mirror of
https://github.com/mpv-player/mpv
synced 2024-07-31 16:29:58 +02:00
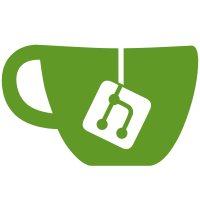
sd_ass contains some code that treats subtitle events with duration 0
specially, and adjust their duration so that they will disappear with
the next event.
This is most likely not needed anymore. Some subtitle formats allow
omitting the duration so that the event is visible until the next one,
but both subreader.c as well as libavformat subtitle demuxers already
handle this.
Subtitles embedded in mp4 files (movtext) used to trigger this code. But
these files appear to export subtitle duration correctly (at least
libavcodec's movtext decoder is using this assumption). Since commit
6dbedd2
changed demux_lavf to actually copy the packet duration field,
the code removed with this commit isn't needed anymore for correct
display of movtext subtitles. (The change in sd_movtext is for dropping
empty subtitle events, which would now be "displayed" - libavcodec does
the same.)
On the other hand, this code incorrectly displayed hidden events in .srt
subtitles. See for example the first event in SubRip_capability_tester.srt
(part of FFmpeg's FATE). These intentionally have a duration of 0, and
should not be displayed. (As of with this commit, they are still
displayed in external .srt subs because of subreader.c hacks.)
However, we can't be 100% sure that this code is really unneeded, so
just comment the code. Hopefully it can be removed if there are no
regressions after some weeks or months.
57 lines
1.5 KiB
C
57 lines
1.5 KiB
C
/*
|
|
* This file is part of mpv.
|
|
*
|
|
* mpv is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* mpv is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with mpv. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <stdlib.h>
|
|
#include <assert.h>
|
|
|
|
#include <libavutil/intreadwrite.h>
|
|
#include <libavutil/common.h>
|
|
|
|
#include "sd.h"
|
|
|
|
static bool supports_format(const char *format)
|
|
{
|
|
return format && strcmp(format, "mov_text") == 0;
|
|
}
|
|
|
|
static int init(struct sd *sd)
|
|
{
|
|
sd->output_codec = "text";
|
|
return 0;
|
|
}
|
|
|
|
static void decode(struct sd *sd, struct demux_packet *packet)
|
|
{
|
|
unsigned char *data = packet->buffer;
|
|
int len = packet->len;
|
|
if (len < 2)
|
|
return;
|
|
len = FFMIN(len - 2, AV_RB16(data));
|
|
data += 2;
|
|
if (len > 0)
|
|
sd_conv_add_packet(sd, data, len, packet->pts, packet->duration);
|
|
}
|
|
|
|
const struct sd_functions sd_movtext = {
|
|
.name = "movtext",
|
|
.supports_format = supports_format,
|
|
.init = init,
|
|
.decode = decode,
|
|
.get_converted = sd_conv_def_get_converted,
|
|
.reset = sd_conv_def_reset,
|
|
};
|