mirror of
https://github.com/mpv-player/mpv
synced 2024-10-26 07:22:17 +02:00
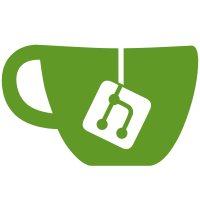
This commit makes audio decoding non-blocking. If e.g. the network is
too slow the playloop will just go to sleep, instead of blocking until
enough data is available.
For video, this was already done with commit 7083f88c
. For audio, it's
unfortunately much more complicated, because the audio decoder was used
in a blocking manner. Large changes are required to get around this.
The whole playback restart mechanism must be turned into a statemachine,
especially since it has close interactions with video restart. Lots of
video code is thus also changed.
(For the record, I don't think switching this code to threads would
make this conceptually easier: the code would still have to deal with
external input while blocked, so these in-between states do get visible
[and thus need to be handled] anyway. On the other hand, it certainly
should be possible to modularize this code a bit better.)
This will probably cause a bunch of regressions.
72 lines
2.3 KiB
C
72 lines
2.3 KiB
C
/*
|
|
* This file is part of MPlayer.
|
|
*
|
|
* MPlayer is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* MPlayer is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with MPlayer; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#ifndef MPLAYER_DEC_AUDIO_H
|
|
#define MPLAYER_DEC_AUDIO_H
|
|
|
|
#include "audio/chmap.h"
|
|
#include "audio/audio.h"
|
|
#include "demux/demux.h"
|
|
#include "demux/stheader.h"
|
|
|
|
struct mp_audio_buffer;
|
|
struct mp_decoder_list;
|
|
|
|
struct dec_audio {
|
|
struct mp_log *log;
|
|
struct MPOpts *opts;
|
|
struct mpv_global *global;
|
|
const struct ad_functions *ad_driver;
|
|
struct sh_stream *header;
|
|
struct mp_audio_buffer *decode_buffer;
|
|
struct af_stream *afilter;
|
|
char *decoder_desc;
|
|
struct replaygain_data *replaygain_data;
|
|
// set by decoder
|
|
struct mp_audio decoded; // decoded audio set by last decode_packet() call
|
|
int bitrate; // input bitrate, can change with VBR sources
|
|
// last known pts value in output from decoder
|
|
double pts;
|
|
// number of samples output by decoder after last known pts
|
|
int pts_offset;
|
|
// For free use by the ad_driver
|
|
void *priv;
|
|
};
|
|
|
|
enum {
|
|
AD_OK = 0,
|
|
AD_ERR = -1,
|
|
AD_EOF = -2,
|
|
AD_NEW_FMT = -3,
|
|
AD_WAIT = -4,
|
|
};
|
|
|
|
struct mp_decoder_list *audio_decoder_list(void);
|
|
int audio_init_best_codec(struct dec_audio *d_audio, char *audio_decoders);
|
|
int audio_decode(struct dec_audio *d_audio, struct mp_audio_buffer *outbuf,
|
|
int minsamples);
|
|
int initial_audio_decode(struct dec_audio *d_audio);
|
|
void audio_reset_decoding(struct dec_audio *d_audio);
|
|
void audio_uninit(struct dec_audio *d_audio);
|
|
|
|
int audio_init_filters(struct dec_audio *d_audio, int in_samplerate,
|
|
int *out_samplerate, struct mp_chmap *out_channels,
|
|
int *out_format);
|
|
|
|
#endif /* MPLAYER_DEC_AUDIO_H */
|