586-i686|BePC) host_arch=i386 ;;
|
|
ia64) host_arch=ia64 ;;
|
|
x86_64|amd64)
|
|
if [ "`$_cc -dumpmachine | grep x86_64 | cut -d- -f1`" = "x86_64" -a \
|
|
-z "`echo $CFLAGS | grep -- -m32`" ]; then
|
|
host_arch=x86_64
|
|
else
|
|
host_arch=i386
|
|
fi
|
|
;;
|
|
macppc|ppc) host_arch=ppc ;;
|
|
alpha) host_arch=alpha ;;
|
|
sparc) host_arch=sparc ;;
|
|
sparc64) host_arch=sparc64 ;;
|
|
parisc*|hppa*|9000*) host_arch=hppa ;;
|
|
arm*) host_arch=arm ;;
|
|
s390) host_arch=s390 ;;
|
|
s390x) host_arch=s390x ;;
|
|
mips) host_arch=mips ;;
|
|
vax) host_arch=vax ;;
|
|
*) host_arch=UNKNOWN ;;
|
|
esac
|
|
;;
|
|
esac
|
|
else
|
|
system_name=`echo $_target | cut -d '-' -f 2`
|
|
case "`echo $system_name | tr A-Z a-z`" in
|
|
linux) system_name=Linux ;;
|
|
freebsd) system_name=FreeBSD ;;
|
|
netbsd) system_name=NetBSD ;;
|
|
bsd/os) system_name=BSD/OS ;;
|
|
openbsd) system_name=OpenBSD ;;
|
|
sunos) system_name=SunOS ;;
|
|
qnx) system_name=QNX ;;
|
|
morphos) system_name=MorphOS ;;
|
|
mingw32msvc) system_name=MINGW32 ;;
|
|
esac
|
|
# We need to convert underscores so that values like k6-2 and pentium-mmx can be passed
|
|
host_arch=`echo $_target | cut -d '-' -f 1 | tr '_' '-'`
|
|
fi
|
|
|
|
echo "Detected operating system: $system_name"
|
|
echo "Detected host architecture: $host_arch"
|
|
|
|
# LGB: temporary files
|
|
for I in "$TMPDIR" "$TEMPDIR" "/tmp" ; do
|
|
test "$I" && break
|
|
done
|
|
|
|
TMPLOG="configure.log"
|
|
rm -f "$TMPLOG"
|
|
TMPC="$I/mplayer-conf-$RANDOM-$$.c"
|
|
TMPCPP="$I/mplayer-conf-$RANDOM-$$.cpp"
|
|
TMPO="$I/mplayer-conf-$RANDOM-$$.o"
|
|
TMPS="$I/mplayer-conf-$RANDOM-$$.S"
|
|
|
|
# config files
|
|
|
|
# FIXME: A lot of stuff is installed under /usr/local
|
|
# NK: But we should never use this stuff implicitly since we call compiler
|
|
# from /usr we should be sure that there no effects from other compilers
|
|
# (libraries) which might be installed into /usr/local. Let users use this
|
|
# stuff explicitly as command line argument. In other words: It would be
|
|
# resonable to have only /usr/include or only /usr/local/include.
|
|
|
|
if freebsd ; then
|
|
_ld_extra="$_ld_extra -L/usr/local/lib"
|
|
_inc_extra="$_inc_extra -I/usr/local/include"
|
|
fi
|
|
|
|
if netbsd ; then
|
|
for I in `echo $_ld_extra | sed 's/-L//g'` ; do
|
|
tmp="$tmp ` echo $I | sed 's/.*/ -L& -Wl,-R&/'`"
|
|
done
|
|
_ld_extra=$tmp
|
|
fi
|
|
|
|
_ldd=ldd
|
|
if darwin; then
|
|
_ldd="otool -L"
|
|
fi
|
|
|
|
# Check how to call 'head' and 'tail'. Newer versions spit out warnings
|
|
# if used as 'head -1' instead of 'head -n 1', but older versions don't
|
|
# know about '-n'.
|
|
if test "`(echo line1 ; echo line2) | head -1 2>/dev/null`" = "line1" ; then
|
|
_head() { head -$1 2>/dev/null ; }
|
|
else
|
|
_head() { head -n $1 2>/dev/null ; }
|
|
fi
|
|
if test "`(echo line1 ; echo line2) | tail -1 2>/dev/null`" = "line2" ; then
|
|
_tail() { tail -$1 2>/dev/null ; }
|
|
else
|
|
_tail() { tail -n $1 2>/dev/null ; }
|
|
fi
|
|
|
|
# Checking CC version...
|
|
if test "$_skip_cc_check" != yes ; then
|
|
# Intel C++ Compilers (no autoselect, use CC=/some/binary ./configure)
|
|
if test "`basename $_cc`" = "icc" || test "`basename $_cc`" = "ecc"; then
|
|
echocheck "$_cc version"
|
|
cc_vendor=intel
|
|
cc_name=`( $_cc -V ) 2>&1 | _head 1 | cut -d ',' -f 1`
|
|
cc_version=`( $_cc -V ) 2>&1 | _head 1 | cut -d ',' -f 2 | cut -d ' ' -f 3`
|
|
_cc_major=`echo $cc_version | cut -d '.' -f 1`
|
|
_cc_minor=`echo $cc_version | cut -d '.' -f 2`
|
|
# TODO verify older icc/ecc compatibility
|
|
case $cc_version in
|
|
'')
|
|
cc_version="v. ?.??, bad"
|
|
cc_verc_fail=yes
|
|
;;
|
|
8.0)
|
|
cc_version="$cc_version, ok"
|
|
cc_verc_fail=no
|
|
;;
|
|
*)
|
|
cc_version="$cc_version, bad"
|
|
cc_verc_fail=yes
|
|
;;
|
|
esac
|
|
echores "$cc_version"
|
|
else
|
|
for _cc in "$_cc" gcc gcc-3.4 gcc-3.3 gcc-3.2 gcc-3.1 gcc3 gcc-3.0 cc ; do
|
|
echocheck "$_cc version"
|
|
cc_vendor=gnu
|
|
cc_name=`( $_cc -v ) 2>&1 | _tail 1 | cut -d ' ' -f 1`
|
|
cc_version=`( $_cc -dumpversion ) 2>&1`
|
|
if test "$?" -gt 0; then
|
|
cc_version="not found"
|
|
fi
|
|
case $cc_version in
|
|
'')
|
|
cc_version="v. ?.??, bad"
|
|
cc_verc_fail=yes
|
|
;;
|
|
2.95.[2-9]|2.95.[2-9][-.]*|[3-4].*)
|
|
_cc_major=`echo $cc_version | cut -d '.' -f 1`
|
|
_cc_minor=`echo $cc_version | cut -d '.' -f 2`
|
|
_cc_mini=`echo $cc_version | cut -d '.' -f 3`
|
|
cc_version="$cc_version, ok"
|
|
cc_verc_fail=no
|
|
;;
|
|
'not found')
|
|
cc_verc_fail=yes
|
|
;;
|
|
*)
|
|
cc_version="$cc_version, bad"
|
|
cc_verc_fail=yes
|
|
;;
|
|
esac
|
|
echores "$cc_version"
|
|
(test "$cc_verc_fail" = "no") && break
|
|
done
|
|
fi # icc
|
|
if test "$cc_verc_fail" = yes ; then
|
|
cat <<EOF
|
|
|
|
*** Please downgrade/upgrade C compiler to version gcc-2.95.x or gcc-3.x! ***
|
|
|
|
You are not using a supported compiler. We do not have the time to make sure
|
|
everything works with compilers other than the ones we use. Use either the
|
|
same compiler as we do, or use --disable-gcc-checking but DO *NOT* REPORT BUGS
|
|
unless you can reproduce them after recompiling with a 2.95.x or 3.x version!
|
|
|
|
Note for gcc 2.96 users: Some versions of this compiler are known to miscompile
|
|
mplayer and lame (which is used for mencoder). If you get compile errors,
|
|
first upgrade to the latest 2.96 release (minimum 2.96-85) and try again.
|
|
If the problem still exists, try with gcc 3.x (or 2.95.x) *BEFORE* reporting
|
|
bugs!
|
|
|
|
GCC 2.96 IS NOT AND WILL NOT BE SUPPORTED BY US !
|
|
|
|
*** For details please read DOCS/HTML/en/users-vs-dev.html ***
|
|
|
|
EOF
|
|
die "Bad gcc version"
|
|
fi
|
|
else
|
|
cat <<EOF
|
|
|
|
******************************************************************************
|
|
|
|
Hmm. You really want to compile MPlayer with an *UNSUPPORTED* C compiler?
|
|
Ok. You know. Do it. Did you read DOCS/HTML/en/users-vs-dev.html???
|
|
|
|
DO NOT SEND BUGREPORTS OR COMPLAIN, it's *YOUR* compiler's fault!
|
|
Get ready for mysterious crashes, no-picture bugs, strange noises... REALLY!
|
|
Lame which is used by mencoder produces weird errors, too.
|
|
|
|
If you have any problem, install a GCC 2.95.x or 3.x version and try again.
|
|
If the problem _still_ exists, then read DOCS/HTML/en/bugreports.html !
|
|
|
|
*** DO NOT SEND BUG REPORTS OR COMPLAIN it's *YOUR* compiler's fault! ***
|
|
|
|
******************************************************************************
|
|
|
|
EOF
|
|
|
|
read _answer
|
|
|
|
fi
|
|
echocheck "host cc"
|
|
if not test "$_host_cc" ; then
|
|
_host_cc=$_cc
|
|
fi
|
|
echores $_host_cc
|
|
|
|
|
|
# ---
|
|
|
|
# now that we know what compiler should be used for compilation, try to find
|
|
# out which assembler is used by the $_cc compiler
|
|
if test "$_as" = auto ; then
|
|
_as=`$_cc -print-prog-name=as`
|
|
test -z "$_as" && _as=as
|
|
fi
|
|
|
|
# XXX: this should be ok..
|
|
_cpuinfo="echo"
|
|
# Cygwin has /proc/cpuinfo, but only supports Intel CPUs
|
|
# FIXME: Remove the cygwin check once AMD CPUs are supported
|
|
if test -r /proc/cpuinfo && not cygwin; then
|
|
# Linux with /proc mounted, extract CPU information from it
|
|
_cpuinfo="cat /proc/cpuinfo"
|
|
elif test -r /compat/linux/proc/cpuinfo && not x86 ; then
|
|
# FreeBSD with Linux emulation /proc mounted,
|
|
# extract CPU information from it
|
|
_cpuinfo="cat /compat/linux/proc/cpuinfo"
|
|
elif darwin ; then
|
|
# use hostinfo on Darwin
|
|
_cpuinfo="hostinfo"
|
|
elif x86; then
|
|
# all other OSes try to extract CPU information from a small helper
|
|
# program TOOLS/cpuinfo instead
|
|
$_cc -o TOOLS/cpuinfo TOOLS/cpuinfo.c
|
|
_cpuinfo="TOOLS/cpuinfo"
|
|
fi
|
|
|
|
x86_exts_check()
|
|
{
|
|
pparam=`$_cpuinfo | grep 'features' | cut -d ':' -f 2 | _head 1`
|
|
if test -z "$pparam" ; then
|
|
pparam=`$_cpuinfo | grep 'flags' | cut -d ':' -f 2 | _head 1`
|
|
fi
|
|
|
|
_mmx=no
|
|
_3dnow=no
|
|
_3dnowex=no
|
|
_mmx2=no
|
|
_sse=no
|
|
_sse2=no
|
|
_mtrr=no
|
|
|
|
for i in $pparam ; do
|
|
case "$i" in
|
|
3dnow) _3dnow=yes ;;
|
|
3dnowext) _3dnow=yes _3dnowex=yes ;;
|
|
mmx) _mmx=yes ;;
|
|
mmxext) _mmx2=yes ;;
|
|
mtrr|k6_mtrr|cyrix_arr) _mtrr=yes ;;
|
|
xmm|sse|kni) _sse=yes _mmx2=yes ;;
|
|
sse2) _sse2=yes ;;
|
|
esac
|
|
done
|
|
}
|
|
|
|
case "$host_arch" in
|
|
i[3-9]86|x86|x86pc|k5|k6|k6-2|k6-3|pentium*|athlon*|i586-i686)
|
|
_def_arch="#define ARCH_X86 1"
|
|
_target_arch="TARGET_ARCH_X86 = yes"
|
|
|
|
pname=`$_cpuinfo | grep 'model name' | cut -d ':' -f 2 | _head 1`
|
|
pvendor=`$_cpuinfo | grep 'vendor_id' | cut -d ':' -f 2 | cut -d ' ' -f 2 | _head 1`
|
|
pfamily=`$_cpuinfo | grep 'cpu family' | cut -d ':' -f 2 | cut -d ' ' -f 2 | _head 1`
|
|
pmodel=`$_cpuinfo | grep -v 'model name' | grep 'model' | cut -d ':' -f 2 | cut -d ' ' -f 2 | _head 1`
|
|
pstepping=`$_cpuinfo | grep 'stepping' | cut -d ':' -f 2 | cut -d ' ' -f 2 | _head 1`
|
|
|
|
x86_exts_check
|
|
|
|
echocheck "CPU vendor"
|
|
echores "$pvendor ($pfamily:$pmodel:$pstepping)"
|
|
|
|
echocheck "CPU type"
|
|
echores "$pname"
|
|
|
|
case "$pvendor" in
|
|
AuthenticAMD)
|
|
case "$pfamily" in
|
|
3) proc=i386 iproc=386 ;;
|
|
4) proc=i486 iproc=486 ;;
|
|
5) iproc=586 # LGB: models are: K5/SSA5 K5 K5 K5 ? ? K6 K6 K6-2 K6-3
|
|
# K6 model 13 are the K6-2+ and K6-III+, only differing in cache size.
|
|
if test "$pmodel" -eq 9 -o "$pmodel" -eq 13; then
|
|
proc=k6-3
|
|
elif test "$pmodel" -ge 8; then
|
|
proc=k6-2
|
|
elif test "$pmodel" -ge 6; then
|
|
proc=k6
|
|
else
|
|
proc=i586
|
|
iproc=586
|
|
fi
|
|
;;
|
|
6) iproc=686
|
|
if test "$pmodel" -ge 7; then
|
|
proc=athlon-4
|
|
elif test "$pmodel" -ge 6; then
|
|
# only Athlon XP supports ssem MP, Duron etc not
|
|
# but most of them are CPUID 666, so check if sse detected
|
|
# btw. there is also athlon-mp opt, but we need extended
|
|
# CPUID to detect if CPU is SMP capable -> athlon-mp ::atmos
|
|
if test "$_sse" = yes && test "$pstepping" -ge 2; then
|
|
proc=athlon-xp
|
|
else
|
|
proc=athlon-4
|
|
fi
|
|
elif test "$pmodel" -ge 4; then
|
|
proc=athlon-tbird
|
|
else
|
|
proc=athlon # TODO: should the Duron Spitfire be considered a Thunderbird instead?
|
|
fi
|
|
;;
|
|
15) iproc=686
|
|
# k8 cpu-type only supported in gcc >= 3.4.0, but that will be
|
|
# caught and remedied in the optimization tests below.
|
|
proc=k8
|
|
;;
|
|
|
|
*) proc=athlon-xp iproc=686 ;;
|
|
esac
|
|
;;
|
|
GenuineIntel)
|
|
case "$pfamily" in
|
|
3) proc=i386 iproc=386 ;;
|
|
4) proc=i486 iproc=486 ;;
|
|
5) iproc=586
|
|
if test "$pmodel" -eq 4 || test "$pmodel" -eq 8; then
|
|
proc=pentium-mmx # 4 is desktop, 8 is mobile
|
|
else
|
|
proc=i586
|
|
fi
|
|
;;
|
|
6) iproc=686
|
|
if test "$pmodel" -ge 7; then
|
|
proc=pentium3
|
|
elif test "$pmodel" -ge 3; then
|
|
proc=pentium2
|
|
else
|
|
proc=i686
|
|
fi
|
|
;;
|
|
15) proc=pentium4 iproc=686 ;;
|
|
*) proc=pentium4 iproc=686 ;;
|
|
esac
|
|
;;
|
|
unknown)
|
|
case "$pfamily" in
|
|
3) proc=i386 iproc=386 ;;
|
|
4) proc=i486 iproc=486 ;;
|
|
*) proc=i586 iproc=586 ;;
|
|
esac
|
|
;;
|
|
*)
|
|
proc=i586 iproc=586 ;;
|
|
esac
|
|
|
|
# check that gcc supports our CPU, if not, fall back to earlier ones
|
|
# LGB: check -mcpu and -march swithing step by step with enabling
|
|
# to fall back till 386.
|
|
|
|
# gcc >= 3.4.0 doesn't support -mcpu, we have to use -mtune instead
|
|
|
|
if [ "$cc_vendor" = "gnu" ] && ([ "$_cc_major" -gt 3 ] || ( [ "$_cc_major" = 3 ] && [ "$_cc_minor" -ge 4 ])) ; then
|
|
cpuopt=-mtune
|
|
else
|
|
cpuopt=-mcpu
|
|
fi
|
|
|
|
echocheck "GCC & CPU optimization abilities"
|
|
cat > $TMPC << EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if test "$_runtime_cpudetection" = no ; then
|
|
if test "$proc" = "k8" -o "$proc" = "opteron" -o "$proc" = "athlon64" -o "$proc" = "athlon-fx" ; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=athlon-xp
|
|
fi
|
|
if test "$proc" = "athlon-xp" || test "$proc" = "athlon-4" || test "$proc" = "athlon-tbird"; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=athlon
|
|
fi
|
|
if test "$proc" = "k6-3" || test "$proc" = "k6-2"; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=k6
|
|
fi
|
|
if test "$proc" = "k6"; then
|
|
if not cc_check -march=$proc $cpuopt=$proc; then
|
|
if cc_check -march=i586 $cpuopt=i686; then
|
|
proc=i586-i686
|
|
else
|
|
proc=i586
|
|
fi
|
|
fi
|
|
fi
|
|
if test "$proc" = "pentium4" || test "$proc" = "pentium3" || test "$proc" = "pentium2" || test "$proc" = "athlon"; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=i686
|
|
fi
|
|
if test "$proc" = "i686" || test "$proc" = "pentium-mmx"; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=i586
|
|
fi
|
|
if test "$proc" = "i586" ; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=i486
|
|
fi
|
|
if test "$proc" = "i486" ; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=i386
|
|
fi
|
|
if test "$proc" = "i386" ; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=error
|
|
fi
|
|
if test "$proc" = "error" ; then
|
|
echores "Your $_cc does not even support \"i386\" for '-march' and '$cpuopt'."
|
|
_mcpu=""
|
|
_march=""
|
|
_optimizing=""
|
|
elif test "$proc" = "i586-i686"; then
|
|
_march="-march=i586"
|
|
_mcpu="$cpuopt=i686"
|
|
_optimizing="$proc"
|
|
else
|
|
_march="-march=$proc"
|
|
_mcpu="$cpuopt=$proc"
|
|
_optimizing="$proc"
|
|
fi
|
|
else
|
|
# i686 is probably the most common CPU - optimize for it
|
|
_mcpu="$cpuopt=i686"
|
|
# at least i486 required, for bswap instruction
|
|
_march="-march=i486"
|
|
cc_check $_mcpu || _mcpu=""
|
|
cc_check $_march $_mcpu || _march=""
|
|
fi
|
|
|
|
## Gabucino : --target takes effect here (hopefully...) by overwriting
|
|
## autodetected mcpu/march parameters
|
|
if test "$_target" ; then
|
|
# TODO: it may be a good idea to check GCC and fall back in all cases
|
|
if test "$host_arch" = "i586-i686"; then
|
|
_march="-march=i586"
|
|
_mcpu="$cpuopt=i686"
|
|
else
|
|
_march="-march=$host_arch"
|
|
_mcpu="$cpuopt=$host_arch"
|
|
fi
|
|
|
|
proc="$host_arch"
|
|
|
|
case "$proc" in
|
|
i386) iproc=386 ;;
|
|
i486) iproc=486 ;;
|
|
i586|k5|k6|k6-2|k6-3|pentium|pentium-mmx) iproc=586 ;;
|
|
i686|athlon*|pentium*) iproc=686 ;;
|
|
*) iproc=586 ;;
|
|
esac
|
|
fi
|
|
|
|
echores "$proc"
|
|
;;
|
|
|
|
ia64)
|
|
_def_arch='#define ARCH_IA64 1'
|
|
_target_arch='TARGET_ARCH_IA64 = yes'
|
|
iproc='ia64'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
;;
|
|
|
|
x86_64|amd64)
|
|
_def_arch='#define ARCH_X86_64 1'
|
|
_target_arch='TARGET_ARCH_X86_64 = yes'
|
|
iproc='x86_64'
|
|
|
|
# gcc >= 3.4.0 doesn't support -mcpu, we have to use -mtune instead
|
|
if test "$cc_vendor" = "gnu" && test "$_cc_major" -gt 3 -o "$_cc_major" -eq 3 -a "$_cc_minor" -ge 4 ; then
|
|
cpuopt=-mtune
|
|
else
|
|
cpuopt=-mcpu
|
|
fi
|
|
# k8 cpu-type only supported in gcc >= 3.4.0, but that will be
|
|
# caught and remedied in the optimization tests below.
|
|
proc=k8
|
|
|
|
echocheck "GCC & CPU optimization abilities"
|
|
cat > $TMPC << EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
# This is a stripped-down version of the i386 fallback.
|
|
if test "$_runtime_cpudetection" = no ; then
|
|
if test "$proc" = "k8" -o "$proc" = "opteron" -o "$proc" = "athlon64" -o "$proc" = "athlon-fx" ; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=athlon-xp
|
|
fi
|
|
# This will fail if gcc version < 3.3, which is ok because earlier
|
|
# versions don't really support 64-bit on amd64.
|
|
# Is this a valid assumption? -Corey
|
|
if test "$proc" = "athlon-xp" || test "$proc" = "athlon-4" ; then
|
|
cc_check -march=$proc $cpuopt=$proc || proc=error
|
|
fi
|
|
_march="-march=$proc"
|
|
_mcpu="$cpuopt=$proc"
|
|
if test "$proc" = "error" ; then
|
|
echores "Your $_cc does not even support \"athlon-xp\" for '-march' and '$cpuopt'."
|
|
_mcpu=""
|
|
_march=""
|
|
fi
|
|
else
|
|
_march=""
|
|
_mcpu=""
|
|
fi
|
|
|
|
_optimizing=""
|
|
|
|
echores "$proc"
|
|
|
|
x86_exts_check
|
|
;;
|
|
|
|
sparc)
|
|
_def_arch='#define ARCH_SPARC 1'
|
|
_target_arch='TARGET_ARCH_SPARC = yes'
|
|
iproc='sparc'
|
|
if sunos ; then
|
|
echocheck "CPU type"
|
|
karch=`uname -m`
|
|
case "`echo $karch`" in
|
|
sun4) proc=v7 ;;
|
|
sun4c) proc=v7 ;;
|
|
sun4d) proc=v8 ;;
|
|
sun4m) proc=v8 ;;
|
|
sun4u) proc=v9 _vis='yes' _def_vis='#define HAVE_VIS = yes' ;;
|
|
*) ;;
|
|
esac
|
|
echores "$proc"
|
|
else
|
|
proc=v8
|
|
fi
|
|
_march=''
|
|
_mcpu="-mcpu=$proc"
|
|
_optimizing="$proc"
|
|
;;
|
|
|
|
sparc64)
|
|
_def_arch='#define ARCH_SPARC 1'
|
|
_target_arch='TARGET_ARCH_SPARC = yes'
|
|
_vis='yes'
|
|
_def_vis='#define HAVE_VIS = yes'
|
|
iproc='sparc'
|
|
proc='v9'
|
|
_march=''
|
|
_mcpu="-mcpu=$proc"
|
|
_optimizing="$proc"
|
|
;;
|
|
|
|
arm|armv4l|armv5tel)
|
|
_def_arch='#define ARCH_ARMV4L 1'
|
|
_target_arch='TARGET_ARCH_ARMV4L = yes'
|
|
iproc='arm'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
;;
|
|
|
|
ppc)
|
|
_def_arch='#define ARCH_POWERPC 1'
|
|
_def_dcbzl='#define NO_DCBZL 1'
|
|
_target_arch='TARGET_ARCH_POWERPC = yes'
|
|
iproc='ppc'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
_altivec=no
|
|
|
|
echocheck "CPU type"
|
|
if linux && test -n "$_cpuinfo"; then
|
|
proc=`$_cpuinfo | grep 'cpu' | cut -d ':' -f 2 | cut -d ',' -f 1 | cut -b 2- | _head 1`
|
|
if test -n "`$_cpuinfo | grep altivec`"; then
|
|
_altivec=yes
|
|
fi
|
|
fi
|
|
if darwin ; then
|
|
proc=`$_cpuinfo | grep "Processor type" | cut -f 3 -d ' ' | sed 's/ppc//'`
|
|
if [ `sysctl -n hw.vectorunit` -eq 1 ]; then
|
|
_altivec=yes
|
|
elif [ "`sysctl -n hw.optional.altivec 2>/dev/null`" = 1 ]; then
|
|
_altivec=yes
|
|
fi
|
|
fi
|
|
# only gcc 3.4 works reliably with altivec code under netbsd
|
|
if netbsd ; then
|
|
case $cc_version in
|
|
2*|3.0*|3.1*|3.2*|3.3*)
|
|
;;
|
|
*)
|
|
if [ `sysctl -n machdep.altivec` -eq 1 ]; then
|
|
_altivec=yes
|
|
fi
|
|
;;
|
|
esac
|
|
fi
|
|
if test "$_altivec" = yes; then
|
|
echores "$proc altivec"
|
|
else
|
|
echores "$proc"
|
|
fi
|
|
|
|
echocheck "GCC & CPU optimization abilities"
|
|
|
|
if test -n "$proc"; then
|
|
case "$proc" in
|
|
601) _march='-mcpu=601' _mcpu='-mtune=601' ;;
|
|
603) _march='-mcpu=603' _mcpu='-mtune=603' ;;
|
|
603e|603ev) _march='-mcpu=603e' _mcpu='-mtune=603e' ;;
|
|
604|604e|604r|604ev) _march='-mcpu=604' _mcpu='-mtune=604' ;;
|
|
740|740/750|745/755) _march='-mcpu=740' _mcpu='-mtune=740' ;;
|
|
750|750CX) _march='-mcpu=750' _mcpu='-mtune=750' ;;
|
|
*) ;;
|
|
esac
|
|
# gcc 3.1(.1) and up supports 7400 and 7450
|
|
if test "$_cc_major" -ge "3" && test "$_cc_minor" -ge "1" || test "$_cc_major" -ge "4"; then
|
|
case "$proc" in
|
|
7400*|7410*) _march='-mcpu=7400' _mcpu='-mtune=7400' ;;
|
|
7450*|7455*) _march='-mcpu=7450' _mcpu='-mtune=7450' ;;
|
|
*) ;;
|
|
esac
|
|
fi
|
|
# gcc 3.2 and up supports 970
|
|
if test "$_cc_major" -ge "3" && test "$_cc_minor" -ge "3" || test "$_cc_major" -ge "4"; then
|
|
case "$proc" in
|
|
970*) _march='-mcpu=970' _mcpu='-mtune=970'
|
|
_def_dcbzl='#undef NO_DCBZL' ;;
|
|
*) ;;
|
|
esac
|
|
fi
|
|
fi
|
|
|
|
if test -n "$_mcpu"; then
|
|
_optimizing=`echo $_mcpu | cut -c 8-`
|
|
echores "$_optimizing"
|
|
else
|
|
echores "none"
|
|
fi
|
|
|
|
;;
|
|
|
|
alpha)
|
|
_def_arch='#define ARCH_ALPHA 1'
|
|
_target_arch='TARGET_ARCH_ALPHA = yes'
|
|
iproc='alpha'
|
|
_march=''
|
|
|
|
echocheck "CPU type"
|
|
cat > $TMPC << EOF
|
|
int main() {
|
|
unsigned long ver, mask;
|
|
asm ("implver %0" : "=r" (ver));
|
|
asm ("amask %1, %0" : "=r" (mask) : "r" (-1));
|
|
printf("%ld-%x\n", ver, ~mask);
|
|
return 0;
|
|
}
|
|
EOF
|
|
$_cc -o "$TMPO" "$TMPC"
|
|
case `"$TMPO"` in
|
|
|
|
0-0) proc="ev4"; cpu_understands_mvi="0";;
|
|
1-0) proc="ev5"; cpu_understands_mvi="0";;
|
|
1-1) proc="ev56"; cpu_understands_mvi="0";;
|
|
1-101) proc="pca56"; cpu_understands_mvi="1";;
|
|
2-303) proc="ev6"; cpu_understands_mvi="1";;
|
|
2-307) proc="ev67"; cpu_understands_mvi="1";;
|
|
2-1307) proc="ev68"; cpu_understands_mvi="1";;
|
|
esac
|
|
echores "$proc"
|
|
|
|
echocheck "GCC & CPU optimization abilities"
|
|
if test "$proc" = "ev68" ; then
|
|
cc_check -mcpu=$proc || proc=ev67
|
|
fi
|
|
if test "$proc" = "ev67" ; then
|
|
cc_check -mcpu=$proc || proc=ev6
|
|
fi
|
|
_mcpu="-mcpu=$proc"
|
|
echores "$proc"
|
|
|
|
_optimizing="$proc"
|
|
|
|
echocheck "MVI instruction support in GCC"
|
|
if test "$_cc_major" -ge "3" && test "$cpu_understands_mvi" = "1" ; then
|
|
_def_gcc_mvi_support="#define CAN_COMPILE_ALPHA_MVI 1"
|
|
echores "yes"
|
|
else
|
|
_def_gcc_mvi_support="#undef CAN_COMPILE_ALPHA_MVI"
|
|
echores "no, GCC = `( $_cc -dumpversion ) 2>&1` (must be >= 3), CPU = $proc (must be pca56 or later)"
|
|
fi
|
|
;;
|
|
|
|
mips)
|
|
_def_arch='#define ARCH_SGI_MIPS 1'
|
|
_target_arch='TARGET_ARCH_SGI_MIPS = yes'
|
|
iproc='sgi-mips'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
|
|
if irix ; then
|
|
echocheck "CPU type"
|
|
proc=`hinv -c processor | grep CPU | cut -d " " -f3`
|
|
case "`echo $proc`" in
|
|
R3000) _march='-mips1' _mcpu='-mtune=r2000' ;;
|
|
R4000) _march='-mips3' _mcpu='-mtune=r4000' ;;
|
|
R4400) _march='-mips3' _mcpu='-mtune=r4400' ;;
|
|
R4600) _march='-mips3' _mcpu='-mtune=r4600' ;;
|
|
R5000) _march='-mips4' _mcpu='-mtune=r5000' ;;
|
|
R8000|R10000|R12000|R14000|R16000) _march='-mips4' _mcpu='-mtune=r8000' ;;
|
|
esac
|
|
echores "$proc"
|
|
fi
|
|
|
|
;;
|
|
|
|
hppa)
|
|
_def_arch='#define ARCH_PA_RISC 1'
|
|
_target_arch='TARGET_ARCH_PA_RISC = yes'
|
|
iproc='PA-RISC'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
;;
|
|
|
|
s390)
|
|
_def_arch='#define ARCH_S390 1'
|
|
_target_arch='TARGET_ARCH_S390 = yes'
|
|
iproc='390'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
;;
|
|
|
|
s390x)
|
|
_def_arch='#define ARCH_S390X 1'
|
|
_target_arch='TARGET_ARCH_S390X = yes'
|
|
iproc='390x'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
;;
|
|
|
|
vax)
|
|
_def_arch='#define ARCH_VAX 1'
|
|
_target_arch='TARGET_ARCH_VAX = yes'
|
|
iproc='vax'
|
|
proc=''
|
|
_march=''
|
|
_mcpu=''
|
|
_optimizing=''
|
|
;;
|
|
|
|
*)
|
|
echo "The architecture of your CPU ($host_arch) is not supported by this configure script"
|
|
echo "It seems nobody has ported MPlayer to your OS or CPU type yet."
|
|
die "unsupported architecture $host_arch"
|
|
;;
|
|
esac
|
|
|
|
if test "$_runtime_cpudetection" = yes ; then
|
|
if x86; then
|
|
_mmx=yes
|
|
_3dnow=yes
|
|
_3dnowex=yes
|
|
_mmx2=yes
|
|
_sse=yes
|
|
_sse2=yes
|
|
_mtrr=yes
|
|
fi
|
|
if ppc; then
|
|
_altivec=yes
|
|
fi
|
|
fi
|
|
|
|
if x86 && test "$_runtime_cpudetection" = no ; then
|
|
extcheck() {
|
|
if test "$1" = yes ; then
|
|
echocheck "kernel support of $2"
|
|
cat > $TMPC <<EOF
|
|
#include <signal.h>
|
|
void catch() { exit(1); }
|
|
int main(void){
|
|
signal(SIGILL, catch);
|
|
__asm__ __volatile__ ("$3":::"memory");return(0);
|
|
}
|
|
EOF
|
|
|
|
if ( cc_check && $TMPO ) > /dev/null 2>&1 ; then
|
|
echores "yes"
|
|
_optimizing="$_optimizing $2"
|
|
return 0
|
|
else
|
|
echores "failed"
|
|
echo "It seems that your kernel does not correctly support $2."
|
|
echo "To use $2 extensions in MPlayer, you have to upgrade/recompile your kernel!"
|
|
return 1
|
|
fi
|
|
fi
|
|
return 0
|
|
}
|
|
|
|
extcheck $_mmx "mmx" "emms" || _mmx=no
|
|
extcheck $_mmx2 "mmx2" "sfence" || _mmx2=no
|
|
extcheck $_3dnow "3dnow" "femms" || _3dnow=no
|
|
extcheck $_3dnowex "3dnowex" "pswapd %%mm0, %%mm0" || _3dnowex=no
|
|
extcheck $_sse "sse" "xorps %%xmm0, %%xmm0" || _sse=no _gcc3_ext="$_gcc3_ext -mno-sse"
|
|
extcheck $_sse2 "sse2" "xorpd %%xmm0, %%xmm0" || _sse2=no _gcc3_ext="$_gcc3_ext -mno-sse2"
|
|
echocheck "mtrr support"
|
|
echores "$_mtrr"
|
|
|
|
if test "$_mtrr" = yes ; then
|
|
_optimizing="$_optimizing mtrr"
|
|
fi
|
|
|
|
if test "$_gcc3_ext" != ""; then
|
|
# if we had to disable sse/sse2 because the active kernel does not
|
|
# support this instruction set extension, we also have to tell
|
|
# gcc3 to not generate sse/sse2 instructions for normal C code
|
|
cat > $TMPC << EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check $_march $_gcc3_ext && _march="$_march $_gcc3_ext"
|
|
fi
|
|
|
|
fi
|
|
|
|
echocheck "assembler support of -pipe option"
|
|
cat > $TMPC << EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check -pipe && _pipe="-pipe" && echores "yes" || echores "no"
|
|
|
|
_prefix="/usr/local"
|
|
_xvmclib="XvMCNVIDIA"
|
|
|
|
# GOTCHA: the variables below defines the default behavior for autodetection
|
|
# and have - unless stated otherwise - at least 2 states : yes no
|
|
# If autodetection is available then the third state is: auto
|
|
_libavcodec=auto
|
|
_amr_nb=auto
|
|
_amr_nb_fixed=auto
|
|
_amr_wb=auto
|
|
_libavcodecs=`grep 'register_avcodec(&[a-z]' libavcodec/allcodecs.c | sed 's/.*&\(.*\)).*/\1/'`
|
|
_libavcodecso=auto
|
|
_libavformat=auto
|
|
_fame=auto
|
|
_mp1e=no
|
|
_mencoder=yes
|
|
_x11=auto
|
|
_dga=auto # 1 2 no auto
|
|
_xv=auto
|
|
_xvmc=no #auto when complete
|
|
_sdl=auto
|
|
_directx=auto
|
|
_win32waveout=auto
|
|
_nas=auto
|
|
_png=auto
|
|
_jpg=auto
|
|
_pnm=yes
|
|
_md5sum=yes
|
|
_gif=auto
|
|
_gl=auto
|
|
_ggi=auto
|
|
_ggiwmh=auto
|
|
_aa=auto
|
|
_caca=auto
|
|
_svga=auto
|
|
_vesa=auto
|
|
_fbdev=auto
|
|
_dvb=auto
|
|
_dvbhead=auto
|
|
_dxr2=auto
|
|
_dxr3=auto
|
|
_iconv=auto
|
|
_langinfo=auto
|
|
_rtc=auto
|
|
_ossaudio=auto
|
|
_arts=auto
|
|
_esd=auto
|
|
_polyp=auto
|
|
_jack=auto
|
|
_liblzo=auto
|
|
_mad=auto
|
|
_toolame=auto
|
|
_twolame=auto
|
|
_tremor_internal=yes
|
|
_tremor_low=no
|
|
_vorbis=auto
|
|
_theora=auto
|
|
_mp3lib=yes
|
|
_liba52=yes
|
|
_libdts=auto
|
|
_libmpeg2=yes
|
|
_matroska_internal=yes
|
|
_tremor=no
|
|
_faad_internal=auto
|
|
_faad_external=auto
|
|
_faac=auto
|
|
_ladspa=auto
|
|
_xmms=no
|
|
# dvdnav disabled, it does not work
|
|
#_dvdnav=no
|
|
_dvdread=auto
|
|
_dvdkit=auto
|
|
_xanim=auto
|
|
_real=auto
|
|
_live=auto
|
|
_xinerama=auto
|
|
_mga=auto
|
|
_xmga=auto
|
|
_vm=auto
|
|
_xf86keysym=auto
|
|
_mlib=auto
|
|
_sgiaudio=auto
|
|
_sunaudio=auto
|
|
_alsa=auto
|
|
_fastmemcpy=yes
|
|
_unrarlib=yes
|
|
_win32=auto
|
|
_dshow=yes
|
|
_select=yes
|
|
_tv=yes
|
|
_tv_v4l=auto
|
|
_tv_v4l2=auto
|
|
_tv_bsdbt848=auto
|
|
_edl=yes
|
|
_network=yes
|
|
_winsock2=auto
|
|
_smbsupport=auto
|
|
_vidix=auto
|
|
_joystick=no
|
|
_xvid=auto
|
|
_x264=auto
|
|
_divx4linux=auto
|
|
_opendivx=no
|
|
_lirc=auto
|
|
_lircc=auto
|
|
_gui=no
|
|
_termcap=auto
|
|
_termios=auto
|
|
_3dfx=no
|
|
_tdfxfb=no
|
|
_tdfxvid=no
|
|
_tga=yes
|
|
_directfb=auto
|
|
_zr=auto
|
|
_bl=no
|
|
_largefiles=no
|
|
#_language=en
|
|
_shm=auto
|
|
_linux_devfs=no
|
|
#_charset=utf8
|
|
_i18n=auto
|
|
_dynamic_plugins=no
|
|
_crash_debug=auto
|
|
_sighandler=yes
|
|
_libdv=auto
|
|
_cdparanoia=auto
|
|
_big_endian=auto
|
|
_freetype=auto
|
|
_fontconfig=auto
|
|
_shared_pp=no
|
|
_menu=no
|
|
_qtx=auto
|
|
_macosx=auto
|
|
_macosx_finder_support=no
|
|
_macosx_bundle=auto
|
|
_sortsub=yes
|
|
_freetypeconfig='freetype-config'
|
|
_fribidi=no
|
|
_fribidiconfig='fribidi-config'
|
|
_enca=auto
|
|
_inet6=auto
|
|
_gethostbyname2=auto
|
|
_ftp=yes
|
|
_vstream=auto
|
|
_pthreads=yes
|
|
for ac_option do
|
|
case "$ac_option" in
|
|
# Skip 1st pass
|
|
--target=*) ;;
|
|
--cc=*) ;;
|
|
--host-cc=*) ;;
|
|
--as=*) ;;
|
|
--enable-gcc-checking) ;;
|
|
--disable-gcc-checking) ;;
|
|
--enable-static*) ;;
|
|
--disable-static*) ;;
|
|
--with-extraincdir=*) ;;
|
|
--with-extralibdir=*) ;;
|
|
--enable-runtime-cpudetection) ;;
|
|
--disable-runtime-cpudetection) ;;
|
|
--install-path=*) ;;
|
|
--with-install=*) ;;
|
|
|
|
# Real 2nd pass
|
|
--enable-mencoder) _mencoder=yes ;;
|
|
--disable-mencoder) _mencoder=no ;;
|
|
--enable-i18n) _i18n=yes ;;
|
|
--disable-i18n) _i18n=no ;;
|
|
--enable-dynamic-plugins) _dynamic_plugins=yes ;;
|
|
--disable-dynamic-plugins) _dynamic_plugins=no ;;
|
|
--enable-x11) _x11=yes ;;
|
|
--disable-x11) _x11=no ;;
|
|
--enable-xv) _xv=yes ;;
|
|
--disable-xv) _xv=no ;;
|
|
--enable-xvmc) _xvmc=yes ;;
|
|
--disable-xvmc) _xvmc=no ;;
|
|
--enable-sdl) _sdl=yes ;;
|
|
--disable-sdl) _sdl=no ;;
|
|
--enable-directx) _directx=yes ;;
|
|
--disable-directx) _directx=no ;;
|
|
--enable-win32waveout) _win32waveout=yes ;;
|
|
--disable-win32waveout) _win32waveout=no ;;
|
|
--enable-nas) _nas=yes ;;
|
|
--disable-nas) _nas=no ;;
|
|
--enable-png) _png=yes ;;
|
|
--disable-png) _png=no ;;
|
|
--enable-jpeg) _jpg=yes ;;
|
|
--disable-jpeg) _jpg=no ;;
|
|
--enable-pnm) _pnm=yes ;;
|
|
--disable-pnm) _pnm=no ;;
|
|
--enable-md5sum) _md5sum=yes ;;
|
|
--disable-md5sum) _md5sum=no ;;
|
|
--enable-gif) _gif=yes ;;
|
|
--disable-gif) _gif=no ;;
|
|
--enable-gl) _gl=yes ;;
|
|
--disable-gl) _gl=no ;;
|
|
--enable-ggi) _ggi=yes ;;
|
|
--disable-ggi) _ggi=no ;;
|
|
--enable-ggiwmh) _ggiwmh=yes ;;
|
|
--disable-ggiwmh) _ggiwmh=no ;;
|
|
--enable-aa) _aa=yes ;;
|
|
--disable-aa) _aa=no ;;
|
|
--enable-caca) _caca=yes ;;
|
|
--disable-caca) _caca=no ;;
|
|
--enable-svga) _svga=yes ;;
|
|
--disable-svga) _svga=no ;;
|
|
--enable-vesa) _vesa=yes ;;
|
|
--disable-vesa) _vesa=no ;;
|
|
--enable-fbdev) _fbdev=yes ;;
|
|
--disable-fbdev) _fbdev=no ;;
|
|
--enable-dvb) _dvb=yes ;;
|
|
--disable-dvb) _dvb=no ;;
|
|
--enable-dvbhead) _dvbhead=yes ;;
|
|
--disable-dvbhead) _dvbhead=no ;;
|
|
--enable-dxr2) _dxr2=yes ;;
|
|
--disable-dxr2) _dxr2=no ;;
|
|
--enable-dxr3) _dxr3=yes ;;
|
|
--disable-dxr3) _dxr3=no ;;
|
|
--enable-iconv) _iconv=yes ;;
|
|
--disable-iconv) _iconv=no ;;
|
|
--enable-langinfo) _langinfo=yes ;;
|
|
--disable-langinfo) _langinfo=no ;;
|
|
--enable-rtc) _rtc=yes ;;
|
|
--disable-rtc) _rtc=no ;;
|
|
--enable-mp1e) _mp1e=yes ;;
|
|
--disable-mp1e) _mp1e=no ;;
|
|
--enable-libdv) _libdv=yes ;;
|
|
--disable-libdv) _libdv=no ;;
|
|
--enable-ossaudio) _ossaudio=yes ;;
|
|
--disable-ossaudio) _ossaudio=no ;;
|
|
--enable-arts) _arts=yes ;;
|
|
--disable-arts) _arts=no ;;
|
|
--enable-esd) _esd=yes ;;
|
|
--disable-esd) _esd=no ;;
|
|
--enable-polyp) _polyp=yes ;;
|
|
--disable-polyp) _polyp=no ;;
|
|
--enable-jack) _jack=yes ;;
|
|
--disable-jack) _jack=no ;;
|
|
--enable-mad) _mad=yes ;;
|
|
--disable-mad) _mad=no ;;
|
|
--disable-toolame) _toolame=no ;;
|
|
--disable-twolame) _twolame=no ;;
|
|
--enable-liblzo) _liblzo=yes ;;
|
|
--disable-liblzo) _liblzo=no ;;
|
|
--enable-vorbis) _vorbis=yes ;;
|
|
--disable-vorbis) _vorbis=no ;;
|
|
--enable-internal-tremor) _tremor_internal=yes ;;
|
|
--disable-internal-tremor) _tremor_internal=no ;;
|
|
--enable-tremor-low) _tremor_low=yes ;;
|
|
--disable-tremor-low) _tremor_low=no ;;
|
|
--enable-external-tremor) _tremor=yes ;;
|
|
--disable-external-tremor) _tremor=no ;;
|
|
--enable-theora) _theora=yes ;;
|
|
--disable-theora) _theora=no ;;
|
|
--enable-mp3lib) _mp3lib=yes ;;
|
|
--disable-mp3lib) _mp3lib=no ;;
|
|
--enable-liba52) _liba52=yes ;;
|
|
--disable-liba52) _liba52=no ;;
|
|
--enable-libdts) _libdts=yes ;;
|
|
--disable-libdts) _libdts=no ;;
|
|
--enable-libmpeg2) _libmpeg2=yes ;;
|
|
--disable-libmpeg2) _libmpeg2=no ;;
|
|
--enable-internal-matroska) _matroska_internal=yes ;;
|
|
--disable-internal-matroska) _matroska_internal=no ;;
|
|
--enable-internal-faad) _faad_internal=yes _faad_external=no ;;
|
|
--disable-internal-faad) _faad_internal=no ;;
|
|
--enable-external-faad) _faad_external=yes _faad_internal=no ;;
|
|
--disable-external-faad) _faad_external=no ;;
|
|
--enable-faac) _faac=yes ;;
|
|
--disable-faac) _faac=no ;;
|
|
--enable-ladspa) _ladspa=yes ;;
|
|
--disable-ladspa) _ladspa=no ;;
|
|
--enable-xmms) _xmms=yes ;;
|
|
--disable-xmms) _xmms=no ;;
|
|
--enable-dvdread) _dvdread=yes ;;
|
|
--disable-dvdread) _dvdread=no ;;
|
|
--enable-mpdvdkit) _dvdkit=yes ;;
|
|
--disable-mpdvdkit) _dvdkit=no ;;
|
|
# dvdnav disabled, it does not work
|
|
# --enable-dvdnav) _dvdnav=yes ;;
|
|
# --disable-dvdnav) _dvdnav=no ;;
|
|
--enable-xanim) _xanim=yes ;;
|
|
--disable-xanim) _xanim=no ;;
|
|
--enable-real) _real=yes ;;
|
|
--disable-real) _real=no ;;
|
|
--enable-live) _live=yes ;;
|
|
--disable-live) _live=no ;;
|
|
--enable-xinerama) _xinerama=yes ;;
|
|
--disable-xinerama) _xinerama=no ;;
|
|
--enable-mga) _mga=yes ;;
|
|
--disable-mga) _mga=no ;;
|
|
--enable-xmga) _xmga=yes ;;
|
|
--disable-xmga) _xmga=no ;;
|
|
--enable-vm) _vm=yes ;;
|
|
--disable-vm) _vm=no ;;
|
|
--enable-xf86keysym) _xf86keysym=yes ;;
|
|
--disable-xf86keysym) _xf86keysym=no ;;
|
|
--enable-mlib) _mlib=yes ;;
|
|
--disable-mlib) _mlib=no ;;
|
|
--enable-sunaudio) _sunaudio=yes ;;
|
|
--disable-sunaudio) _sunaudio=no ;;
|
|
--enable-sgiaudio) _sgiaudio=yes ;;
|
|
--disable-sgiaudio) _sgiaudio=no ;;
|
|
--enable-alsa) _alsa=yes ;;
|
|
--disable-alsa) _alsa=no ;;
|
|
--enable-tv) _tv=yes ;;
|
|
--disable-tv) _tv=no ;;
|
|
--enable-edl) _edl=yes ;;
|
|
--disable-edl) _edl=no ;;
|
|
--enable-tv-bsdbt848) _tv_bsdbt848=yes ;;
|
|
--disable-tv-bsdbt848) _tv_bsdbt848=no ;;
|
|
--enable-tv-v4l) _tv_v4l=yes ;;
|
|
--disable-tv-v4l) _tv_v4l=no ;;
|
|
--enable-tv-v4l2) _tv_v4l2=yes ;;
|
|
--disable-tv-v4l2) _tv_v4l2=no ;;
|
|
--enable-fastmemcpy) _fastmemcpy=yes ;;
|
|
--disable-fastmemcpy) _fastmemcpy=no ;;
|
|
--enable-network) _network=yes ;;
|
|
--disable-network) _network=no ;;
|
|
--enable-winsock2) _winsock2=yes ;;
|
|
--disable-winsock2) _winsock2=no ;;
|
|
--enable-smb) _smbsupport=yes ;;
|
|
--disable-smb) _smbsupport=no ;;
|
|
--enable-vidix) _vidix=yes ;;
|
|
--disable-vidix) _vidix=no ;;
|
|
--enable-joystick) _joystick=yes ;;
|
|
--disable-joystick) _joystick=no ;;
|
|
--enable-xvid) _xvid=yes ;;
|
|
--disable-xvid) _xvid=no ;;
|
|
--enable-x264) _x264=yes ;;
|
|
--disable-x264) _x264=no ;;
|
|
--enable-divx4linux) _divx4linux=yes ;;
|
|
--disable-divx4linux) _divx4linux=no ;;
|
|
--enable-opendivx) _opendivx=yes ;;
|
|
--disable-opendivx) _opendivx=no ;;
|
|
--enable-libavcodec) _libavcodec=yes ;;
|
|
--disable-libavcodec) _libavcodec=no ;;
|
|
--enable-amr_nb) _amr_nb=yes ;;
|
|
--disable-amr_nb) _amr_nb=no ;;
|
|
--enable-amr_nb-fixed) _amr_nb_fixed=yes ;;
|
|
--disable-amr_nb-fixed) _amr_nb_fixed=no ;;
|
|
--enable-amr_wb) _amr_wb=yes ;;
|
|
--disable-amr_wb) _amr_wb=no ;;
|
|
--enable-codec=*) _libavcodecs="$_libavcodecs `echo $ac_option | cut -d '=' -f 2`" ;;
|
|
--disable-codec=*) _libavcodecs=`echo $_libavcodecs | sed "s/\`echo $ac_option | cut -d '=' -f 2\`//g"` ;;
|
|
--enable-libavformat) _libavformat=yes;;
|
|
--disable-libavformat) _libavformat=no ;;
|
|
--enable-libfame) _fame=yes ;;
|
|
--disable-libfame) _fame=no ;;
|
|
--enable-lirc) _lirc=yes ;;
|
|
--disable-lirc) _lirc=no ;;
|
|
--enable-lircc) _lircc=yes ;;
|
|
--disable-lircc) _lircc=no ;;
|
|
--enable-gui) _gui=yes ;;
|
|
--disable-gui) _gui=no ;;
|
|
--enable-termcap) _termcap=yes ;;
|
|
--disable-termcap) _termcap=no ;;
|
|
--enable-termios) _termios=yes ;;
|
|
--disable-termios) _termios=no ;;
|
|
--enable-3dfx) _3dfx=yes ;;
|
|
--disable-3dfx) _3dfx=no ;;
|
|
--enable-tdfxfb) _tdfxfb=yes ;;
|
|
--disable-tdfxvid) _tdfxvid=no ;;
|
|
--enable-tdfxvid) _tdfxvid=yes ;;
|
|
--disable-tga) _tga=no ;;
|
|
--enable-tga) _tga=yes ;;
|
|
--disable-tdfxfb) _tdfxfb=no ;;
|
|
--enable-directfb) _directfb=yes ;;
|
|
--disable-directfb) _directfb=no ;;
|
|
--enable-zr) _zr=yes ;;
|
|
--disable-zr) _zr=no ;;
|
|
--enable-bl) _bl=yes ;;
|
|
--disable-bl) _bl=no ;;
|
|
--enable-mtrr) _mtrr=yes ;;
|
|
--disable-mtrr) _mtrr=no ;;
|
|
--enable-largefiles) _largefiles=yes ;;
|
|
--disable-largefiles) _largefiles=no ;;
|
|
--enable-shm) _shm=yes ;;
|
|
--disable-shm) _shm=no ;;
|
|
--enable-select) _select=yes ;;
|
|
--disable-select) _select=no ;;
|
|
--enable-linux-devfs) _linux_devfs=yes ;;
|
|
--disable-linux-devfs) _linux_devfs=no ;;
|
|
--enable-cdparanoia) _cdparanoia=yes ;;
|
|
--disable-cdparanoia) _cdparanoia=no ;;
|
|
--enable-big-endian) _big_endian=yes ;;
|
|
--disable-big-endian) _big_endian=no ;;
|
|
--enable-freetype) _freetype=yes ;;
|
|
--disable-freetype) _freetype=no ;;
|
|
--enable-fontconfig) _fontconfig=yes ;;
|
|
--disable-fontconfig) _fontconfig=no ;;
|
|
--enable-unrarlib) _unrarlib=yes ;;
|
|
--disable-unrarlib) _unrarlib=no ;;
|
|
--enable-ftp) _ftp=yes ;;
|
|
--disable-ftp) _ftp=no ;;
|
|
--enable-vstream) _vstream=yes ;;
|
|
--disable-vstream) _vstream=no ;;
|
|
--enable-pthreads) _pthreads=yes ;;
|
|
--disable-pthreads) _pthreads=no ;;
|
|
|
|
--enable-fribidi) _fribidi=yes ;;
|
|
--disable-fribidi) _fribidi=no ;;
|
|
|
|
--enable-enca) _enca=yes ;;
|
|
--disable-enca) _enca=no ;;
|
|
|
|
--enable-inet6) _inet6=yes ;;
|
|
--disable-inet6) _inet6=no ;;
|
|
|
|
--enable-gethostbyname2) _gethostbyname2=yes ;;
|
|
--disable-gethostbyname2) _gethostbyname2=no ;;
|
|
|
|
--enable-dga) _dga=auto ;; # as we don't know if it's 1 or 2
|
|
--enable-dga=*) _dga=`echo $ac_option | cut -d '=' -f 2` ;;
|
|
--disable-dga) _dga=no ;;
|
|
|
|
--enable-shared-pp) _shared_pp=yes ;;
|
|
--disable-shared-pp) _shared_pp=no ;;
|
|
|
|
--enable-menu) _menu=yes ;;
|
|
--disable-menu) _menu=no ;;
|
|
|
|
--enable-qtx) _qtx=yes ;;
|
|
--disable-qtx) _qtx=no ;;
|
|
|
|
--enable-macosx) _macosx=yes ;;
|
|
--disable-macosx) _macosx=no ;;
|
|
--enable-macosx-finder-support) _macosx_finder_support=yes ;;
|
|
--disable-macosx-finder-support) _macosx_finder_support=no ;;
|
|
--enable-macosx-bundle) _macosx_bundle=yes;;
|
|
--disable-macosx-bundle) _macosx_bundle=no;;
|
|
|
|
--enable-sortsub) _sortsub=yes ;;
|
|
--disable-sortsub) _sortsub=no ;;
|
|
|
|
--charset=*)
|
|
_charset=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--language=*)
|
|
_language=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
# dvdnav disabled, it does not work
|
|
# --with-libdvdnav=*)
|
|
# _dvdnavdir=`echo $ac_option | cut -d '=' -f 2`
|
|
# _dvdnav=yes
|
|
# ;;
|
|
|
|
--with-codecsdir=*)
|
|
_win32libdir=`echo $ac_option | cut -d '=' -f 2`
|
|
_xanimlibdir=`echo $ac_option | cut -d '=' -f 2`
|
|
_reallibdir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-win32libdir=*)
|
|
_win32libdir=`echo $ac_option | cut -d '=' -f 2`
|
|
_win32=yes
|
|
;;
|
|
--with-xanimlibdir=*)
|
|
_xanimlibdir=`echo $ac_option | cut -d '=' -f 2`
|
|
_xanim=yes
|
|
;;
|
|
--with-reallibdir=*)
|
|
_reallibdir=`echo $ac_option | cut -d '=' -f 2`
|
|
_real=yes
|
|
;;
|
|
--with-livelibdir=*)
|
|
_livelibdir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-toolamedir=*)
|
|
_toolamedir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-mlibdir=*)
|
|
_mlibdir=`echo $ac_option | cut -d '=' -f 2`
|
|
_mlib=yes
|
|
;;
|
|
|
|
--with-xmmslibdir=*)
|
|
_xmmslibdir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
|
|
--with-xmmsplugindir=*)
|
|
_xmmsplugindir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
|
|
--enable-profile)
|
|
_profile='-p'
|
|
;;
|
|
--disable-profile)
|
|
_profile=
|
|
;;
|
|
--enable-debug)
|
|
_debug='-g'
|
|
;;
|
|
--enable-debug=*)
|
|
_debug=`echo $_echo_n '-g'$_echo_c; echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--disable-debug)
|
|
_debug=
|
|
;;
|
|
--enable-crash-debug)
|
|
_crash_debug=yes
|
|
;;
|
|
--disable-crash-debug)
|
|
_crash_debug=no
|
|
;;
|
|
--enable-sighandler)
|
|
_sighandler=yes
|
|
;;
|
|
--disable-sighandler)
|
|
_sighandler=no
|
|
;;
|
|
|
|
--enable-sse) _sse=yes ;;
|
|
--disable-sse) _sse=no ;;
|
|
--enable-sse2) _sse2=yes ;;
|
|
--disable-sse2) _sse2=no ;;
|
|
--enable-mmx2) _mmx2=yes ;;
|
|
--disable-mmx2) _mmx2=no ;;
|
|
--enable-3dnow) _3dnow=yes ;;
|
|
--disable-3dnow) _3dnow=no _3dnowex=no ;;
|
|
--enable-3dnowex) _3dnow=yes _3dnowex=yes ;;
|
|
--disable-3dnowex) _3dnowex=no ;;
|
|
--enable-altivec) _altivec=yes ;;
|
|
--disable-altivec) _altivec=no ;;
|
|
--enable-mmx) _mmx=yes ;;
|
|
--disable-mmx) # 3Dnow! and MMX2 require MMX
|
|
_3dnow=no _3dnowex=no _mmx=no _mmx2=no ;;
|
|
|
|
--enable-win32) _win32=yes ;;
|
|
--disable-win32) _win32=no _dshow=no ;;
|
|
--enable-dshow) _win32=yes _dshow=yes ;;
|
|
--disable-dshow) _dshow=no ;;
|
|
|
|
--with-x11incdir=*)
|
|
_inc_x11=-I`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -I,g'`
|
|
;;
|
|
--with-x11libdir=*)
|
|
_x11_paths=`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -L,g'`
|
|
;;
|
|
--with-dxr2incdir=*)
|
|
_inc_dxr2=-I`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -I,g'`
|
|
;;
|
|
--with-xvmclib=*)
|
|
_xvmclib=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-dvbincdir=*)
|
|
_inc_dvb=-I`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -I,g'`
|
|
;;
|
|
--with-xvidlibdir=*)
|
|
_ld_xvid=-L`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -L,g'`
|
|
;;
|
|
--with-xvidincdir=*)
|
|
_inc_xvid=-I`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -I,g'`
|
|
;;
|
|
--with-dtslibdir=*)
|
|
_ld_libdts=-L`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -L,g'`
|
|
;;
|
|
--with-dtsincdir=*)
|
|
_inc_libdts=-I`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -I,g'`
|
|
;;
|
|
--with-x264libdir=*)
|
|
_ld_x264=-L`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -L,g'`
|
|
;;
|
|
--with-x264incdir=*)
|
|
_inc_x264=-I`echo $ac_option | cut -d '=' -f 2 |sed 's,:, -I,g'`
|
|
;;
|
|
--with-sdl-config=*)
|
|
_sdlconfig=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-freetype-config=*)
|
|
_freetypeconfig=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-fribidi-config=*)
|
|
_fribidiconfig=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-gtk-config=*)
|
|
_gtkconfig=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--with-glib-config=*)
|
|
_glibconfig=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
# dvdnav disabled, it does not work
|
|
# --with-dvdnav-config=*)
|
|
# _dvdnavconfig=`echo $ac_option | cut -d '=' -f 2`
|
|
# ;;
|
|
--with-madlibdir=*)
|
|
_ld_mad=-L`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -L,g'`
|
|
;;
|
|
--with-cdparanoiaincdir=*)
|
|
_inc_cdparanoia=-I`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -I,g'`
|
|
;;
|
|
--with-cdparanoialibdir=*)
|
|
_ld_cdparanoia=-L`echo $ac_option | cut -d '=' -f 2 | sed 's,:, -L,g'`
|
|
;;
|
|
--with-termcaplib=*)
|
|
_ld_termcap=-l`echo $ac_option | cut -d '=' -f 2`
|
|
_termcap=yes
|
|
;;
|
|
--prefix=*)
|
|
_prefix=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--bindir=*)
|
|
_bindir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--datadir=*)
|
|
_datadir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--mandir=*)
|
|
_mandir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--confdir=*)
|
|
_confdir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
--libdir=*)
|
|
_libdir=`echo $ac_option | cut -d '=' -f 2`
|
|
;;
|
|
|
|
*)
|
|
echo "Unknown parameter: $ac_option"
|
|
exit 1
|
|
;;
|
|
|
|
esac
|
|
done
|
|
|
|
# Atmos: moved this here, to be correct, if --prefix is specified
|
|
test -z "$_bindir" && _bindir="$_prefix/bin"
|
|
test -z "$_datadir" && _datadir="$_prefix/share/mplayer"
|
|
test -z "$_mandir" && _mandir="$_prefix/man"
|
|
test -z "$_confdir" && _confdir="$_prefix/etc/mplayer"
|
|
test -z "$_libdir" && _libdir="$_prefix/lib"
|
|
test -z "$_mlibdir" && _mlibdir="$MLIBHOME"
|
|
|
|
if x86 ; then
|
|
# Checking assembler (_as) compatibility...
|
|
# Added workaround for older as that reads from stdin by default - atmos
|
|
as_version=`echo '' | $_as -version 2>&1 | sed -n 's/^.*assembler \(version \)*\([0-9.]*\).*$/\2/p'`
|
|
echocheck "assembler ($_as $as_version)"
|
|
|
|
_pref_as_version='2.9.1'
|
|
echo 'nop' > $TMPS
|
|
if test "$_mmx" = yes ; then
|
|
echo 'emms' >> $TMPS
|
|
fi
|
|
if test "$_3dnow" = yes ; then
|
|
_pref_as_version='2.10.1'
|
|
echo 'femms' >> $TMPS
|
|
fi
|
|
if test "$_3dnowex" = yes ; then
|
|
_pref_as_version='2.10.1'
|
|
echo 'pswapd %mm0, %mm0' >> $TMPS
|
|
fi
|
|
if test "$_mmx2" = yes ; then
|
|
_pref_as_version='2.10.1'
|
|
echo 'movntq %mm0, (%eax)' >> $TMPS
|
|
fi
|
|
if test "$_sse" = yes ; then
|
|
_pref_as_version='2.10.1'
|
|
echo 'xorps %xmm0, %xmm0' >> $TMPS
|
|
fi
|
|
#if test "$_sse2" = yes ; then
|
|
# _pref_as_version='2.11'
|
|
# echo 'xorpd %xmm0, %xmm0' >> $TMPS
|
|
#fi
|
|
$_as $TMPS -o $TMPO > /dev/null 2>&1 || as_verc_fail=yes
|
|
|
|
if test "$as_verc_fail" != yes ; then
|
|
echores "ok"
|
|
else
|
|
echores "failed"
|
|
echo "Upgrade binutils to ${_pref_as_version} ..."
|
|
die "obsolete binutils version"
|
|
fi
|
|
fi
|
|
|
|
if ppc ; then
|
|
|
|
# check if altivec is supported by the compiler, and how to enable it
|
|
|
|
_altivec_gcc_flags=''
|
|
|
|
if test "$_altivec" = yes -o "$_runtime_cpudetection" = yes ; then
|
|
echocheck "GCC altivec support"
|
|
|
|
p=''
|
|
cat > $TMPC << EOF
|
|
int main() {
|
|
return 0;
|
|
}
|
|
EOF
|
|
FSF_flags='-maltivec -mabi=altivec'
|
|
Darwin_flags='-faltivec -D__APPLE_ALTIVEC__'
|
|
|
|
# check for Darwin-style flags first, since
|
|
# gcc-3.3 (August Update from Apple) on MacOS 10.2.8
|
|
# accepts but ignores FSF-style flags...
|
|
|
|
if test -z "$p"; then
|
|
cc_check $Darwin_flags && p='Darwin'
|
|
fi
|
|
if test -z "$p"; then
|
|
cc_check $FSF_flags && p='FSF'
|
|
fi
|
|
|
|
case $p in
|
|
FSF) _altivec_gcc_flags="$FSF_flags" _altivec=yes ;;
|
|
Darwin) _altivec_gcc_flags="$Darwin_flags" _altivec=yes ;;
|
|
*) _altivec=no ;;
|
|
esac
|
|
|
|
if test -z "$p"; then
|
|
p=none
|
|
else
|
|
p="$p-style ($_altivec_gcc_flags)"
|
|
fi
|
|
|
|
echores "$p"
|
|
fi
|
|
|
|
# check if <altivec.h> should be included
|
|
|
|
_def_altivec_h='#undef HAVE_ALTIVEC_H'
|
|
|
|
if test "$_altivec" = yes ; then
|
|
echocheck "altivec.h"
|
|
cat > $TMPC << EOF
|
|
#include <altivec.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_have_altivec_h=no
|
|
cc_check $_altivec_gcc_flags && _have_altivec_h=yes
|
|
if test "$_have_altivec_h" = yes ; then
|
|
_def_altivec_h='#define HAVE_ALTIVEC_H 1'
|
|
fi
|
|
echores "$_have_altivec_h"
|
|
fi
|
|
|
|
# disable runtime cpudetection if
|
|
# - we cannot generate altivec code
|
|
# - altivec is disabled by the user
|
|
|
|
if test "$_runtime_cpudetection" = yes -a "$_altivec" = no ; then
|
|
_runtime_cpudetection=no
|
|
fi
|
|
|
|
# show that we are optimizing for altivec (if enabled and supported)
|
|
|
|
if test "$_runtime_cpudetection" = no -a "$_altivec" = yes ; then
|
|
_optimizing="$_optimizing altivec"
|
|
fi
|
|
|
|
# if altivec is enabled, make sure the correct flags turn up in CFLAGS
|
|
|
|
if test "$_altivec" = yes ; then
|
|
_mcpu="$_mcpu $_altivec_gcc_flags"
|
|
fi
|
|
|
|
# setup _def_altivec correctly
|
|
|
|
if test "$_altivec" = yes ; then
|
|
_def_altivec='#define HAVE_ALTIVEC 1'
|
|
else
|
|
_def_altivec='#undef HAVE_ALTIVEC'
|
|
fi
|
|
fi
|
|
|
|
_def_mmx='#undef HAVE_MMX'
|
|
test "$_mmx" = yes && _def_mmx='#define HAVE_MMX 1'
|
|
_def_mmx2='#undef HAVE_MMX2'
|
|
test "$_mmx2" = yes && _def_mmx2='#define HAVE_MMX2 1'
|
|
_def_3dnow='#undef HAVE_3DNOW'
|
|
test "$_3dnow" = yes && _def_3dnow='#define HAVE_3DNOW 1'
|
|
_def_3dnowex='#undef HAVE_3DNOWEX'
|
|
test "$_3dnowex" = yes && _def_3dnowex='#define HAVE_3DNOWEX 1'
|
|
_def_sse='#undef HAVE_SSE'
|
|
test "$_sse" = yes && _def_sse='#define HAVE_SSE 1'
|
|
_def_sse2='#undef HAVE_SSE2'
|
|
test "$_sse2" = yes && _def_sse2='#define HAVE_SSE2 1'
|
|
|
|
# Checking kernel version...
|
|
if x86 && linux ; then
|
|
_k_verc_problem=no
|
|
kernel_version=`uname -r 2>&1`
|
|
echocheck "$system_name kernel version"
|
|
case "$kernel_version" in
|
|
'') kernel_version="?.??"; _k_verc_fail=yes;;
|
|
[0-1].[0-9].[0-9]*|2.[0-3].[0-9]*)
|
|
_k_verc_problem=yes;;
|
|
esac
|
|
if test "$_k_verc_problem" = yes && test "$_sse" = yes ; then
|
|
_k_verc_fail=yes
|
|
fi
|
|
if test "$_k_verc_fail" ; then
|
|
echores "$kernel_version, fail"
|
|
echo "WARNING! If you want to run MPlayer on this system, get prepared for problems!"
|
|
echo "2.2.x has limited SSE support. Upgrade the kernel or use --disable-sse if you"
|
|
echo "experience crashes. MPlayer tries to autodetect if your kernel correctly"
|
|
echo "supports SSE, but you have been warned! If you are using a kernel older than"
|
|
echo "2.2.x you must upgrade it to get SSE support!"
|
|
# die "Your kernel is too old for this CPU." # works fine on some 2.2.x so don't die (later check will test)
|
|
else
|
|
echores "$kernel_version, ok"
|
|
fi
|
|
fi
|
|
|
|
if test "$_vidix" = auto ; then
|
|
_vidix=no
|
|
# should check for x86 systems supporting VIDIX (does QNX have VIDIX?)
|
|
x86 && _vidix=yes
|
|
ppc && linux && _vidix=yes
|
|
alpha && linux && _vidix=yes
|
|
qnx && _vidix=no
|
|
sunos && _vidix=no
|
|
beos && _vidix=no
|
|
fi
|
|
|
|
echocheck "mplayer binary name"
|
|
if win32 ; then
|
|
_prg="mplayer.exe"
|
|
_prg_mencoder="mencoder.exe"
|
|
else
|
|
_prg="mplayer"
|
|
_prg_mencoder="mencoder"
|
|
fi
|
|
echores $_prg
|
|
|
|
|
|
# On QNX we must link to libph - Gabucino
|
|
if qnx ; then
|
|
_ld_arch="$_ld_arch -lph"
|
|
fi
|
|
|
|
# checking for a working awk, I'm using mawk first, because it's fastest - atmos
|
|
_awk=
|
|
if test "$_vidix" = yes ; then
|
|
_awk_verc_fail=yes
|
|
echocheck "awk"
|
|
for _awk in mawk gawk nawk awk; do
|
|
if ( $_awk 'BEGIN{testme();}function testme(){print"";}' ) >> "$TMPLOG" 2>&1; then
|
|
_awk_verc_fail=no
|
|
break
|
|
fi
|
|
done
|
|
test "$_awk_verc_fail" = yes && _awk=no
|
|
echores "$_awk"
|
|
if test "$_awk_verc_fail" = yes; then
|
|
echo "VIDIX needs awk, but no working implementation was found!"
|
|
echo "Try the GNU version, which can be downloaded from:"
|
|
echo "ftp://ftp.gnu.org/gnu/gawk/"
|
|
echo "If you don't need VIDIX, you can use configure --disable-vidix instead."
|
|
die "no awk"
|
|
fi
|
|
fi
|
|
|
|
# If IRIX we must use ar instead of ranlib (not present on IRIX systems)
|
|
if irix ; then
|
|
_ranlib='ar -r'
|
|
elif linux ; then
|
|
_ranlib='true'
|
|
fi
|
|
|
|
######################
|
|
# MAIN TESTS GO HERE #
|
|
######################
|
|
|
|
|
|
echocheck "extra headers"
|
|
if test "$_inc_extra" ; then
|
|
echores "$_inc_extra"
|
|
else
|
|
echores "none"
|
|
fi
|
|
|
|
|
|
echocheck "extra libs"
|
|
if test "$_ld_extra" ; then
|
|
echores "$_ld_extra"
|
|
else
|
|
echores "none"
|
|
fi
|
|
|
|
echocheck "-lposix"
|
|
cat > $TMPC <<EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if cc_check -lposix ; then
|
|
_ld_arch="$_ld_arch -lposix"
|
|
echores "yes"
|
|
else
|
|
echores "no"
|
|
fi
|
|
|
|
echocheck "-lm"
|
|
cat > $TMPC <<EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if cc_check -lm ; then
|
|
_ld_lm="-lm"
|
|
echores "yes"
|
|
else
|
|
_ld_lm=""
|
|
echores "no"
|
|
fi
|
|
|
|
# Checking for localization ...
|
|
# CSAK EGY MARADHAT - A HEGYLAKO
|
|
echocheck "i18n"
|
|
if test "$_i18n" != no ; then
|
|
cat > $TMPC <<EOF
|
|
#include <libintl.h>
|
|
int main(void) { gettext("test"); return 0; }
|
|
EOF
|
|
_i18n=no
|
|
_i18n_libs=""
|
|
if test "$_i18n" = auto ; then
|
|
cc_check && _i18n=yes
|
|
else
|
|
for i18n_lib in "" "-lintl"; do
|
|
cc_check $i18n_lib && _i18n=yes && _i18n_libs=$i18n_lib && break
|
|
done
|
|
fi
|
|
fi
|
|
if test "$_i18n" = yes ; then
|
|
_def_i18n='#define USE_I18N 1'
|
|
else
|
|
_def_i18n='#undef USE_I18N'
|
|
fi
|
|
if test -z "$_i18n_libs" ; then
|
|
echores "$_i18n"
|
|
else
|
|
echores "$_i18n (using $_i18n_libs)"
|
|
fi
|
|
|
|
|
|
echocheck "iconv"
|
|
if test "$_iconv" = auto ; then
|
|
_iconv_tmp='#include <iconv.h>'
|
|
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <unistd.h>
|
|
$_iconv_tmp
|
|
#define INBUFSIZE 1024
|
|
#define OUTBUFSIZE 4096
|
|
|
|
char inbuffer[INBUFSIZE];
|
|
char outbuffer[OUTBUFSIZE];
|
|
|
|
int main(void) {
|
|
size_t numread;
|
|
iconv_t icdsc;
|
|
char *tocode="UTF-8";
|
|
char *fromcode="cp1250";
|
|
if ((icdsc = iconv_open (tocode, fromcode)) != (iconv_t)(-1)) {
|
|
while ((numread = read (0, inbuffer, INBUFSIZE))) {
|
|
char *iptr=inbuffer;
|
|
char *optr=outbuffer;
|
|
size_t inleft=numread;
|
|
size_t outleft=OUTBUFSIZE;
|
|
if (iconv(icdsc, (const char **)&iptr, &inleft, &optr, &outleft)
|
|
!= (size_t)(-1)) {
|
|
write (1, outbuffer, OUTBUFSIZE - outleft);
|
|
}
|
|
}
|
|
if (iconv_close(icdsc) == -1)
|
|
;
|
|
}
|
|
}
|
|
EOF
|
|
_iconv=no
|
|
if cc_check $_ld_lm ; then
|
|
_iconv=yes
|
|
elif cc_check $_ld_lm -liconv ; then
|
|
_iconv=yes
|
|
_ld_iconv='-liconv'
|
|
fi
|
|
fi
|
|
if test "$_iconv" = yes ; then
|
|
_def_iconv='#define USE_ICONV 1'
|
|
else
|
|
_def_iconv='#undef USE_ICONV'
|
|
fi
|
|
echores "$_iconv"
|
|
|
|
|
|
echocheck "langinfo"
|
|
if test "$_langinfo" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <langinfo.h>
|
|
int main(void) { nl_langinfo(CODESET); return 0; }
|
|
EOF
|
|
_langinfo=no
|
|
cc_check && _langinfo=yes
|
|
fi
|
|
if test "$_langinfo" = yes ; then
|
|
_def_langinfo='#define USE_LANGINFO 1'
|
|
else
|
|
_def_langinfo='#undef USE_LANGINFO'
|
|
fi
|
|
echores "$_langinfo"
|
|
|
|
|
|
echocheck "language"
|
|
test -z "$_language" && _language=$LINGUAS
|
|
_language=`echo $_language | sed 's/,/ /g'`
|
|
echo $_language | grep all > /dev/null || LANGUAGES="$_language en"
|
|
for lang in $_language ; do
|
|
test "$lang" = all && lang=en
|
|
if test -f "help/help_mp-${lang}.h" ; then
|
|
_language=$lang
|
|
break
|
|
else
|
|
echo -n "$lang not found, "
|
|
_language=`echo $_language | sed "s/$lang *//"`
|
|
fi
|
|
done
|
|
test -z "$_language" && _language=en
|
|
_mp_help="help/help_mp-${_language}.h"
|
|
test -f $_mp_help || die "$_mp_help not found"
|
|
for lang in $LANGUAGES ; do
|
|
if test -f "DOCS/man/$lang/mplayer.1" ; then
|
|
MAN_LANG="$MAN_LANG $lang"
|
|
fi
|
|
done
|
|
_doc_lang=$_language
|
|
test -d DOCS/xml/$_doc_lang || _doc_lang=en
|
|
echores "using $_language (man pages: $MAN_LANG)"
|
|
|
|
|
|
echocheck "enable sighandler"
|
|
if test "$_sighandler" = yes ; then
|
|
_def_sighandler='#define ENABLE_SIGHANDLER 1'
|
|
else
|
|
_def_sighandler='#undef ENABLE_SIGHANDLER'
|
|
fi
|
|
echores "$_sighandler"
|
|
|
|
echocheck "runtime cpudetection"
|
|
if test "$_runtime_cpudetection" = yes ; then
|
|
_optimizing="Runtime CPU-Detection enabled"
|
|
_def_runtime_cpudetection='#define RUNTIME_CPUDETECT 1'
|
|
else
|
|
_def_runtime_cpudetection='#undef RUNTIME_CPUDETECT'
|
|
fi
|
|
echores "$_runtime_cpudetection"
|
|
|
|
|
|
echocheck "restrict keyword"
|
|
for restrict_keyword in restrict __restrict __restrict__ ; do
|
|
echo "void foo(char * $restrict_keyword p); int main(){}" > $TMPC
|
|
if cc_check; then
|
|
_def_restrict_keyword=$restrict_keyword
|
|
break;
|
|
fi
|
|
done
|
|
if [ -n "$_def_restrict_keyword" ]; then
|
|
echores "$_def_restrict_keyword"
|
|
else
|
|
echores "none"
|
|
fi
|
|
# Avoid infinite recursion loop ("#define restrict restrict")
|
|
if [ "$_def_restrict_keyword" != "restrict" ]; then
|
|
_def_restrict_keyword="#define restrict $_def_restrict_keyword"
|
|
else
|
|
_def_restrict_keyword=""
|
|
fi
|
|
|
|
|
|
echocheck "__builtin_expect"
|
|
# GCC branch prediction hint
|
|
cat > $TMPC << EOF
|
|
int foo (int a) {
|
|
a = __builtin_expect (a, 10);
|
|
return a == 10 ? 0 : 1;
|
|
}
|
|
int main() { return foo(10) && foo(0); }
|
|
EOF
|
|
_builtin_expect=no
|
|
cc_check && _builtin_expect=yes
|
|
if test "$_builtin_expect" = yes ; then
|
|
_def_builtin_expect='#define HAVE_BUILTIN_EXPECT 1'
|
|
else
|
|
_def_builtin_expect='#undef HAVE_BUILTIN_EXPECT'
|
|
fi
|
|
echores "$_builtin_expect"
|
|
|
|
|
|
echocheck "kstat"
|
|
cat > $TMPC << EOF
|
|
#include <kstat.h>
|
|
int main(void) { (void) kstat_open(); (void) kstat_close(0); return 0; }
|
|
EOF
|
|
_kstat=no
|
|
cc_check -lkstat && _kstat=yes
|
|
if test "$_kstat" = yes ; then
|
|
_ld_arch="-lkstat $_ld_arch"
|
|
fi
|
|
if test "$_kstat" = yes ; then
|
|
_def_kstat="#define HAVE_LIBKSTAT 1"
|
|
else
|
|
_def_kstat="#undef HAVE_LIBKSTAT"
|
|
fi
|
|
echores "$_kstat"
|
|
|
|
|
|
echocheck "posix4"
|
|
# required for nanosleep on some systems
|
|
cat > $TMPC << EOF
|
|
#include <time.h>
|
|
int main(void) { (void) nanosleep(0, 0); return 0; }
|
|
EOF
|
|
_posix4=no
|
|
cc_check -lposix4 && _posix4=yes
|
|
if test "$_posix4" = yes ; then
|
|
_ld_arch="-lposix4 $_ld_arch"
|
|
fi
|
|
echores "$_posix4"
|
|
|
|
echocheck "-std=gnu99"
|
|
cat > $TMPC << EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_gnu99=no
|
|
cc_check -std=gnu99 && _gnu99=yes
|
|
if test "$_gnu99" = yes ; then
|
|
_opt_gnu99="-std=gnu99"
|
|
else
|
|
_opt_gnu99=""
|
|
fi
|
|
echores "$_gnu99"
|
|
|
|
echocheck "lrintf"
|
|
cat > $TMPC << EOF
|
|
#include <math.h>
|
|
int main(void) { long (*foo)(float); foo = lrintf; (void)(*foo)(0.0); return 0; }
|
|
EOF
|
|
_lrintf=no
|
|
cc_check $_opt_gnu99 -D_GNU_SOURCE $_ld_lm && _lrintf=yes
|
|
if test "$_lrintf" = yes ; then
|
|
_def_lrintf="#define HAVE_LRINTF 1"
|
|
else
|
|
_def_lrintf="#undef HAVE_LRINTF"
|
|
fi
|
|
echores "$_lrintf"
|
|
|
|
echocheck "round"
|
|
cat > $TMPC << EOF
|
|
#include <math.h>
|
|
int main(void) { (void) round(0.0); return 0; }
|
|
EOF
|
|
_round=no
|
|
cc_check $_ld_lm && _round=yes
|
|
if test "$_round" = yes ; then
|
|
_def_round="#define HAVE_ROUND 1"
|
|
else
|
|
_def_round="#undef HAVE_ROUND"
|
|
fi
|
|
echores "$_round"
|
|
|
|
echocheck "nanosleep"
|
|
# also check for nanosleep
|
|
cat > $TMPC << EOF
|
|
#include <time.h>
|
|
int main(void) { (void) nanosleep(0, 0); return 0; }
|
|
EOF
|
|
_nanosleep=no
|
|
cc_check $_ld_arch && _nanosleep=yes
|
|
if test "$_nanosleep" = yes ; then
|
|
_def_nanosleep='#define HAVE_NANOSLEEP 1'
|
|
else
|
|
_def_nanosleep='#undef HAVE_NANOSLEEP'
|
|
fi
|
|
echores "$_nanosleep"
|
|
|
|
|
|
echocheck "socklib"
|
|
# for Solaris (socket stuff is in -lsocket, gethostbyname and friends in -lnsl):
|
|
# for BeOS (socket stuff is in -lsocket, gethostbyname and friends in -lbind):
|
|
cat > $TMPC << EOF
|
|
#include <netdb.h>
|
|
int main(void) { (void) gethostbyname(0); return 0; }
|
|
EOF
|
|
cc_check -lsocket && _ld_sock="-lsocket"
|
|
cc_check -lnsl && _ld_sock="-lnsl"
|
|
cc_check -lsocket -lnsl && _ld_sock="-lsocket -lnsl"
|
|
cc_check -lsocket -ldnet && _ld_sock="-lsocket -ldnet"
|
|
cc_check -lsocket -lbind && _ld_sock="-lsocket -lbind"
|
|
if test $_winsock2 = auto && not cygwin ; then
|
|
_winsock2=no
|
|
cat > $TMPC << EOF
|
|
#include <winsock2.h>
|
|
int main(void) { (void) gethostbyname(0); return 0; }
|
|
EOF
|
|
cc_check -lws2_32 && _ld_sock="-lws2_32" && _winsock2=yes
|
|
fi
|
|
if test "$_ld_sock" ; then
|
|
echores "yes (using $_ld_sock)"
|
|
else
|
|
echores "no"
|
|
fi
|
|
|
|
|
|
if test $_winsock2 = yes ; then
|
|
_ld_sock="-lws2_32"
|
|
_def_winsock2='#define HAVE_WINSOCK2 1'
|
|
else
|
|
_def_winsock2='#undef HAVE_WINSOCK2'
|
|
fi
|
|
|
|
|
|
_use_aton=no
|
|
echocheck "inet_pton()"
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/socket.h>
|
|
#include <arpa/inet.h>
|
|
int main(void) { (void) inet_pton(0, 0, 0); return 0; }
|
|
EOF
|
|
if test "$_winsock2" = yes ; then
|
|
echores "not needed (using winsock2 functions)"
|
|
elif cc_check $_ld_sock ; then
|
|
# NOTE: Linux has libresolv but does not need it
|
|
:
|
|
echores "yes (using $_ld_sock)"
|
|
elif cc_check $_ld_sock -lresolv ; then
|
|
# NOTE: needed for SunOS at least
|
|
_ld_sock="$_ld_sock -lresolv"
|
|
echores "yes (using $_ld_sock)"
|
|
else
|
|
echores "no (=> i'll try inet_aton next)"
|
|
|
|
echocheck "inet_aton()"
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/socket.h>
|
|
#include <arpa/inet.h>
|
|
int main(void) { (void) inet_aton(0, 0); return 0; }
|
|
EOF
|
|
_use_aton=yes
|
|
if cc_check $_ld_sock ; then
|
|
# NOTE: Linux has libresolv but does not need it
|
|
:
|
|
echores "yes (using $_ld_sock)"
|
|
elif cc_check $_ld_sock -lresolv ; then
|
|
# NOTE: needed for SunOS at least
|
|
_ld_sock="$_ld_sock -lresolv"
|
|
echores "yes (using $_ld_sock)"
|
|
else
|
|
_use_aton=no
|
|
_network=no
|
|
echores "no (=> network support disabled)"
|
|
fi
|
|
fi
|
|
|
|
_def_use_aton='#undef USE_ATON'
|
|
if test "$_use_aton" != no; then
|
|
_def_use_aton='#define USE_ATON 1'
|
|
fi
|
|
|
|
|
|
echocheck "inttypes.h (required)"
|
|
cat > $TMPC << EOF
|
|
#include <inttypes.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_inttypes=no
|
|
cc_check && _inttypes=yes
|
|
if test "$_inttypes" = yes ; then
|
|
# nothing to do
|
|
:
|
|
else
|
|
echores "no"
|
|
echocheck "bitypes.h (inttypes.h predecessor)"
|
|
cat > $TMPC << EOF
|
|
#include <sys/bitypes.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_inttypes=no
|
|
cc_check && _inttypes=yes
|
|
if test "$_inttypes" = yes ; then
|
|
die "You don't have inttypes.h, but sys/bitypes.h is present. Please copy etc/inttypes.h into the include path, and re-run configure."
|
|
else
|
|
die "Cannot find header either inttypes.h or bitypes.h (see DOCS/HTML/$_doc_lang/faq.html)."
|
|
fi
|
|
fi
|
|
echores "$_inttypes"
|
|
|
|
|
|
echocheck "int_fastXY_t in inttypes.h"
|
|
cat > $TMPC << EOF
|
|
#include <inttypes.h>
|
|
int main(void) {
|
|
volatile int_fast16_t v= 0;
|
|
return v; }
|
|
EOF
|
|
_fast_inttypes=no
|
|
cc_check && _fast_inttypes=yes
|
|
if test "$_fast_inttypes" = yes ; then
|
|
# nothing to do
|
|
:
|
|
else
|
|
_def_fast_inttypes='
|
|
typedef signed char int_fast8_t;
|
|
typedef signed int int_fast16_t;
|
|
typedef signed int int_fast32_t;
|
|
typedef unsigned char uint_fast8_t;
|
|
typedef unsigned int uint_fast16_t;
|
|
typedef unsigned int uint_fast32_t;'
|
|
fi
|
|
echores "$_fast_inttypes"
|
|
|
|
|
|
echocheck "word size"
|
|
_mp_wordsize="#undef MP_WORDSIZE"
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <sys/types.h>
|
|
int main(void) { printf("%d\n", sizeof(size_t)*8); return 0; }
|
|
EOF
|
|
cc_check && _wordsize=`$TMPO` && _mp_wordsize="#define MP_WORDSIZE $_wordsize"
|
|
echores "$_wordsize"
|
|
|
|
|
|
echocheck "stddef.h"
|
|
cat > $TMPC << EOF
|
|
#include <stddef.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_stddef=no
|
|
cc_check && _stddef=yes
|
|
if test "$_stddef" = yes ; then
|
|
_def_stddef='#define HAVE_STDDEF_H 1'
|
|
else
|
|
_def_stddef='#undef HAVE_STDDEF_H'
|
|
fi
|
|
echores "$_stddef"
|
|
|
|
|
|
echocheck "malloc.h"
|
|
cat > $TMPC << EOF
|
|
#include <malloc.h>
|
|
int main(void) { (void) malloc(0); return 0; }
|
|
EOF
|
|
_malloc=no
|
|
cc_check && _malloc=yes
|
|
if test "$_malloc" = yes ; then
|
|
_def_malloc='#define HAVE_MALLOC_H 1'
|
|
else
|
|
_def_malloc='#undef HAVE_MALLOC_H'
|
|
fi
|
|
# malloc.h emits a warning in FreeBSD and OpenBSD
|
|
(freebsd || openbsd) && _def_malloc='#undef HAVE_MALLOC_H'
|
|
echores "$_malloc"
|
|
|
|
|
|
echocheck "memalign()"
|
|
# XXX restrict to x86 ? extend to other CPUs/cacheline sizes ?
|
|
cat > $TMPC << EOF
|
|
#include <malloc.h>
|
|
int main (void) { (void) memalign(64, sizeof(char)); return 0; }
|
|
EOF
|
|
_memalign=no
|
|
cc_check && _memalign=yes
|
|
if test "$_memalign" = yes ; then
|
|
_def_memalign='#define HAVE_MEMALIGN 1'
|
|
else
|
|
_def_memalign='#undef HAVE_MEMALIGN'
|
|
fi
|
|
echores "$_memalign"
|
|
|
|
|
|
echocheck "alloca.h"
|
|
cat > $TMPC << EOF
|
|
#include <alloca.h>
|
|
int main(void) { (void) alloca(0); return 0; }
|
|
EOF
|
|
_alloca=no
|
|
cc_check && _alloca=yes
|
|
if cc_check ; then
|
|
_def_alloca='#define HAVE_ALLOCA_H 1'
|
|
else
|
|
_def_alloca='#undef HAVE_ALLOCA_H'
|
|
fi
|
|
echores "$_alloca"
|
|
|
|
|
|
echocheck "mman.h"
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/mman.h>
|
|
int main(void) { (void) mmap(0, 0, 0, 0, 0, 0); return 0; }
|
|
EOF
|
|
_mman=no
|
|
cc_check && _mman=yes
|
|
if test "$_mman" = yes ; then
|
|
_def_mman='#define HAVE_SYS_MMAN_H 1'
|
|
else
|
|
_def_mman='#undef HAVE_SYS_MMAN_H'
|
|
fi
|
|
echores "$_mman"
|
|
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/mman.h>
|
|
int main(void) { void *p = MAP_FAILED; return 0; }
|
|
EOF
|
|
_mman_has_map_failed=no
|
|
cc_check && _mman_has_map_failed=yes
|
|
if test "$_mman_has_map_failed" = yes ; then
|
|
_def_mman_has_map_failed=''
|
|
else
|
|
_def_mman_has_map_failed='#define MAP_FAILED ((void *) -1)'
|
|
fi
|
|
|
|
echocheck "dynamic loader"
|
|
cat > $TMPC << EOF
|
|
#include <dlfcn.h>
|
|
int main(void) { dlopen(0, 0); dlclose(0); dlsym(0, 0); return 0; }
|
|
EOF
|
|
_dl=no
|
|
if cc_check ; then
|
|
_dl=yes
|
|
elif cc_check -ldl ; then
|
|
_dl=yes
|
|
_ld_dl='-ldl'
|
|
fi
|
|
if test "$_dl" = yes ; then
|
|
_def_dl='#define HAVE_LIBDL 1'
|
|
else
|
|
_def_dl='#undef HAVE_LIBDL'
|
|
fi
|
|
echores "$_dl"
|
|
|
|
|
|
echocheck "dynamic a/v plugins support"
|
|
if test "$_dl" = no ; then
|
|
_dynamic_plugins=no
|
|
fi
|
|
if test "$_dynamic_plugins" = yes ; then
|
|
_def_dynamic_plugins='#define DYNAMIC_PLUGINS 1'
|
|
else
|
|
_def_dynamic_plugins='#undef DYNAMIC_PLUGINS'
|
|
fi
|
|
echores "$_dynamic_plugins"
|
|
|
|
|
|
#echocheck "dynamic linking"
|
|
# FIXME !! make this dynamic detection work and modify at the end (search _ld_dl_dynamic)
|
|
# also gcc flags are different, but ld flags aren't (-Bdynamic/-Bstatic/-Bsymbolic)
|
|
#cat > $TMPC << EOF
|
|
#int main(void) { return 0; }
|
|
#EOF
|
|
#if cc_check -rdynamic ; then
|
|
# _ld_dl_dynamic='-rdynamic'
|
|
#elif cc_check -Bdynamic ; then
|
|
# _ld_dl_dynamic='-Bdynamic'
|
|
#elif cc_check ; then
|
|
# _ld_dl_dynamic=''
|
|
#fi
|
|
#echores "using $_ld_dl_dynamic"
|
|
|
|
_def_threads='#undef HAVE_THREADS'
|
|
|
|
echocheck "pthread"
|
|
_ld_pthread=''
|
|
if test "$_pthreads" != no ; then
|
|
cat > $TMPC << EOF
|
|
#include <pthread.h>
|
|
void* func(void *arg) { return arg; }
|
|
int main(void) { pthread_t tid; return pthread_create (&tid, 0, func, 0) == 0 ? 0 : 1; }
|
|
EOF
|
|
if hpux ; then
|
|
_ld_pthread=''
|
|
elif ( cc_check -lpthreadGC2 && $TMPO ) ; then # mingw pthreads-win32
|
|
_ld_pthread='-lpthreadGC2'
|
|
elif ( cc_check && $TMPO ) ; then # QNX
|
|
_ld_pthread=' ' # _ld_pthread='' would disable pthreads, but the test worked
|
|
elif ( cc_check -lpthread && $TMPO ) ; then
|
|
_ld_pthread='-lpthread'
|
|
elif ( cc_check -pthread && $TMPO ) ; then
|
|
_ld_pthread='-pthread'
|
|
else
|
|
if test "$_ld_static" ; then
|
|
# for crosscompilation, we cannot execute the program, be happy if we can link statically
|
|
if ( cc_check -lpthread ) ; then
|
|
_ld_pthread='-lpthread'
|
|
elif ( cc_check -pthread ) ; then
|
|
_ld_pthread='-pthread'
|
|
else
|
|
_ld_pthread=''
|
|
fi
|
|
else
|
|
_ld_pthread=''
|
|
fi
|
|
fi
|
|
fi
|
|
if test "$_ld_pthread" != '' ; then
|
|
echores "yes (using $_ld_pthread)"
|
|
_pthreads='yes'
|
|
_def_pthreads='#define HAVE_PTHREADS 1'
|
|
_def_threads='#define HAVE_THREADS 1'
|
|
else
|
|
echores "no (v4l, vo_gl, ao_alsa, ao_nas, ao_macosx, win32 loader disabled)"
|
|
_pthreads=''
|
|
_def_pthreads='#undef HAVE_PTHREADS'
|
|
_nas=no ; _tv_v4l=no ; _macosx=no
|
|
if not mingw32 ; then
|
|
_win32=no
|
|
fi
|
|
fi
|
|
|
|
|
|
echocheck "sys/soundcard.h"
|
|
cat > $TMPC << EOF
|
|
#include <sys/soundcard.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_sys_soundcard=no
|
|
cc_check && _sys_soundcard=yes
|
|
if test "$_sys_soundcard" = yes ; then
|
|
_def_sys_soundcard='#define HAVE_SYS_SOUNDCARD_H 1'
|
|
_inc_soundcard='#include <sys/soundcard.h>'
|
|
else
|
|
_def_sys_soundcard='#undef HAVE_SYS_SOUNDCARD_H'
|
|
fi
|
|
echores "$_sys_soundcard"
|
|
|
|
if test "$_sys_soundcard" != yes ; then
|
|
echocheck "soundcard.h"
|
|
cat > $TMPC << EOF
|
|
#include <soundcard.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_soundcard=no
|
|
cc_check && _soundcard=yes
|
|
if linux || test "$_ossaudio" != no ; then
|
|
# use soundcard.h on Linux, or when OSS support is enabled
|
|
echores "$_soundcard"
|
|
else
|
|
# we don't want to use soundcard.h on non-Linux if OSS support not enabled!
|
|
echores "$_soundcard, but ignored!"
|
|
_soundcard=no
|
|
fi
|
|
if test "$_soundcard" = yes ; then
|
|
_def_soundcard='#define HAVE_SOUNDCARD_H 1'
|
|
_inc_soundcard='#include <soundcard.h>'
|
|
else
|
|
_def_soundcard='#undef HAVE_SOUNDCARD_H'
|
|
fi
|
|
else
|
|
_def_soundcard='#undef HAVE_SOUNDCARD_H'
|
|
fi
|
|
|
|
|
|
echocheck "sys/dvdio.h"
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
#include <sys/dvdio.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_dvdio=no
|
|
cc_check && _dvdio=yes
|
|
if test "$_dvdio" = yes ; then
|
|
_def_dvdio='#define DVD_STRUCT_IN_SYS_DVDIO_H 1'
|
|
else
|
|
_def_dvdio='#undef DVD_STRUCT_IN_SYS_DVDIO_H'
|
|
fi
|
|
echores "$_dvdio"
|
|
|
|
|
|
echocheck "sys/cdio.h"
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
#include <sys/cdio.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_cdio=no
|
|
cc_check && _cdio=yes
|
|
if test "$_cdio" = yes ; then
|
|
_def_cdio='#define DVD_STRUCT_IN_SYS_CDIO_H 1'
|
|
else
|
|
_def_cdio='#undef DVD_STRUCT_IN_SYS_CDIO_H'
|
|
fi
|
|
echores "$_cdio"
|
|
|
|
|
|
echocheck "linux/cdrom.h"
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <linux/cdrom.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_cdrom=no
|
|
cc_check && _cdrom=yes
|
|
if test "$_cdrom" = yes ; then
|
|
_def_cdrom='#define DVD_STRUCT_IN_LINUX_CDROM_H 1'
|
|
else
|
|
_def_cdrom='#undef DVD_STRUCT_IN_LINUX_CDROM_H'
|
|
fi
|
|
echores "$_cdrom"
|
|
|
|
|
|
echocheck "dvd.h"
|
|
cat > $TMPC << EOF
|
|
#include <dvd.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_dvd=no
|
|
cc_check && _dvd=yes
|
|
if test "$_dvd" = yes ; then
|
|
_def_dvd='#define DVD_STRUCT_IN_DVD_H 1'
|
|
else
|
|
_def_dvd='#undef DVD_STRUCT_IN_DVD_H'
|
|
fi
|
|
echores "$_dvd"
|
|
|
|
|
|
echocheck "BSDI dvd.h"
|
|
cat > $TMPC << EOF
|
|
#include <dvd.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_bsdi_dvd=no
|
|
cc_check && _bsdi_dvd=yes
|
|
if test "$_bsdi_dvd" = yes ; then
|
|
_def_bsdi_dvd='#define DVD_STRUCT_IN_BSDI_DVDIOCTL_DVD_H 1'
|
|
else
|
|
_def_bsdi_dvd='#undef DVD_STRUCT_IN_BSDI_DVDIOCTL_DVD_H'
|
|
fi
|
|
echores "$_bsdi_dvd"
|
|
|
|
|
|
echocheck "HPUX SCSI header"
|
|
cat > $TMPC << EOF
|
|
#include <sys/scsi.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_hpux_scsi_h=no
|
|
cc_check && _hpux_scsi_h=yes
|
|
if test "$_hpux_scsi_h" = yes ; then
|
|
_def_hpux_scsi_h='#define HPUX_SCTL_IO 1'
|
|
else
|
|
_def_hpux_scsi_h='#undef HPUX_SCTL_IO'
|
|
fi
|
|
echores "$_hpux_scsi_h"
|
|
|
|
|
|
echocheck "userspace SCSI headers (Solaris)"
|
|
cat > $TMPC << EOF
|
|
# include <unistd.h>
|
|
# include <stropts.h>
|
|
# include <sys/scsi/scsi_types.h>
|
|
# include <sys/scsi/impl/uscsi.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_sol_scsi_h=no
|
|
cc_check && _sol_scsi_h=yes
|
|
if test "$_sol_scsi_h" = yes ; then
|
|
_def_sol_scsi_h='#define SOLARIS_USCSI 1'
|
|
else
|
|
_def_sol_scsi_h='#undef SOLARIS_USCSI'
|
|
fi
|
|
echores "$_sol_scsi_h"
|
|
|
|
|
|
echocheck "termcap"
|
|
if test "$_termcap" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_termcap=no
|
|
cc_check -ltermcap && _termcap=yes && _ld_termcap='-ltermcap'
|
|
cc_check -ltinfo && _termcap=yes && _ld_termcap='-ltinfo'
|
|
fi
|
|
if test "$_termcap" = yes ; then
|
|
_def_termcap='#define USE_TERMCAP 1'
|
|
echores "yes (using $_ld_termcap)"
|
|
else
|
|
_def_termcap='#undef USE_TERMCAP'
|
|
echores no
|
|
fi
|
|
|
|
|
|
echocheck "termios"
|
|
if test "$_termios" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <sys/termios.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_termios=auto
|
|
cc_check && _termios=yes
|
|
_def_termios_h_name='sys/termios.h'
|
|
fi
|
|
# second test:
|
|
if test "$_termios" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <termios.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_termios=no
|
|
cc_check && _termios=yes
|
|
_def_termios_h_name='termios.h'
|
|
fi
|
|
|
|
if test "$_termios" = yes ; then
|
|
_def_termios='#define HAVE_TERMIOS 1'
|
|
_def_termios_h='#undef HAVE_TERMIOS_H'
|
|
_def_termios_sys_h='#undef HAVE_SYS_TERMIOS_H'
|
|
|
|
if test "$_def_termios_h_name" = 'sys/termios.h' ; then
|
|
_def_termios_sys_h='#define HAVE_SYS_TERMIOS_H 1'
|
|
elif test "$_def_termios_h_name" = 'termios.h' ; then
|
|
_def_termios_h='#define HAVE_TERMIOS_H 1'
|
|
fi
|
|
echores "yes (using $_def_termios_h_name)"
|
|
else
|
|
_def_termios='#undef HAVE_TERMIOS'
|
|
_def_termios_h_name=''
|
|
echores "no"
|
|
fi
|
|
|
|
|
|
echocheck "shm"
|
|
if test "$_shm" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/shm.h>
|
|
int main(void) { shmget(0, 0, 0); shmat(0, 0, 0); shmctl(0, 0, 0); return 0; }
|
|
EOF
|
|
_shm=no
|
|
cc_check && _shm=yes
|
|
fi
|
|
if test "$_shm" = yes ; then
|
|
_def_shm='#define HAVE_SHM 1'
|
|
else
|
|
_def_shm='#undef HAVE_SHM'
|
|
fi
|
|
echores "$_shm"
|
|
|
|
|
|
# XXX: FIXME, add runtime checking
|
|
echocheck "linux devfs"
|
|
echores "$_linux_devfs"
|
|
|
|
|
|
echocheck "scandir()"
|
|
cat > $TMPC << EOF
|
|
int main (void) { scandir("", 0, 0, 0); alphasort(0, 0); return 0; }
|
|
EOF
|
|
_scandir=no
|
|
cc_check && _scandir=yes
|
|
if test "$_scandir" = yes ; then
|
|
_def_scandir='#define HAVE_SCANDIR 1'
|
|
else
|
|
_def_scandir='#undef HAVE_SCANDIR'
|
|
fi
|
|
echores "$_scandir"
|
|
|
|
|
|
echocheck "strsep()"
|
|
cat > $TMPC << EOF
|
|
#include <string.h>
|
|
int main (void) { char *s = "Hello, world!"; (void) strsep(&s, ","); return 0; }
|
|
EOF
|
|
_strsep=no
|
|
cc_check && _strsep=yes
|
|
if test "$_strsep" = yes ; then
|
|
_def_strsep='#define HAVE_STRSEP 1'
|
|
else
|
|
_def_strsep='#undef HAVE_STRSEP'
|
|
fi
|
|
echores "$_strsep"
|
|
|
|
echocheck "strlcpy()"
|
|
cat > $TMPC << EOF
|
|
#include <string.h>
|
|
int main (void) { char *s = "Hello, world!", t[20]; (void) strlcpy(t, s, sizeof( t )); return 0; }
|
|
EOF
|
|
_strlcpy=no
|
|
cc_check && _strlcpy=yes
|
|
if test "$_strlcpy" = yes ; then
|
|
_def_strlcpy='#define HAVE_STRLCPY 1'
|
|
else
|
|
_def_strlcpy='#undef HAVE_STRLCPY'
|
|
fi
|
|
echores "$_strlcpy"
|
|
|
|
echocheck "strlcat()"
|
|
cat > $TMPC << EOF
|
|
#include <string.h>
|
|
int main (void) { char *s = "Hello, world!", t[20]; (void) strlcat(t, s, sizeof( t )); return 0; }
|
|
EOF
|
|
_strlcat=no
|
|
cc_check && _strlcat=yes
|
|
if test "$_strlcat" = yes ; then
|
|
_def_strlcat='#define HAVE_STRLCAT 1'
|
|
else
|
|
_def_strlcat='#undef HAVE_STRLCAT'
|
|
fi
|
|
echores "$_strlcat"
|
|
|
|
echocheck "fseeko()"
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
int main (void) { int i; i = fseeko(stdin, 0, 0); return 0; }
|
|
EOF
|
|
_fseeko=no
|
|
cc_check && _fseeko=yes
|
|
if test "$_fseeko" = yes ; then
|
|
_def_fseeko='#define HAVE_FSEEKO 1'
|
|
else
|
|
_def_fseeko='#undef HAVE_FSEEKO'
|
|
fi
|
|
echores "$_fseeko"
|
|
|
|
echocheck "localtime_r()"
|
|
cat > $TMPC << EOF
|
|
#include <time.h>
|
|
int main( void ) { localtime_r(NULL, NULL); }
|
|
EOF
|
|
_localtime_r=no
|
|
cc_check && _localtime_r=yes
|
|
if test "$_localtime_r" = yes ; then
|
|
_def_localtime_r='#define HAVE_LOCALTIME_R 1'
|
|
else
|
|
_def_localtime_r='#undef HAVE_LOCALTIME_R'
|
|
fi
|
|
echores "$_localtime_r"
|
|
|
|
echocheck "vsscanf()"
|
|
cat > $TMPC << EOF
|
|
#include <stdarg.h>
|
|
int main(void) { vsscanf(0, 0, 0); return 0; }
|
|
EOF
|
|
_vsscanf=no
|
|
cc_check && _vsscanf=yes
|
|
if test "$_vsscanf" = yes ; then
|
|
_def_vsscanf='#define HAVE_VSSCANF 1'
|
|
else
|
|
_def_vsscanf='#undef HAVE_VSSCANF'
|
|
fi
|
|
echores "$_vsscanf"
|
|
|
|
|
|
echocheck "swab()"
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
int main(void) { swab(0, 0, 0); return 0; }
|
|
EOF
|
|
_swab=no
|
|
cc_check && _swab=yes
|
|
if test "$_swab" = yes ; then
|
|
_def_swab='#define HAVE_SWAB 1'
|
|
else
|
|
_def_swab='#undef HAVE_SWAB'
|
|
fi
|
|
echores "$_swab"
|
|
|
|
echocheck "posix select()"
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <sys/types.h>
|
|
#include <string.h>
|
|
#include <sys/time.h>
|
|
#include <unistd.h>
|
|
int main(void) {int nfds = 1; fd_set readfds; struct timeval timeout; select(nfds,&readfds,NULL,NULL,&timeout); return 0; }
|
|
EOF
|
|
_posix_select=no
|
|
cc_check && _posix_select=yes
|
|
if test "$_posix_select" = no ; then
|
|
_def_no_posix_select='#define HAVE_NO_POSIX_SELECT 1'
|
|
else
|
|
_def_no_posix_select='#undef HAVE_NO_POSIX_SELECT'
|
|
fi
|
|
echores "$_posix_select"
|
|
|
|
|
|
echocheck "gettimeofday()"
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <sys/time.h>
|
|
int main(void) {struct timeval tv_start; gettimeofday(&tv_start, NULL); return 0; }
|
|
EOF
|
|
_gettimeofday=no
|
|
cc_check && _gettimeofday=yes
|
|
if test "$_gettimeofday" = yes ; then
|
|
_def_gettimeofday='#define HAVE_GETTIMEOFDAY 1'
|
|
else
|
|
_def_gettimeofday='#undef HAVE_GETTIMEOFDAY'
|
|
fi
|
|
echores "$_gettimeofday"
|
|
|
|
|
|
echocheck "glob()"
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <glob.h>
|
|
int main(void) { glob_t gg; glob("filename",0,NULL,&gg); return 0; }
|
|
EOF
|
|
_glob=no
|
|
cc_check && _glob=yes
|
|
if test "$_glob" = yes ; then
|
|
_def_glob='#define HAVE_GLOB 1'
|
|
else
|
|
_def_glob='#undef HAVE_GLOB'
|
|
fi
|
|
echores "$_glob"
|
|
|
|
|
|
echocheck "sys/sysinfo.h"
|
|
cat > $TMPC << EOF
|
|
#include <sys/sysinfo.h>
|
|
int main(void) {
|
|
struct sysinfo s_info;
|
|
sysinfo(&s_info);
|
|
return 0;
|
|
}
|
|
EOF
|
|
_sys_sysinfo=no
|
|
cc_check && _sys_sysinfo=yes
|
|
if test "$_sys_sysinfo" = yes ; then
|
|
_def_sys_sysinfo='#define HAVE_SYS_SYSINFO_H 1'
|
|
_inc_sysinfo='#include <sys/sysinfo.h>'
|
|
else
|
|
_def_sys_sysinfo='#undef HAVE_SYS_SYSINFO_H'
|
|
fi
|
|
echores "$_sys_sysinfo"
|
|
|
|
|
|
echocheck "Mac OS X APIs"
|
|
if test "$_macosx" = auto ; then
|
|
if darwin && ppc; then
|
|
_macosx=yes
|
|
else
|
|
_macosx=no
|
|
_def_macosx='#undef MACOSX'
|
|
_noaomodules="macosx $_noaomodules"
|
|
_novomodules="quartz $_novomodules"
|
|
fi
|
|
fi
|
|
if test "$_macosx" = yes ; then
|
|
cat > $TMPC <<EOF
|
|
#include <Carbon/Carbon.h>
|
|
#include <QuickTime/QuickTime.h>
|
|
#include <CoreAudio/CoreAudio.h>
|
|
int main(void) {
|
|
EnterMovies();
|
|
ExitMovies();
|
|
CFRunLoopRunInMode(kCFRunLoopDefaultMode, 0, false);
|
|
}
|
|
EOF
|
|
if cc_check -framework Carbon -framework QuickTime -framework CoreAudio; then
|
|
_macosx=yes
|
|
_macosx_frameworks="-framework Carbon -framework QuickTime -framework CoreAudio -framework AudioUnit -framework AudioToolbox"
|
|
_def_macosx='#define MACOSX 1'
|
|
_aosrc="$_aosrc ao_macosx.c"
|
|
_aomodules="macosx $_aomodules"
|
|
_vosrc="$_vosrc vo_quartz.c"
|
|
_vomodules="quartz $_vomodules"
|
|
else
|
|
_macosx=no
|
|
_def_macosx='#undef MACOSX'
|
|
_noaomodules="macosx $_noaomodules"
|
|
_novomodules="quartz $_novomodules"
|
|
fi
|
|
cat > $TMPC <<EOF
|
|
#include <Carbon/Carbon.h>
|
|
#include <QuartzCore/CoreVideo.h>
|
|
int main(void) {}
|
|
EOF
|
|
if cc_check -framework Carbon -framework QuartzCore -framework OpenGL; then
|
|
_vosrc="$_vosrc vo_macosx.m"
|
|
_vomodules="macosx $_vomodules"
|
|
_macosx_frameworks="$_macosx_frameworks -framework Cocoa -framework QuartzCore -framework OpenGL"
|
|
_def_macosx_corevideo='#define MACOSX_COREVIDEO 1'
|
|
_macosx_corevideo=yes
|
|
else
|
|
_novomodules="macosx $_novomodules"
|
|
_def_macosx_corevideo='#undef MACOSX_COREVIDEO'
|
|
_macosx_corevideo=no
|
|
fi
|
|
fi
|
|
echores "$_macosx"
|
|
|
|
echocheck "Mac OS X Finder Support"
|
|
if test "$_macosx_finder_support" = auto ; then
|
|
_macosx_finder_support=$_macosx
|
|
fi
|
|
if test "$_macosx_finder_support" = yes; then
|
|
_def_macosx_finder_support='#define MACOSX_FINDER_SUPPORT 1'
|
|
_macosx_finder_support=yes
|
|
else
|
|
_def_macosx_finder_support='#undef MACOSX_FINDER_SUPPORT'
|
|
_macosx_finder_support=no
|
|
fi
|
|
echores "$_macosx_finder_support"
|
|
|
|
echocheck "Mac OS X Bundle file locations"
|
|
if test "$_macosx_bundle" = auto ; then
|
|
_macosx_bundle=$_macosx_finder_support
|
|
fi
|
|
if test "$_macosx_bundle" = yes; then
|
|
_def_macosx_bundle='#define MACOSX_BUNDLE 1'
|
|
else
|
|
_def_macosx_bundle='#undef MACOSX_BUNDLE'
|
|
_macosx_bundle=no
|
|
fi
|
|
echores "$_macosx_bundle"
|
|
|
|
echocheck "Samba support (libsmbclient)"
|
|
if test "$_smbsupport" = yes; then
|
|
_ld_smb="-lsmbclient"
|
|
fi
|
|
if test "$_smbsupport" = auto; then
|
|
_smbsupport=no
|
|
cat > $TMPC << EOF
|
|
#include <libsmbclient.h>
|
|
int main(void) { smbc_opendir("smb://"); return 0; }
|
|
EOF
|
|
if cc_check -lsmbclient ; then
|
|
_smbsupport=yes
|
|
_ld_smb="-lsmbclient"
|
|
else
|
|
if cc_check -lsmbclient $_ld_dl ; then
|
|
_smbsupport=yes
|
|
_ld_smb="-lsmbclient $_ld_dl"
|
|
else
|
|
if cc_check -lsmbclient $_ld_dl -lnsl ; then
|
|
_smbsupport=yes
|
|
_ld_smb="-lsmbclient $_ld_dl -lnsl"
|
|
else
|
|
if cc_check -lsmbclient $_ld_dl -lssl -lnsl ; then
|
|
_smbsupport=yes
|
|
_ld_smb="-lsmbclient $_ld_dl -lssl -lnsl"
|
|
fi
|
|
fi
|
|
fi
|
|
fi
|
|
fi
|
|
|
|
if test "$_smbsupport" = yes; then
|
|
_def_smbsupport="#define LIBSMBCLIENT"
|
|
_inputmodules="smb $_inputmodules"
|
|
else
|
|
_def_smbsupport="#undef LIBSMBCLIENT"
|
|
_noinputmodules="smb $_noinputmodules"
|
|
fi
|
|
echores "$_smbsupport"
|
|
|
|
|
|
#########
|
|
# VIDEO #
|
|
#########
|
|
|
|
|
|
echocheck "3dfx"
|
|
if test "$_3dfx" = yes ; then
|
|
_def_3dfx='#define HAVE_3DFX 1'
|
|
_vosrc="$_vosrc vo_3dfx.c"
|
|
_vomodules="3dfx $_vomodules"
|
|
else
|
|
_def_3dfx='#undef HAVE_3DFX'
|
|
_novomodules="3dfx $_novomodules"
|
|
fi
|
|
echores "$_3dfx"
|
|
|
|
|
|
echocheck "tdfxfb"
|
|
if test "$_tdfxfb" = yes ; then
|
|
_def_tdfxfb='#define HAVE_TDFXFB 1'
|
|
_vosrc="$_vosrc vo_tdfxfb.c"
|
|
_vomodules="tdfxfb $_vomodules"
|
|
else
|
|
_def_tdfxfb='#undef HAVE_TDFXFB'
|
|
_novomodules="tdfxfb $_novomodules"
|
|
fi
|
|
echores "$_tdfxfb"
|
|
|
|
echocheck "tdfxvid"
|
|
if test "$_tdfxvid" = yes ; then
|
|
_def_tdfxvid='#define HAVE_TDFX_VID 1'
|
|
_vosrc="$_vosrc vo_tdfx_vid.c"
|
|
_vomodules="tdfx_vid $_vomodules"
|
|
else
|
|
_def_tdfxvid='#undef HAVE_TDFX_VID'
|
|
_novomodules="tdfx_vid $_novomodules"
|
|
fi
|
|
echores "$_tdfxfb"
|
|
|
|
echocheck "tga"
|
|
if test "$_tga" = yes ; then
|
|
_def_tga='#define HAVE_TGA 1'
|
|
_vosrc="$_vosrc vo_tga.c"
|
|
_vomodules="tga $_vomodules"
|
|
else
|
|
_def_tga='#undef HAVE_TGA'
|
|
_novomodules="tga $_novomodules"
|
|
fi
|
|
echores "$_tga"
|
|
|
|
echocheck "DirectFB headers presence"
|
|
if test -z "$_inc_directfb" ; then
|
|
for I in /usr/include /usr/local/include $_inc_extra; do
|
|
if test -d "$I/directfb" && test -f "$I/directfb/directfb.h" ; then
|
|
_inc_directfb="-I$I/directfb"
|
|
echores "yes (using $_inc_directfb)"
|
|
break
|
|
fi
|
|
if test -d "$I" && test -f "$I/directfb.h" ; then
|
|
_inc_directfb="-I$I"
|
|
echores "yes (using $_inc_directfb)"
|
|
break
|
|
fi
|
|
done
|
|
if test -z "$_inc_directfb" ; then
|
|
_directfb=no
|
|
echores "not found"
|
|
fi
|
|
else
|
|
echores "yes (using $_inc_directfb)"
|
|
fi
|
|
if test "$_inc_directfb" = "-I/usr/include" ; then
|
|
_inc_directfb=""
|
|
fi
|
|
|
|
echocheck "DirectFB"
|
|
if test "$_directfb" = auto ; then
|
|
_directfb=no
|
|
cat > $TMPC <<EOF
|
|
#include <directfb.h>
|
|
int main(void) { IDirectFB *foo; return 0; }
|
|
EOF
|
|
linux && test -c /dev/fb0 && cc_check $_inc_directfb -ldirectfb && _directfb=yes
|
|
fi
|
|
|
|
if test "$_directfb" = yes; then
|
|
cat > $TMPC <<EOF
|
|
#include <directfb.h>
|
|
int main(void) {
|
|
printf ("%i",(directfb_major_version*100+directfb_minor_version)*100+directfb_micro_version);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if cc_check $_inc_directfb -ldirectfb && "$TMPO" >> "$TMPLOG" ; then
|
|
_directfb_version=`"$TMPO"`
|
|
_def_directfb_version="#define DIRECTFBVERSION $_directfb_version"
|
|
if test "$_directfb_version" -ge 913; then
|
|
echores "yes ($_directfb_version)"
|
|
else
|
|
_def_directfb_version='#undef DIRECTFBVERSION'
|
|
_directfb=no
|
|
echores "no (version >=0.9.13 required)"
|
|
fi
|
|
else
|
|
_directfb=no
|
|
echores "no (failed to get version)"
|
|
fi
|
|
else
|
|
echores "$_directfb"
|
|
fi
|
|
|
|
if test "$_directfb" = yes ; then
|
|
_def_directfb='#define HAVE_DIRECTFB 1'
|
|
if test "$_directfb_version" -ge 913; then
|
|
_vosrc="$_vosrc vo_directfb2.c"
|
|
_vomodules="directfb $_vomodules"
|
|
_ld_directfb='-ldirectfb'
|
|
fi
|
|
|
|
if test "$_directfb_version" -ge 915; then
|
|
_vosrc="$_vosrc vo_dfbmga.c"
|
|
_vomodules="dfbmga $_vomodules"
|
|
fi
|
|
else
|
|
_def_directfb='#undef HAVE_DIRECTFB'
|
|
_novomodules="directfb $_novomodules"
|
|
_inc_directfb=""
|
|
fi
|
|
|
|
|
|
echocheck "X11 headers presence"
|
|
if test -z "$_inc_x11" ; then
|
|
for I in /usr/X11/include /usr/X11R6/include /usr/include/X11R6 /usr/include /usr/openwin/include ; do
|
|
if test -d "$I/X11" && test -f "$I/X11/Xlib.h" ; then
|
|
_inc_x11="-I$I"
|
|
echores "yes (using $I)"
|
|
break
|
|
fi
|
|
done
|
|
if test -z "$_inc_x11" ; then
|
|
_x11=no
|
|
echores "not found (check if the dev(el) packages are installed)"
|
|
fi
|
|
else
|
|
echores "yes (using $_inc_x11)"
|
|
fi
|
|
if test "$_inc_x11" = "-I/usr/include" ; then
|
|
_inc_x11=""
|
|
fi
|
|
|
|
|
|
echocheck "X11"
|
|
if test "$_x11" != no ; then
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/Xutil.h>
|
|
int main(void) { (void) XCreateWindow(0,0,0,0,0,0,0,0,0,0,0,0); return 0; }
|
|
EOF
|
|
if test -z "$_x11_paths" ; then
|
|
_x11_paths="/usr/X11R6/lib /usr/lib/X11R6 /usr/X11/lib /usr/lib32 /usr/openwin/lib /usr/X11R6/lib64 /usr/lib"
|
|
fi
|
|
for I in $_x11_paths ; do
|
|
_ld_x11="-L$I -lXext -lX11 $_ld_sock"
|
|
if netbsd; then
|
|
_ld_x11="$_ld_x11 -Wl,-R$I"
|
|
fi
|
|
if test -d "$I" && cc_check $_inc_x11 $_ld_x11 ; then
|
|
_x11=yes
|
|
break
|
|
fi
|
|
done
|
|
fi
|
|
if test "$_x11" = yes ; then
|
|
_def_x11='#define HAVE_X11 1'
|
|
_vosrc="$_vosrc x11_common.c vo_x11.c vo_xover.c"
|
|
_vomodules="x11 xover $_vomodules"
|
|
echores "yes (using $I)"
|
|
else
|
|
_x11=no
|
|
_def_x11='#undef HAVE_X11'
|
|
_inc_x11=''
|
|
_ld_x11=''
|
|
_novomodules="x11 $_novomodules"
|
|
echores "no (check if the dev(el) packages are installed)"
|
|
fi
|
|
|
|
|
|
echocheck "DPMS"
|
|
_xdpms3=no
|
|
if test "$_x11" = yes ; then
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xmd.h>
|
|
#include <X11/Xlib.h>
|
|
#include <X11/Xutil.h>
|
|
#include <X11/Xatom.h>
|
|
#include <X11/extensions/dpms.h>
|
|
int main(void) {
|
|
(void) DPMSQueryExtension(0, 0, 0);
|
|
}
|
|
EOF
|
|
cc_check $_inc_x11 -lXdpms $_ld_x11 && _xdpms3=yes
|
|
fi
|
|
_xdpms4=no
|
|
if test "$_x11" = yes ; then
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/extensions/dpms.h>
|
|
int main(void) {
|
|
(void) DPMSQueryExtension(0, 0, 0);
|
|
}
|
|
EOF
|
|
cc_check $_inc_x11 $_ld_x11 && _xdpms4=yes
|
|
fi
|
|
if test "$_xdpms4" = yes ; then
|
|
_def_xdpms='#define HAVE_XDPMS 1'
|
|
echores "yes (using Xdpms 4)"
|
|
elif test "$_xdpms3" = yes ; then
|
|
_def_xdpms='#define HAVE_XDPMS 1'
|
|
_ld_x11="-lXdpms $_ld_x11"
|
|
echores "yes (using Xdpms 3)"
|
|
else
|
|
_def_xdpms='#undef HAVE_XDPMS'
|
|
echores "no"
|
|
fi
|
|
|
|
|
|
echocheck "Xv"
|
|
if test "$_x11" = yes && test "$_xv" != no ; then
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/extensions/Xvlib.h>
|
|
int main(void) {
|
|
(void) XvGetPortAttribute(0, 0, 0, 0);
|
|
(void) XvQueryPortAttributes(0, 0, 0);
|
|
return 0; }
|
|
EOF
|
|
_xv=no
|
|
cc_check $_inc_x11 -lXv $_ld_x11 && _xv=yes
|
|
else
|
|
_xv=no
|
|
fi
|
|
if test "$_xv" = yes ; then
|
|
_def_xv='#define HAVE_XV 1'
|
|
_ld_xv='-lXv'
|
|
_vosrc="$_vosrc vo_xv.c"
|
|
_vomodules="xv $_vomodules"
|
|
else
|
|
_def_xv='#undef HAVE_XV'
|
|
_novomodules="xv $_novomodules"
|
|
fi
|
|
echores "$_xv"
|
|
|
|
|
|
echocheck "XvMC"
|
|
if test "$_x11" = yes && test "$_xv" = yes && test "$_xvmc" != no ; then
|
|
_xvmc=no
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/extensions/Xvlib.h>
|
|
#include <X11/extensions/XvMClib.h>
|
|
int main(void) {
|
|
(void) XvMCQueryExtension(0,0,0);
|
|
(void) XvMCCreateContext(0,0,0,0,0,0,0);
|
|
return 0; }
|
|
EOF
|
|
cc_check $_inc_x11 -lXvMC -l$_xvmclib $_ld_xv $_ld_x11 && _xvmc=yes
|
|
fi
|
|
if test "$_xvmc" = yes ; then
|
|
_def_xvmc='#define HAVE_XVMC 1'
|
|
_ld_xvmc="-lXvMC -l$_xvmclib"
|
|
_vosrc="$_vosrc vo_xvmc.c"
|
|
_vomodules="xvmc $_vomodules"
|
|
else
|
|
_def_xvmc='#undef HAVE_XVMC'
|
|
_novomodules="xvmc $_novomodules"
|
|
fi
|
|
echores "$_xvmc"
|
|
|
|
|
|
echocheck "Xinerama"
|
|
if test "$_x11" = yes && test "$_xinerama" != no ; then
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/extensions/Xinerama.h>
|
|
int main(void) { (void) XineramaIsActive(0); return 0; }
|
|
EOF
|
|
_xinerama=no
|
|
cc_check $_inc_x11 -lXinerama $_ld_x11 && _xinerama=yes
|
|
else
|
|
_xinerama=no
|
|
fi
|
|
if test "$_xinerama" = yes ; then
|
|
_def_xinerama='#define HAVE_XINERAMA 1'
|
|
_ld_xinerama='-lXinerama'
|
|
else
|
|
_def_xinerama='#undef HAVE_XINERAMA'
|
|
fi
|
|
echores "$_xinerama"
|
|
|
|
|
|
# Note: the -lXxf86vm library is the VideoMode extension and though it's not
|
|
# needed for DGA, AFAIK every distribution packages together with DGA stuffs
|
|
# named 'X extensions' or something similar.
|
|
# This check may be useful for future mplayer versions (to change resolution)
|
|
# If you run into problems, remove '-lXxf86vm'.
|
|
echocheck "Xxf86vm"
|
|
if test "$_x11" = yes && test "$_vm" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/extensions/xf86vmode.h>
|
|
int main(void) { (void) XF86VidModeQueryExtension(0, 0, 0); return 0; }
|
|
EOF
|
|
_vm=no
|
|
cc_check $_inc_x11 -lXxf86vm $_ld_x11 && _vm=yes
|
|
fi
|
|
if test "$_vm" = yes ; then
|
|
_def_vm='#define HAVE_XF86VM 1'
|
|
_ld_vm='-lXxf86vm'
|
|
else
|
|
_def_vm='#undef HAVE_XF86VM'
|
|
fi
|
|
echores "$_vm"
|
|
|
|
# Check for the presence of special keycodes, like audio control buttons
|
|
# that XFree86 might have. Used to be bundled with the xf86vm check, but
|
|
# has nothing to do with xf86vm and XFree 3.x has xf86vm but does NOT
|
|
# have these new keycodes.
|
|
echocheck "XF86keysym"
|
|
if test "$_xf86keysym" = auto; then
|
|
_xf86keysym=no
|
|
if test "$_x11" = yes ; then
|
|
cat > $TMPC <<EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/XF86keysym.h>
|
|
int main(void) { return XF86XK_AudioPause; }
|
|
EOF
|
|
cc_check $_inc_x11 $_ld_x11 && _xf86keysym=yes
|
|
fi
|
|
fi
|
|
if test "$_xf86keysym" = yes ; then
|
|
_def_xf86keysym='#define HAVE_XF86XK 1'
|
|
else
|
|
_def_xf86keysym='#undef HAVE_XF86XK'
|
|
fi
|
|
echores "$_xf86keysym"
|
|
|
|
echocheck "DGA"
|
|
if test "$_x11" = no ; then
|
|
_dga=no
|
|
fi
|
|
# Version 2 is preferred to version 1 if available
|
|
if test "$_dga" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/extensions/xf86dga.h>
|
|
int main (void) { (void) XF86DGASetViewPort(0, 0, 0, 0); return 0; }
|
|
EOF
|
|
_dga=no
|
|
cc_check $_inc_x11 -lXxf86dga -lXxf86vm $_ld_x11 && _dga=1
|
|
|
|
cat > $TMPC << EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/extensions/xf86dga.h>
|
|
int main (void) { (void) XDGASetViewport(0, 0, 0, 0, 0); return 0; }
|
|
EOF
|
|
cc_check $_inc_x11 -lXxf86dga $_ld_x11 && _dga=2
|
|
fi
|
|
|
|
_def_dga='#undef HAVE_DGA'
|
|
_def_dga2='#undef HAVE_DGA2'
|
|
if test "$_dga" = 1 ; then
|
|
_def_dga='#define HAVE_DGA 1'
|
|
_ld_dga='-lXxf86dga'
|
|
_vosrc="$_vosrc vo_dga.c"
|
|
_vomodules="dga $_vomodules"
|
|
echores "yes (using DGA 1.0)"
|
|
elif test "$_dga" = 2 ; then
|
|
_def_dga='#define HAVE_DGA 1'
|
|
_def_dga2='#define HAVE_DGA2 1'
|
|
_ld_dga='-lXxf86dga'
|
|
_vosrc="$_vosrc vo_dga.c"
|
|
_vomodules="dga $_vomodules"
|
|
echores "yes (using DGA 2.0)"
|
|
elif test "$_dga" = no ; then
|
|
echores "no"
|
|
_novomodules="dga $_novomodules"
|
|
else
|
|
die "DGA version must be 1 or 2"
|
|
fi
|
|
|
|
|
|
echocheck "OpenGL"
|
|
#Note: this test is run even with --enable-gl since we autodetect $_ld_gl
|
|
if (test "$_x11" = yes || win32) && test "$_gl" != no ; then
|
|
cat > $TMPC << EOF
|
|
#include <GL/gl.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_gl=no
|
|
if cc_check $_inc_x11 $_ld_x11 -lGL $_ld_lm ; then
|
|
_gl=yes
|
|
_ld_gl="-lGL"
|
|
elif cc_check $_inc_x11 $_ld_x11 -lGL $_ld_lm $_ld_pthread ; then
|
|
_gl=yes
|
|
_ld_gl="-lGL $_ld_pthread"
|
|
elif cc_check -lopengl32 ; then
|
|
_gl=yes
|
|
_gl_win32=yes
|
|
_ld_gl="-lopengl32 -lgdi32"
|
|
fi
|
|
else
|
|
_gl=no
|
|
fi
|
|
if test "$_gl" = yes ; then
|
|
_def_gl='#define HAVE_GL 1'
|
|
if test "$_gl_win32" = yes ; then
|
|
_def_gl_win32='#define GL_WIN32 1'
|
|
_vosrc="$_vosrc vo_gl2.c w32_common.c gl_common.c"
|
|
else
|
|
_vosrc="$_vosrc vo_gl.c vo_gl2.c gl_common.c"
|
|
fi
|
|
_vomodules="opengl $_vomodules"
|
|
else
|
|
_def_gl='#undef HAVE_GL'
|
|
_def_gl_win32='#undef GL_WIN32'
|
|
_novomodules="opengl $_novomodules"
|
|
fi
|
|
echores "$_gl"
|
|
|
|
|
|
echocheck "/dev/mga_vid"
|
|
if test "$_mga" = auto ; then
|
|
_mga=no
|
|
test -c /dev/mga_vid && _mga=yes
|
|
fi
|
|
if test "$_mga" = yes ; then
|
|
_def_mga='#define HAVE_MGA 1'
|
|
_vosrc="$_vosrc vo_mga.c"
|
|
_vomodules="mga $_vomodules"
|
|
else
|
|
_def_mga='#undef HAVE_MGA'
|
|
_novomodules="mga $_novomodules"
|
|
fi
|
|
echores "$_mga"
|
|
|
|
|
|
# echocheck "syncfb"
|
|
# _syncfb=no
|
|
# test "$_mga" = yes && _syncfb=yes
|
|
# if test "$_syncfb" = yes ; then
|
|
# _def_syncfb='#define HAVE_SYNCFB 1'
|
|
# _vosrc="$_vosrc vo_syncfb.c"
|
|
# else
|
|
# _def_syncfb='#undef HAVE_SYNCFB'
|
|
# fi
|
|
# echores "$_syncfb"
|
|
|
|
|
|
echocheck "xmga"
|
|
if test "$_xmga" = auto ; then
|
|
_xmga=no
|
|
test "$_x11" = yes && test "$_mga" = yes && _xmga=yes
|
|
fi
|
|
if test "$_xmga" = yes ; then
|
|
_def_xmga='#define HAVE_XMGA 1'
|
|
_vosrc="$_vosrc vo_xmga.c"
|
|
_vomodules="xmga $_vomodules"
|
|
else
|
|
_def_xmga='#undef HAVE_XMGA'
|
|
_novomodules="xmga $_novomodules"
|
|
fi
|
|
echores "$_xmga"
|
|
|
|
|
|
echocheck "GGI"
|
|
if test "$_ggi" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <ggi/ggi.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_ggi=no
|
|
cc_check -lggi && _ggi=yes
|
|
fi
|
|
if test "$_ggi" = yes ; then
|
|
_def_ggi='#define HAVE_GGI 1'
|
|
_ld_ggi='-lggi'
|
|
_vosrc="$_vosrc vo_ggi.c"
|
|
_vomodules="ggi $_vomodules"
|
|
else
|
|
_def_ggi='#undef HAVE_GGI'
|
|
_novomodules="ggi $_novomodules"
|
|
fi
|
|
echores "$_ggi"
|
|
|
|
echocheck "GGI extension: libggiwmh"
|
|
if test "$_ggiwmh" = auto ; then
|
|
_ggiwmh=no
|
|
cat > $TMPC << EOF
|
|
#include <ggi/ggi.h>
|
|
#include <ggi/wmh.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check -lggi -lggiwmh && _ggiwmh=yes
|
|
fi
|
|
# needed to get right output on obscure combination
|
|
# like --disable-ggi --enable-ggiwmh
|
|
if test "$_ggi" = yes && test "$_ggiwmh" = yes ; then
|
|
_def_ggiwmh='#define HAVE_GGIWMH 1'
|
|
_ld_ggi="$_ld_ggi -lggiwmh"
|
|
else
|
|
_ggiwmh=no
|
|
_def_ggiwmh='#undef HAVE_GGIWMH'
|
|
fi
|
|
echores "$_ggiwmh"
|
|
|
|
|
|
echocheck "AA"
|
|
if test "$_aa" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <aalib.h>
|
|
int main(void) { (void) aa_init(0, 0, 0); return 0; }
|
|
EOF
|
|
_aa=no
|
|
if cc_check -laa ; then
|
|
_aa=yes
|
|
_ld_aa="-laa"
|
|
elif cc_check $_ld_x11 -laa ; then
|
|
_aa=yes
|
|
_ld_aa="$_ld_x11 -laa"
|
|
fi
|
|
fi
|
|
if test "$_aa" = yes ; then
|
|
_def_aa='#define HAVE_AA 1'
|
|
if cygwin ; then
|
|
_ld_aa=`aalib-config --libs | cut -d " " -f 2,5,6`
|
|
fi
|
|
_vosrc="$_vosrc vo_aa.c"
|
|
_vomodules="aa $_vomodules"
|
|
else
|
|
_def_aa='#undef HAVE_AA'
|
|
_novomodules="aa $_novomodules"
|
|
fi
|
|
echores "$_aa"
|
|
|
|
|
|
echocheck "CACA"
|
|
if test "$_caca" = auto ; then
|
|
_caca=no
|
|
if ( caca-config --version ) >> "$TMPLOG" 2>&1 ; then
|
|
cat > $TMPC << EOF
|
|
#include <caca.h>
|
|
int main(void) { (void) caca_init(); return 0; }
|
|
EOF
|
|
cc_check `caca-config --libs` && _caca=yes
|
|
fi
|
|
fi
|
|
if test "$_caca" = yes ; then
|
|
_def_caca='#define HAVE_CACA 1'
|
|
_inc_caca=`caca-config --cflags`
|
|
_ld_caca=`caca-config --libs`
|
|
_vosrc="$_vosrc vo_caca.c"
|
|
_vomodules="caca $_vomodules"
|
|
else
|
|
_def_caca='#undef HAVE_CACA'
|
|
_novomodules="caca $_novomodules"
|
|
fi
|
|
echores "$_caca"
|
|
|
|
|
|
echocheck "SVGAlib"
|
|
if test "$_svga" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <vga.h>
|
|
#include <vgagl.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_svga=no
|
|
cc_check -lvgagl -lvga $_ld_lm && _svga=yes
|
|
fi
|
|
if test "$_svga" = yes ; then
|
|
_def_svga='#define HAVE_SVGALIB 1'
|
|
_ld_svga="-lvgagl -lvga $_ld_lm"
|
|
_vosrc="$_vosrc vo_svga.c"
|
|
_vomodules="svga $_vomodules"
|
|
else
|
|
_def_svga='#undef HAVE_SVGALIB'
|
|
_novomodules="svga $_novomodules"
|
|
fi
|
|
echores "$_svga"
|
|
|
|
|
|
echocheck "FBDev"
|
|
if test "$_fbdev" = auto ; then
|
|
_fbdev=no
|
|
linux && test -c /dev/fb0 && _fbdev=yes
|
|
fi
|
|
if test "$_fbdev" = yes ; then
|
|
_def_fbdev='#define HAVE_FBDEV 1'
|
|
_vosrc="$_vosrc vo_fbdev.c vo_fbdev2.c"
|
|
_vomodules="fbdev $_vomodules"
|
|
else
|
|
_def_fbdev='#undef HAVE_FBDEV'
|
|
_novomodules="fbdev $_novomodules"
|
|
fi
|
|
echores "$_fbdev"
|
|
|
|
|
|
|
|
echocheck "DVB"
|
|
if test "$_dvb" != no ; then
|
|
_dvb=no
|
|
cat >$TMPC << EOF
|
|
#include <sys/poll.h>
|
|
#include <sys/ioctl.h>
|
|
#include <stdio.h>
|
|
#include <time.h>
|
|
#include <unistd.h>
|
|
|
|
#include <ost/dmx.h>
|
|
#include <ost/frontend.h>
|
|
#include <ost/sec.h>
|
|
#include <ost/video.h>
|
|
#include <ost/audio.h>
|
|
int main(void) {return 0;}
|
|
EOF
|
|
if cc_check ; then
|
|
_dvb=yes
|
|
echores "yes"
|
|
else
|
|
for I in "$_inc_dvb" "-I/usr/src/DVB/ost/include" ; do
|
|
if cc_check "$I" ; then
|
|
_dvb=yes
|
|
_inc_dvb="$I"
|
|
echores "yes (using $_inc_dvb)"
|
|
break
|
|
fi
|
|
done
|
|
test "$_dvb" = no && echores "no (specify path to DVB/ost/include with --with-dvbincdir=DIR)"
|
|
fi
|
|
else
|
|
echores "no"
|
|
fi
|
|
if test "$_dvb" = yes ; then
|
|
_def_dvb='#define HAVE_DVB 1'
|
|
_def_dvb_in='#define HAS_DVBIN_SUPPORT 1'
|
|
_aomodules="mpegpes(dvb) $_aomodules"
|
|
_vomodules="mpegpes(dvb) $_vomodules"
|
|
fi
|
|
if test "$_dvbhead" != no ; then
|
|
echocheck "DVB HEAD"
|
|
if test "$_dvbhead" != no ; then
|
|
_dvbhead=no
|
|
|
|
cat >$TMPC << EOF
|
|
#include <sys/poll.h>
|
|
#include <sys/ioctl.h>
|
|
#include <stdio.h>
|
|
#include <time.h>
|
|
#include <unistd.h>
|
|
|
|
#include <linux/dvb/dmx.h>
|
|
#include <linux/dvb/frontend.h>
|
|
#include <linux/dvb/video.h>
|
|
#include <linux/dvb/audio.h>
|
|
int main(void) {return 0;}
|
|
EOF
|
|
if cc_check ; then
|
|
_dvbhead=yes
|
|
echores "yes"
|
|
else
|
|
for I in "$_inc_dvb" "-I/usr/src/DVB/include" ; do
|
|
if cc_check "$I" ; then
|
|
_dvbhead=yes
|
|
_inc_dvb="$I"
|
|
echores "yes (using $_inc_dvb)"
|
|
break
|
|
fi
|
|
done
|
|
test "$_dvbhead" = no && echores "no (specify path to DVB/include (HEAD Version) with --with-dvbincdir=DIR)"
|
|
fi
|
|
else
|
|
echores "no"
|
|
fi
|
|
if test "$_dvbhead" = yes ; then
|
|
_def_dvb='#define HAVE_DVB_HEAD 1'
|
|
_def_dvb_in='#define HAS_DVBIN_SUPPORT 1'
|
|
_aomodules="mpegpes(dvb) $_aomodules"
|
|
_vomodules="mpegpes(dvb) $_vomodules"
|
|
fi
|
|
fi
|
|
if test "$_dvbhead" = no && test "$_dvb" = no ; then
|
|
_def_dvb='#undef HAVE_DVB'
|
|
_def_dvb_in='#undef HAS_DVBIN_SUPPORT '
|
|
_aomodules="mpegpes(file) $_aomodules"
|
|
_vomodules="mpegpes(file) $_vomodules"
|
|
fi
|
|
|
|
if test "$_dvb" = yes || test "$_dvbhead" = yes ; then
|
|
_dvbin=yes
|
|
_inputmodules="dvb $_inputmodules"
|
|
else
|
|
_dvbin=no
|
|
_noinputmodules="dvb $_noinputmodules"
|
|
fi
|
|
|
|
echocheck "PNG support"
|
|
if test "$_png" = auto ; then
|
|
_png=no
|
|
if irix ; then
|
|
# Don't check for -lpng on irix since it has its own libpng
|
|
# incompatible with the GNU libpng
|
|
echores "disabled on irix (not GNU libpng)"
|
|
else
|
|
cat > $TMPC << EOF
|
|
#include <png.h>
|
|
#include <string.h>
|
|
int main(void) {
|
|
printf("png.h : %s\n", PNG_LIBPNG_VER_STRING);
|
|
printf("libpng: %s\n", png_libpng_ver);
|
|
return (strcmp(PNG_LIBPNG_VER_STRING, png_libpng_ver));
|
|
}
|
|
EOF
|
|
if cc_check -lpng -lz $_ld_lm ; then
|
|
if "$TMPO" >> "$TMPLOG" ; then
|
|
_png=yes
|
|
echores yes
|
|
else
|
|
echores "no (mismatch of library and header versions)"
|
|
fi
|
|
else
|
|
echores no
|
|
fi
|
|
fi
|
|
else
|
|
echores "$_png"
|
|
fi
|
|
if test "$_png" = yes ; then
|
|
_def_png='#define HAVE_PNG 1'
|
|
_ld_png='-lpng -lz'
|
|
_vosrc="$_vosrc vo_png.c"
|
|
_vomodules="png $_vomodules"
|
|
_mkf_png="yes"
|
|
else
|
|
_def_png='#undef HAVE_PNG'
|
|
_novomodules="png $_novomodules"
|
|
_mkf_png="no"
|
|
fi
|
|
|
|
echocheck "JPEG support"
|
|
if test "$_jpg" = auto ; then
|
|
_jpg=no
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <setjmp.h>
|
|
#include <string.h>
|
|
#include <jpeglib.h>
|
|
int main(void) {
|
|
return 0;
|
|
}
|
|
EOF
|
|
if cc_check -ljpeg $_ld_lm ; then
|
|
if "$TMPO" >> "$TMPLOG" ; then
|
|
_jpg=yes
|
|
fi
|
|
fi
|
|
fi
|
|
echores "$_jpg"
|
|
|
|
if test "$_jpg" = yes ; then
|
|
_def_jpg='#define HAVE_JPEG 1'
|
|
_vosrc="$_vosrc vo_jpeg.c"
|
|
_vomodules="jpeg $_vomodules"
|
|
_ld_jpg="-ljpeg"
|
|
_mkf_jpg="yes"
|
|
else
|
|
_def_jpg='#undef HAVE_JPEG'
|
|
_novomodules="jpeg $_novomodules"
|
|
_mkf_jpg="no"
|
|
fi
|
|
|
|
|
|
|
|
echocheck "PNM support"
|
|
if test "$_pnm" = yes; then
|
|
_def_pnm="#define HAVE_PNM"
|
|
_vosrc="$_vosrc vo_pnm.c"
|
|
_vomodules="pnm $_vomodules"
|
|
else
|
|
_def_pnm="#undef HAVE_PNM"
|
|
_novomodules="pnm $_novomodules"
|
|
fi
|
|
echores "$_pnm"
|
|
|
|
|
|
|
|
echocheck "md5sum support"
|
|
if test "$_md5sum" = yes; then
|
|
_def_md5sum="#define HAVE_MD5SUM"
|
|
_vosrc="$_vosrc vo_md5sum.c md5sum.c"
|
|
_vomodules="md5sum $_vomodules"
|
|
else
|
|
_def_md5sum="#undef HAVE_MD5SUM"
|
|
_novomodules="md5sum $_novomodules"
|
|
fi
|
|
echores "$_md5sum"
|
|
|
|
|
|
|
|
echocheck "GIF support"
|
|
# This is to appease people who want to force gif support.
|
|
# If it is forced to yes, then we still do checks to determine
|
|
# which gif library to use.
|
|
if test "$_gif" = yes ; then
|
|
_force_gif=yes
|
|
_gif=auto
|
|
fi
|
|
|
|
if test "$_gif" = auto ; then
|
|
_gif=no
|
|
cat > $TMPC << EOF
|
|
#include <gif_lib.h>
|
|
int main(void) {
|
|
return 0;
|
|
}
|
|
EOF
|
|
if cc_check -lungif && "$TMPO" >> "$TMPLOG" ; then
|
|
_gif=yes
|
|
_ld_gif="-lungif"
|
|
elif cc_check -lungif $_ld_x11 && "$TMPO" >> "$TMPLOG" ; then
|
|
_gif=yes
|
|
_ld_gif="-lungif $_ld_x11"
|
|
elif cc_check -lgif && "$TMPO" >> "$TMPLOG" ; then
|
|
_gif=yes
|
|
_ld_gif="-lgif"
|
|
elif cc_check -lgif $_ld_x11 && "$TMPO" >> "$TMPLOG" ; then
|
|
_gif=yes
|
|
_ld_gif="-lgif $_ld_x11"
|
|
fi
|
|
fi
|
|
|
|
# If no library was found, and the user wants support forced,
|
|
# then we force it on with libgif, as this is the safest
|
|
# assumption IMHO. (libungif & libregif both create symbolic
|
|
# links to libgif. We also assume that no x11 support is needed,
|
|
# because if you are forcing this, then you _should_ know what
|
|
# you are doing. [ Besides, package maintainers should never
|
|
# have compiled x11 deps into libungif in the first place. ] )
|
|
# </rant>
|
|
# --Joey
|
|
if test "$_force_gif" = yes && test "$_gif" = no ; then
|
|
_gif=yes
|
|
_ld_gif="-lgif"
|
|
fi
|
|
|
|
if test "$_gif" = yes ; then
|
|
_def_gif='#define HAVE_GIF 1'
|
|
_vosrc="$_vosrc vo_gif89a.c"
|
|
_codecmodules="gif $_codecmodules"
|
|
_vomodules="gif89a $_vomodules"
|
|
_mkf_gif="yes"
|
|
_gif="yes (old version, some encoding functions disabled)"
|
|
_def_gif_4='#undef HAVE_GIF_4'
|
|
|
|
cat > $TMPC << EOF
|
|
#include <signal.h>
|
|
#include <gif_lib.h>
|
|
void catch() { exit(1); }
|
|
int main(void) {
|
|
signal(SIGSEGV, catch); // catch segfault
|
|
printf("EGifPutExtensionFirst is at address %p\n", EGifPutExtensionFirst);
|
|
EGifSetGifVersion("89a"); // this will segfault a buggy gif lib.
|
|
return 0;
|
|
}
|
|
EOF
|
|
if cc_check "$_ld_gif" && ( "$TMPO" ) >>"$TMPLOG" 2>&1 ; then
|
|
_def_gif_4='#define HAVE_GIF_4 1'
|
|
_gif="yes"
|
|
fi
|
|
else
|
|
_def_gif='#undef HAVE_GIF'
|
|
_def_gif_4='#undef HAVE_GIF_4'
|
|
_novomodules="gif89a $_novomodules"
|
|
_nocodecmodules="gif $_nocodecmodules"
|
|
_mkf_gif="no"
|
|
fi
|
|
echores "$_gif"
|
|
|
|
|
|
case "$_gif" in yes*)
|
|
echocheck "broken giflib workaround"
|
|
_def_gif_tvt_hack='#define HAVE_GIF_TVT_HACK 1'
|
|
|
|
cat > $TMPC << EOF
|
|
#include <gif_lib.h>
|
|
int main(void) {
|
|
GifFileType gif;
|
|
printf("UserData is at address %p\n", gif.UserData);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if cc_check "$_ld_gif" && ( "$TMPO" ) >>"$TMPLOG" 2>&1 ; then
|
|
_def_gif_tvt_hack='#undef HAVE_GIF_TVT_HACK'
|
|
echores "disabled"
|
|
else
|
|
echores "enabled"
|
|
fi
|
|
;;
|
|
esac
|
|
|
|
|
|
echocheck "VESA support"
|
|
if test "$_vesa" = auto ; then
|
|
if x86 && linux ; then
|
|
_vesa=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/io.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check && _vesa=yes
|
|
fi
|
|
fi
|
|
if test "$_vesa" = yes ; then
|
|
_def_vesa='#define HAVE_VESA 1'
|
|
_vosrc="$_vosrc vo_vesa.c vesa_lvo.c"
|
|
_vomodules="vesa $_vomodules"
|
|
echores "yes"
|
|
else
|
|
_def_vesa='#undef HAVE_VESA'
|
|
echores "no (not supported on this OS/architecture)"
|
|
_novomodules="vesa $_novomodules"
|
|
fi
|
|
|
|
#################
|
|
# VIDEO + AUDIO #
|
|
#################
|
|
|
|
|
|
echocheck "SDL"
|
|
if test -z "$_sdlconfig" ; then
|
|
if ( sdl-config --version ) >>"$TMPLOG" 2>&1 ; then
|
|
_sdlconfig="sdl-config"
|
|
elif ( sdl11-config --version ) >>"$TMPLOG" 2>&1 ; then
|
|
_sdlconfig="sdl11-config"
|
|
else
|
|
_sdlconfig=false
|
|
fi
|
|
fi
|
|
if test "$_sdl" = auto || test "$_sdl" = yes ; then
|
|
cat > $TMPC << EOF
|
|
#include <SDL.h>
|
|
int main(int argc, char *argv[]) { return 0; }
|
|
EOF
|
|
_sdl=no
|
|
if "$_sdlconfig" --version >>"$TMPLOG" 2>&1 ; then
|
|
if cc_check `$_sdlconfig --cflags` `$_sdlconfig --libs` >>"$TMPLOG" 2>&1 ; then
|
|
_sdlversion=`$_sdlconfig --version | sed 's/[^0-9]//g'`
|
|
if test "$_sdlversion" -gt 116 ; then
|
|
if test "$_sdlversion" -lt 121 ; then
|
|
_def_sdlbuggy='#define BUGGY_SDL'
|
|
else
|
|
_def_sdlbuggy='#undef BUGGY_SDL'
|
|
fi
|
|
_sdl=yes
|
|
else
|
|
_sdl=outdated
|
|
fi
|
|
fi
|
|
fi
|
|
fi
|
|
if test "$_sdl" = yes ; then
|
|
_def_sdl='#define HAVE_SDL 1'
|
|
if cygwin ; then
|
|
_ld_sdl=`$_sdlconfig --libs | cut -d " " -f 1,4,6 | sed s/no-cygwin/cygwin/`
|
|
_inc_sdl=`$_sdlconfig --cflags | cut -d " " -f 1,5,6 | sed s/no-cygwin/cygwin/`
|
|
elif mingw32 ; then
|
|
_ld_sdl=`$_sdlconfig --libs | sed s/-mwindows//`
|
|
_inc_sdl=`$_sdlconfig --cflags | sed s/-Dmain=SDL_main//`
|
|
else
|
|
_ld_sdl=`$_sdlconfig --libs`
|
|
_inc_sdl=`$_sdlconfig --cflags`
|
|
fi
|
|
_vosrc="$_vosrc vo_sdl.c"
|
|
_vomodules="sdl $_vomodules"
|
|
_aosrc="$_aosrc ao_sdl.c"
|
|
_aomodules="sdl $_aomodules"
|
|
echores "yes (using $_sdlconfig)"
|
|
else
|
|
_def_sdl='#undef HAVE_SDL'
|
|
_novomodules="sdl $_novomodules"
|
|
_noaomodules="sdl $_noaomodules"
|
|
echores "no"
|
|
fi
|
|
|
|
echocheck "Windows waveout"
|
|
if test "$_win32waveout" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <windows.h>
|
|
#include <mmsystem.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_win32waveout=no
|
|
cc_check -lwinmm && _win32waveout=yes
|
|
fi
|
|
if test "$_win32waveout" = yes ; then
|
|
_def_win32waveout='#define HAVE_WIN32WAVEOUT 1'
|
|
_ld_win32libs="-lwinmm $_ld_win32libs"
|
|
_aosrc="$_aosrc ao_win32.c"
|
|
_aomodules="win32 $_aomodules"
|
|
else
|
|
_def_win32waveout='#undef HAVE_WIN32WAVEOUT'
|
|
_noaomodules="win32 $_noaomodules"
|
|
fi
|
|
echores "$_win32waveout"
|
|
|
|
echocheck "Directx"
|
|
if test "$_directx" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <windows.h>
|
|
#include <ddraw.h>
|
|
#include <dsound.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_directx=no
|
|
cc_check -lgdi32 && _directx=yes
|
|
fi
|
|
if test "$_directx" = yes ; then
|
|
_def_directx='#define HAVE_DIRECTX 1'
|
|
_ld_win32libs="-lgdi32 $_ld_win32libs"
|
|
_vosrc="$_vosrc vo_directx.c"
|
|
_vomodules="directx $_vomodules"
|
|
_aosrc="$_aosrc ao_dsound.c"
|
|
_aomodules="dsound $_aomodules"
|
|
else
|
|
_def_directx='#undef HAVE_DIRECTX'
|
|
_novomodules="directx $_novomodules"
|
|
_noaomodules="dsound $_noaomodules"
|
|
fi
|
|
echores "$_directx"
|
|
|
|
echocheck "NAS"
|
|
if test "$_nas" = auto || test "$_nas" = yes ; then
|
|
cat > $TMPC << EOF
|
|
#include <audio/audiolib.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_nas=no
|
|
cc_check -laudio $_inc_x11 -lXt $_ld_x11 $_ld_lm && _nas=yes
|
|
fi
|
|
if test "$_nas" = yes ; then
|
|
_def_nas='#define HAVE_NAS 1'
|
|
_ld_nas="-laudio -lXt $_ld_x11"
|
|
_aosrc="$_aosrc ao_nas.c"
|
|
_aomodules="nas $_aomodules"
|
|
else
|
|
_noaomodules="nas $_noaomodules"
|
|
_def_nas='#undef HAVE_NAS'
|
|
fi
|
|
echores "$_nas"
|
|
|
|
echocheck "DXR2"
|
|
if test "$_dxr2" = auto; then
|
|
_dxr2=no
|
|
for _inc_dxr2 in "$_inc_dxr2" \
|
|
"-I/usr/local/include/dxr2" \
|
|
"-I/usr/include/dxr2"; do
|
|
cat > $TMPC << EOF
|
|
#include <dxr2ioctl.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check $_inc_dxr2 && _dxr2=yes && break
|
|
done
|
|
fi
|
|
if test "$_dxr2" = yes; then
|
|
_def_dxr2='#define HAVE_DXR2 1'
|
|
_vosrc="$_vosrc vo_dxr2.c"
|
|
_aosrc="$_aosrc ao_dxr2.c"
|
|
_aomodules="dxr2 $_aomodules"
|
|
_vomodules="dxr2 $_vomodules"
|
|
echores "yes (using $_inc_dxr2)"
|
|
else
|
|
_def_dxr2='#undef HAVE_DXR2'
|
|
_noaomodules="dxr2 $_noaomodules"
|
|
_novomodules="dxr2 $_novomodules"
|
|
_inc_dxr2=""
|
|
echores "no"
|
|
fi
|
|
|
|
echocheck "DXR3/H+"
|
|
if test "$_dxr3" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <linux/em8300.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_dxr3=no
|
|
cc_check && _dxr3=yes
|
|
fi
|
|
if test "$_dxr3" = yes ; then
|
|
_def_dxr3='#define HAVE_DXR3 1'
|
|
_vosrc="$_vosrc vo_dxr3.c"
|
|
_vomodules="dxr3 $_vomodules"
|
|
else
|
|
_def_dxr3='#undef HAVE_DXR3'
|
|
_novomodules="dxr3 $_novomodules"
|
|
if test "$_mp1e" = auto ; then
|
|
# we don't need mp1e
|
|
_mp1e=no
|
|
fi
|
|
fi
|
|
echores "$_dxr3"
|
|
|
|
echocheck "libmp1e"
|
|
if test "$_mmx" = no ; then
|
|
# mp1e REQUIRES mmx!
|
|
_mp1e=no
|
|
fi
|
|
if test "$_mp1e" != no ; then
|
|
_mp1e=yes
|
|
_def_mp1e='#define USE_MP1E'
|
|
_ld_mp1e='libmp1e/libmp1e.a'
|
|
_dep_mp1e='libmp1e/libmp1e.a'
|
|
else
|
|
_mp1e=no
|
|
_def_mp1e='#undef USE_MP1E'
|
|
_ld_mp1e=""
|
|
_dep_mp1e=''
|
|
fi
|
|
echores "$_mp1e"
|
|
|
|
|
|
echocheck "libfame"
|
|
if test "$_fame" = auto ; then
|
|
_fame=no
|
|
test "$_dxr2" = yes && _fame=auto
|
|
test "$_dxr3" = yes && _fame=auto
|
|
test "$_dvb" = yes && _fame=auto
|
|
fi
|
|
if test "$_fame" = auto ; then
|
|
_fame=no
|
|
if test -d libfame && test -f libfame/fame.h ; then
|
|
# disable fame on cygwin as no sense to port - atmos
|
|
cygwin || _fame=yes
|
|
echores $_fame
|
|
else
|
|
echores "no (no fame dir)"
|
|
fi
|
|
else
|
|
echores "$_fame"
|
|
fi
|
|
|
|
_def_fame='#undef USE_LIBFAME'
|
|
if test "$_fame" = yes ; then
|
|
_def_fame='#define USE_LIBFAME 1'
|
|
_ld_fame='libfame/libfame.a'
|
|
fi
|
|
|
|
|
|
#########
|
|
# AUDIO #
|
|
#########
|
|
|
|
|
|
echocheck "OSS Audio"
|
|
if test "$_ossaudio" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <sys/ioctl.h>
|
|
$_inc_soundcard
|
|
int main(void) { int arg = SNDCTL_DSP_SETFRAGMENT; return 0; }
|
|
EOF
|
|
_ossaudio=no
|
|
cc_check && _ossaudio=yes
|
|
fi
|
|
if test "$_ossaudio" = yes ; then
|
|
_def_ossaudio='#define USE_OSS_AUDIO 1'
|
|
_aosrc="$_aosrc ao_oss.c"
|
|
_aomodules="oss $_aomodules"
|
|
if test "$_linux_devfs" = yes; then
|
|
_def_ossaudio_devdsp='#define PATH_DEV_DSP "/dev/sound/dsp"'
|
|
_def_ossaudio_devmixer='#define PATH_DEV_MIXER "/dev/sound/mixer"'
|
|
else
|
|
cat > $TMPC << EOF
|
|
#include <sys/ioctl.h>
|
|
$_inc_soundcard
|
|
#ifdef OPEN_SOUND_SYSTEM
|
|
int main(void) { return 0; }
|
|
#else
|
|
#error Not the real thing
|
|
#endif
|
|
EOF
|
|
_real_ossaudio=no
|
|
cc_check && _real_ossaudio=yes
|
|
if test "$_real_ossaudio" = yes; then
|
|
_def_ossaudio_devdsp='#define PATH_DEV_DSP "/dev/dsp"'
|
|
elif netbsd || openbsd ; then
|
|
_def_ossaudio_devdsp='#define PATH_DEV_DSP "/dev/sound"'
|
|
_ld_arch="$_ld_arch -lossaudio"
|
|
else
|
|
_def_ossaudio_devdsp='#define PATH_DEV_DSP "/dev/dsp"'
|
|
fi
|
|
_def_ossaudio_devmixer='#define PATH_DEV_MIXER "/dev/mixer"'
|
|
fi
|
|
else
|
|
_def_ossaudio='#undef USE_OSS_AUDIO'
|
|
_def_ossaudio_devdsp='#define PATH_DEV_DSP ""'
|
|
_def_ossaudio_devmixer='#define PATH_DEV_MIXER ""'
|
|
_noaomodules="oss $_noaomodules"
|
|
fi
|
|
echores "$_ossaudio"
|
|
|
|
|
|
echocheck "aRts"
|
|
if test "$_arts" = auto ; then
|
|
_arts=no
|
|
if ( artsc-config --version ) >> "$TMPLOG" 2>&1 ; then
|
|
|
|
cat > $TMPC << EOF
|
|
#include <artsc.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check `artsc-config --libs` `artsc-config --cflags` && ( "$TMPO" >> "$TMPLOG" 2>&1 ) && _arts=yes
|
|
|
|
fi
|
|
fi
|
|
|
|
if test "$_arts" = yes ; then
|
|
_def_arts='#define USE_ARTS 1'
|
|
_aosrc="$_aosrc ao_arts.c"
|
|
_aomodules="arts $_aomodules"
|
|
_ld_arts=`artsc-config --libs`
|
|
_inc_arts=`artsc-config --cflags`
|
|
else
|
|
_noaomodules="arts $_noaomodules"
|
|
fi
|
|
echores "$_arts"
|
|
|
|
|
|
echocheck "EsounD"
|
|
if test "$_esd" = auto ; then
|
|
_esd=no
|
|
if ( esd-config --version ) >> "$TMPLOG" 2>&1 ; then
|
|
|
|
cat > $TMPC << EOF
|
|
#include <esd.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check `esd-config --libs` `esd-config --cflags` && ( "$TMPO" >> "$TMPLOG" 2>&1 ) && _esd=yes
|
|
|
|
fi
|
|
fi
|
|
echores "$_esd"
|
|
|
|
if test "$_esd" = yes ; then
|
|
_def_esd='#define USE_ESD 1'
|
|
_aosrc="$_aosrc ao_esd.c"
|
|
_aomodules="esd $_aomodules"
|
|
_ld_esd=`esd-config --libs`
|
|
_inc_esd=`esd-config --cflags`
|
|
|
|
echocheck "esd_get_latency()"
|
|
cat > $TMPC << EOF
|
|
#include <esd.h>
|
|
int main(void) { return esd_get_latency(0); }
|
|
EOF
|
|
cc_check `esd-config --libs` `esd-config --cflags` && _esd_latency=yes && _def_esd_latency='#define HAVE_ESD_LATENCY'
|
|
echores "$_esd_latency"
|
|
else
|
|
_def_esd='#undef USE_ESD'
|
|
_def_esd_latency='#undef HAVE_ESD_LATENCY'
|
|
_noaomodules="esd $_noaomodules"
|
|
fi
|
|
|
|
echocheck "Polyp"
|
|
if test "$_polyp" = auto ; then
|
|
_polyp=no
|
|
if ( pkg-config --exists 'polyplib >= 0.6 polyplib-error >= 0.6 polyplib-mainloop >= 0.6' ) >> "$TMPLOG" 2>&1 ; then
|
|
|
|
cat > $TMPC << EOF
|
|
#include <polyp/polyplib.h>
|
|
#include <polyp/mainloop.h>
|
|
#include <polyp/polyplib-error.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check `pkg-config --libs --cflags polyplib polyplib-error polyplib-mainloop` && ( "$TMPO" >> "$TMPLOG" 2>&1 ) && _polyp=yes
|
|
|
|
fi
|
|
fi
|
|
echores "$_polyp"
|
|
|
|
if test "$_polyp" = yes ; then
|
|
_def_polyp='#define USE_POLYP 1'
|
|
_aosrc="$_aosrc ao_polyp.c"
|
|
_aomodules="polyp $_aomodules"
|
|
_ld_polyp=`pkg-config --libs polyplib polyplib-error polyplib-mainloop`
|
|
_inc_polyp=`pkg-config --cflags polyplib polyplib-error polyplib-mainloop`
|
|
else
|
|
_def_polyp='#undef USE_POLYP'
|
|
_noaomodules="polyp $_noaomodules"
|
|
fi
|
|
|
|
|
|
echocheck "JACK"
|
|
if test "$_jack" = auto ; then
|
|
_jack=no
|
|
if ( ( pkg-config --modversion jack ) > /dev/null 2>&1 ) &&
|
|
( jackd --version | grep version | awk '{ print $3 }' ) >> "$TMPLOG" 2>&1 ; then
|
|
|
|
cat > $TMPC << EOF
|
|
#include <jack/jack.h>
|
|
int main(void) { jack_client_new("test"); return 0; }
|
|
EOF
|
|
cc_check `pkg-config --libs --cflags jack` && ( "$TMPO" >> "$TMPLOG" 2>&1 ) && _jack=yes
|
|
fi
|
|
fi
|
|
|
|
if test "$_jack" = yes ; then
|
|
_def_jack='#define USE_JACK 1'
|
|
_aosrc="$_aosrc ao_jack.c"
|
|
_aomodules="jack $_aomodules"
|
|
_ld_jack="`pkg-config --libs jack`"
|
|
_inc_jack=`pkg-config --cflags jack`
|
|
else
|
|
_noaomodules="jack $_noaomodules"
|
|
fi
|
|
echores "$_jack"
|
|
|
|
|
|
echocheck "ALSA audio"
|
|
if test "$_alsa" != no ; then
|
|
_alsa=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/asoundlib.h>
|
|
int main(void) { return (!(SND_LIB_MAJOR==0 && SND_LIB_MINOR==5)); }
|
|
EOF
|
|
cc_check -lasound $_ld_dl $_ld_pthread && $TMPO && _alsaver='0.5.x'
|
|
|
|
cat > $TMPC << EOF
|
|
#include <sys/asoundlib.h>
|
|
int main(void) { return (!(SND_LIB_MAJOR==0 && SND_LIB_MINOR==9)); }
|
|
EOF
|
|
cc_check -lasound $_ld_dl $_ld_pthread && $TMPO && _alsaver='0.9.x-sys'
|
|
cat > $TMPC << EOF
|
|
#include <alsa/asoundlib.h>
|
|
int main(void) { return (!(SND_LIB_MAJOR==0 && SND_LIB_MINOR==9)); }
|
|
EOF
|
|
cc_check -lasound $_ld_dl $_ld_pthread && $TMPO && _alsaver='0.9.x-alsa'
|
|
|
|
cat > $TMPC << EOF
|
|
#include <sys/asoundlib.h>
|
|
int main(void) { return (!(SND_LIB_MAJOR==1 && SND_LIB_MINOR==0)); }
|
|
EOF
|
|
cc_check -lasound $_ld_dl $_ld_pthread && $TMPO && _alsaver='1.0.x-sys'
|
|
cat > $TMPC << EOF
|
|
#include <alsa/asoundlib.h>
|
|
int main(void) { return (!(SND_LIB_MAJOR==1 && SND_LIB_MINOR==0)); }
|
|
EOF
|
|
cc_check -lasound $_ld_dl $_ld_pthread && $TMPO && _alsaver='1.0.x-alsa'
|
|
fi
|
|
_def_alsa5='#undef HAVE_ALSA5'
|
|
_def_alsa9='#undef HAVE_ALSA9'
|
|
_def_alsa1x='#undef HAVE_ALSA1X'
|
|
_def_sys_asoundlib_h='#undef HAVE_SYS_ASOUNDLIB_H'
|
|
_def_alsa_asoundlib_h='#undef HAVE_ALSA_ASOUNDLIB_H'
|
|
if test "$_alsaver" ; then
|
|
if test "$_alsaver" = '0.5.x' ; then
|
|
_aosrc="$_aosrc ao_alsa5.c"
|
|
_aomodules="alsa5 $_aomodules"
|
|
_def_alsa5='#define HAVE_ALSA5 1'
|
|
_def_sys_asoundlib_h='#define HAVE_SYS_ASOUNDLIB_H 1'
|
|
echores "yes (using alsa 0.5.x and sys/asoundlib.h)"
|
|
elif test "$_alsaver" = '0.9.x-sys' ; then
|
|
_aosrc="$_aosrc ao_alsa.c"
|
|
_aomodules="alsa $_aomodules"
|
|
_def_alsa9='#define HAVE_ALSA9 1'
|
|
_def_sys_asoundlib_h='#define HAVE_SYS_ASOUNDLIB_H 1'
|
|
echores "yes (using alsa 0.9.x and sys/asoundlib.h)"
|
|
elif test "$_alsaver" = '0.9.x-alsa' ; then
|
|
_aosrc="$_aosrc ao_alsa.c"
|
|
_aomodules="alsa $_aomodules"
|
|
_def_alsa9='#define HAVE_ALSA9 1'
|
|
_def_alsa_asoundlib_h='#define HAVE_ALSA_ASOUNDLIB_H 1'
|
|
echores "yes (using alsa 0.9.x and alsa/asoundlib.h)"
|
|
elif test "$_alsaver" = '1.0.x-sys' ; then
|
|
_aosrc="$_aosrc ao_alsa.c"
|
|
_aomodules="alsa $_aomodules"
|
|
_def_alsa1x="#define HAVE_ALSA1X 1"
|
|
_def_alsa_asoundlib_h='#define HAVE_SYS_ASOUNDLIB_H 1'
|
|
echores "yes (using alsa 1.0.x and sys/asoundlib.h)"
|
|
elif test "$_alsaver" = '1.0.x-alsa' ; then
|
|
_aosrc="$_aosrc ao_alsa.c"
|
|
_aomodules="alsa $_aomodules"
|
|
_def_alsa1x="#define HAVE_ALSA1X 1"
|
|
_def_alsa_asoundlib_h='#define HAVE_ALSA_ASOUNDLIB_H 1'
|
|
echores "yes (using alsa 1.0.x and alsa/asoundlib.h)"
|
|
fi
|
|
_ld_alsa="-lasound $_ld_dl $_ld_pthread"
|
|
else
|
|
_noaomodules="alsa $_noaomodules"
|
|
echores "no"
|
|
fi
|
|
|
|
|
|
echocheck "Sun audio"
|
|
if test "$_sunaudio" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/audioio.h>
|
|
int main(void) { audio_info_t info; AUDIO_INITINFO(&info); return 0; }
|
|
EOF
|
|
_sunaudio=no
|
|
cc_check && _sunaudio=yes
|
|
fi
|
|
if test "$_sunaudio" = yes ; then
|
|
_def_sunaudio='#define USE_SUN_AUDIO 1'
|
|
_aosrc="$_aosrc ao_sun.c"
|
|
_aomodules="sun $_aomodules"
|
|
else
|
|
_def_sunaudio='#undef USE_SUN_AUDIO'
|
|
_noaomodules="sun $_noaomodules"
|
|
fi
|
|
echores "$_sunaudio"
|
|
|
|
|
|
echocheck "Sun mediaLib"
|
|
if test "$_mlib" = auto ; then
|
|
_mlib=no
|
|
test -z "$_mlibdir" && _mlibdir=/opt/SUNWmlib
|
|
cat > $TMPC << EOF
|
|
#include <mlib.h>
|
|
int main(void) { mlib_VideoColorYUV2ABGR420(0,0,0,0,0,0,0,0,0); return 0; }
|
|
EOF
|
|
cc_check -I${_mlibdir}/include -L${_mlibdir}/lib -lmlib && _mlib=yes
|
|
fi
|
|
if test "$_mlib" = yes ; then
|
|
_def_mlib='#define HAVE_MLIB 1'
|
|
_inc_mlib=" -I${_mlibdir}/include "
|
|
_ld_mlib=" -L${_mlibdir}/lib -R${_mlibdir}/lib -lmlib "
|
|
else
|
|
_def_mlib='#undef HAVE_MLIB'
|
|
fi
|
|
echores "$_mlib"
|
|
|
|
|
|
echocheck "SGI audio"
|
|
if test "$_sgiaudio" = auto ; then
|
|
# check for SGI audio
|
|
cat > $TMPC << EOF
|
|
#include <dmedia/audio.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_sgiaudio=no
|
|
cc_check && _sgiaudio=yes
|
|
fi
|
|
if test "$_sgiaudio" = "yes" ; then
|
|
_def_sgiaudio='#define USE_SGI_AUDIO 1'
|
|
_ld_sgiaudio='-laudio'
|
|
_aosrc="$_aosrc ao_sgi.c"
|
|
_aomodules="sgi $_aomodules"
|
|
else
|
|
_def_sgiaudio='#undef USE_SGI_AUDIO'
|
|
_noaomodules="sgi $_noaomodules"
|
|
fi
|
|
echores "$_sgiaudio"
|
|
|
|
|
|
echocheck "VCD support"
|
|
if linux || bsdos || freebsd || netbsd || sunos || darwin ; then
|
|
_inputmodules="vcd $_inputmodules"
|
|
_def_vcd='#define HAVE_VCD 1'
|
|
echores "ok"
|
|
else
|
|
_def_vcd='#undef HAVE_VCD'
|
|
_noinputmodules="vcd $_noinputmodules"
|
|
echores "not supported on this OS"
|
|
fi
|
|
|
|
echocheck "DVD support (libmpdvdkit)"
|
|
if test "$_dvdkit" = auto ; then
|
|
_dvdkit=no
|
|
if linux || freebsd || netbsd || darwin || openbsd || win32 || sunos || hpux; then
|
|
test -f "./libmpdvdkit2/Makefile" && _dvdkit=yes
|
|
test -f "./libmpdvdkit/Makefile" && _dvdkit=yes
|
|
fi
|
|
fi
|
|
if test "$_dvdkit" = yes ; then
|
|
if test "$_dvd" = yes || test "$_cdrom" = yes || test "$_cdio" = yes || test "$_dvdio" = yes || test "$_bsdi_dvd" = yes || test "$_hpux_scsi_h" = yes || darwin || win32 ; then
|
|
if test -f "./libmpdvdkit2/Makefile" ; then
|
|
_inputmodules="mpdvdkit2 $_inputmodules"
|
|
_dvdread=libmpdvdkit2
|
|
_dvdkit2=yes
|
|
_dvdkit=no
|
|
else
|
|
_inputmodules="mpdvdkit $_inputmodules"
|
|
_dvdread=libmpdvdkit
|
|
fi
|
|
else
|
|
_noinputmodules="mpdvdkit $_noinputmodules"
|
|
fi
|
|
_def_dvd_linux='#undef HAVE_LINUX_DVD_STRUCT'
|
|
_def_dvd_bsd='#undef HAVE_BSD_DVD_STRUCT'
|
|
_dev_dvd_openbsd='#undef HAVE_OPENBSD_DVD_STRUCT'
|
|
_def_dvd_darwin='#undef DARWIN_DVD_IOCTL'
|
|
if linux || netbsd || openbsd || bsdos ; then
|
|
_def_dvd_linux='#define HAVE_LINUX_DVD_STRUCT 1'
|
|
if openbsd ; then
|
|
_dev_dvd_openbsd='#define HAVE_OPENBSD_DVD_STRUCT 1'
|
|
fi
|
|
else
|
|
if freebsd ; then
|
|
_def_dvd_bsd='#define HAVE_BSD_DVD_STRUCT 1'
|
|
else
|
|
if darwin ; then
|
|
_def_dvd_darwin='#define DARWIN_DVD_IOCTL'
|
|
fi
|
|
fi
|
|
fi
|
|
else
|
|
_noinputmodules="mpdvdkit $_noinputmodules"
|
|
fi
|
|
if test "$_dvdkit" = yes || test "$_dvdkit2" = yes; then
|
|
echores "yes"
|
|
else
|
|
echores "no"
|
|
fi
|
|
|
|
echocheck "DVD support (libdvdread)"
|
|
if test "$_dvdread" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <inttypes.h>
|
|
#include <dvdread/dvd_reader.h>
|
|
#include <dvdread/ifo_types.h>
|
|
#include <dvdread/ifo_read.h>
|
|
#include <dvdread/nav_read.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_dvdread=no
|
|
if test "$_dl" = yes; then
|
|
cc_check \
|
|
-D_LARGEFILE_SOURCE -D_FILE_OFFSET_BITS=64 -D_LARGEFILE64_SOURCE -ldvdread $_ld_dl && \
|
|
_dvdread=yes
|
|
fi
|
|
fi
|
|
_def_mpdvdkit="#undef USE_MPDVDKIT"
|
|
case "$_dvdread" in
|
|
yes)
|
|
_largefiles=yes
|
|
_def_dvdread='#define USE_DVDREAD 1'
|
|
_ld_dvdread='-ldvdread'
|
|
_inputmodules="dvdread $_inputmodules"
|
|
echores "yes"
|
|
;;
|
|
no)
|
|
_def_dvdread='#undef USE_DVDREAD'
|
|
_noinputmodules="dvdread $_noinputmodules"
|
|
echores "no"
|
|
;;
|
|
libmpdvdkit)
|
|
_largefiles=yes
|
|
_def_dvdread='#define USE_DVDREAD 1'
|
|
_ld_dvdread='-Llibmpdvdkit -lmpdvdkit'
|
|
_noinputmodules="dvdread $_noinputmodules"
|
|
_def_mpdvdkit="#define USE_MPDVDKIT 1"
|
|
echores "disabled by libmpdvdkit"
|
|
;;
|
|
libmpdvdkit2)
|
|
_largefiles=yes
|
|
_def_dvdread='#define USE_DVDREAD 1'
|
|
_ld_dvdread='-Llibmpdvdkit2 -lmpdvdkit'
|
|
_noinputmodules="dvdread $_noinputmodules"
|
|
_def_mpdvdkit="#define USE_MPDVDKIT 2"
|
|
echores "disabled by libmpdvdkit2"
|
|
;;
|
|
esac
|
|
|
|
# dvdnav disabled, it does not work
|
|
# echocheck "DVD support (libdvdnav)"
|
|
# if test "$_dvdnav" = yes ; then
|
|
# cat > $TMPC <<EOF
|
|
# #include <dvdnav.h>
|
|
# int main(void) { dvdnav_t *dvd=0; return 0; }
|
|
# EOF
|
|
# _dvdnav=no
|
|
# test -n "$_dvdnavdir" && _legal_dvdnavdir=-L$_dvdnavdir/.libs
|
|
# if test -z "$_dvdnavconfig" ; then
|
|
# if ( dvdnav-config --version ) >/dev/null 2>&1 ; then
|
|
# _dvdnavconfig="dvdnav-config"
|
|
# fi
|
|
# fi
|
|
# test -z "$_dvdnavdir" && test -n "$_dvdnavconfig" && _dvdnavdir=`$_dvdnavconfig --cflags`
|
|
# _used_css=
|
|
# test "$_dvdkit" = no && test "$_dvdkit2" = no && _used_css=$_ld_css
|
|
# cc_check $_inc_extra -I$_dvdnavdir $_legal_dvdnavdir -ldvdnav $_used_css $_ld_dl $_ld_pthread && _dvdnav=yes
|
|
# fi
|
|
# if test "$_dvdnav" = yes ; then
|
|
# _largefiles=yes
|
|
# _def_dvdnav='#define USE_DVDNAV 1'
|
|
# if test -n "$_legal_dvdnavdir" ; then
|
|
# _ld_css="$_ld_css $_legal_dvdnavdir -ldvdnav"
|
|
# elif test -n "$_dvdnavconfig" ; then
|
|
# _ld_css="$_ld_css `$_dvdnavconfig --libs`"
|
|
# else
|
|
# _ld_css="$_ld_css -ldvdnav"
|
|
# fi
|
|
# if test -n "$_dvdnavconfig" ; then
|
|
# _dvdnav_version=`$_dvdnavconfig --version | sed "s/\.//g"`
|
|
# _def_dvdnav_version="#define DVDNAVVERSION $_dvdnav_version"
|
|
# fi
|
|
# if test -n "$_dvdnavdir" ; then
|
|
# _inc_extra="$_inc_extra -I$_dvdnavdir"
|
|
# fi
|
|
# _inputmodules="dvdnav $_inputmodules"
|
|
# echores "yes"
|
|
# else
|
|
# _def_dvdnav='#undef USE_DVDNAV'
|
|
# _noinputmodules="dvdnav $_noinputmodules"
|
|
# echores "no"
|
|
# fi
|
|
|
|
echocheck "cdparanoia"
|
|
if test "$_cdparanoia" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <cdda_interface.h>
|
|
#include <cdda_paranoia.h>
|
|
// This need a better test. How ?
|
|
int main(void) { return 1; }
|
|
EOF
|
|
_cdparanoia=no
|
|
if cc_check $_inc_cdparanoia $_ld_cdparanoia -lcdda_interface -lcdda_paranoia $_ld_lm ; then
|
|
_cdparanoia=yes
|
|
else
|
|
for I in /usr/include/cdda /usr/local/include/cdda ; do
|
|
if cc_check -I$I $_ld_cdparanoia -lcdda_interface -lcdda_paranoia $_ld_lm ; then
|
|
_cdparanoia=yes; _inc_cdparanoia="-I$I"; break
|
|
fi
|
|
done
|
|
fi
|
|
fi
|
|
if test "$_cdparanoia" = yes ; then
|
|
_def_cdparanoia='#define HAVE_CDDA'
|
|
_inputmodules="cdda $_inputmodules"
|
|
_ld_cdparanoia="$_ld_cdparanoia -lcdda_interface -lcdda_paranoia"
|
|
openbsd && _ld_cdparanoia="$_ld_cdparanoia -lutil"
|
|
else
|
|
_def_cdparanoia='#undef HAVE_CDDA'
|
|
_noinputmodules="cdda $_noinputmodules"
|
|
fi
|
|
echores "$_cdparanoia"
|
|
|
|
|
|
echocheck "freetype >= 2.0.9"
|
|
|
|
# freetype depends on iconv
|
|
if test "$_iconv" = no ; then
|
|
_freetype="no (iconv support needed)"
|
|
fi
|
|
|
|
if test "$_freetype" = auto ; then
|
|
if ( $_freetypeconfig --version ) >/dev/null 2>&1 ; then
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <ft2build.h>
|
|
#include FT_FREETYPE_H
|
|
#if ((FREETYPE_MAJOR < 2) || ((FREETYPE_MINOR == 0) && (FREETYPE_PATCH < 9)))
|
|
#error "Need FreeType 2.0.9 or newer"
|
|
#endif
|
|
int main()
|
|
{
|
|
FT_Library library;
|
|
FT_Int major=-1,minor=-1,patch=-1;
|
|
int err=FT_Init_FreeType(&library);
|
|
if(err){
|
|
printf("Couldn't initialize freetype2 lib, err code: %d\n",err);
|
|
exit(err);
|
|
}
|
|
FT_Library_Version(library,&major,&minor,&patch); // in v2.1.0+ only :(((
|
|
printf("freetype2 header version: %d.%d.%d library version: %d.%d.%d\n",
|
|
FREETYPE_MAJOR,FREETYPE_MINOR,FREETYPE_PATCH,
|
|
(int)major,(int)minor,(int)patch );
|
|
if(major!=FREETYPE_MAJOR || minor!=FREETYPE_MINOR){
|
|
printf("Library and header version mismatch! Fix it in your distribution!\n");
|
|
exit(1);
|
|
}
|
|
return 0;
|
|
}
|
|
EOF
|
|
_freetype=no
|
|
cc_check `$_freetypeconfig --cflags` `$_freetypeconfig --libs` && ( $TMPO >> "$TMPLOG" ) && _freetype=yes
|
|
else
|
|
_freetype=no
|
|
fi
|
|
fi
|
|
if test "$_freetype" = yes ; then
|
|
_def_freetype='#define HAVE_FREETYPE'
|
|
_inc_freetype=`$_freetypeconfig --cflags`
|
|
_ld_freetype=`$_freetypeconfig --libs`
|
|
else
|
|
_def_freetype='#undef HAVE_FREETYPE'
|
|
fi
|
|
echores "$_freetype"
|
|
|
|
if test "$_freetype" = no ; then
|
|
_fontconfig=no
|
|
fi
|
|
echocheck "fontconfig"
|
|
if test "$_fontconfig" = auto ; then
|
|
if ( pkg-config --modversion fontconfig) > /dev/null 2>&1 ; then
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <fontconfig/fontconfig.h>
|
|
int main()
|
|
{
|
|
int err = FcInit();
|
|
if(err == FcFalse){
|
|
printf("Couldn't initialize fontconfig lib\n");
|
|
exit(err);
|
|
}
|
|
return 0;
|
|
|
|
}
|
|
EOF
|
|
_fontconfig=no
|
|
cc_check `pkg-config --cflags --libs fontconfig` && ( $TMPO >> "$TMPLOG" ) && _fontconfig=yes
|
|
else
|
|
_fontconfig=no
|
|
fi
|
|
fi
|
|
if test "$_fontconfig" = yes ; then
|
|
_def_fontconfig='#define HAVE_FONTCONFIG'
|
|
_inc_fontconfig=`pkg-config --cflags fontconfig`
|
|
_ld_fontconfig=`pkg-config --libs fontconfig`
|
|
else
|
|
_def_fontconfig='#undef HAVE_FONTCONFIG'
|
|
fi
|
|
echores "$_fontconfig"
|
|
|
|
echocheck "fribidi with charsets"
|
|
if test "$_fribidi" = yes ; then
|
|
if ( $_fribidiconfig --version ) >/dev/null 2>&1 ; then
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
/* workaround for fribidi 0.10.4 and below */
|
|
#define FRIBIDI_CHARSET_UTF8 FRIBIDI_CHAR_SET_UTF8
|
|
#include <fribidi/fribidi.h>
|
|
int main()
|
|
{
|
|
if(fribidi_parse_charset("UTF-8") != FRIBIDI_CHAR_SET_UTF8) {
|
|
printf("Fribidi headers are not consistents with the library!\n");
|
|
exit(1);
|
|
}
|
|
return 0;
|
|
}
|
|
EOF
|
|
_fribidi=no
|
|
cc_check `$_fribidiconfig --cflags` `$_fribidiconfig --libs` && ( $TMPO >> "$TMPLOG" ) && _fribidi=yes
|
|
else
|
|
_fribidi=no
|
|
fi
|
|
fi
|
|
if test "$_fribidi" = yes ; then
|
|
_def_fribidi='#define USE_FRIBIDI'
|
|
_inc_fribidi=`$_fribidiconfig --cflags`
|
|
_ld_fribidi=`$_fribidiconfig --libs`
|
|
else
|
|
_def_fribidi='#undef USE_FRIBIDI'
|
|
fi
|
|
echores "$_fribidi"
|
|
|
|
|
|
echocheck "ENCA"
|
|
if test "$_enca" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <enca.h>
|
|
int main()
|
|
{
|
|
const char **langs;
|
|
size_t langcnt;
|
|
langs = enca_get_languages(&langcnt);
|
|
return 0;
|
|
}
|
|
EOF
|
|
_enca=no
|
|
cc_check -lenca && _enca=yes
|
|
fi
|
|
if test "$_enca" = yes ; then
|
|
_def_enca='#define HAVE_ENCA 1'
|
|
_ld_enca='-lenca'
|
|
else
|
|
_def_enca='#undef HAVE_ENCA'
|
|
fi
|
|
echores "$_enca"
|
|
|
|
|
|
echocheck "zlib"
|
|
cat > $TMPC << EOF
|
|
#include <zlib.h>
|
|
int main(void) { (void) inflate(0, Z_NO_FLUSH); return 0; }
|
|
EOF
|
|
_zlib=no
|
|
cc_check -lz && _zlib=yes
|
|
if test "$_zlib" = yes ; then
|
|
_def_zlib='#define HAVE_ZLIB 1'
|
|
_ld_zlib='-lz'
|
|
else
|
|
_def_zlib='#undef HAVE_ZLIB'
|
|
fi
|
|
echores "$_zlib"
|
|
|
|
|
|
echocheck "RTC"
|
|
if test "$_rtc" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <sys/ioctl.h>
|
|
#ifdef __linux__
|
|
#include <linux/rtc.h>
|
|
#else
|
|
#include <rtc.h>
|
|
#define RTC_PIE_ON RTCIO_PIE_ON
|
|
#endif
|
|
int main(void) { return RTC_PIE_ON; }
|
|
EOF
|
|
_rtc=no
|
|
cc_check && _rtc=yes
|
|
fi
|
|
if test "$_rtc" = yes ; then
|
|
_def_rtc='#define HAVE_RTC 1'
|
|
else
|
|
_def_rtc='#undef HAVE_RTC'
|
|
fi
|
|
echores "$_rtc"
|
|
|
|
|
|
echocheck "external liblzo support"
|
|
if test "$_liblzo" = auto ; then
|
|
_liblzo=no
|
|
cat > $TMPC << EOF
|
|
#include <lzo1x.h>
|
|
int main(void) { lzo_init();return 0; }
|
|
EOF
|
|
cc_check -llzo && _liblzo=yes
|
|
fi
|
|
if test "$_liblzo" = yes ; then
|
|
_def_liblzo='#define USE_LIBLZO 1'
|
|
_ld_liblzo='-llzo'
|
|
_codecmodules="liblzo $_codecmodules"
|
|
else
|
|
_def_liblzo='#undef USE_LIBLZO'
|
|
_nocodecmodules="liblzo $_nocodecmodules"
|
|
fi
|
|
echores "$_liblzo"
|
|
|
|
|
|
echocheck "mad support"
|
|
if test "$_mad" = auto ; then
|
|
_mad=no
|
|
cat > $TMPC << EOF
|
|
#include <mad.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check $_madlibdir -lmad && _mad=yes
|
|
fi
|
|
if test "$_mad" = yes ; then
|
|
_def_mad='#define USE_LIBMAD 1'
|
|
_ld_mad='-lmad'
|
|
_codecmodules="libmad $_codecmodules"
|
|
else
|
|
_def_mad='#undef USE_LIBMAD'
|
|
_nocodecmodules="libmad $_nocodecmodules"
|
|
fi
|
|
echores "$_mad"
|
|
|
|
echocheck "Toolame"
|
|
if test "$_toolame" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <toolame.h>
|
|
int main(void) { toolame_init(); return 0; }
|
|
EOF
|
|
_toolame=no
|
|
_toolame_extraflags=""
|
|
_toolame_lib="-ltoolame"
|
|
if test -n "$_toolamedir"; then
|
|
_toolame_extraflags="-I$_toolamedir -L$_toolamedir"
|
|
fi
|
|
cc_check $_toolame_extraflags $_toolame_lib $_ld_lm && _toolame=yes
|
|
fi
|
|
if test "$_toolame" = yes ; then
|
|
_def_toolame='#define HAVE_TOOLAME 1'
|
|
_codecmodules="$_codecmodules toolame"
|
|
else
|
|
_def_toolame='#undef HAVE_TOOLAME'
|
|
_toolame_lib=""
|
|
_nocodecmodules="toolame $_nocodecmodules"
|
|
fi
|
|
if test -z "$_toolamedir" ; then
|
|
echores "$_toolame"
|
|
else
|
|
echores "$_toolame (using $_toolamedir)"
|
|
fi
|
|
|
|
echocheck "Twolame"
|
|
if test "$_twolame" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <twolame.h>
|
|
int main(void) { twolame_init(); return 0; }
|
|
EOF
|
|
_twolame=no
|
|
_twolame_lib="-ltwolame"
|
|
cc_check $_twolame_lib $_ld_lm && _twolame=yes
|
|
fi
|
|
if test "$_twolame" = yes ; then
|
|
_def_twolame='#define HAVE_TWOLAME 1'
|
|
_codecmodules="$_codecmodules twolame"
|
|
else
|
|
_def_twolame='#undef HAVE_TWOLAME'
|
|
_twolame_lib=""
|
|
_nocodecmodules="twolame $_nocodecmodules"
|
|
fi
|
|
echores "$_twolame"
|
|
|
|
echocheck "OggVorbis support"
|
|
if test "$_tremor_internal" = yes; then
|
|
_vorbis=yes
|
|
elif test "$_vorbis" = auto; then
|
|
_vorbis=no
|
|
cat > $TMPC << EOF
|
|
#include <vorbis/codec.h>
|
|
int main(void) { vorbis_packet_blocksize(0,0); return 0; }
|
|
EOF
|
|
cc_check -lvorbis -logg $_ld_lm && _vorbis=yes
|
|
fi
|
|
if test "$_vorbis" = yes ; then
|
|
_def_vorbis='#define HAVE_OGGVORBIS 1'
|
|
if test "$_tremor_internal" = yes ; then
|
|
# do not set _ld_vorbis as it is resolved separately
|
|
# mp3lame support for vorbis is deprecated so don't care
|
|
_def_tremor='#define TREMOR 1'
|
|
if test "$_tremor_low" = yes ; then
|
|
_tremor_flags='-D_LOW_ACCURACY_'
|
|
else
|
|
_tremor_flags=''
|
|
fi
|
|
_codecmodules="tremor(internal) $_codecmodules"
|
|
elif test "$_tremor" = yes ; then
|
|
_def_tremor='#define TREMOR 1'
|
|
_ld_vorbis='-lvorbisidec'
|
|
_codecmodules="tremor $_codecmodules"
|
|
else
|
|
_def_tremor='#undef TREMOR'
|
|
_ld_vorbis='-lvorbis -logg'
|
|
_codecmodules="libvorbis $_codecmodules"
|
|
fi
|
|
else
|
|
_def_vorbis='#undef HAVE_OGGVORBIS'
|
|
_def_tremor='#undef TREMOR'
|
|
_nocodecmodules="libvorbis $_nocodecmodules"
|
|
fi
|
|
if test "$_vorbis" = yes -a "$_tremor_internal" = yes -a "$_tremor_low" = yes ; then
|
|
echores "$_vorbis (internal low accuracy Tremor)"
|
|
elif test "$_vorbis" = yes -a "$_tremor_internal" = yes ; then
|
|
echores "$_vorbis (internal Tremor)"
|
|
elif test "$_vorbis" = yes -a "$_tremor" = yes ; then
|
|
echores "$_vorbis (Tremor)"
|
|
else
|
|
echores "$_vorbis"
|
|
fi
|
|
|
|
echocheck "OggTheora support (only the CVS version!)"
|
|
if test "$_theora" = auto ; then
|
|
_theora=no
|
|
cat > $TMPC << EOF
|
|
#include <theora/theora.h>
|
|
#include <string.h>
|
|
int main(void)
|
|
{
|
|
/* theora is in flux, make sure that all interface routines and
|
|
* datatypes exist and work the way we expect it, so we don't break
|
|
* mplayer */
|
|
ogg_packet op;
|
|
theora_comment tc;
|
|
theora_info inf;
|
|
theora_state st;
|
|
yuv_buffer yuv;
|
|
int r;
|
|
double t;
|
|
|
|
theora_info_init (&inf);
|
|
theora_comment_init (&tc);
|
|
|
|
return 0;
|
|
|
|
/* we don't want to execute this kind of nonsense; just for making sure
|
|
* that compilation works... */
|
|
memset(&op, 0, sizeof(op));
|
|
r = theora_decode_header (&inf, &tc, &op);
|
|
r = theora_decode_init (&st, &inf);
|
|
t = theora_granule_time (&st, op.granulepos);
|
|
r = theora_decode_packetin (&st, &op);
|
|
r = theora_decode_YUVout (&st, &yuv);
|
|
theora_clear (&st);
|
|
|
|
return 0;
|
|
}
|
|
EOF
|
|
cc_check -ltheora -logg $_ld_lm && _theora=yes
|
|
fi
|
|
if test "$_theora" = yes ; then
|
|
_def_theora='#define HAVE_OGGTHEORA 1'
|
|
_codecmodules="libtheora $_codecmodules"
|
|
_ld_theora="-ltheora -logg"
|
|
else
|
|
_def_theora='#undef HAVE_OGGTHEORA'
|
|
_nocodecmodules="libtheora $_nocodecmodules"
|
|
fi
|
|
echores "$_theora"
|
|
|
|
echocheck "mp3lib support"
|
|
if test "$_mp3lib" = yes ; then
|
|
_def_mp3lib='#define USE_MP3LIB 1'
|
|
_codecmodules="mp3lib $_codecmodules"
|
|
else
|
|
_def_mp3lib='#undef USE_MP3LIB'
|
|
_nocodecmodules="mp3lib $_nocodecmodules"
|
|
fi
|
|
echores "$_mp3lib"
|
|
|
|
echocheck "liba52 support"
|
|
if test "$_liba52" = yes ; then
|
|
_def_liba52='#define USE_LIBA52 1'
|
|
_codecmodules="liba52 $_codecmodules"
|
|
else
|
|
_def_liba52='#undef USE_LIBA52'
|
|
_nocodecmodules="liba52 $_nocodecmodules"
|
|
fi
|
|
echores "$_liba52"
|
|
|
|
echocheck "libdts support"
|
|
if test "$_libdts" = auto ; then
|
|
_libdts=no
|
|
cat > $TMPC << EOF
|
|
#include <inttypes.h>
|
|
#include <dts.h>
|
|
int main(void) { dts_init (0); return 0; }
|
|
EOF
|
|
cc_check $_inc_libdts $_ld_libdts -ldts $_ld_lm && _libdts=yes
|
|
fi
|
|
if test "$_libdts" = yes ; then
|
|
_def_libdts='#define CONFIG_DTS 1'
|
|
_ld_libdts="$_ld_libdts -ldts $_ld_lm"
|
|
_codecmodules="libdts $_codecmodules"
|
|
else
|
|
_def_libdts='#undef CONFIG_DTS'
|
|
_nocodecmodules="libdts $_nocodecmodules"
|
|
fi
|
|
echores "$_libdts"
|
|
|
|
echocheck "libmpeg2 support"
|
|
if test "$_libmpeg2" = yes ; then
|
|
_def_libmpeg2='#define USE_LIBMPEG2 1'
|
|
_codecmodules="libmpeg2 $_codecmodules"
|
|
else
|
|
_def_libmpeg2='#undef USE_LIBMPEG2'
|
|
_nocodecmodules="libmpeg2 $_nocodecmodules"
|
|
fi
|
|
echores "$_libmpeg2"
|
|
|
|
|
|
echocheck "Matroska support"
|
|
if test "$_matroska_internal" = yes ; then
|
|
_inputmodules="matroska $_inputmodules"
|
|
_def_matroska='#define HAVE_MATROSKA 1'
|
|
else
|
|
_noinputmodules="matroska $_noinputmodules"
|
|
_def_matroska='#undef HAVE_MATROSKA'
|
|
fi
|
|
echores "$_matroska_internal"
|
|
|
|
echocheck "FAAC (AAC encoder) support"
|
|
if test "$_faac" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <inttypes.h>
|
|
#include <faac.h>
|
|
int main(void) { unsigned long x, y; faacEncOpen(48000, 2, &x, &y); return 0; }
|
|
EOF
|
|
if ( cc_check -c -O4 -lfaac $_ld_lm ); then
|
|
_faac=yes
|
|
elif ( cc_check -c -O4 -lfaac -lmp4v2 -lstdc++ $_ld_lm ); then
|
|
_faac=yes
|
|
_ld_faac="-lmp4v2 -lstdc++"
|
|
else
|
|
_faac=no
|
|
fi
|
|
fi
|
|
if test "$_faac" = yes ; then
|
|
_def_faac="#define HAVE_FAAC 1"
|
|
_codecmodules="$_codecmodules faac"
|
|
_ld_faac="-lfaac $_ld_faac"
|
|
else
|
|
_def_faac="#undef HAVE_FAAC"
|
|
_nocodecmodules="$_nocodecmodules faac"
|
|
fi
|
|
echores "$_faac"
|
|
|
|
echocheck "internal FAAD2 (AAC) support"
|
|
_inc_faad="-I`pwd`/libfaad2"
|
|
if test "$_faad_internal" = auto ; then
|
|
# the faad check needs a config.h file
|
|
if not test -f "config.h" ; then
|
|
cat > config.h << EOF
|
|
/* C99 lrintf function available */
|
|
$_def_lrintf
|
|
EOF
|
|
fi
|
|
# internal faad: check if our dear gcc is able to compile it...
|
|
cp "`pwd`/libfaad2/cfft.c" $TMPC
|
|
if ( cc_check -c -O4 $_march $_mcpu $_pipe -ffast-math -fomit-frame-pointer $_opt_gnu99 -D_GNU_SOURCE $_inc_faad ); then
|
|
_faad_internal=yes
|
|
else
|
|
_faad_internal="no (broken gcc)"
|
|
fi
|
|
fi
|
|
if test "$_faad_internal" = yes ; then
|
|
_def_faad_internal="#define USE_INTERNAL_FAAD 1"
|
|
_faad_external=no
|
|
else
|
|
_def_faad_internal="#undef USE_INTERNAL_FAAD"
|
|
_inc_faad=
|
|
fi
|
|
echores "$_faad_internal"
|
|
|
|
|
|
echocheck "external FAAD2 (AAC) support"
|
|
if test "$_faad_external" != no ; then
|
|
_ld_faad='-lfaad'
|
|
_inc_faad="$_inc_extra"
|
|
# external faad: check if it's really faad2 :)
|
|
if test "$_faad_external" = auto ; then
|
|
_faad_external=no
|
|
cat > $TMPC << EOF
|
|
#include <faad.h>
|
|
#ifndef FAAD_MIN_STREAMSIZE
|
|
#error Too old version
|
|
#endif
|
|
int main(void) { faacDecHandle testhand; faacDecFrameInfo testinfo; testhand = faacDecOpen(); faacDecInit(0, 0, 0, 0, 0); return 0; }
|
|
EOF
|
|
cc_check $_inc_faad $_ld_faad $_ld_lm && _faad_external=yes
|
|
fi
|
|
echores "$_faad_external"
|
|
else
|
|
echores "no"
|
|
fi
|
|
|
|
if test "$_faad_external" = yes; then
|
|
_def_faad='#define HAVE_FAAD 1'
|
|
_codecmodules="faad2(external) $_codecmodules"
|
|
elif test "$_faad_internal" = yes; then
|
|
_def_faad='#define HAVE_FAAD 1'
|
|
_codecmodules="faad2(internal) $_codecmodules"
|
|
else
|
|
_def_faad='#undef HAVE_FAAD'
|
|
_nocodecmodules="faad2 $_nocodecmodules"
|
|
_ld_faad=
|
|
fi
|
|
|
|
|
|
echocheck "LADSPA plugin support"
|
|
if test "$_ladspa" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdio.h>
|
|
#include <ladspa.h>
|
|
int main(void) {
|
|
const LADSPA_Descriptor *ld = NULL;
|
|
return 0;
|
|
}
|
|
EOF
|
|
_ladspa=no
|
|
cc_check && _ladspa=yes
|
|
fi
|
|
if test "$_ladspa" = yes; then
|
|
_def_ladspa="#define HAVE_LADSPA"
|
|
_afsrc="$_afsrc af_ladspa.c"
|
|
_afmodules="ladspa $_afmodules"
|
|
else
|
|
_def_ladspa="#undef HAVE_LADSPA"
|
|
_noafmodules="ladspa $_noafmodules"
|
|
fi
|
|
echores "$_ladspa"
|
|
|
|
|
|
if test "$_win32" = auto ; then
|
|
if x86 ; then
|
|
qnx && _win32=no
|
|
else
|
|
_win32=no # x86 arch only
|
|
fi
|
|
fi
|
|
|
|
if test "$_win32" != no ; then
|
|
if test -z "$_win32libdir" ; then
|
|
for I in "$_libdir/codecs" "$_libdir/win32" /usr/local/lib/codecs /usr/lib/codecs /usr/local/lib/win32 /usr/lib/win32 ; do
|
|
if test -d "$I" ; then
|
|
_win32libdir="$I"
|
|
break;
|
|
fi;
|
|
done
|
|
fi
|
|
fi
|
|
|
|
echocheck "Win32 codec DLL support"
|
|
if test "$_win32" = auto ; then
|
|
_win32=no
|
|
test -n "$_win32libdir" && _win32=yes
|
|
fi
|
|
if test "$_win32" = yes ; then
|
|
_def_win32='#define USE_WIN32DLL 1'
|
|
echores "yes (using $_win32libdir)"
|
|
else
|
|
_def_win32='#undef USE_WIN32DLL'
|
|
_nocodecmodules="win32 $_nocodecmodules"
|
|
_dshow=no
|
|
echores "no"
|
|
fi
|
|
|
|
if test "$_win32" != no ; then
|
|
_def_win32_loader='#undef WIN32_LOADER'
|
|
echocheck "Win32 loader support"
|
|
_ld_win32='loader/libloader.a'
|
|
_dep_win32='loader/libloader.a'
|
|
_codecmodules="win32 $_codecmodules"
|
|
if openbsd ; then
|
|
x86 && _ld_win32="$_ld_win32 -li386"
|
|
fi
|
|
if not win32 ; then
|
|
_def_win32_loader='#define WIN32_LOADER 1'
|
|
echores "yes"
|
|
else
|
|
_ld_win32libs="$_ld_win32libs -ladvapi32 -lole32"
|
|
echores "no (using native windows)"
|
|
fi
|
|
fi
|
|
|
|
echocheck "DirectShow"
|
|
if false ; then
|
|
|
|
if test "$_dshow" != no ; then
|
|
_dshow=no
|
|
# check if compiler supports C++ and C++-libs are installed correctly
|
|
cat > "$TMPCPP" << EOF
|
|
#include <string>
|
|
class myclass {
|
|
private: int ret;
|
|
public: int myreturn(void);
|
|
};
|
|
int myclass::myreturn(void) { ret = 0; return ret ; }
|
|
int main(void) { myclass myobject; return myobject.myreturn(); }
|
|
EOF
|
|
echo "------------------------------------------------" >> "$TMPLOG"
|
|
cat "$TMPCPP" >> "$TMPLOG"
|
|
if ( $_cc "$TMPCPP" -o "$TMPO" && "$TMPO" ) >> "$TMPLOG" 2>&1 ; then
|
|
_dshow=yes
|
|
echores "yes (C++ is ok)"
|
|
else
|
|
echores "no"
|
|
cat << EOF
|
|
|
|
Your C++ runtime environment is broken.
|
|
|
|
Hints: Does $_cc support C++? Do you have you a C++ compiler installed?
|
|
Are the C++ libraries correctly installed?
|
|
Check for libstdc++ and in (/etc/)ld.so.conf.
|
|
|
|
If you do not need DirectShow support, you can also use:
|
|
./configure --disable-dshow <your-normal-configure-options>
|
|
to disable building the C++ based DirectShow code.
|
|
|
|
EOF
|
|
die "$_cc's C++ is broken"
|
|
fi
|
|
fi
|
|
|
|
fi
|
|
|
|
echores "$_dshow"
|
|
|
|
if test "$_dshow" = yes ; then
|
|
_def_dshow='#define USE_DIRECTSHOW 1'
|
|
_ld_dshow='loader/dshow/libDS_Filter.a loader/dmo/libDMO_Filter.a'
|
|
_dep_dshow='loader/dshow/libDS_Filter.a loader/dmo/libDMO_Filter.a'
|
|
_codecmodules="dshow/dmo $_codecmodules"
|
|
else
|
|
_def_dshow='#undef USE_DIRECTSHOW'
|
|
_nocodecmodules="dshow/dmo $_nocodecmodules"
|
|
fi
|
|
|
|
|
|
echocheck "XAnim DLL"
|
|
if test "$_xanim" = auto ; then
|
|
_xanim=no
|
|
if test "$_dl" = yes ; then
|
|
if test -z "$_xanimlibdir" ; then
|
|
for I in "$_libdir/codecs" /usr/local/lib/xanim/mods /usr/lib/xanim/mods /usr/lib/xanim $XANIM_MOD_DIR ; do
|
|
if test -d "$I" ; then
|
|
_xanimlibdir="$I"
|
|
break;
|
|
fi;
|
|
done
|
|
fi
|
|
test "$_xanimlibdir" && _xanim=yes
|
|
if test "$_xanim" = yes ; then
|
|
echores "yes (using $_xanimlibdir)"
|
|
else
|
|
echores "no (no suitable directory found - see DOCS/HTML/$_doc_lang/codecs.html)"
|
|
fi
|
|
else
|
|
echores "no (dynamic loader support needed)"
|
|
fi
|
|
else
|
|
echores "$_xanim (using $_xanimlibdir)"
|
|
fi
|
|
if test "$_xanim" = yes ; then
|
|
_def_xanim='#define USE_XANIM 1'
|
|
_def_xanim_path="#define XACODEC_PATH \"$_xanimlibdir\""
|
|
_codecmodules="xanim $_codecmodules"
|
|
else
|
|
_def_xanim='#undef USE_XANIM'
|
|
_def_xanim_path='#undef XACODEC_PATH'
|
|
_nocodecmodules="xanim $_nocodecmodules"
|
|
fi
|
|
|
|
echocheck "RealPlayer DLL"
|
|
if test "$_real" = auto ; then
|
|
_real=no
|
|
if test "$_dl" = yes || test "$_win32" = yes ; then
|
|
# if test "$_dl" = yes ; then
|
|
if linux || freebsd || netbsd || win32 || darwin ; then
|
|
_real=yes
|
|
else
|
|
echores "no (tested only on Linux/FreeBSD/NetBSD/Cygwin/MinGW/Darwin)"
|
|
fi
|
|
if test "$_real" = yes ; then
|
|
if test -z "$_reallibdir" ; then
|
|
for I in "$_libdir/codecs" "$_libdir/real" /usr/lib/real \
|
|
/usr/lib/RealPlayer{9,8,}/Codecs /usr/local/RealPlayer{9,8,}/Codecs \
|
|
/usr/local/lib/RealPlayer{9,8,}/Codecs /opt/RealPlayer{9,8,}/{Real/,}Codecs \
|
|
{~,}/Applications/RealOne\ Player.app/Contents/MacOS/Library/Codecs \
|
|
"$_win32libdir"; do
|
|
if test -d "$I" ; then
|
|
_reallibdir="$I"
|
|
break
|
|
fi
|
|
done
|
|
fi
|
|
test "$_reallibdir" || _real=no
|
|
if test "$_real" = yes ; then
|
|
echores "yes (using $_reallibdir)"
|
|
else
|
|
echores "no (no suitable directory found - see DOCS/HTML/$_doc_lang/codecs.html)"
|
|
fi
|
|
fi
|
|
else
|
|
echores "no (dynamic loader support needed)"
|
|
fi
|
|
else
|
|
echores "$_real (using $_reallibdir)"
|
|
fi
|
|
if test "$_real" = yes ; then
|
|
_def_real='#define USE_REALCODECS 1'
|
|
_def_real_path="#define REALCODEC_PATH \"$_reallibdir\""
|
|
_codecmodules="real $_codecmodules"
|
|
else
|
|
_def_real='#undef USE_REALCODECS'
|
|
_def_real_path="#undef REALCODEC_PATH"
|
|
_nocodecmodules="real $_nocodecmodules"
|
|
fi
|
|
|
|
|
|
echocheck "LIVE.COM Streaming Media libraries"
|
|
if test "$_live" = auto && test "$_network" = yes ; then
|
|
_TMPC=$TMPC
|
|
TMPC=$TMPCPP
|
|
cat >$TMPC <<EOF
|
|
#include <liveMedia.hh>
|
|
#if (LIVEMEDIA_LIBRARY_VERSION_INT < 1090195200)
|
|
#error Please upgrade to version 2004.07.19 or later of the "LIVE.COM Streaming Media" libraries - available from <www.live.com/liveMedia/>
|
|
#endif
|
|
int main(void) {}
|
|
EOF
|
|
|
|
_live_dist=no
|
|
if test -z "$_livelibdir" ; then
|
|
if cc_check -I/usr/include/liveMedia -I/usr/include/UsageEnvironment -I/usr/include/groupsock; then
|
|
_live_dist=yes
|
|
else
|
|
for I in $_libdir/live /usr/lib/live /usr/local/live /usr/local/lib/live; do
|
|
if test -d "$I" ; then
|
|
_livelibdir="$I"
|
|
break;
|
|
fi;
|
|
done
|
|
fi
|
|
fi
|
|
|
|
if test "$_live_dist" = no && test "$_livelibdir" && cc_check -I$_livelibdir/liveMedia/include \
|
|
-I$_livelibdir/UsageEnvironment/include -I$_livelibdir/groupsock/include; then
|
|
_live=yes
|
|
else
|
|
_live=no
|
|
fi
|
|
TMPC=$_TMPC
|
|
fi
|
|
if test "$_live" = yes && test "$_network" = yes ; then
|
|
echores "yes (using $_livelibdir)"
|
|
_def_live='#define STREAMING_LIVE_DOT_COM 1'
|
|
_live_libs_def="# LIVE.COM Streaming Media libraries:
|
|
LIVE_LIB_DIR = $_livelibdir
|
|
LIVE_LIBS = \$(LIVE_LIB_DIR)/liveMedia/libliveMedia.a
|
|
LIVE_LIBS += \$(LIVE_LIB_DIR)/groupsock/libgroupsock.a
|
|
LIVE_LIBS += \$(LIVE_LIB_DIR)/UsageEnvironment/libUsageEnvironment.a
|
|
LIVE_LIBS += \$(LIVE_LIB_DIR)/BasicUsageEnvironment/libBasicUsageEnvironment.a
|
|
LIVE_LIBS += -lstdc++
|
|
LIVE_INCLUDES = -I\$(LIVE_LIB_DIR)/liveMedia/include
|
|
LIVE_INCLUDES += -I\$(LIVE_LIB_DIR)/UsageEnvironment/include
|
|
LIVE_INCLUDES += -I\$(LIVE_LIB_DIR)/BasicUsageEnvironment/include
|
|
LIVE_INCLUDES += -I\$(LIVE_LIB_DIR)/groupsock/include"
|
|
_ld_live='$(LIVE_LIBS)'
|
|
_inputmodules="live.com $_inputmodules"
|
|
elif test "$_live_dist" = yes && test "$_network" = yes ; then
|
|
echores "yes (using distribution version)"
|
|
_live="yes"
|
|
_def_live='#define STREAMING_LIVE_DOT_COM 1'
|
|
_live_libs_def="# LIVE.COM Streaming Media libraries:
|
|
LIVE_LIB_DIR = $_livelibdir
|
|
LIVE_LIBS = -lliveMedia
|
|
LIVE_LIBS += -lgroupsock
|
|
LIVE_LIBS += -lUsageEnvironment
|
|
LIVE_LIBS += -lBasicUsageEnvironment
|
|
LIVE_LIBS += -lstdc++
|
|
LIVE_INCLUDES = -I/usr/include/liveMedia
|
|
LIVE_INCLUDES += -I/usr/include/UsageEnvironment
|
|
LIVE_INCLUDES += -I/usr/include/BasicUsageEnvironment
|
|
LIVE_INCLUDES += -I/usr/include/groupsock"
|
|
_ld_live='$(LIVE_LIBS)'
|
|
_inputmodules="live.com $_inputmodules"
|
|
else
|
|
echores "no"
|
|
_def_live='#undef STREAMING_LIVE_DOT_COM'
|
|
_noinputmodules="live.com $_noinputmodules"
|
|
fi
|
|
|
|
|
|
echocheck "FFmpeg libavcodec (static)"
|
|
if test "$_libavcodec" = auto ; then
|
|
# Note: static linking is preferred to dynamic linking
|
|
_libavcodec=no
|
|
if test -d libavcodec && test -f libavcodec/utils.c ; then
|
|
if grep avcodec_find_encoder_by_name libavcodec/utils.c > /dev/null 2>&1 ; then
|
|
_libavcodec=yes
|
|
echores "yes"
|
|
else
|
|
echores "no (old ffmpeg version, use CVS !)"
|
|
fi
|
|
else
|
|
echores "no (see DOCS/HTML/$_doc_lang/codecs.html)"
|
|
fi
|
|
else
|
|
echores "$_libavcodec"
|
|
fi
|
|
|
|
echocheck "FFmpeg libavformat (static)"
|
|
if test "$_libavformat" = auto ; then
|
|
# Note: static linking is preferred to dynamic linking
|
|
_libavformat=no
|
|
if test -d libavformat && test -f libavformat/utils.c ; then
|
|
_libavformat=yes
|
|
echores "yes"
|
|
else
|
|
echores "no"
|
|
fi
|
|
else
|
|
echores "$_libavformat"
|
|
fi
|
|
|
|
_def_haveffpostprocess='no'
|
|
if test -d libavcodec && test -f libavcodec/libpostproc/postprocess.h ; then
|
|
_def_haveffpostprocess='yes'
|
|
fi
|
|
|
|
if test "$_libavcodec" != yes ; then
|
|
echocheck "FFmpeg libavcodec (dynamic)"
|
|
if test "$_libavcodecso" = auto ; then
|
|
_libavcodecso=no
|
|
# FIXME : check for avcodec_find_encoder_by_name() for mencoder
|
|
cat > $TMPC << EOF
|
|
#define FF_POSTPROCESS 1
|
|
#include <ffmpeg/avcodec.h>
|
|
int main(void) {
|
|
avcodec_find_encoder_by_name("");
|
|
return 0;
|
|
}
|
|
EOF
|
|
if cc_check -lavcodec $_ld_lm ; then
|
|
_libavcodecso=yes
|
|
echores "yes (using libavcodec.so)"
|
|
else
|
|
echores "no (libavcodec.so is broken/obsolete)"
|
|
fi
|
|
else
|
|
echores "$_libavcodecso"
|
|
fi
|
|
fi
|
|
|
|
_def_libavcodec='#undef USE_LIBAVCODEC'
|
|
_def_libavcodecso='#undef USE_LIBAVCODEC_SO'
|
|
_def_ffpostprocess='#undef FF_POSTPROCESS'
|
|
if test "$_libavcodec" = yes ; then
|
|
_def_libavcodec='#define USE_LIBAVCODEC 1'
|
|
_ld_libavcodec='libavcodec/libavcodec.a'
|
|
_dep_libavcodec='libavcodec/libavcodec.a'
|
|
_def_ffpostprocess='#define FF_POSTPROCESS 1'
|
|
_codecmodules="libavcodec $_codecmodules"
|
|
elif test "$_libavcodecso" = yes ; then
|
|
_def_libavcodec='#define USE_LIBAVCODEC 1'
|
|
_def_libavcodecso='#define USE_LIBAVCODEC_SO 1'
|
|
_ld_libavcodec='-lavcodec'
|
|
_codecmodules="libavcodec.so $_codecmodules"
|
|
else
|
|
_nocodecmodules="libavcodec $_nocodecmodules"
|
|
fi
|
|
|
|
_def_libavformat='#undef USE_LIBAVFORMAT'
|
|
_def_libavformat_win32='#undef CONFIG_WIN32'
|
|
if test "$_libavformat" = yes ; then
|
|
_def_libavformat='#define USE_LIBAVFORMAT 1'
|
|
_ld_libavformat='libavformat/libavformat.a'
|
|
_dep_libavformat='libavformat/libavformat.a'
|
|
if win32 ; then
|
|
_def_libavformat_win32='#define CONFIG_WIN32 1'
|
|
fi
|
|
fi
|
|
|
|
echocheck "amr narrowband"
|
|
_echomsg=""
|
|
if test "$_amr_nb" = auto ; then
|
|
if test -f libavcodec/amr_float/sp_dec.c ; then
|
|
if test "$_libavcodec" = yes ; then
|
|
_amr_nb=yes
|
|
else
|
|
_amr_nb=no
|
|
_echomsg "libavcodec (static) is required by amr_nb, sorry"
|
|
fi
|
|
else
|
|
_amr_nb=no
|
|
fi
|
|
fi
|
|
if test "$_amr_nb" = yes ; then
|
|
_def_amr_nb='#define AMR_NB 1'
|
|
else
|
|
_def_amr_nb='#undef AMR_NB'
|
|
fi
|
|
echores "$_amr_nb $_echomsg"
|
|
|
|
echocheck "amr narrowband, fixed point"
|
|
_echomsg=""
|
|
if test "$_amr_nb_fixed" = auto ; then
|
|
if test -f libavcodec/amr/dtx_dec.c ; then
|
|
if test "$_libavcodec" = yes ; then
|
|
if test "$_amr_nb" = no ; then
|
|
_amr_nb_fixed=yes
|
|
else
|
|
_amr_nb_fixed=no
|
|
_echomsg="(disabled by amr_nb)"
|
|
fi
|
|
else
|
|
_amr_nb_fixed=no
|
|
_echomsg "libavcodec (static) is required by amr_nb-fixed, sorry"
|
|
fi
|
|
else
|
|
_amr_nb_fixed=no
|
|
fi
|
|
fi
|
|
if test "$_amr_nb_fixed" = yes ; then
|
|
_def_amr_nb='#define AMR_NB 1'
|
|
_def_amr_nb_fixed='#define AMR_NB_FIXED 1'
|
|
_amr_nb=yes;
|
|
else
|
|
_def_amr_nb_fixed='#undef AMR_NB_FIXED'
|
|
fi
|
|
echores "$_amr_nb_fixed $_echomsg"
|
|
|
|
if test "$_amr_nb" = yes ; then
|
|
_codecmodules="amr_nb $_codecmodules"
|
|
else
|
|
_nocodecmodules="amr_nb $_nocodecmodules"
|
|
fi
|
|
|
|
echocheck "amr wideband"
|
|
_echomsg=""
|
|
if test "$_amr_wb" = auto ; then
|
|
if test -f libavcodec/amrwb_float/dec_dtx.c ; then
|
|
if test "$_libavcodec" = yes ; then
|
|
_amr_wb=yes
|
|
else
|
|
_amr_wb=no
|
|
_echomsg="libavcodec (static) is required by amr_wb, sorry"
|
|
fi
|
|
else
|
|
_amr_wb=no
|
|
fi
|
|
fi
|
|
if test "$_amr_wb" = yes ; then
|
|
_def_amr_wb='#define AMR_WB 1'
|
|
_codecmodules="amr_wb $_codecmodules"
|
|
else
|
|
_def_amr_wb='#undef AMR_WB'
|
|
_nocodecmodules="amr_wb $_nocodecmodules"
|
|
fi
|
|
echores "$_amr_wb $_echomsg"
|
|
|
|
echocheck "libdv-0.9.5+"
|
|
if test "$_libdv" = auto ; then
|
|
_libdv=no
|
|
cat > $TMPC <<EOF
|
|
#include <libdv/dv.h>
|
|
int main(void) { dv_encoder_t* enc=dv_encoder_new(1,1,1); return 0; }
|
|
EOF
|
|
cc_check -ldv $_ld_lm && _libdv=yes
|
|
fi
|
|
if test "$_libdv" = yes ; then
|
|
_def_libdv='#define HAVE_LIBDV095 1'
|
|
_ld_libdv="-ldv"
|
|
_codecmodules="libdv $_codecmodules"
|
|
else
|
|
_def_libdv='#undef HAVE_LIBDV095'
|
|
_nocodecmodules="libdv $_nocodecmodules"
|
|
fi
|
|
echores "$_libdv"
|
|
|
|
echocheck "zr"
|
|
if test "$_zr" = auto ; then
|
|
#36067's seem to identify themselves as 36057PQC's, so the line
|
|
#below should work for 36067's and 36057's.
|
|
if grep -e "Multimedia video controller: Zoran Corporation ZR36057" /proc/pci > /dev/null 2>&1; then
|
|
_zr=yes
|
|
else
|
|
_zr=no
|
|
fi
|
|
fi
|
|
if test "$_zr" = yes ; then
|
|
if test "$_libavcodec" = yes ; then
|
|
_def_zr='#define HAVE_ZR 1'
|
|
_vosrc="$_vosrc vo_zr2.c vo_zr.c jpeg_enc.c"
|
|
_vomodules="zr zr2 $_vomodules"
|
|
echores "$_zr"
|
|
else
|
|
echores "libavcodec (static) is required by zr, sorry"
|
|
_novomodules="zr $_novomodules"
|
|
_def_zr='#undef HAVE_ZR'
|
|
fi
|
|
else
|
|
_def_zr='#undef HAVE_ZR'
|
|
_novomodules="zr zr2 $_novomodules"
|
|
echores "$_zr"
|
|
fi
|
|
|
|
echocheck "bl"
|
|
if test "$_bl" = yes ; then
|
|
_def_bl='#define HAVE_BL 1'
|
|
_vosrc="$_vosrc vo_bl.c"
|
|
_vomodules="bl $_vomodules"
|
|
else
|
|
_def_bl='#undef HAVE_BL'
|
|
_novomodules="bl $_novomodules"
|
|
fi
|
|
echores "$_bl"
|
|
|
|
echocheck "XviD"
|
|
cat > $TMPC << EOF
|
|
#include <xvid.h>
|
|
int main(void) { xvid_init(0, 0, 0, 0); return 0; }
|
|
EOF
|
|
_ld_xvid="$_ld_xvid -lxvidcore $_ld_lm"
|
|
if test "$_xvid" != no && cc_check $_inc_xvid $_ld_xvid ; then
|
|
_xvid=yes
|
|
_def_xvid3='#define HAVE_XVID3 1'
|
|
_def_xvid4='#undef HAVE_XVID4'
|
|
_codecmodules="xvid $_codecmodules"
|
|
else
|
|
cat > $TMPC << EOF
|
|
#include <xvid.h>
|
|
int main(void) { xvid_global(0, 0, 0, 0); return 0; }
|
|
EOF
|
|
if test "$_xvid" != no && cc_check $_inc_xvid $_ld_xvid ; then
|
|
_xvid=yes
|
|
_def_xvid3='#undef HAVE_XVID3'
|
|
_def_xvid4='#define HAVE_XVID4 1'
|
|
_codecmodules="xvid $_codecmodules"
|
|
else
|
|
_xvid=no
|
|
_ld_xvid=''
|
|
_def_xvid3='#undef HAVE_XVID3'
|
|
_def_xvid4='#undef HAVE_XVID4'
|
|
_nocodecmodules="xvid $_nocodecmodules"
|
|
fi
|
|
fi
|
|
echores "$_xvid"
|
|
|
|
_xvidcompat=no
|
|
_def_decore_xvid='#undef DECORE_XVID'
|
|
_def_encore_xvid='#undef ENCORE_XVID'
|
|
if test "$_xvid" = yes ; then
|
|
echocheck "DivX4 compatibility in XviD"
|
|
cat > $TMPC << EOF
|
|
#include <divx4.h>
|
|
int main(void) { (void) decore(0, 0, 0, 0); return 0; }
|
|
EOF
|
|
cc_check $_ld_lm "$_ld_xvid" && _xvidcompat=yes
|
|
echores "$_xvidcompat"
|
|
fi
|
|
|
|
echocheck "x264"
|
|
cat > $TMPC << EOF
|
|
#include <inttypes.h>
|
|
#include <x264.h>
|
|
#if X264_BUILD < 29
|
|
#error We do not support old versions of x264. Get the latest from SVN.
|
|
#endif
|
|
int main(void) { x264_encoder_open((void*)0); return 0; }
|
|
EOF
|
|
_ld_x264="$_ld_x264 -lx264 $_ld_lm $_ld_pthread"
|
|
if test "$_x264" != no && cc_check $_inc_x264 $_ld_x264 ; then
|
|
_x264=yes
|
|
_def_x264='#define HAVE_X264 1'
|
|
_codecmodules="x264 $_codecmodules"
|
|
else
|
|
_x264=no
|
|
_ld_x264=''
|
|
_def_x264='#undef HAVE_X264'
|
|
_nocodecmodules="x264 $_nocodecmodules"
|
|
fi
|
|
echores "$_x264"
|
|
|
|
echocheck "DivX4linux/DivX5linux/OpenDivX decore"
|
|
# DivX5: DEC_OPT_MEMORY_REQS - DivX4: DEC_OPT_FRAME_311
|
|
cat > $TMPC << EOF
|
|
#include <decore.h>
|
|
int main(void) { (void) decore(0, 0, 0, 0); return DEC_OPT_FRAME_311; }
|
|
EOF
|
|
if test "$_divx4linux" != no && cc_check $_ld_lm -ldivxdecore ; then
|
|
_opendivx=no
|
|
_ld_decore='-ldivxdecore'
|
|
_def_decore='#define NEW_DECORE 1'
|
|
_def_divx='#define USE_DIVX'
|
|
_def_divx5='#undef DECORE_DIVX5'
|
|
_def_odivx_postprocess='#undef HAVE_ODIVX_POSTPROCESS'
|
|
_codecmodules="divx4linux $_codecmodules"
|
|
echores "DivX4linux (with libdivxdecore.so)"
|
|
else
|
|
# if test "$_divx4linux" != no ; then
|
|
# DivX5 check
|
|
# OdivxPP disabled because of:
|
|
# ld: Warning: type of symbol `dering' changed from 1 to 2 in opendivx/postprocess.o
|
|
cat > $TMPC << EOF
|
|
#include <decore.h>
|
|
int main(void) { (void) decore(0, 0, 0, 0); return DEC_OPT_INIT; }
|
|
EOF
|
|
if test "$_divx4linux" != no && cc_check $_ld_lm -ldivxdecore ; then
|
|
_opendivx=no
|
|
# _ld_decore='-ldivxdecore opendivx/postprocess.o'
|
|
_ld_decore='-ldivxdecore'
|
|
_def_decore='#define NEW_DECORE 1'
|
|
_def_divx='#define USE_DIVX'
|
|
_def_divx5='#define DECORE_DIVX5 1'
|
|
# _def_odivx_postprocess='#define HAVE_ODIVX_POSTPROCESS 1'
|
|
_def_odivx_postprocess='#undef HAVE_ODIVX_POSTPROCESS'
|
|
_codecmodules="divx5linux $_codecmodules"
|
|
_nocodecmodules="divx4linux $_nocodecmodules"
|
|
echores "DivX5linux (with libdivxdecore.so)"
|
|
elif test "$_opendivx" != no ; then
|
|
_opendivx=yes
|
|
_ld_decore='opendivx/libdecore.a'
|
|
_def_decore='#undef NEW_DECORE'
|
|
_def_divx='#define USE_DIVX'
|
|
_def_divx5='#undef DECORE_DIVX5'
|
|
_def_odivx_postprocess='#define HAVE_ODIVX_POSTPROCESS 1'
|
|
_codecmodules="opendivx $_codecmodules"
|
|
_nocodecmodules="divx5linux $_nocodecmodules"
|
|
echores "OpenDivX"
|
|
elif test "$_xvidcompat" = yes ; then
|
|
_opendivx=no
|
|
_ld_decore=''
|
|
_def_decore='#define NEW_DECORE 1'
|
|
_def_divx='#define USE_DIVX 1'
|
|
_def_divx5='#undef DECORE_DIVX5'
|
|
_def_decore_xvid='#define DECORE_XVID 1'
|
|
_def_odivx_postprocess='#undef HAVE_ODIVX_POSTPROCESS'
|
|
_nocodecmodules="opendivx divx5linux divx4linux $_nocodecmodules"
|
|
echores "XviD compat."
|
|
else
|
|
_opendivx=no
|
|
_ld_decore=''
|
|
_def_decore='#undef NEW_DECORE'
|
|
_def_divx='#undef USE_DIVX'
|
|
_def_divx5='#undef DECORE_DIVX5'
|
|
_def_odivx_postprocess='#undef HAVE_ODIVX_POSTPROCESS'
|
|
_nocodecmodules="opendivx $_nocodecmodules"
|
|
echores "no"
|
|
fi # DivX5 check
|
|
fi
|
|
|
|
|
|
# mencoder requires (optional) those libs: libmp3lame and divx4linux encore
|
|
if test "$_mencoder" != no ; then
|
|
|
|
echocheck "libmp3lame (for mencoder)"
|
|
_mp3lame=no
|
|
cat > $TMPC <<EOF
|
|
#include <lame/lame.h>
|
|
int main(void) { lame_version_t lv; (void) lame_init(); get_lame_version_numerical(&lv); printf("%d%d\n",lv.major,lv.minor); return 0; }
|
|
EOF
|
|
# Note: libmp3lame usually depends on vorbis
|
|
cc_check -lmp3lame $_ld_vorbis $_ld_lm && ( "$TMPO" >> "$TMPLOG" 2>&1 ) && _mp3lame=yes
|
|
if test "$_mp3lame" = yes ; then
|
|
_def_mp3lame="#define HAVE_MP3LAME `$TMPO`"
|
|
_def_cfg_mp3lame="#define CONFIG_MP3LAME `$TMPO`"
|
|
_ld_mp3lame="-lmp3lame $_ld_vorbis"
|
|
else
|
|
_def_mp3lame='#undef HAVE_MP3LAME'
|
|
_def_cfg_mp3lame='#undef CONFIG_MP3LAME'
|
|
fi
|
|
echores "$_mp3lame"
|
|
|
|
|
|
echocheck "DivX4linux encore (for mencoder)"
|
|
cat > $TMPC << EOF
|
|
#include <encore2.h>
|
|
int main(void) { (void) encore(0, 0, 0, 0); return 0; }
|
|
EOF
|
|
if test "$_divx4linux" != no && cc_check -ldivxencore $_ld_lm ; then
|
|
_def_encore='#define HAVE_DIVX4ENCORE 1'
|
|
_ld_encore='-ldivxencore'
|
|
echores "DivX4linux (with libdivxencore.so)"
|
|
elif test "$_xvidcompat" = yes ; then
|
|
_def_encore='#define HAVE_DIVX4ENCORE 1'
|
|
_ld_encore=''
|
|
_def_encore_xvid='#define ENCORE_XVID 1'
|
|
echores "XviD compat."
|
|
else
|
|
_def_encore='#undef HAVE_DIVX4ENCORE'
|
|
echores "no"
|
|
fi
|
|
|
|
fi
|
|
|
|
echocheck "mencoder"
|
|
_mencoder_flag='#undef HAVE_MENCODER'
|
|
if test "$_mencoder" = yes ; then
|
|
_mencoder_flag='#define HAVE_MENCODER'
|
|
fi
|
|
echores "$_mencoder"
|
|
|
|
echocheck "fastmemcpy"
|
|
# fastmemcpy check is done earlier with tests of CPU & binutils features
|
|
if test "$_fastmemcpy" = yes ; then
|
|
_def_fastmemcpy='#define USE_FASTMEMCPY 1'
|
|
else
|
|
_def_fastmemcpy='#undef USE_FASTMEMCPY'
|
|
fi
|
|
echores "$_fastmemcpy"
|
|
|
|
echocheck "UniquE RAR File Library"
|
|
if test "$_unrarlib" = yes ; then
|
|
_def_unrarlib='#define USE_UNRARLIB 1'
|
|
else
|
|
_def_unrarlib='#undef USE_UNRARLIB'
|
|
fi
|
|
echores "$_unrarlib"
|
|
|
|
echocheck "TV interface"
|
|
if test "$_tv" = yes ; then
|
|
_def_tv='#define USE_TV 1'
|
|
_inputmodules="tv $_inputmodules"
|
|
else
|
|
_noinputmodules="tv $_noinputmodules"
|
|
_def_tv='#undef USE_TV'
|
|
fi
|
|
echores "$_tv"
|
|
|
|
echocheck "EDL support"
|
|
if test "$_edl" = yes ; then
|
|
_def_edl='#define USE_EDL'
|
|
_inputmodules="edl $_inputmodules"
|
|
else
|
|
_noinputmodules="edl $_noinputmodules"
|
|
_def_edl='#undef USE_EDL'
|
|
fi
|
|
echores "$_edl"
|
|
|
|
echocheck "*BSD BrookTree 848 TV interface"
|
|
if test "$_tv_bsdbt848" = auto ; then
|
|
_tv_bsdbt848=no
|
|
if test "$_tv" = yes ; then
|
|
cat > $TMPC <<EOF
|
|
#include <sys/types.h>
|
|
#if defined(__NetBSD__)
|
|
#include <dev/ic/bt8xx.h>
|
|
#else
|
|
#include <machine/ioctl_bt848.h>
|
|
#endif
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check && _tv_bsdbt848=yes
|
|
fi
|
|
fi
|
|
if test "$_tv_bsdbt848" = yes ; then
|
|
_def_tv_bsdbt848='#define HAVE_TV_BSDBT848 1'
|
|
_inputmodules="tv-bsdbt848 $_inputmodules"
|
|
else
|
|
_def_tv_bsdbt848='#undef HAVE_TV_BSDBT848'
|
|
_noinputmodules="tv-bsdbt848 $_noinputmodules"
|
|
fi
|
|
echores "$_tv_bsdbt848"
|
|
|
|
echocheck "Video 4 Linux TV interface"
|
|
if test "$_tv_v4l" = auto ; then
|
|
_tv_v4l=no
|
|
if test "$_tv" = yes && linux ; then
|
|
for I in /dev/video /dev/video? ; do
|
|
if test -c $I ; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdlib.h>
|
|
#include <linux/videodev.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check && _tv_v4l=yes
|
|
break
|
|
fi
|
|
done
|
|
fi
|
|
fi
|
|
if test "$_tv_v4l" = yes ; then
|
|
_def_tv_v4l='#define HAVE_TV_V4L 1'
|
|
_inputmodules="tv-v4l $_inputmodules"
|
|
else
|
|
_noinputmodules="tv-v4l $_noinputmodules"
|
|
_def_tv_v4l='#undef HAVE_TV_V4L'
|
|
fi
|
|
echores "$_tv_v4l"
|
|
|
|
|
|
echocheck "Video 4 Linux 2 TV interface"
|
|
if test "$_tv_v4l2" = auto ; then
|
|
_tv_v4l2=no
|
|
if test "$_tv" = yes && linux ; then
|
|
for I in /dev/video /dev/video? ; do
|
|
if test -c $I ; then
|
|
_tv_v4l2=yes
|
|
break
|
|
fi
|
|
done
|
|
fi
|
|
fi
|
|
if test "$_tv_v4l2" = yes ; then
|
|
_def_tv_v4l2='#define HAVE_TV_V4L2 1'
|
|
_inputmodules="tv-v4l2 $_inputmodules"
|
|
else
|
|
_noinputmodules="tv-v4l2 $_noinputmodules"
|
|
_def_tv_v4l2='#undef HAVE_TV_V4L2'
|
|
fi
|
|
echores "$_tv_v4l2"
|
|
|
|
|
|
echocheck "audio select()"
|
|
if test "$_select" = no ; then
|
|
_def_select='#undef HAVE_AUDIO_SELECT'
|
|
elif test "$_select" = yes ; then
|
|
_def_select='#define HAVE_AUDIO_SELECT 1'
|
|
fi
|
|
echores "$_select"
|
|
|
|
|
|
echocheck "network"
|
|
# FIXME network check
|
|
if test "$_network" != no ; then
|
|
_def_network='#define MPLAYER_NETWORK 1'
|
|
_ld_network="$_ld_sock"
|
|
_inputmodules="network $_inputmodules"
|
|
else
|
|
_noinputmodules="network $_noinputmodules"
|
|
_def_network='#undef MPLAYER_NETWORK'
|
|
_ftp=no
|
|
fi
|
|
echores "$_network"
|
|
|
|
echocheck "ftp"
|
|
if (not beos) && (test "$_ftp" != no) ; then
|
|
_def_ftp='#define HAVE_FTP 1'
|
|
_inputmodules="ftp $_inputmodules"
|
|
else
|
|
_noinputmodules="ftp $_noinputmodules"
|
|
_def_ftp='#undef HAVE_FTP'
|
|
fi
|
|
echores "$_ftp"
|
|
|
|
echocheck "vstream client"
|
|
if test "$_vstream" = auto ; then
|
|
_vstream=no
|
|
cat > $TMPC <<EOF
|
|
#include <vstream-client.h>
|
|
void vstream_error(const char *format, ... ) {}
|
|
int main(void) { vstream_start(); return 0; }
|
|
EOF
|
|
cc_check -lvstream-client && _vstream=yes
|
|
fi
|
|
if test "$_vstream" = yes ; then
|
|
_def_vstream='#define HAVE_VSTREAM 1'
|
|
_inputmodules="vstream $_inputmodules"
|
|
_ld_vstream='-lvstream-client'
|
|
else
|
|
_noinputmodules="vstream $_noinputmodules"
|
|
_def_vstream='#undef HAVE_VSTREAM'
|
|
fi
|
|
echores "$_vstream"
|
|
|
|
# endian testing
|
|
echocheck "byte order"
|
|
if test "$_big_endian" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
short ascii_name[] = { (('M'<<8)|'P'),(('l'<<8)|'a'),(('y'<<8)|'e'),(('r'<<8)|'B'),
|
|
(('i'<<8)|'g'),(('E'<<8)|'n'),(('d'<<8)|'i'),(('a'<<8)|'n'),0};
|
|
int main(){
|
|
char* s = (char*)ascii_name;
|
|
return 0;
|
|
}
|
|
EOF
|
|
if cc_check ; then
|
|
if strings $TMPO | grep -l MPlayerBigEndian >/dev/null ; then
|
|
_big_endian=yes
|
|
else
|
|
_big_endian=no
|
|
fi
|
|
else
|
|
echo -n "failed to autodetect byte order, defaulting to "
|
|
fi
|
|
fi
|
|
if test "$_big_endian" = yes ; then
|
|
_byte_order='big-endian'
|
|
_def_words_endian='#define WORDS_BIGENDIAN 1'
|
|
else
|
|
_byte_order='little-endian'
|
|
_def_words_endian='#undef WORDS_BIGENDIAN'
|
|
fi
|
|
echores "$_byte_order"
|
|
|
|
echocheck "shared postprocess lib"
|
|
echores "$_shared_pp"
|
|
|
|
echocheck "OSD menu"
|
|
if test "$_menu" = yes ; then
|
|
_def_menu='#define HAVE_MENU 1'
|
|
else
|
|
_def_menu='#undef HAVE_MENU'
|
|
fi
|
|
echores "$_menu"
|
|
|
|
# Check to see if they want QTX codecs enabled
|
|
echocheck "QTX codecs"
|
|
if test "$_qtx" = auto ; then
|
|
_qtx=$_win32
|
|
fi
|
|
if test "$_qtx" = yes ; then
|
|
_def_qtx='#define USE_QTX_CODECS 1'
|
|
_codecmodules="qtx $_codecmodules"
|
|
else
|
|
_def_qtx='#undef USE_QTX_CODECS'
|
|
_nocodecmodules="qtx $_nocodecmodules"
|
|
fi
|
|
echores "$_qtx"
|
|
|
|
|
|
echocheck "Subtitles sorting"
|
|
if test "$_sortsub" = yes ; then
|
|
_def_sortsub='#define USE_SORTSUB 1'
|
|
else
|
|
_def_sortsub='#undef USE_SORTSUB'
|
|
fi
|
|
echores "$_sortsub"
|
|
|
|
|
|
echocheck "XMMS inputplugin support"
|
|
if test "$_xmms" = yes ; then
|
|
|
|
if ( xmms-config --version ) >/dev/null 2>&1 ; then
|
|
if test -z "$_xmmsplugindir" ; then
|
|
_xmmsplugindir=`xmms-config --input-plugin-dir`
|
|
fi
|
|
if test -z "$_xmmslibdir" ; then
|
|
_xmmslibdir=`xmms-config --exec-prefix`/lib
|
|
fi
|
|
else
|
|
if test -z "$_xmmsplugindir" ; then
|
|
_xmmsplugindir=/usr/lib/xmms/Input
|
|
fi
|
|
if test -z "$_xmmslibdir" ; then
|
|
_xmmslibdir=/usr/lib
|
|
fi
|
|
fi
|
|
|
|
_def_xmms='#define HAVE_XMMS 1'
|
|
if darwin ; then
|
|
_xmms_lib="${_xmmslibdir}/libxmms.dylib"
|
|
else
|
|
_xmms_lib="${_xmmslibdir}/libxmms.so.1 -export-dynamic"
|
|
fi
|
|
else
|
|
_def_xmms='#undef HAVE_XMMS'
|
|
fi
|
|
echores "$_xmms"
|
|
|
|
echocheck "inet6"
|
|
if test "$_inet6" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/socket.h>
|
|
int main(void) { socket(AF_INET6, SOCK_STREAM, AF_INET6); }
|
|
EOF
|
|
_inet6=no
|
|
if cc_check ; then
|
|
_inet6=yes
|
|
fi
|
|
fi
|
|
if test "$_inet6" = yes ; then
|
|
_def_inet6='#define HAVE_AF_INET6 1'
|
|
else
|
|
_def_inet6='#undef HAVE_AF_INET6'
|
|
fi
|
|
echores "$_inet6"
|
|
|
|
|
|
echocheck "gethostbyname2"
|
|
if test "$_gethostbyname2" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/socket.h>
|
|
#include <netdb.h>
|
|
int main(void) { gethostbyname2("", AF_INET); }
|
|
EOF
|
|
_gethostbyname2=no
|
|
if cc_check ; then
|
|
_gethostbyname2=yes
|
|
fi
|
|
fi
|
|
|
|
if test "$_gethostbyname2" = yes ; then
|
|
_def_gethostbyname2='#define HAVE_GETHOSTBYNAME2 1'
|
|
else
|
|
_def_gethostbyname2='#undef HAVE_GETHOSTBYNAME2'
|
|
fi
|
|
echores "$_gethostbyname2"
|
|
|
|
# --------------- GUI specific tests begin -------------------
|
|
echocheck "GUI"
|
|
echo "$_gui"
|
|
if test "$_gui" = yes ; then
|
|
|
|
# Required libraries
|
|
test "$_png" != yes && die "PNG support required for GUI compilation, please install libpng and libpng-dev packages."
|
|
test "$_x11" != yes && die "X11 support required for GUI compilation"
|
|
|
|
echocheck "XShape extension"
|
|
_xshape=no
|
|
if test "$_x11" = yes ; then
|
|
cat > $TMPC << EOF
|
|
#include <X11/Xlib.h>
|
|
#include <X11/Xproto.h>
|
|
#include <X11/Xutil.h>
|
|
#include <X11/extensions/shape.h>
|
|
#include <stdlib.h>
|
|
int main(void) {
|
|
char *name = ":0.0";
|
|
Display *wsDisplay;
|
|
int exitvar = 0;
|
|
int eventbase, errorbase;
|
|
if (getenv("DISPLAY"))
|
|
name=getenv("DISPLAY");
|
|
wsDisplay=XOpenDisplay(name);
|
|
if (!XShapeQueryExtension(wsDisplay,&eventbase,&errorbase))
|
|
exitvar=1;
|
|
XCloseDisplay(wsDisplay);
|
|
return exitvar;
|
|
}
|
|
EOF
|
|
cc_check $_inc_x11 $_ld_x11 && _xshape=yes
|
|
fi
|
|
if test "$_xshape" = yes ; then
|
|
_def_xshape='#define HAVE_XSHAPE 1'
|
|
else
|
|
die "The GUI requires the X11 extension XShape (which was not found)."
|
|
fi
|
|
echores "$_xshape"
|
|
|
|
|
|
# Check for GTK:
|
|
echocheck "GTK version"
|
|
if test -z "$_gtkconfig" ; then
|
|
if ( gtk-config --version ) >/dev/null 2>&1 ; then
|
|
_gtkconfig="gtk-config"
|
|
elif ( gtk12-config --version ) >/dev/null 2>&1 ; then
|
|
_gtkconfig="gtk12-config"
|
|
else
|
|
die "The GUI requires GTK devel packages (which were not found)."
|
|
fi
|
|
fi
|
|
_gtk=`$_gtkconfig --version 2>&1`
|
|
_inc_gtk=`$_gtkconfig --cflags 2>&1`
|
|
_ld_gtk=`$_gtkconfig --libs 2>&1`
|
|
echores "$_gtk (using $_gtkconfig)"
|
|
|
|
# Check for GLIB
|
|
echocheck "glib version"
|
|
if test -z "$_glibconfig" ; then
|
|
if ( glib-config --version ) >/dev/null 2>&1 ; then
|
|
_glibconfig="glib-config"
|
|
elif ( glib12-config --version ) >/dev/null 2>&1 ; then
|
|
_glibconfig="glib12-config"
|
|
else
|
|
die "The GUI requires GLib devel packages (which were not found)"
|
|
fi
|
|
fi
|
|
_glib=`$_glibconfig --version 2>&1`
|
|
_inc_glib=`$_glibconfig --cflags 2>&1`
|
|
_ld_glib=`$_glibconfig --libs 2>&1`
|
|
echores "$_glib (using $_glibconfig)"
|
|
|
|
_def_gui='#define HAVE_NEW_GUI 1'
|
|
_ld_gui='$(GTKLIB) $(GLIBLIB)'
|
|
|
|
echo "Creating Gui/config.mak"
|
|
cat > Gui/config.mak << EOF
|
|
# -------- Generated by configure -----------
|
|
|
|
GTKINC = $_inc_gtk
|
|
GTKLIBS = $_ld_gtk
|
|
GLIBINC = $_inc_glib
|
|
GLIBLIBS = $_ld_glib
|
|
|
|
EOF
|
|
|
|
else
|
|
_def_gui='#undef HAVE_NEW_GUI'
|
|
fi
|
|
# --------------- GUI specific tests end -------------------
|
|
|
|
|
|
|
|
#############################################################################
|
|
|
|
# Checking for CFLAGS
|
|
_stripbinaries=yes
|
|
if test "$_profile" != "" || test "$_debug" != "" ; then
|
|
CFLAGS="-W -Wall -O2 $_march $_mcpu $_debug $_profile"
|
|
if test "$_cc_major" -ge "3" ; then
|
|
CFLAGS=`echo "$CFLAGS" | sed -e 's/\(-Wall\)/\1 -Wno-unused-parameter/'`
|
|
fi
|
|
_stripbinaries=no
|
|
elif test -z "$CFLAGS" ; then
|
|
CFLAGS="-O4 $_march $_mcpu $_pipe -ffast-math -fomit-frame-pointer"
|
|
# always compile with '-g' if .developer:
|
|
if test -f ".developer" ; then
|
|
CFLAGS="-g $CFLAGS"
|
|
if (test "$_crash_debug" = auto) && (not mingw32) ; then
|
|
_crash_debug=yes
|
|
fi
|
|
_stripbinaries=no
|
|
fi
|
|
else
|
|
cat <<EOF
|
|
|
|
MPlayer compilation will use the CFLAGS set by you, but:
|
|
|
|
*** *** DO NOT REPORT BUGS IF IT DOES NOT COMPILE/WORK! *** ***
|
|
|
|
It is strongly recommended to let MPlayer choose the correct CFLAGS!
|
|
To do so, execute 'CFLAGS= ./configure <options>'
|
|
|
|
EOF
|
|
fi
|
|
|
|
echocheck "automatic gdb attach"
|
|
if test "$_crash_debug" = yes ; then
|
|
_def_crash_debug='#define CRASH_DEBUG 1'
|
|
else
|
|
_def_crash_debug='#undef CRASH_DEBUG'
|
|
_crash_debug=no
|
|
fi
|
|
echores "$_crash_debug"
|
|
|
|
if darwin ; then
|
|
CFLAGS="$CFLAGS -mdynamic-no-pic -falign-loops=16 -DSYS_DARWIN"
|
|
if [ "$_cc_major" = 3 ] && [ "$_cc_minor" -lt 1 ]; then
|
|
CFLAGS="$CFLAGS -no-cpp-precomp"
|
|
fi
|
|
|
|
# libavcodec (from ffmpeg) requires CONFIG_DARWIN to enable AltiVec on Darwin/MacOSX
|
|
test "$_altivec" = yes && CFLAGS="$CFLAGS -DCONFIG_DARWIN"
|
|
fi
|
|
if hpux ; then
|
|
# use flag for HPUX missing setenv()
|
|
CFLAGS="$CFLAGS -DHPUX"
|
|
fi
|
|
# Thread support
|
|
if linux ; then
|
|
CFLAGS="$CFLAGS -D_REENTRANT"
|
|
elif bsd ; then
|
|
# FIXME bsd needs this so maybe other OS'es
|
|
CFLAGS="$CFLAGS -D_THREAD_SAFE"
|
|
fi
|
|
# 64 bit file offsets?
|
|
if test "$_largefiles" = yes || freebsd ; then
|
|
CFLAGS="$CFLAGS -D_LARGEFILE_SOURCE -D_FILE_OFFSET_BITS=64"
|
|
if test "$_dvdread" = yes ; then
|
|
# dvdread support requires this (for off64_t)
|
|
CFLAGS="$CFLAGS -D_LARGEFILE64_SOURCE"
|
|
fi
|
|
fi
|
|
|
|
echocheck "compiler support for -fno-PIC"
|
|
if x86; then
|
|
cat > $TMPC <<EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if cc_check -fno-PIC ; then
|
|
CFLAGS="-fno-PIC $CFLAGS"
|
|
echores "yes"
|
|
else
|
|
echores "no"
|
|
fi
|
|
else
|
|
echores "only used for x86"
|
|
fi
|
|
|
|
echocheck "compiler support for noexecstack"
|
|
cat > $TMPC <<EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if cc_check -Wl,-z,noexecstack ; then
|
|
_ld_extra="-Wl,-z,noexecstack $_ld_extra"
|
|
echores "yes"
|
|
else
|
|
echores "no"
|
|
fi
|
|
|
|
echocheck "ftello()"
|
|
# if we don't have ftello use the osdep/ compatibility module
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <sys/types.h>
|
|
int main (void) { ftello(stdin); return 0; }
|
|
EOF
|
|
_ftello=no
|
|
cc_check && _ftello=yes
|
|
if test "$_ftello" = yes ; then
|
|
_def_ftello='#define HAVE_FTELLO 1'
|
|
else
|
|
_def_ftello='#undef HAVE_FTELLO'
|
|
fi
|
|
echores "$_ftello"
|
|
|
|
# Determine OS dependent libs
|
|
if cygwin ; then
|
|
_def_confwin32='#define WIN32'
|
|
#CFLAGS="$CFLAGS -D__CYGWIN__ -D__CYGWIN_USE_BIG_TYPES__"
|
|
# stat.st_size with BIG_TYPES is broken (not set) ::atmos
|
|
CFLAGS="$CFLAGS -D__CYGWIN__"
|
|
fi
|
|
|
|
if win32 ; then
|
|
_confwin32='TARGET_WIN32 = yes'
|
|
else
|
|
_confwin32='TARGET_WIN32 = no'
|
|
fi
|
|
|
|
# Dynamic linking flags
|
|
# (FIXME: 'echocheck "dynamic linking"' above and modify here accordingly)
|
|
_ld_dl_dynamic=''
|
|
bsd && _ld_dl_dynamic='-rdynamic'
|
|
if test "$_real" = yes || test "$_xanim" = yes && not win32 && not qnx ; then
|
|
_ld_dl_dynamic='-rdynamic'
|
|
fi
|
|
|
|
_ld_arch="$_ld_arch $_ld_pthread $_ld_dl $_ld_dl_dynamic"
|
|
bsdos && _ld_arch="$_ld_arch -ldvd"
|
|
if netbsd ; then
|
|
x86 && _ld_arch="$_ld_arch -li386"
|
|
fi
|
|
|
|
_def_debug='#undef MP_DEBUG'
|
|
test "$_debug" != "" && _def_debug='#define MP_DEBUG 1'
|
|
|
|
_def_linux='#undef TARGET_LINUX'
|
|
linux && _def_linux='#define TARGET_LINUX 1'
|
|
|
|
# TODO cleanup the VIDIX stuff here
|
|
_def_vidix='#define CONFIG_VIDIX 1'
|
|
test "$_vidix" = no && _def_vidix='#undef CONFIG_VIDIX'
|
|
if test "$_vidix" = yes; then
|
|
_vosrc="$_vosrc vo_cvidix.c"
|
|
_vomodules="cvidix $_vomodules"
|
|
else
|
|
_novomodules="cvidix $_novomodules"
|
|
fi
|
|
if test "$_vidix" = yes && (win32); then
|
|
_vosrc="$_vosrc vo_winvidix.c"
|
|
_vomodules="winvidix $_vomodules"
|
|
_ld_win32libs="-lgdi32 $_ld_win32libs"
|
|
else
|
|
_novomodules="winvidix $_novomodules"
|
|
fi
|
|
if test "$_vidix" = yes && test "$_x11" = yes; then
|
|
_vosrc="$_vosrc vo_xvidix.c"
|
|
_vomodules="xvidix $_vomodules"
|
|
else
|
|
_novomodules="xvidix $_novomodules"
|
|
fi
|
|
echo Checking for VIDIX ... "$_vidix"
|
|
|
|
_def_joystick='#undef HAVE_JOYSTICK'
|
|
if test "$_joystick" = yes ; then
|
|
if linux ; then
|
|
# TODO add some check
|
|
_def_joystick='#define HAVE_JOYSTICK 1'
|
|
else
|
|
_joystick="no (unsupported under $system_name)"
|
|
fi
|
|
fi
|
|
echo Checking for joystick ... "$_joystick"
|
|
|
|
echocheck "lirc"
|
|
if test "$_lirc" = auto ; then
|
|
_lirc=no
|
|
if test -c /dev/lirc -o -c /dev/lirc/0 ; then
|
|
cat > $TMPC <<EOF
|
|
#include <lirc/lirc_client.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check -llirc_client && _lirc=yes
|
|
fi
|
|
fi
|
|
if test "$_lirc" = yes ; then
|
|
_def_lirc='#define HAVE_LIRC 1'
|
|
_ld_lirc='-llirc_client'
|
|
else
|
|
_def_lirc='#undef HAVE_LIRC'
|
|
fi
|
|
echores "$_lirc"
|
|
|
|
echocheck "lircc"
|
|
if test "$_lircc" = auto ; then
|
|
_lircc=no
|
|
cat > $TMPC <<EOF
|
|
#include <lirc/lircc.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
cc_check -llircc && _lircc=yes
|
|
fi
|
|
if test "$_lircc" = yes ; then
|
|
_def_lircc='#define HAVE_LIRCC 1'
|
|
_ld_lircc='-llircc'
|
|
else
|
|
_def_lircc='#undef HAVE_LIRCC'
|
|
fi
|
|
echores "$_lircc"
|
|
|
|
#############################################################################
|
|
echo "Creating config.mak"
|
|
cat > config.mak << EOF
|
|
# -------- Generated by configure -----------
|
|
|
|
LANG = C
|
|
MAN_LANG = $MAN_LANG
|
|
TARGET_OS = $system_name
|
|
DESTDIR =
|
|
prefix = \$(DESTDIR)$_prefix
|
|
BINDIR = \$(DESTDIR)$_bindir
|
|
DATADIR = \$(DESTDIR)$_datadir
|
|
MANDIR = \$(DESTDIR)$_mandir
|
|
CONFDIR = \$(DESTDIR)$_confdir
|
|
LIBDIR = \$(DESTDIR)$_libdir
|
|
# FFmpeg uses libdir instead of LIBDIR
|
|
libdir = \$(LIBDIR)
|
|
#AR = ar
|
|
CC = $_cc
|
|
HOST_CC = $_host_cc
|
|
AWK = $_awk
|
|
RANLIB = $_ranlib
|
|
INSTALL = $_install
|
|
# OPTFLAGS = -O4 $_profile $_debug $_march $_mcpu $_pipe -fomit-frame-pointer -ffast-math
|
|
EXTRA_INC = $_inc_extra $_inc_gtk
|
|
OPTFLAGS = -I../libvo -I../../libvo $_inc_x11 $CFLAGS \$(EXTRA_INC) $_opt_gnu99
|
|
STRIPBINARIES = $_stripbinaries
|
|
CHARSET = $_charset
|
|
HELP_FILE = $_mp_help
|
|
|
|
PRG = $_prg
|
|
PRG_MENCODER = $_prg_mencoder
|
|
|
|
$_live_libs_def
|
|
|
|
MPLAYER_NETWORK = $_network
|
|
STREAMING_LIVE_DOT_COM = $_live
|
|
MPLAYER_NETWORK_LIB = $_ld_live $_ld_vstream $_ld_network
|
|
DVBIN = $_dvbin
|
|
VIDIX = $_vidix
|
|
SHARED_PP = $_shared_pp
|
|
CONFIG_PP = yes
|
|
CONFIG_RISKY = yes
|
|
CONFIG_MP3LAME = $_mp3lame
|
|
LIBMENU = $_menu
|
|
I18NLIBS = $_i18n_libs
|
|
MATROSKA = $_matroska_internal
|
|
|
|
OPENDIVX = $_opendivx
|
|
|
|
MP3LIB = $_mp3lib
|
|
LIBA52 = $_liba52
|
|
LIBMPEG2 = $_libmpeg2
|
|
TREMOR = $_tremor_internal
|
|
TREMOR_FLAGS = $_tremor_flags
|
|
|
|
UNRARLIB = $_unrarlib
|
|
HAVE_FFPOSTPROCESS = $_def_haveffpostprocess
|
|
PNG = $_mkf_png
|
|
JPEG = $_mkf_jpg
|
|
GIF = $_mkf_gif
|
|
|
|
EXTRA_LIB = $_ld_extra
|
|
Z_LIB = $_ld_static $_ld_zlib
|
|
HAVE_MLIB = $_mlib
|
|
WIN32_LIB = $_ld_win32libs
|
|
STATIC_LIB = $_ld_static
|
|
ENCA_LIB = $_ld_enca
|
|
HAVE_PTHREADS = $_pthreads
|
|
MATH_LIB = $_ld_lm
|
|
|
|
X11_INC = $_inc_x11
|
|
X11DIR = $_ld_x11
|
|
|
|
HAVE_XVMC_ACCEL = $_xvmc
|
|
|
|
# for libavcodec:
|
|
SRC_PATH=..
|
|
LIBPREF=lib
|
|
LIBSUF=.a
|
|
SLIBPREF=lib
|
|
SLIBSUF=.so
|
|
|
|
# video output
|
|
X_LIB = $_ld_gl $_ld_dga $_ld_xv $_ld_xvmc $_ld_vm $_ld_xinerama $_ld_x11 $_ld_sock
|
|
GGI_LIB = $_ld_ggi
|
|
MLIB_LIB = $_ld_mlib
|
|
MLIB_INC = $_inc_mlib
|
|
DXR2_INC = $_inc_dxr2
|
|
DVB_INC = $_inc_dvb
|
|
PNG_LIB = $_ld_png
|
|
JPEG_LIB = $_ld_jpg
|
|
GIF_LIB = $_ld_gif
|
|
SDL_LIB = $_ld_sdl
|
|
SVGA_LIB = $_ld_svga
|
|
AA_LIB = $_ld_aa
|
|
CACA_INC = $_inc_caca
|
|
CACA_LIB = $_ld_caca
|
|
|
|
# audio output
|
|
ALSA_LIB = $_ld_alsa
|
|
NAS_LIB = $_ld_nas
|
|
ARTS_LIB = $_ld_arts
|
|
ARTS_INC = $_inc_arts
|
|
ESD_LIB = $_ld_esd
|
|
ESD_INC = $_inc_esd
|
|
POLYP_LIB = $_ld_polyp
|
|
POLYP_INC = $_inc_polyp
|
|
JACK_LIB = $_ld_jack
|
|
JACK_INC = $_inc_jack
|
|
SGIAUDIO_LIB = $_ld_sgiaudio
|
|
|
|
# input/demuxer/codecs
|
|
TERMCAP_LIB = $_ld_termcap
|
|
LIRC_LIB = $_ld_lirc
|
|
LIRCC_LIB = $_ld_lircc
|
|
DVDREAD_LIB = $_ld_dvdread
|
|
DVDKIT = $_dvdkit
|
|
DVDKIT2 = $_dvdkit2
|
|
DVDKIT_SHARED = no
|
|
SDL_INC = $_inc_sdl
|
|
W32_DEP = $_dep_win32
|
|
W32_LIB = $_ld_win32
|
|
DS_DEP = $_dep_dshow
|
|
DS_LIB = $_ld_dshow
|
|
AV_DEP = $_dep_libavcodec $_dep_libavformat
|
|
AV_LIB = $_ld_libavcodec $_ld_libavformat
|
|
CONFIG_LIBAVCODEC = $_libavcodec
|
|
CONFIG_LIBAVFORMAT = $_libavformat
|
|
ZORAN = $_zr
|
|
FAME = $_fame
|
|
FAME_LIB = $_ld_fame
|
|
MP1E_DEP = $_dep_mp1e
|
|
MP1E_LIB = $_ld_mp1e
|
|
ARCH_LIB = $_ld_arch $_ld_iconv
|
|
XVID = $_xvid
|
|
XVID_INC = $_inc_xvid
|
|
XVID_LIB = $_ld_xvid
|
|
X264 = $_x264
|
|
X264_INC = $_inc_x264
|
|
X264_LIB = $_ld_x264
|
|
CONFIG_DTS = $_libdts
|
|
DTS_INC = $_inc_libdts
|
|
DTS_LIB = $_ld_libdts
|
|
DECORE_LIB = $_ld_decore $_ld_mp3lame
|
|
MENCODER = $_mencoder
|
|
ENCORE_LIB = $_ld_encore $_ld_mp3lame
|
|
DIRECTFB_INC = $_inc_directfb
|
|
DIRECTFB_LIB = $_ld_directfb
|
|
CDPARANOIA_INC = $_inc_cdparanoia
|
|
CDPARANOIA_LIB = $_ld_cdparanoia
|
|
FREETYPE_INC = $_inc_freetype
|
|
FREETYPE_LIB = $_ld_freetype
|
|
FONTCONFIG_INC = $_inc_fontconfig
|
|
FONTCONFIG_LIB = $_ld_fontconfig
|
|
FRIBIDI_INC = $_inc_fribidi
|
|
FRIBIDI_LIB = $_ld_fribidi
|
|
LIBLZO_LIB= $_ld_liblzo
|
|
MAD_LIB = $_ld_mad
|
|
VORBIS_LIB = $_ld_vorbis $_ld_libdv
|
|
THEORA_LIB = $_ld_theora
|
|
FAAD_LIB = $_ld_faad
|
|
INTERNAL_FAAD = $_faad_internal
|
|
SMBSUPPORT_LIB = $_ld_smb
|
|
XMMS_PLUGINS = $_xmms
|
|
XMMS_LIB = $_xmms_lib
|
|
MACOSX = $_macosx
|
|
MACOSX_FINDER_SUPPORT = $_macosx_finder_support
|
|
MACOSX_BUNDLE = $_macosx_bundle
|
|
MACOSX_FRAMEWORKS = $_macosx_frameworks
|
|
MACOSX_COREVIDEO = $_macosx_corevideo
|
|
TOOLAME=$_toolame
|
|
TOOLAME_EXTRAFLAGS=$_toolame_extraflags
|
|
TOOLAME_LIB=$_toolame_lib
|
|
TWOLAME=$_twolame
|
|
TWOLAME_LIB=$_twolame_lib
|
|
FAAC=$_faac
|
|
FAAC_LIB=$_ld_faac
|
|
AMR_NB=$_amr_nb
|
|
AMR_NB_FIXED=$_amr_nb_fixed
|
|
AMR_WB=$_amr_wb
|
|
`echo $_libavcodecs | tr 'a-z ' 'A-Z\n' | sed 's/^/CONFIG_/;s/$/=yes/'`
|
|
|
|
# --- Some stuff for autoconfigure ----
|
|
$_target_arch
|
|
$_confwin32
|
|
TARGET_CPU=$iproc
|
|
TARGET_MMX = $_mmx
|
|
TARGET_MMX2 = $_mmx2
|
|
TARGET_3DNOW = $_3dnow
|
|
TARGET_3DNOWEX = $_3dnowex
|
|
TARGET_SSE = $_sse
|
|
TARGET_ALTIVEC = $_altivec
|
|
TARGET_VIS = $_vis
|
|
|
|
# --- GUI stuff ---
|
|
GTKLIB = $_ld_static $_ld_gtk
|
|
GLIBLIB = $_ld_static $_ld_glib
|
|
GTK_LIBS = $_ld_static $_ld_gui
|
|
GUI = $_gui
|
|
DEBUG = -DDEBUG
|
|
|
|
EOF
|
|
|
|
#############################################################################
|
|
echo "Creating config.h"
|
|
cat > config.h << EOF
|
|
/* -------- This file has been automatically generated by configure ---------
|
|
Note: Any changes in it will be lost when you run configure again. */
|
|
|
|
/* Protect against multiple inclusion */
|
|
#ifndef MPLAYER_CONFIG_H
|
|
#define MPLAYER_CONFIG_H 1
|
|
|
|
/* use GNU internationalization */
|
|
$_def_i18n
|
|
|
|
/* Runtime CPU detection */
|
|
$_def_runtime_cpudetection
|
|
|
|
/* Dynamic a/v plugins */
|
|
$_def_dynamic_plugins
|
|
|
|
/* "restrict" keyword */
|
|
$_def_restrict_keyword
|
|
|
|
/* __builtin_expect branch prediction hint */
|
|
$_def_builtin_expect
|
|
|
|
/* attribute(used) as needed by some compilers */
|
|
#if (__GNUC__ * 100 + __GNUC_MINOR__ >= 300)
|
|
# define attribute_used __attribute__((used))
|
|
#else
|
|
# define attribute_used
|
|
#endif
|
|
|
|
#define PREFIX "$_prefix"
|
|
|
|
#define USE_OSD 1
|
|
#define USE_SUB 1
|
|
|
|
/* enable/disable SIGHANDLER */
|
|
$_def_sighandler
|
|
|
|
/* enable/disable automatic gdb attach on crash, requires SIGHANDLER */
|
|
$_def_crash_debug
|
|
|
|
/* Toggles debugging informations */
|
|
$_def_debug
|
|
|
|
/* Indicates that Ogle's libdvdread is available for DVD playback */
|
|
$_def_dvdread
|
|
|
|
/* Indicates that dvdread is from libmpdvdkit */
|
|
$_def_mpdvdkit
|
|
|
|
/* Additional options for libmpdvdkit*/
|
|
$_def_dvd
|
|
$_def_cdrom
|
|
$_def_cdio
|
|
$_def_dvdio
|
|
$_def_bsdi_dvd
|
|
$_def_dvd_bsd
|
|
$_def_dvd_linux
|
|
$_dev_dvd_openbsd
|
|
$_def_dvd_darwin
|
|
$_def_sol_scsi_h
|
|
$_def_hpux_scsi_h
|
|
$_def_stddef
|
|
|
|
/* Common data directory (for fonts, etc) */
|
|
#define MPLAYER_DATADIR "$_datadir"
|
|
#define MPLAYER_CONFDIR "$_confdir"
|
|
#define MPLAYER_LIBDIR "$_libdir"
|
|
|
|
/* Define this to compile stream-caching support, it can be enabled via
|
|
-cache <kilobytes> */
|
|
#define USE_STREAM_CACHE 1
|
|
|
|
/* Define to include support for XviD/Divx4Linux/OpenDivx */
|
|
$_def_divx
|
|
|
|
/* Define to use the new XviD/DivX4Linux library instead of open source OpenDivX */
|
|
/* You have to change DECORE_LIBS in config.mak, too! */
|
|
$_def_decore
|
|
|
|
/* Define if you are using DivX5Linux Decore library */
|
|
$_def_divx5
|
|
|
|
/* Define if you are using XviD library */
|
|
$_def_xvid3
|
|
$_def_xvid4
|
|
$_def_decore_xvid
|
|
$_def_encore_xvid
|
|
|
|
/* Define if you are using the X.264 library */
|
|
$_def_x264
|
|
|
|
/* Define to include support for libdv-0.9.5 */
|
|
$_def_libdv
|
|
|
|
/* If build mencoder */
|
|
$_mencoder_flag
|
|
|
|
/* Indicates if XviD/Divx4linux encore is available
|
|
Note: for mencoder */
|
|
$_def_encore
|
|
|
|
/* Indicates if libmp3lame is available
|
|
Note: for mencoder */
|
|
$_def_mp3lame
|
|
$_def_cfg_mp3lame
|
|
|
|
/* Define libmp1e for realtime mpeg encoding (for DXR3 and DVB cards) */
|
|
$_def_mp1e
|
|
|
|
/* Define this to enable avg. byte/sec-based AVI sync method by default:
|
|
(use -bps or -nobps commandline option for run-time method selection)
|
|
-bps gives better sync for vbr mp3 audio, it is now default */
|
|
#define AVI_SYNC_BPS 1
|
|
|
|
/* Undefine this if you do not want to select mono audio (left or right)
|
|
with a stereo MPEG layer 2/3 audio stream. The command line option
|
|
-stereo has three possible values (0 for stereo, 1 for left-only, 2 for
|
|
right-only), with 0 being the default.
|
|
*/
|
|
#define USE_FAKE_MONO 1
|
|
|
|
/* Undefine this if your sound card driver has no working select().
|
|
If you have kernel Oops, player hangups, or just no audio, you should
|
|
try to recompile MPlayer with this option disabled! */
|
|
$_def_select
|
|
|
|
/* define this to use iconv(3) function to codepage conversions */
|
|
$_def_iconv
|
|
|
|
/* define this to use nl_langinfo function */
|
|
$_def_langinfo
|
|
|
|
/* define this to use RTC (/dev/rtc) for video timers */
|
|
$_def_rtc
|
|
|
|
/* set up max. outburst. use 65536 for ALSA 0.5, for others 16384 is enough */
|
|
#define MAX_OUTBURST 65536
|
|
|
|
/* set up audio OUTBURST. Do not change this! */
|
|
#define OUTBURST 512
|
|
|
|
/* Define this if your system has the header file for the OSS sound interface */
|
|
$_def_sys_soundcard
|
|
|
|
/* Define this if your system has the header file for the OSS sound interface
|
|
* in /usr/include */
|
|
$_def_soundcard
|
|
|
|
/* Define this if your system has the sysinfo header */
|
|
$_def_sys_sysinfo
|
|
|
|
/* Define this if your system has ftello() */
|
|
|
|
$_def_ftello
|
|
#ifndef HAVE_FTELLO
|
|
/* Need these for FILE and off_t an config.h is usually before other includes*/
|
|
#include <stdio.h>
|
|
#include <sys/types.h>
|
|
off_t ftello(FILE *);
|
|
#endif
|
|
|
|
/* Define this if your system has the "malloc.h" header file */
|
|
$_def_malloc
|
|
|
|
/* memalign is mapped to malloc if unsupported */
|
|
$_def_memalign
|
|
#ifndef HAVE_MEMALIGN
|
|
# define memalign(a,b) malloc(b)
|
|
#define MEMALIGN_HACK 1
|
|
#endif
|
|
|
|
/* Define this if your system has the "alloca.h" header file */
|
|
$_def_alloca
|
|
|
|
/* Define this if your system has the "sys/mman.h" header file */
|
|
$_def_mman
|
|
$_def_mman_has_map_failed
|
|
|
|
/* Define this if you have the elf dynamic linker -ldl library */
|
|
$_def_dl
|
|
|
|
/* Define this if you have the kstat kernel statistics library */
|
|
$_def_kstat
|
|
|
|
/* Define this if you have zlib */
|
|
$_def_zlib
|
|
#ifdef HAVE_ZLIB
|
|
#define CONFIG_ZLIB 1
|
|
#endif
|
|
|
|
/* Define this if you have shm support */
|
|
$_def_shm
|
|
|
|
/* Define this if your system has scandir & alphasort */
|
|
$_def_scandir
|
|
|
|
/* Define this if your system has strsep */
|
|
$_def_strsep
|
|
|
|
/* Define this if your system has strlcpy */
|
|
$_def_strlcpy
|
|
#ifndef HAVE_STRLCPY
|
|
unsigned int strlcpy (char *dest, const char *src, unsigned int size);
|
|
#endif
|
|
|
|
/* Define this if your system has strlcat */
|
|
$_def_strlcat
|
|
#ifndef HAVE_STRLCAT
|
|
unsigned int strlcat (char *dest, const char *src, unsigned int size);
|
|
#endif
|
|
|
|
/* Define this if your system has fseeko */
|
|
$_def_fseeko
|
|
#ifndef HAVE_FSEEKO
|
|
/* Need these for FILE and off_t an config.h is usually before other includes*/
|
|
#include <stdio.h>
|
|
#include <sys/types.h>
|
|
int fseeko(FILE *, off_t, int);
|
|
#endif
|
|
|
|
$_def_localtime_r
|
|
|
|
/* Define this if your system has vsscanf */
|
|
$_def_vsscanf
|
|
|
|
/* Define this if your system has swab */
|
|
$_def_swab
|
|
|
|
/* Define this if your system has no posix select */
|
|
$_def_no_posix_select
|
|
|
|
/* Define this if your system has gettimeofday */
|
|
$_def_gettimeofday
|
|
|
|
/* Define this if your system has glob */
|
|
$_def_glob
|
|
|
|
/* Define this if your system has pthreads */
|
|
$_def_pthreads
|
|
|
|
/* Define this if you enabled thread support for libavcodec */
|
|
$_def_threads
|
|
|
|
/* LIRC (remote control, see www.lirc.org) support: */
|
|
$_def_lirc
|
|
|
|
/*
|
|
* LIRCCD (LIRC client daemon)
|
|
* See http://www.dolda2000.cjb.net/~fredrik/lirccd/
|
|
*/
|
|
$_def_lircc
|
|
|
|
/* DVD navigation support using libdvdnav */
|
|
$_def_dvdnav
|
|
$_def_dvdnav_version
|
|
|
|
/* Define this to enable MPEG 1/2 image postprocessing (requires a FAST CPU!) */
|
|
#define MPEG12_POSTPROC 1
|
|
|
|
/* Define this to enable image postprocessing in libavcodec (requires a FAST CPU!) */
|
|
$_def_ffpostprocess
|
|
|
|
/* Define to include support for OpenDivx postprocessing */
|
|
$_def_odivx_postprocess
|
|
|
|
/* Win32 DLL support */
|
|
$_def_win32
|
|
#define WIN32_PATH "$_win32libdir"
|
|
|
|
/* DirectShow support */
|
|
$_def_dshow
|
|
|
|
/* Mac OS X specific features */
|
|
$_def_macosx
|
|
$_def_macosx_finder_support
|
|
$_def_macosx_bundle
|
|
$_def_macosx_corevideo
|
|
|
|
/* Build our Win32-loader */
|
|
$_def_win32_loader
|
|
|
|
/* ffmpeg's libavcodec support (requires libavcodec source) */
|
|
$_def_libavcodec
|
|
$_def_libavcodecso
|
|
|
|
/* ffmpeg's libavformat support (requires libavformat source) */
|
|
$_def_libavformat
|
|
$_def_libavformat_win32
|
|
|
|
/* risky codecs */
|
|
#define CONFIG_RISKY 1
|
|
|
|
/* Use libavcodec's decoders */
|
|
#define CONFIG_DECODERS 1
|
|
/* Use libavcodec's encoders */
|
|
#define CONFIG_ENCODERS 1
|
|
|
|
#define CONFIG_MPEGAUDIO_HP 1
|
|
|
|
/* Use amr codecs from libavcodec (requires amr sources) */
|
|
$_def_amr_nb
|
|
$_def_amr_nb_fixed
|
|
$_def_amr_wb
|
|
|
|
/* Use specific codecs from libavcodec */
|
|
`echo $_libavcodecs | tr 'a-z ' 'A-Z\n' | sed 's/^/#define CONFIG_/;s/$/ 1/'`
|
|
|
|
/* Use codec libs included in mplayer CVS / source dist: */
|
|
$_def_mp3lib
|
|
$_def_liba52
|
|
$_def_libdts
|
|
$_def_libmpeg2
|
|
|
|
/* Use libfame encoder filter */
|
|
$_def_fame
|
|
|
|
/* XAnim DLL support */
|
|
$_def_xanim
|
|
/* Default search path */
|
|
$_def_xanim_path
|
|
|
|
/* RealPlayer DLL support */
|
|
$_def_real
|
|
/* Default search path */
|
|
$_def_real_path
|
|
|
|
/* LIVE.COM Streaming Media library support */
|
|
$_def_live
|
|
|
|
/* Use 3dnow/mmxext/sse/mmx optimized fast memcpy() [maybe buggy... signal 4]*/
|
|
$_def_fastmemcpy
|
|
|
|
/* Use unrarlib for Vobsubs */
|
|
$_def_unrarlib
|
|
|
|
/* gui support, please do not edit this option */
|
|
$_def_gui
|
|
|
|
/* Audio output drivers */
|
|
$_def_ossaudio
|
|
$_def_ossaudio_devdsp
|
|
$_def_ossaudio_devmixer
|
|
$_def_alsa5
|
|
$_def_alsa9
|
|
$_def_alsa1x
|
|
$_def_arts
|
|
$_def_esd
|
|
$_def_esd_latency
|
|
$_def_polyp
|
|
$_def_jack
|
|
$_def_sys_asoundlib_h
|
|
$_def_alsa_asoundlib_h
|
|
$_def_sunaudio
|
|
$_def_sgiaudio
|
|
$_def_win32waveout
|
|
$_def_nas
|
|
|
|
/* Enable fast OSD/SUB renderer (looks ugly, but uses less CPU power) */
|
|
#undef FAST_OSD
|
|
#undef FAST_OSD_TABLE
|
|
|
|
/* Enable TV Interface support */
|
|
$_def_tv
|
|
|
|
/* Enable EDL support */
|
|
$_def_edl
|
|
|
|
/* Enable Video 4 Linux TV interface support */
|
|
$_def_tv_v4l
|
|
|
|
/* Enable Video 4 Linux 2 TV interface support */
|
|
$_def_tv_v4l2
|
|
|
|
/* Enable *BSD BrookTree TV interface support */
|
|
$_def_tv_bsdbt848
|
|
|
|
/* Define if your processor stores words with the most significant
|
|
byte first (like Motorola and SPARC, unlike Intel and VAX). */
|
|
$_def_words_endian
|
|
|
|
$_def_arch
|
|
|
|
/* For the PPC. G5 has the dcbzl when in 64bit mode but G4s and earlier do not
|
|
have the instruction. */
|
|
$_def_dcbzl
|
|
|
|
/* libmpeg2 wants ARCH_PPC and the rest of mplayer use ARCH_POWERPC,
|
|
* define ARCH_PPC if ARCH_POWERPC is set to cope with that.
|
|
*/
|
|
#ifdef ARCH_POWERPC
|
|
#define ARCH_PPC 1
|
|
#endif
|
|
|
|
/* the same issue as with ARCH_POWERPC but with ffmpeg/libavcodec */
|
|
#ifdef ARCH_ARMV4L
|
|
#define ARCH_ARM 1
|
|
#endif
|
|
|
|
/* only gcc3 can compile mvi instructions */
|
|
$_def_gcc_mvi_support
|
|
|
|
/* Define this for Cygwin build for win32 */
|
|
$_def_confwin32
|
|
|
|
/* Define this to any prefered value from 386 up to infinity with step 100 */
|
|
#define __CPU__ $iproc
|
|
|
|
$_mp_wordsize
|
|
|
|
$_def_linux
|
|
|
|
$_def_vcd
|
|
|
|
#ifdef sun
|
|
#define DEFAULT_CDROM_DEVICE "/vol/dev/aliases/cdrom0"
|
|
#define DEFAULT_DVD_DEVICE DEFAULT_CDROM_DEVICE
|
|
#elif defined(HPUX)
|
|
#define DEFAULT_CDROM_DEVICE "/dev/cdrom"
|
|
#define DEFAULT_DVD_DEVICE "/dev/dvd"
|
|
#elif defined(WIN32)
|
|
#define DEFAULT_CDROM_DEVICE "D:"
|
|
#define DEFAULT_DVD_DEVICE "D:"
|
|
#elif defined(SYS_DARWIN)
|
|
#define DEFAULT_CDROM_DEVICE "/dev/disk1"
|
|
#define DEFAULT_DVD_DEVICE "/dev/rdiskN"
|
|
#elif defined(__OpenBSD__)
|
|
#define DEFAULT_CDROM_DEVICE "/dev/rcd0a"
|
|
#define DEFAULT_DVD_DEVICE DEFAULT_CDROM_DEVICE
|
|
#else
|
|
#define DEFAULT_CDROM_DEVICE "/dev/cdrom"
|
|
#define DEFAULT_DVD_DEVICE "/dev/dvd"
|
|
#endif
|
|
|
|
|
|
/*----------------------------------------------------------------------------
|
|
**
|
|
** NOTE: Instead of modifying these definitions here, use the
|
|
** --enable/--disable options of the ./configure script!
|
|
** See ./configure --help for details.
|
|
**
|
|
*---------------------------------------------------------------------------*/
|
|
|
|
/* C99 lrintf function available */
|
|
$_def_lrintf
|
|
|
|
/* round function is available */
|
|
$_def_round
|
|
|
|
/* yes, we have inttypes.h */
|
|
#define HAVE_INTTYPES_H 1
|
|
|
|
/* int_fastXY_t emulation */
|
|
$_def_fast_inttypes
|
|
|
|
/* nanosleep support */
|
|
$_def_nanosleep
|
|
|
|
/* SMB support */
|
|
$_def_smbsupport
|
|
|
|
/* termcap flag for getch2.c */
|
|
$_def_termcap
|
|
|
|
/* termios flag for getch2.c */
|
|
$_def_termios
|
|
$_def_termios_h
|
|
$_def_termios_sys_h
|
|
|
|
/* enable PNG support */
|
|
$_def_png
|
|
|
|
/* enable JPEG support */
|
|
$_def_jpg
|
|
|
|
/* enable PNM support */
|
|
$_def_pnm
|
|
|
|
/* enable md5sum support */
|
|
$_def_md5sum
|
|
|
|
/* enable GIF support */
|
|
$_def_gif
|
|
$_def_gif_4
|
|
$_def_gif_tvt_hack
|
|
|
|
/* enable FreeType support */
|
|
$_def_freetype
|
|
|
|
/* enable Fontconfig support */
|
|
$_def_fontconfig
|
|
|
|
/* enable FriBiDi usage */
|
|
$_def_fribidi
|
|
|
|
/* enable ENCA usage */
|
|
$_def_enca
|
|
|
|
/* liblzo support */
|
|
$_def_liblzo
|
|
|
|
/* libmad support */
|
|
$_def_mad
|
|
|
|
/* enable OggVorbis support */
|
|
$_def_vorbis
|
|
|
|
/* enable Tremor as vorbis decoder */
|
|
$_def_tremor
|
|
|
|
/* enable OggTheora support */
|
|
$_def_theora
|
|
|
|
/* enable Matroska support */
|
|
$_def_matroska
|
|
|
|
/* enable FAAD (AAC) support */
|
|
$_def_faad
|
|
$_def_faad_internal
|
|
|
|
/* enable FAAC (AAC encoder) support */
|
|
$_def_faac
|
|
|
|
/* enable LADSPA plugin support */
|
|
$_def_ladspa
|
|
|
|
/* enable network */
|
|
$_def_network
|
|
|
|
/* enable ftp support */
|
|
$_def_ftp
|
|
|
|
/* enable vstream support */
|
|
$_def_vstream
|
|
|
|
/* enable winsock2 instead of Unix functions*/
|
|
$_def_winsock2
|
|
|
|
/* define this to use inet_aton() instead of inet_pton() */
|
|
$_def_use_aton
|
|
|
|
/* enables / disables cdparanoia support */
|
|
$_def_cdparanoia
|
|
|
|
/* enables / disables VIDIX usage */
|
|
$_def_vidix
|
|
|
|
/* enables / disables new input joystick support */
|
|
$_def_joystick
|
|
|
|
/* enables / disables QTX codecs */
|
|
$_def_qtx
|
|
|
|
/* enables / disables osd menu */
|
|
$_def_menu
|
|
|
|
/* enables / disables subtitles sorting */
|
|
$_def_sortsub
|
|
|
|
/* XMMS input plugin support */
|
|
$_def_xmms
|
|
#define XMMS_INPUT_PLUGIN_DIR "$_xmmsplugindir"
|
|
|
|
/* enables inet6 support */
|
|
$_def_inet6
|
|
|
|
/* do we have gethostbyname2? */
|
|
$_def_gethostbyname2
|
|
|
|
/* Extension defines */
|
|
$_def_3dnow // only define if you have 3DNOW (AMD k6-2, AMD Athlon, iDT WinChip, etc.)
|
|
$_def_3dnowex // only define if you have 3DNOWEX (AMD Athlon, etc.)
|
|
$_def_mmx // only define if you have MMX (newer x86 chips, not P54C/PPro)
|
|
$_def_mmx2 // only define if you have MMX2 (Athlon/PIII/4/CelII)
|
|
$_def_sse // only define if you have SSE (Intel Pentium III/4 or Celeron II)
|
|
$_def_sse2 // only define if you have SSE2 (Intel Pentium 4)
|
|
$_def_altivec // only define if you have Altivec (G4)
|
|
|
|
$_def_altivec_h // enables usage of altivec.h
|
|
|
|
|
|
$_def_mlib // Sun mediaLib, available only on solaris
|
|
$_def_vis // only define if you have VIS ( ultrasparc )
|
|
|
|
/* libmpeg2 uses a different feature test macro for mediaLib */
|
|
#ifdef HAVE_MLIB
|
|
#define LIBMPEG2_MLIB 1
|
|
#endif
|
|
|
|
/* libvo options */
|
|
#define SCREEN_SIZE_X 1
|
|
#define SCREEN_SIZE_Y 1
|
|
$_def_x11
|
|
$_def_xv
|
|
$_def_xvmc
|
|
$_def_vm
|
|
$_def_xf86keysym
|
|
$_def_xinerama
|
|
$_def_gl
|
|
$_def_gl_win32
|
|
$_def_dga
|
|
$_def_dga2
|
|
$_def_sdl
|
|
/* defined for SDLlib with keyrepeat bugs (before 1.2.1) */
|
|
$_def_sdlbuggy
|
|
$_def_directx
|
|
$_def_ggi
|
|
$_def_ggiwmh
|
|
$_def_3dfx
|
|
$_def_tdfxfb
|
|
$_def_tdfxvid
|
|
$_def_directfb
|
|
$_def_directfb_version
|
|
$_def_zr
|
|
$_def_bl
|
|
$_def_mga
|
|
$_def_xmga
|
|
$_def_syncfb
|
|
$_def_fbdev
|
|
$_def_dxr2
|
|
$_def_dxr3
|
|
$_def_dvb
|
|
$_def_dvb_in
|
|
$_def_svga
|
|
$_def_vesa
|
|
$_def_xdpms
|
|
$_def_aa
|
|
$_def_caca
|
|
$_def_tga
|
|
$_def_toolame
|
|
$_def_twolame
|
|
|
|
/* used by GUI: */
|
|
$_def_xshape
|
|
|
|
#if defined(HAVE_GL) || defined(HAVE_X11) || defined(HAVE_XV)
|
|
#define X11_FULLSCREEN 1
|
|
#endif
|
|
|
|
#endif /* MPLAYER_CONFIG_H */
|
|
EOF
|
|
|
|
#############################################################################
|
|
|
|
echo "Creating libvo/config.mak"
|
|
_voobj=`echo $_vosrc | sed -e 's/\.c/\.o/g;s/\.m/\.o/g'`
|
|
cat > libvo/config.mak << EOF
|
|
include ../config.mak
|
|
OPTIONAL_SRCS = $_vosrc
|
|
OPTIONAL_OBJS = $_voobj
|
|
EOF
|
|
|
|
#############################################################################
|
|
|
|
echo "Creating libao2/config.mak"
|
|
_aoobj=`echo $_aosrc | sed -e 's/\.c/\.o/g'`
|
|
cat > libao2/config.mak << EOF
|
|
include ../config.mak
|
|
OPTIONAL_SRCS = $_aosrc
|
|
OPTIONAL_OBJS = $_aoobj
|
|
EOF
|
|
|
|
#############################################################################
|
|
|
|
echo "Creating libaf/config.mak"
|
|
_afobj=`echo $_afsrc | sed -e 's/\.c/\.o/g'`
|
|
cat > libaf/config.mak << EOF
|
|
include ../config.mak
|
|
OPTIONAL_SRCS = $_afsrc
|
|
OPTIONAL_OBJS = $_afobj
|
|
EOF
|
|
|
|
#############################################################################
|
|
|
|
cat << EOF
|
|
|
|
Config files successfully generated by ./configure !
|
|
|
|
Install prefix: $_prefix
|
|
Data directory: $_datadir
|
|
Config direct.: $_confdir
|
|
|
|
Byte order: $_byte_order
|
|
Optimizing for: $_optimizing
|
|
|
|
Languages:
|
|
Messages/GUI: $_language
|
|
EOF
|
|
|
|
echo -n " Manual pages: $MAN_LANG"
|
|
test "$LANGUAGES" = en && echo -n " (no localization selected, use --language=all)"
|
|
echo
|
|
|
|
cat << EOF
|
|
|
|
Enabled optional drivers:
|
|
Input: $_inputmodules
|
|
Codecs: $_codecmodules
|
|
Audio output: $_aomodules
|
|
Video output: $_vomodules
|
|
Audio filters: $_afmodules
|
|
Disabled optional drivers:
|
|
Input: $_noinputmodules
|
|
Codecs: $_nocodecmodules
|
|
Audio output: $_noaomodules
|
|
Video output: $_novomodules
|
|
Audio filters: $_noafmodules
|
|
|
|
'config.h' and 'config.mak' contain your configuration options.
|
|
Note: If you alter theses files (for instance CFLAGS) MPlayer may no longer
|
|
compile *** DO NOT REPORT BUGS if you tweak these files ***
|
|
|
|
'make' will now compile MPlayer and 'make install' will install it.
|
|
Note: On non-Linux systems you might need to use 'gmake' instead of 'make'.
|
|
|
|
EOF
|
|
|
|
|
|
if test "$_mtrr" = yes ; then
|
|
echo "Please check mtrr settings at /proc/mtrr (see DOCS/HTML/$_doc_lang/video.html#mtrr)"
|
|
echo
|
|
fi
|
|
|
|
if test "$_sdl" = "outdated" ; then
|
|
cat <<EOF
|
|
You have an outdated version of libSDL installed (older than v1.1.7) and SDL
|
|
support has therefore been disabled.
|
|
|
|
Please upgrade to a more recent version (version 1.1.8 and above are known to
|
|
work). You may get this library from: http://www.libsdl.org
|
|
|
|
You need to rerun ./configure and recompile after updating SDL. If you are
|
|
only interested in the libSDL audio drivers, then an older version might work.
|
|
|
|
Use --enable-sdl to force usage of libSDL.
|
|
|
|
EOF
|
|
fi
|
|
|
|
if x86; then
|
|
if test "$_win32" = no ; then
|
|
if test "$_win32libdir" ; then
|
|
echo "Failed to find a Win32 codecs dir at $_win32libdir!"
|
|
else
|
|
echo "Failed to find a Win32 codecs directory! (default: /usr/local/lib/codecs/)"
|
|
fi
|
|
cat << EOF
|
|
Create it and copy the DLL files there! You can download the codecs from our
|
|
homepage at http://www.mplayerhq.hu/MPlayer/releases/codecs/
|
|
|
|
EOF
|
|
fi
|
|
else
|
|
cat <<EOF
|
|
NOTE: Win32 codec DLLs are not supported on your CPU ($host_arch) or your
|
|
operating system ($system_name). You may encounter a few files that cannot
|
|
be played due to missing open source video/audio codec support.
|
|
|
|
EOF
|
|
fi
|
|
|
|
|
|
cat <<EOF
|
|
|
|
Check $TMPLOG if you wonder why an autodetection failed (check whether
|
|
the development headers/packages are installed).
|
|
|
|
If you suspect a bug, please read DOCS/HTML/$_doc_lang/bugreports.html.
|
|
|
|
EOF
|
|
|
|
if test "$_vidix" = no ; then
|
|
cat <<EOF
|
|
You've disabled VIDIX. Although it would be better to PORT it instead.
|
|
Have a look at the documentation for supported cards!
|
|
|
|
EOF
|
|
fi
|
|
|
|
# Last move:
|
|
rm -f "$TMPO" "$TMPC" "$TMPS" "$TMPCPP"
|