mirror of
https://github.com/mpv-player/mpv
synced 2024-08-04 14:59:58 +02:00
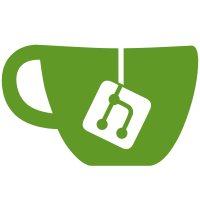
The af_lavrresample commit made compilation fail on Libav 10, so I think it's time to require somewhat more recent dependencies. Libav 11 is the latest release, and FFmpeg 2.4 seems to correspond to Libav 11. So use these. Also adjust the configure failure message. Instead of (accidentally) printing the pkg-config versions twice, print the release version numbers too. This is helpful, because the release version numbers are completely different from the pkg-config ones. I will probably remove some compatibility hacks in the following commits too.
148 lines
2.8 KiB
Ruby
Executable File
148 lines
2.8 KiB
Ruby
Executable File
#!/usr/bin/ruby
|
|
|
|
class TravisDepsBuilder
|
|
def self.make(name)
|
|
instance = klass.new(name)
|
|
instance.fill_data
|
|
instance.deps
|
|
instance.build
|
|
end
|
|
|
|
def self.klass
|
|
Module.const_get([self.name, self.os.capitalize].join)
|
|
rescue NameError
|
|
self
|
|
end
|
|
|
|
def self.os
|
|
ENV['TRAVIS_OS_NAME']
|
|
end
|
|
|
|
attr_reader :name, :url, :action, :os
|
|
|
|
def initialize(name)
|
|
@name, @os = name, self.class.os
|
|
end
|
|
|
|
def fill_data
|
|
data = build_map.fetch(name)
|
|
@url, @action = data[:url], data[:action]
|
|
end
|
|
|
|
def build
|
|
send(action)
|
|
end
|
|
|
|
def deps; end
|
|
|
|
private
|
|
def package_manager_update
|
|
# yes class variable, you wanna update only once across all instances
|
|
@@updated ||= false
|
|
return if @@updated
|
|
sh({'linux' => 'sudo apt-get update -y', 'osx' => 'brew update'}[os])
|
|
@@updated = true
|
|
end
|
|
|
|
def package_install(*packages)
|
|
cmd = {
|
|
'linux' => 'sudo apt-get install %s -y',
|
|
'osx' => 'brew install %s'
|
|
}[os] % [packages.join(" ")]
|
|
|
|
sh cmd
|
|
end
|
|
|
|
def git
|
|
sh "git clone --depth=1 #{url} #{name}"
|
|
compile name
|
|
end
|
|
|
|
def stable
|
|
filename = File.basename(url)
|
|
sh "wget #{url}"
|
|
sh "tar -xzvf #{filename}"
|
|
dirname = File.basename(url, ".tar.gz" )
|
|
compile dirname
|
|
end
|
|
|
|
def package
|
|
package_install(url)
|
|
end
|
|
|
|
def compile(dirname)
|
|
sh "cd #{dirname} && #{configure} && make && sudo make install"
|
|
sh "cd $TRAVIS_BUILD_DIR"
|
|
end
|
|
|
|
def configure
|
|
"./configure"
|
|
end
|
|
|
|
def sh(command)
|
|
`#{command}`
|
|
end
|
|
end
|
|
|
|
class Libav < TravisDepsBuilder
|
|
def build_map
|
|
{
|
|
"libav-stable" => {
|
|
:action => :stable,
|
|
:url => 'http://libav.org/releases/libav-11.tar.gz'
|
|
},
|
|
"libav-git" => {
|
|
:action => :git,
|
|
:url => "git://git.libav.org/libav.git"
|
|
},
|
|
"ffmpeg-stable" => {
|
|
:action => :stable,
|
|
:url => 'http://www.ffmpeg.org/releases/ffmpeg-2.4.tar.gz'
|
|
},
|
|
"ffmpeg-git" => {
|
|
:action => :git,
|
|
:url => "git://github.com/FFmpeg/FFmpeg.git"
|
|
}
|
|
}
|
|
end
|
|
|
|
def configure
|
|
[super, "--cc=#{ENV['CC']}"].join(" ")
|
|
end
|
|
end
|
|
|
|
class LibavOsx < Libav
|
|
def build_map
|
|
{
|
|
"ffmpeg-stable" => { :action => :package, :url => 'ffmpeg' },
|
|
}
|
|
end
|
|
end
|
|
|
|
class Libass < TravisDepsBuilder
|
|
def build_map
|
|
{
|
|
"libass-stable" => {
|
|
:action => :stable,
|
|
:url => 'https://github.com/libass/libass/releases/download/0.12.1/libass-0.12.1.tar.gz'
|
|
}
|
|
}
|
|
end
|
|
end
|
|
|
|
class Dependencies < TravisDepsBuilder
|
|
def deps
|
|
packages = {
|
|
'linux' => 'pkg-config fontconfig libfribidi-dev yasm',
|
|
'osx' => 'pkg-config fontconfig freetype fribidi yasm'
|
|
}
|
|
package_manager_update
|
|
package_install(packages.fetch(os))
|
|
end
|
|
end
|
|
|
|
Dependencies.new(:deps).deps
|
|
|
|
Libass.make(ARGV[0])
|
|
Libav.make(ARGV[1])
|