mirror of
https://github.com/mpv-player/mpv
synced 2024-10-30 04:46:41 +01:00
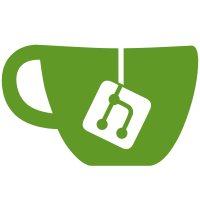
patch replaces '()' for the correct '(void)' in function declarations/prototypes which have no parameters. The '()' syntax tell thats there is a variable list of arguments, so that the compiler cannot check this. The extra CFLAG '-Wstrict-declarations' shows those cases. Comments about a similar patch applied to ffmpeg: That in C++ these mean the same, but in ANSI C the semantics are different; function() is an (obsolete) K&R C style forward declaration, it basically means that the function can have any number and any types of parameters, effectively completely preventing the compiler from doing any sort of type checking. -- Erik Slagter Defining functions with unspecified arguments is allowed but bad. With arguments unspecified the compiler can't report an error/warning if the function is called with incorrect arguments. -- Måns Rullgård git-svn-id: svn://svn.mplayerhq.hu/mplayer/trunk@17567 b3059339-0415-0410-9bf9-f77b7e298cf2
102 lines
2.0 KiB
C
102 lines
2.0 KiB
C
#ifndef __MPLAYER_FONT_LOAD_H
|
|
#define __MPLAYER_FONT_LOAD_H
|
|
|
|
#ifdef HAVE_FREETYPE
|
|
#include <ft2build.h>
|
|
#include FT_FREETYPE_H
|
|
#endif
|
|
|
|
typedef struct {
|
|
unsigned char *bmp;
|
|
unsigned char *pal;
|
|
int w,h,c;
|
|
#ifdef HAVE_FREETYPE
|
|
int charwidth,charheight,pen,baseline,padding;
|
|
int current_count, current_alloc;
|
|
#endif
|
|
} raw_file;
|
|
|
|
typedef struct {
|
|
#ifdef HAVE_FREETYPE
|
|
int dynamic;
|
|
#endif
|
|
char *name;
|
|
char *fpath;
|
|
int spacewidth;
|
|
int charspace;
|
|
int height;
|
|
// char *fname_a;
|
|
// char *fname_b;
|
|
raw_file* pic_a[16];
|
|
raw_file* pic_b[16];
|
|
short font[65536];
|
|
int start[65536]; // short is not enough for unicode fonts
|
|
short width[65536];
|
|
int freetype;
|
|
|
|
#ifdef HAVE_FREETYPE
|
|
int face_cnt;
|
|
|
|
FT_Face faces[16];
|
|
FT_UInt glyph_index[65536];
|
|
|
|
int max_width, max_height;
|
|
|
|
struct
|
|
{
|
|
int g_r;
|
|
int o_r;
|
|
int g_w;
|
|
int o_w;
|
|
int o_size;
|
|
unsigned volume;
|
|
|
|
unsigned *g;
|
|
unsigned *gt2;
|
|
unsigned *om;
|
|
unsigned char *omt;
|
|
unsigned short *tmp;
|
|
} tables;
|
|
#endif
|
|
|
|
} font_desc_t;
|
|
|
|
extern font_desc_t* vo_font;
|
|
|
|
#ifdef HAVE_FREETYPE
|
|
|
|
extern char *subtitle_font_encoding;
|
|
extern float text_font_scale_factor;
|
|
extern float osd_font_scale_factor;
|
|
extern float subtitle_font_radius;
|
|
extern float subtitle_font_thickness;
|
|
extern int subtitle_autoscale;
|
|
|
|
extern int vo_image_width;
|
|
extern int vo_image_height;
|
|
|
|
extern int force_load_font;
|
|
|
|
int init_freetype(void);
|
|
int done_freetype(void);
|
|
|
|
font_desc_t* read_font_desc_ft(char* fname,int movie_width, int movie_height);
|
|
void free_font_desc(font_desc_t *desc);
|
|
|
|
void render_one_glyph(font_desc_t *desc, int c);
|
|
int kerning(font_desc_t *desc, int prevc, int c);
|
|
|
|
void load_font_ft(int width, int height);
|
|
|
|
#else
|
|
|
|
static void render_one_glyph(font_desc_t *desc, int c) {}
|
|
static int kerning(font_desc_t *desc, int prevc, int c) { return 0; }
|
|
|
|
#endif
|
|
|
|
raw_file* load_raw(char *name,int verbose);
|
|
font_desc_t* read_font_desc(char* fname,float factor,int verbose);
|
|
|
|
#endif /* ! __MPLAYER_FONT_LOAD_H */
|