mirror of
https://github.com/mpv-player/mpv
synced 2024-07-31 16:29:58 +02:00
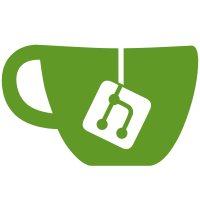
The plan is to make the whole OSD thread-safe, and we start with this. We just put locks on all entry points (fortunately, dec_sub.c and all sd_*.c decoders are very closed off, and only the entry points in dec_sub.h let you access it). I think this is pretty ugly, but at least it's very simple. There's a special case with sub_get_bitmaps(): this function returns pointers to decoder data (specifically, libass images). There's no way to synchronize this internally, so expose sub_lock/sub_unlock functions. To make things simpler, and especially because the lock is sort-of exposed to the outside world, make the locks recursive. Although the only case where this is actually needed (although trivial) is sub_set_extradata(). One corner case are ASS subtitles: for some reason, we keep a single ASS_Renderer instance for subtitles around (probably to avoid rescanning fonts with ordered chapters), and this ASS_Renderer instance is not synchronized. Also, demux_libass.c loads ASS_Track objects, which are directly passed to sd_ass.c. These things are not synchronized (and would be hard to synchronize), and basically we're out of luck. But I think for now, accesses happen reasonably serialized, so there is no actual problem yet, even if we start to access OSD from other threads.
51 lines
1.5 KiB
C
51 lines
1.5 KiB
C
#ifndef MPLAYER_DEC_SUB_H
|
|
#define MPLAYER_DEC_SUB_H
|
|
|
|
#include <stdbool.h>
|
|
#include <stdint.h>
|
|
|
|
#include "osd.h"
|
|
|
|
struct sh_stream;
|
|
struct ass_track;
|
|
struct mpv_global;
|
|
struct demux_packet;
|
|
struct ass_library;
|
|
struct ass_renderer;
|
|
|
|
struct dec_sub;
|
|
struct sd;
|
|
|
|
enum sd_ctrl {
|
|
SD_CTRL_SUB_STEP,
|
|
SD_CTRL_SET_VIDEO_PARAMS,
|
|
SD_CTRL_GET_RESOLUTION,
|
|
};
|
|
|
|
struct dec_sub *sub_create(struct mpv_global *global);
|
|
void sub_destroy(struct dec_sub *sub);
|
|
void sub_lock(struct dec_sub *sub);
|
|
void sub_unlock(struct dec_sub *sub);
|
|
|
|
void sub_set_video_res(struct dec_sub *sub, int w, int h);
|
|
void sub_set_video_fps(struct dec_sub *sub, double fps);
|
|
void sub_set_extradata(struct dec_sub *sub, void *data, int data_len);
|
|
void sub_set_ass_renderer(struct dec_sub *sub, struct ass_library *ass_library,
|
|
struct ass_renderer *ass_renderer);
|
|
void sub_init_from_sh(struct dec_sub *sub, struct sh_stream *sh);
|
|
|
|
bool sub_is_initialized(struct dec_sub *sub);
|
|
|
|
bool sub_read_all_packets(struct dec_sub *sub, struct sh_stream *sh);
|
|
bool sub_accept_packets_in_advance(struct dec_sub *sub);
|
|
void sub_decode(struct dec_sub *sub, struct demux_packet *packet);
|
|
void sub_get_bitmaps(struct dec_sub *sub, struct mp_osd_res dim, double pts,
|
|
struct sub_bitmaps *res);
|
|
bool sub_has_get_text(struct dec_sub *sub);
|
|
char *sub_get_text(struct dec_sub *sub, double pts);
|
|
void sub_reset(struct dec_sub *sub);
|
|
|
|
int sub_control(struct dec_sub *sub, enum sd_ctrl cmd, void *arg);
|
|
|
|
#endif
|