mirror of
https://github.com/mpv-player/mpv
synced 2024-11-14 22:48:35 +01:00
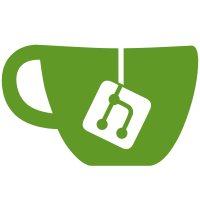
Not needed anymore. I'm not opposed to having asm, but inline asm is too much of a pain, and it was planned long ago to eventually get rid fo all inline asm uses. For the note, the inline asm use that was removed with the previous commits was almost worthless. It was confined to video filters, and most video filtering is now done with libavfilter. Some mpv filters (like vf_pullup) actually redirect to libavfilter if possible. If asm is added in the future, it should happen in the form of external files.
3260 lines
83 KiB
Bash
Executable File
3260 lines
83 KiB
Bash
Executable File
#! /bin/sh
|
|
#
|
|
# Original version (C) 2000 Pontscho/fresh!mindworkz
|
|
# pontscho@makacs.poliod.hu
|
|
#
|
|
# History / Contributors: Check the Subversion log.
|
|
#
|
|
# Cleanups all over the place (c) 2001 pl
|
|
#
|
|
#
|
|
# This configure script is *not* autoconf-based and has different semantics.
|
|
# It attempts to autodetect all settings and options where possible. It is
|
|
# possible to override autodetection with the --enable-option/--disable-option
|
|
# command line parameters. --enable-option forces the option on skipping
|
|
# autodetection. Yes, this means that compilation may fail and yes, this is not
|
|
# how autoconf-based configure scripts behave.
|
|
#
|
|
# configure generates a series of configuration files:
|
|
# - config.h contains #defines that are used in the C code.
|
|
# - config.mak is included from the Makefiles.
|
|
#
|
|
# If you want to add a new check for $feature, look at the existing checks
|
|
# and try to use helper functions where you can.
|
|
#
|
|
# Furthermore you need to add the variable _feature to the list of default
|
|
# settings and set it to one of yes/no/auto. Also add appropriate
|
|
# --enable-feature/--disable-feature command line options.
|
|
# The results of the check should be written to config.h and config.mak
|
|
# at the end of this script. The variable names used for this should be
|
|
# uniform, i.e. if the option is named 'feature':
|
|
#
|
|
# _feature : should have a value of yes/no/auto
|
|
# def_feature : '#define ... 1' or '#undef ...' for conditional compilation
|
|
# _ld_feature : '-L/path/dir -lfeature' GCC options
|
|
#
|
|
#############################################################################
|
|
|
|
# Prevent locale nonsense from breaking basic text processing utils
|
|
export LC_ALL=C
|
|
|
|
# Store the configure line that was used
|
|
configuration="$*"
|
|
|
|
# Prefer these macros to full length text !
|
|
# These macros only return an error code - NO display is done
|
|
command_check() {
|
|
echo >> "$TMPLOG"
|
|
echo "$@" >> "$TMPLOG"
|
|
"$@" >> "$TMPLOG" 2>&1
|
|
TMPRES="$?"
|
|
echo >> "$TMPLOG"
|
|
return "$TMPRES"
|
|
}
|
|
|
|
compile_check() {
|
|
source="$1"
|
|
shift
|
|
echo >> "$TMPLOG"
|
|
cat "$source" >> "$TMPLOG"
|
|
echo >> "$TMPLOG"
|
|
echo "$_cc $WARNFLAGS $WARN_CFLAGS $CFLAGS $source $extra_cflags $_ld_static $extra_ldflags $libs_mplayer -o $TMPEXE $@" >> "$TMPLOG"
|
|
rm -f "$TMPEXE"
|
|
$_cc $WARNFLAGS $WARN_CFLAGS $CFLAGS "$source" $extra_cflags $_ld_static $extra_ldflags $libs_mplayer -o "$TMPEXE" "$@" >> "$TMPLOG" 2>&1
|
|
TMPRES="$?"
|
|
echo >> "$TMPLOG"
|
|
echo >> "$TMPLOG"
|
|
return "$TMPRES"
|
|
}
|
|
|
|
cc_check() {
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
cxx_check() {
|
|
compile_check $TMPCPP $@ -lstdc++
|
|
}
|
|
|
|
cflag_check() {
|
|
cat > $TMPC << EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
header_check() {
|
|
cat > $TMPC << EOF
|
|
#include <$1>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
shift
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
return_check() {
|
|
cat > $TMPC << EOF
|
|
#include <$1>
|
|
int main(void) { return $2; }
|
|
EOF
|
|
shift 2
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
statement_check() {
|
|
cat > $TMPC << EOF
|
|
#include <$1>
|
|
int main(void) { $2; return 0; }
|
|
EOF
|
|
shift
|
|
shift
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
define_statement_check() {
|
|
cat > $TMPC << EOF
|
|
#define $1
|
|
#include <$2>
|
|
int main(void) { $3; return 0; }
|
|
EOF
|
|
shift 3
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
return_statement_check() {
|
|
cat > $TMPC << EOF
|
|
#include <$1>
|
|
int main(void) { $2; return $3; }
|
|
EOF
|
|
shift 3
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
# The following checks are special and should only be used with broken and
|
|
# non-selfsufficient headers that do not include all of their dependencies.
|
|
|
|
header_check_broken() {
|
|
cat > $TMPC << EOF
|
|
#include <$1>
|
|
#include <$2>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
shift
|
|
shift
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
statement_check_broken() {
|
|
cat > $TMPC << EOF
|
|
#include <$1>
|
|
#include <$2>
|
|
int main(void) { $3; return 0; }
|
|
EOF
|
|
shift 3
|
|
compile_check $TMPC $@
|
|
}
|
|
|
|
pkg_config_add() {
|
|
unset IFS # shell should not be used for programming
|
|
echo >> "$TMPLOG"
|
|
echo "$_pkg_config --cflags $@" >> "$TMPLOG"
|
|
ctmp=$($_pkg_config --cflags "$@" 2>> "$TMPLOG") || return $?
|
|
echo >> "$TMPLOG"
|
|
echo "$_pkg_config --libs $@" >> "$TMPLOG"
|
|
ltmp=$($_pkg_config --libs "$@" 2>> "$TMPLOG") || return $?
|
|
echo >> "$TMPLOG"
|
|
echo "cflags: $ctmp" >> "$TMPLOG"
|
|
echo "libs: $ltmp" >> "$TMPLOG"
|
|
echo >> "$TMPLOG"
|
|
extra_cflags="$extra_cflags $ctmp"
|
|
libs_mplayer="$libs_mplayer $ltmp"
|
|
}
|
|
|
|
tmp_run() {
|
|
"$TMPEXE" >> "$TMPLOG" 2>&1
|
|
}
|
|
|
|
# Display error message, flushes tempfile, exit
|
|
die () {
|
|
echo
|
|
echo "Error: $@" >&2
|
|
echo >&2
|
|
rm -f "$TMPEXE" "$TMPC" "$TMPS" "$TMPCPP"
|
|
echo "Check \"$TMPLOG\" if you do not understand why it failed."
|
|
exit 1
|
|
}
|
|
|
|
# OS test booleans functions
|
|
issystem() {
|
|
test "$(echo $system_name | tr A-Z a-z)" = "$(echo $1 | tr A-Z a-z)"
|
|
}
|
|
cygwin() { issystem "CYGWIN"; }
|
|
darwin() { issystem "Darwin"; }
|
|
dragonfly() { issystem "DragonFly"; }
|
|
freebsd() { issystem "FreeBSD" || issystem "GNU/kFreeBSD"; }
|
|
gnu() { issystem "GNU"; }
|
|
linux() { issystem "Linux"; }
|
|
mingw32() { issystem "MINGW32"; }
|
|
morphos() { issystem "MorphOS"; }
|
|
netbsd() { issystem "NetBSD"; }
|
|
openbsd() { issystem "OpenBSD"; }
|
|
win32() { cygwin || mingw32; }
|
|
|
|
# Use this before starting a check
|
|
echocheck() {
|
|
echo "============ Checking for $@ ============" >> "$TMPLOG"
|
|
echo ${_echo_n} "Checking for $@ ... ${_echo_c}"
|
|
}
|
|
|
|
# Use this to echo the results of a check
|
|
echores() {
|
|
if test "$res_comment" ; then
|
|
res_comment="($res_comment)"
|
|
fi
|
|
echo "Result is: $@ $res_comment" >> "$TMPLOG"
|
|
echo "##########################################" >> "$TMPLOG"
|
|
echo "" >> "$TMPLOG"
|
|
echo "$@ $res_comment"
|
|
res_comment=""
|
|
}
|
|
#############################################################################
|
|
|
|
# Check how echo works in this /bin/sh
|
|
case $(echo -n) in
|
|
-n) _echo_n= _echo_c='\c' ;; # SysV echo
|
|
*) _echo_n='-n ' _echo_c= ;; # BSD echo
|
|
esac
|
|
|
|
|
|
show_help(){
|
|
cat << EOF
|
|
Usage: $0 [OPTIONS]...
|
|
|
|
Configuration:
|
|
-h, --help display this help and exit
|
|
|
|
Installation directories:
|
|
--prefix=DIR prefix directory for installation [/usr/local]
|
|
--bindir=DIR directory for installing binaries [PREFIX/bin]
|
|
--datadir=DIR directory for installing machine independent
|
|
data files (skins, etc) [PREFIX/share/mpv]
|
|
--mandir=DIR directory for installing man pages [PREFIX/share/man]
|
|
--docdir=DIR directory for installing docs [PREFIX/share/doc/mpv]
|
|
--confdir=DIR directory for installing configuration files
|
|
[PREFIX/etc/mpv]
|
|
|
|
Optional features:
|
|
--disable-encoding disable encoding functionality [enable]
|
|
--disable-lua disable Lua scripting support [autodetect]
|
|
--lua=LUA select Lua package which should be autodetected
|
|
Choices: 51 51deb 52 52deb luajit
|
|
--disable-libguess disable libguess [autodetect]
|
|
--enable-terminfo use terminfo database for key codes [autodetect]
|
|
--enable-termcap use termcap database for key codes [autodetect]
|
|
--enable-termios use termios database for key codes [autodetect]
|
|
--disable-iconv disable iconv for encoding conversion [autodetect]
|
|
--enable-lirc enable LIRC (remote control) support [autodetect]
|
|
--enable-joystick enable joystick support [disable]
|
|
--disable-vm disable X video mode extensions [autodetect]
|
|
--disable-xf86keysym disable support for multimedia keys [autodetect]
|
|
--disable-tv disable TV interface (TV/DVB grabbers) [enable]
|
|
--disable-tv-v4l2 disable Video4Linux2 TV interface [autodetect]
|
|
--disable-libv4l2 disable libv4l2 [autodetect]
|
|
--disable-pvr disable Video4Linux2 MPEG PVR [autodetect]
|
|
--enable-smb enable Samba (SMB) input [autodetect]
|
|
--disable-libquvi4 disable libquvi 0.4.x [autodetect]
|
|
--disable-libquvi9 disable libquvi 0.9.x [autodetect]
|
|
--enable-lcms2 enable LCMS2 support [autodetect]
|
|
--disable-vcd disable VCD support [autodetect]
|
|
--disable-bluray disable Blu-ray support [autodetect]
|
|
--disable-dvdread disable libdvdread [autodetect]
|
|
--disable-dvdnav disable libdvdnav [autodetect]
|
|
--disable-enca disable ENCA charset oracle library [autodetect]
|
|
--disable-pthreads disable Posix threads support [autodetect]
|
|
--disable-libass disable subtitle rendering with libass [autodetect]
|
|
--disable-libass-osd disable OSD rendering with libass [autodetect]
|
|
--enable-rpath enable runtime linker path for extra libs [disabled]
|
|
--disable-libpostproc disable postprocess filter (vf_pp) [autodetect]
|
|
--disable-libavdevice disable libavdevice demuxers [autodetect]
|
|
--disable-libavfilter disable libavfilter [autodetect]
|
|
--disable-vapoursynth disable VapourSynth filter bridge [autodetect]
|
|
|
|
Codecs:
|
|
--enable-jpeg enable JPEG input/output support [autodetect]
|
|
--enable-libcdio enable libcdio support [autodetect]
|
|
--enable-libav skip Libav autodetection [autodetect]
|
|
--disable-ladspa disable LADSPA plugin support [autodetect]
|
|
--disable-libbs2b disable libbs2b audio filter support [autodetect]
|
|
--disable-mpg123 disable libmpg123 MP3 decoding support [autodetect]
|
|
|
|
Resampler:
|
|
--disable-libavresample check for libswresample only [autodetect]
|
|
|
|
Video output:
|
|
--enable-gl enable OpenGL video output [autodetect]
|
|
--enable-caca enable CACA video output [autodetect]
|
|
--enable-direct3d enable Direct3D video output [autodetect]
|
|
--enable-sdl enable SDL audio output [disable]
|
|
--enable-sdl2 enable SDL 2.0+ audio and video output [disable]
|
|
--enable-xv enable Xv video output [autodetect]
|
|
--enable-vdpau enable VDPAU acceleration [autodetect]
|
|
--enable-vda enable VDA acceleration [autodetect]
|
|
--enable-vaapi enable VAAPI acceleration [autodetect]
|
|
--enable-vm enable XF86VidMode support [autodetect]
|
|
--enable-xinerama enable Xinerama support [autodetect]
|
|
--enable-x11 enable X11 video output [autodetect]
|
|
--enable-wayland enable Wayland video output [autodetect]
|
|
--disable-xss disable screensaver support via xss [autodetect]
|
|
--disable-corevideo disable CoreVideo video output [autodetect]
|
|
--disable-cocoa disable Cocoa OpenGL backend [autodetect]
|
|
|
|
Audio output:
|
|
--disable-alsa disable ALSA audio output [autodetect]
|
|
--disable-ossaudio disable OSS audio output [autodetect]
|
|
--disable-rsound disable RSound audio output [autodetect]
|
|
--disable-sndio disable sndio audio output [autodetect]
|
|
--disable-pulse disable Pulseaudio audio output [autodetect]
|
|
--disable-portaudio disable PortAudio audio output [autodetect]
|
|
--disable-jack disable JACK audio output [autodetect]
|
|
--enable-openal enable OpenAL audio output [disable]
|
|
--disable-coreaudio disable CoreAudio audio output [autodetect]
|
|
--disable-dsound disable DirectSound audio output [autodetect]
|
|
--disable-wasapi disable WASAPI (event mode) audio output [autodetect]
|
|
--disable-select disable using select() on the audio device [enable]
|
|
|
|
Miscellaneous options:
|
|
--enable-cross-compile enable cross-compilation [disable]
|
|
--cc=COMPILER C compiler to build mpv [gcc]
|
|
--pkg-config=PKGCONFIG pkg-config to find some libraries [pkg-config]
|
|
--windres=WINDRES windres to build mpv [windres]
|
|
--target=PLATFORM target platform (i386-linux, arm-linux, etc)
|
|
--enable-static build a statically linked binary
|
|
--with-install=PATH path to a custom install program
|
|
--disable-manpage do not build and install manpage [auto]
|
|
--disable-pdf do not build and install PDF manual [auto]
|
|
--disable-build-date do not include binary compile time
|
|
|
|
Advanced options:
|
|
--enable-shm enable shm [autodetect]
|
|
--disable-debug compile-in debugging information [enable]
|
|
--disable-optimization compile without -O2 [enable]
|
|
|
|
Use these options if autodetection fails:
|
|
--extra-cflags=FLAGS extra CFLAGS
|
|
--extra-ldflags=FLAGS extra LDFLAGS
|
|
--extra-libs=FLAGS extra linker flags
|
|
--extra-libs-mpv=FLAGS extra linker flags for mpv
|
|
|
|
This configure script is NOT autoconf-based, even though its output is similar.
|
|
It will try to autodetect all configuration options. If you --enable an option
|
|
it will be forcefully turned on, skipping autodetection. This can break
|
|
compilation, so you need to know what you are doing.
|
|
EOF
|
|
exit 0
|
|
} #show_help()
|
|
|
|
# GOTCHA: the variables below defines the default behavior for autodetection
|
|
# and have - unless stated otherwise - at least 2 states : yes no
|
|
# If autodetection is available then the third state is: auto
|
|
_install=install
|
|
_pkg_config=auto
|
|
_windres=auto
|
|
_cc=auto
|
|
test "$CC" && _cc="$CC"
|
|
_debug=-g
|
|
_opt=-O2
|
|
_cross_compile=no
|
|
_prefix="/usr/local"
|
|
ffmpeg=auto
|
|
_encoding=yes
|
|
_disable_avresample=no
|
|
_x11=auto
|
|
_wayland=auto
|
|
_xss=auto
|
|
_xv=auto
|
|
_vdpau=auto
|
|
_vda=auto
|
|
_vda_refcounting=auto
|
|
_vaapi=auto
|
|
_direct3d=auto
|
|
_sdl=no
|
|
_sdl2=no
|
|
_dsound=auto
|
|
_wasapi=auto
|
|
_jpeg=auto
|
|
_gl=auto
|
|
_aa=auto
|
|
_caca=auto
|
|
_dvb=auto
|
|
_iconv=auto
|
|
_ossaudio=auto
|
|
_rsound=auto
|
|
_pulse=auto
|
|
_portaudio=auto
|
|
_jack=auto
|
|
_openal=no
|
|
_libcdio=auto
|
|
_mpg123=auto
|
|
_ladspa=auto
|
|
_libbs2b=auto
|
|
_vcd=auto
|
|
_bluray=auto
|
|
_dvdread=auto
|
|
_dvdnav=auto
|
|
_lcms2=auto
|
|
_vapoursynth=auto
|
|
_xinerama=auto
|
|
_vm=auto
|
|
_xf86keysym=auto
|
|
_sndio=auto
|
|
_alsa=auto
|
|
_select=yes
|
|
_tv=yes
|
|
_tv_v4l2=auto
|
|
_libv4l2=auto
|
|
_pvr=auto
|
|
_smb=auto
|
|
_libquvi4=auto
|
|
_libquvi9=auto
|
|
_libguess=auto
|
|
_joystick=no
|
|
_lirc=auto
|
|
_terminfo=auto
|
|
_termcap=auto
|
|
_termios=auto
|
|
_shm=auto
|
|
_cdda=auto
|
|
_coreaudio=auto
|
|
_corevideo=auto
|
|
_cocoa=auto
|
|
_enca=auto
|
|
_pthreads=auto
|
|
_ass=auto
|
|
_libass_osd=auto
|
|
_rpath=no
|
|
lua=auto
|
|
libpostproc=auto
|
|
libavfilter=auto
|
|
libavdevice=auto
|
|
_stream_cache=yes
|
|
_priority=no
|
|
def_dos_paths="#define HAVE_DOS_PATHS 0"
|
|
def_priority="#define HAVE_PRIORITY 0"
|
|
_build_man=auto
|
|
_build_pdf=auto
|
|
_build_date=yes
|
|
for ac_option do
|
|
case "$ac_option" in
|
|
--help|-help|-h)
|
|
show_help
|
|
;;
|
|
--prefix=*)
|
|
_prefix=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--bindir=*)
|
|
_bindir=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--mandir=*)
|
|
_mandir=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--docdir=*)
|
|
_docdir=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--confdir=*)
|
|
_confdir=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
|
|
--with-install=*)
|
|
_install=$(echo $ac_option | cut -d '=' -f 2 )
|
|
;;
|
|
|
|
--extra-cflags=*)
|
|
extra_cflags="$extra_cflags $(echo $ac_option | cut -d '=' -f 2-)"
|
|
;;
|
|
--extra-ldflags=*)
|
|
extra_ldflags="$extra_ldflags $(echo $ac_option | cut -d '=' -f 2-)"
|
|
;;
|
|
--extra-libs=*)
|
|
extra_libs=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--extra-libs-mpv=*)
|
|
libs_mplayer=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
|
|
--target=*)
|
|
_target=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--cc=*)
|
|
_cc=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--pkg-config=*)
|
|
_pkg_config=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--windres=*)
|
|
_windres=$(echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
|
|
--enable-static)
|
|
_ld_static='-static'
|
|
;;
|
|
--disable-static)
|
|
_ld_static=''
|
|
;;
|
|
--enable-debug)
|
|
_debug='-g'
|
|
;;
|
|
--enable-debug=*)
|
|
_debug=$(echo $_echo_n '-g'$_echo_c; echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--disable-debug)
|
|
_debug=
|
|
;;
|
|
--enable-optimization)
|
|
_opt='-O2'
|
|
;;
|
|
--enable-optimization=*)
|
|
_opt=$(echo $_echo_n '-O'$_echo_c; echo $ac_option | cut -d '=' -f 2)
|
|
;;
|
|
--disable-optimization)
|
|
_opt=
|
|
;;
|
|
--enable-cross-compile) _cross_compile=yes ;;
|
|
--disable-cross-compile) _cross_compile=no ;;
|
|
--enable-encoding) _encoding=yes ;;
|
|
--disable-encoding) _encoding=no ;;
|
|
--enable-wayland) _wayland=yes ;;
|
|
--disable-wayland) _wayland=no ;;
|
|
--enable-x11) _x11=yes ;;
|
|
--disable-x11) _x11=no ;;
|
|
--enable-xss) _xss=yes ;;
|
|
--disable-xss) _xss=no ;;
|
|
--enable-xv) _xv=yes ;;
|
|
--disable-xv) _xv=no ;;
|
|
--enable-vdpau) _vdpau=yes ;;
|
|
--disable-vdpau) _vdpau=no ;;
|
|
--enable-vda) _vda=yes ;;
|
|
--disable-vda) _vda=no ;;
|
|
--enable-vaapi) _vaapi=yes ;;
|
|
--disable-vaapi) _vaapi=no ;;
|
|
--enable-direct3d) _direct3d=yes ;;
|
|
--disable-direct3d) _direct3d=no ;;
|
|
--enable-sdl) _sdl=yes ;;
|
|
--disable-sdl) _sdl=no ;;
|
|
--enable-sdl2) _sdl2=yes ;;
|
|
--disable-sdl2) _sdl2=no ;;
|
|
--enable-dsound) _dsound=yes ;;
|
|
--disable-dsound) _dsound=no ;;
|
|
--enable-wasapi) _wasapi=yes ;;
|
|
--disable-wasapi) _wasapi=no ;;
|
|
--enable-jpeg) _jpeg=yes ;;
|
|
--disable-jpeg) _jpeg=no ;;
|
|
--enable-gl) _gl=yes ;;
|
|
--disable-gl) _gl=no ;;
|
|
--enable-caca) _caca=yes ;;
|
|
--disable-caca) _caca=no ;;
|
|
--enable-dvb) _dvb=yes ;;
|
|
--disable-dvb) _dvb=no ;;
|
|
--enable-iconv) _iconv=yes ;;
|
|
--disable-iconv) _iconv=no ;;
|
|
--enable-ossaudio) _ossaudio=yes ;;
|
|
--disable-ossaudio) _ossaudio=no ;;
|
|
--enable-rsound) _rsound=yes ;;
|
|
--disable-rsound) _rsound=no ;;
|
|
--enable-sndio) _sndio=yes ;;
|
|
--disable-sndio) _sndio=no ;;
|
|
--enable-pulse) _pulse=yes ;;
|
|
--disable-pulse) _pulse=no ;;
|
|
--enable-portaudio) _portaudio=yes ;;
|
|
--disable-portaudio) _portaudio=no ;;
|
|
--enable-jack) _jack=yes ;;
|
|
--disable-jack) _jack=no ;;
|
|
--enable-openal) _openal=auto ;;
|
|
--disable-openal) _openal=no ;;
|
|
--enable-libcdio) _libcdio=yes ;;
|
|
--disable-libcdio) _libcdio=no ;;
|
|
--enable-mpg123) _mpg123=yes ;;
|
|
--disable-mpg123) _mpg123=no ;;
|
|
--enable-ladspa) _ladspa=yes ;;
|
|
--disable-ladspa) _ladspa=no ;;
|
|
--enable-libbs2b) _libbs2b=yes ;;
|
|
--disable-libbs2b) _libbs2b=no ;;
|
|
--enable-vcd) _vcd=yes ;;
|
|
--disable-vcd) _vcd=no ;;
|
|
--enable-bluray) _bluray=yes ;;
|
|
--disable-bluray) _bluray=no ;;
|
|
--enable-dvdread) _dvdread=yes ;;
|
|
--disable-dvdread) _dvdread=no ;;
|
|
--enable-dvdnav) _dvdnav=yes ;;
|
|
--disable-dvdnav) _dvdnav=no ;;
|
|
--enable-lcms2) _lcms2=yes ;;
|
|
--disable-lcms2) _lcms2=no ;;
|
|
--enable-vapoursynth) _vapoursynth=yes ;;
|
|
--dsiable-vapoursynth)_vapoursynth=no ;;
|
|
--enable-xinerama) _xinerama=yes ;;
|
|
--disable-xinerama) _xinerama=no ;;
|
|
--enable-vm) _vm=yes ;;
|
|
--disable-vm) _vm=no ;;
|
|
--enable-xf86keysym) _xf86keysym=yes ;;
|
|
--disable-xf86keysym) _xf86keysym=no ;;
|
|
--enable-alsa) _alsa=yes ;;
|
|
--disable-alsa) _alsa=no ;;
|
|
--enable-tv) _tv=yes ;;
|
|
--disable-tv) _tv=no ;;
|
|
--enable-tv-v4l2) _tv_v4l2=yes ;;
|
|
--disable-tv-v4l2) _tv_v4l2=no ;;
|
|
--enable-libv4l2) _libv4l2=yes ;;
|
|
--disable-libv4l2) _libv4l2=no ;;
|
|
--enable-pvr) _pvr=yes ;;
|
|
--disable-pvr) _pvr=no ;;
|
|
--enable-smb) _smb=yes ;;
|
|
--disable-smb) _smb=no ;;
|
|
--enable-libquvi4) _libquvi4=yes ;;
|
|
--disable-libquvi4) _libquvi4=no ;;
|
|
--enable-libquvi9) _libquvi9=yes ;;
|
|
--disable-libquvi9) _libquvi9=no ;;
|
|
--enable-libguess) _libguess=yes ;;
|
|
--disable-libguess) _libguess=no ;;
|
|
--enable-joystick) _joystick=yes ;;
|
|
--disable-joystick) _joystick=no ;;
|
|
--enable-libav) ffmpeg=yes ;;
|
|
--disable-libavresample) _disable_avresample=yes ;;
|
|
--enable-libavresample) _disable_avresample=no ;;
|
|
|
|
--enable-lua) lua=yes ;;
|
|
--disable-lua) lua=no ;;
|
|
--lua=*) lua_pkg=$(echo $ac_option | cut -d '=' -f 2) ;;
|
|
--enable-lirc) _lirc=yes ;;
|
|
--disable-lirc) _lirc=no ;;
|
|
--enable-terminfo) _terminfo=yes ;;
|
|
--disable-terminfo) _terminfo=no ;;
|
|
--enable-termcap) _termcap=yes ;;
|
|
--disable-termcap) _termcap=no ;;
|
|
--enable-termios) _termios=yes ;;
|
|
--disable-termios) _termios=no ;;
|
|
--enable-shm) _shm=yes ;;
|
|
--disable-shm) _shm=no ;;
|
|
--enable-select) _select=yes ;;
|
|
--disable-select) _select=no ;;
|
|
--enable-pthreads) _pthreads=yes ;;
|
|
--disable-pthreads) _pthreads=no ;;
|
|
--enable-libass) _ass=yes ;;
|
|
--disable-libass) _ass=no ;;
|
|
--enable-libass-osd) _libass_osd=yes ;;
|
|
--disable-libass-osd) _libass_osd=no ;;
|
|
--enable-rpath) _rpath=yes ;;
|
|
--disable-rpath) _rpath=no ;;
|
|
--enable-libpostproc) libpostproc=yes ;;
|
|
--disable-libpostproc) libpostproc=no ;;
|
|
--enable-libavdevice) libavdevice=auto ;;
|
|
--disable-libavdevice) libavdevice=no ;;
|
|
--enable-libavfilter) libavfilter=auto ;;
|
|
--disable-libavfilter) libavfilter=no ;;
|
|
|
|
--enable-enca) _enca=yes ;;
|
|
--disable-enca) _enca=no ;;
|
|
|
|
--enable-coreaudio) _coreaudio=yes ;;
|
|
--disable-coreaudio) _coreaudio=no ;;
|
|
--enable-corevideo) _corevideo=yes ;;
|
|
--disable-corevideo) _corevideo=no ;;
|
|
--enable-cocoa) _cocoa=yes ;;
|
|
--disable-cocoa) _cocoa=no ;;
|
|
|
|
--enable-manpage) _build_man=yes ;;
|
|
--disable-manpage) _build_man=no ;;
|
|
--enable-pdf) _build_pdf=yes ;;
|
|
--disable-pdf) _build_pdf=no ;;
|
|
|
|
--enable-build-date) _build_date=yes ;;
|
|
--disable-build-date) _build_date=no ;;
|
|
*)
|
|
echo "Unknown parameter: $ac_option" >&2
|
|
exit 1
|
|
;;
|
|
|
|
esac
|
|
done
|
|
|
|
# Atmos: moved this here, to be correct, if --prefix is specified
|
|
test -z "$_bindir" && _bindir="$_prefix/bin"
|
|
test -z "$_mandir" && _mandir="$_prefix/share/man"
|
|
test -z "$_docdir" && _docdir="$_prefix/share/doc/mpv"
|
|
test -z "$_confdir" && _confdir="$_prefix/etc/mpv"
|
|
|
|
for tmpdir in "$TMPDIR" "$TEMPDIR" "/tmp" ; do
|
|
test "$tmpdir" && break
|
|
done
|
|
|
|
mplayer_tmpdir="$tmpdir/mpv-configure-$RANDOM-$$"
|
|
mkdir $mplayer_tmpdir || die "Unable to create tmpdir."
|
|
|
|
TMPLOG="config.log"
|
|
|
|
rm -f "$TMPLOG"
|
|
echo Parameters configure was run with: > "$TMPLOG"
|
|
if test -n "$CFLAGS" ; then
|
|
echo ${_echo_n} CFLAGS="'$CFLAGS' ${_echo_c}" >> "$TMPLOG"
|
|
fi
|
|
if test -n "$PKG_CONFIG_PATH" ; then
|
|
echo ${_echo_n} PKG_CONFIG_PATH="'$PKG_CONFIG_PATH' ${_echo_c}" >> "$TMPLOG"
|
|
fi
|
|
echo ./configure $configuration >> "$TMPLOG"
|
|
echo >> "$TMPLOG"
|
|
|
|
|
|
echocheck "cross compilation"
|
|
echores $_cross_compile
|
|
|
|
if test $_cross_compile = yes; then
|
|
tmp_run() {
|
|
return 0
|
|
}
|
|
fi
|
|
|
|
tool_prefix=""
|
|
|
|
if test $_cross_compile = yes ; then
|
|
# Usually cross-compiler prefixes match with the target triplet
|
|
test -n "$_target" && tool_prefix="$_target"-
|
|
fi
|
|
|
|
test "$_windres" = auto && _windres="$tool_prefix"windres
|
|
test "$_pkg_config" = auto && _pkg_config="$tool_prefix"pkg-config
|
|
|
|
if test "$_cc" = auto ; then
|
|
if test -n "$tool_prefix" ; then
|
|
_cc="$tool_prefix"gcc
|
|
else
|
|
_cc=cc
|
|
fi
|
|
fi
|
|
|
|
# Determine our OS name
|
|
if test -z "$_target" ; then
|
|
# OS name
|
|
system_name=$(uname -s 2>&1)
|
|
case "$system_name" in
|
|
Linux|FreeBSD|NetBSD|OpenBSD|DragonFly|Darwin|GNU|MorphOS)
|
|
;;
|
|
Haiku)
|
|
system_name=Haiku
|
|
;;
|
|
GNU/kFreeBSD)
|
|
system_name=FreeBSD
|
|
;;
|
|
[cC][yY][gG][wW][iI][nN]*)
|
|
system_name=CYGWIN
|
|
;;
|
|
MINGW32*)
|
|
system_name=MINGW32
|
|
;;
|
|
*)
|
|
system_name="$system_name-UNKNOWN"
|
|
;;
|
|
esac
|
|
|
|
else # if test -z "$_target"
|
|
for i in 2 3; do
|
|
system_name=$(echo $_target | cut -d '-' -f $i)
|
|
case "$(echo $system_name | tr A-Z a-z)" in
|
|
linux) system_name=Linux ;;
|
|
freebsd) system_name=FreeBSD ;;
|
|
gnu/kfreebsd) system_name=FreeBSD ;;
|
|
netbsd) system_name=NetBSD ;;
|
|
openbsd) system_name=OpenBSD ;;
|
|
dragonfly) system_name=DragonFly ;;
|
|
morphos) system_name=MorphOS ;;
|
|
mingw32*) system_name=MINGW32 ;;
|
|
*) continue ;;
|
|
esac
|
|
break
|
|
done
|
|
fi
|
|
|
|
extra_cflags="-I. -D_GNU_SOURCE $extra_cflags"
|
|
_timer=timer-linux.c
|
|
_getch=terminal-unix.c
|
|
|
|
if freebsd || openbsd ; then
|
|
extra_ldflags="$extra_ldflags -L/usr/local/lib"
|
|
extra_cflags="$extra_cflags -I/usr/local/include"
|
|
fi
|
|
|
|
if netbsd || dragonfly ; then
|
|
extra_ldflags="$extra_ldflags -L/usr/pkg/lib"
|
|
extra_cflags="$extra_cflags -I/usr/pkg/include"
|
|
fi
|
|
|
|
if darwin; then
|
|
extra_cflags="-mdynamic-no-pic $extra_cflags"
|
|
_timer=timer-darwin.c
|
|
fi
|
|
|
|
_win32=no
|
|
if win32 ; then
|
|
_win32=yes
|
|
_exesuf=".exe"
|
|
extra_cflags="$extra_cflags -fno-common"
|
|
# -lwinmm is always needed for osdep/timer-win2.c
|
|
libs_mplayer="$libs_mplayer -lwinmm"
|
|
_pe_executable=yes
|
|
_timer=timer-win2.c
|
|
_priority=yes
|
|
def_dos_paths="#define HAVE_DOS_PATHS 1"
|
|
def_priority="#define HAVE_PRIORITY 1"
|
|
fi
|
|
|
|
if mingw32 ; then
|
|
_getch=terminal-win.c
|
|
extra_cflags="$extra_cflags -D__USE_MINGW_ANSI_STDIO=1"
|
|
# Hack for missing BYTE_ORDER declarations in <sys/types.h>.
|
|
# (For some reason, they are in <sys/param.h>, but we don't bother switching
|
|
# the includes based on whether we're compiling for MinGW.)
|
|
extra_cflags="$extra_cflags -DBYTE_ORDER=1234 -DLITTLE_ENDIAN=1234 -DBIG_ENDIAN=4321"
|
|
fi
|
|
|
|
if cygwin ; then
|
|
extra_cflags="$extra_cflags -mwin32"
|
|
fi
|
|
|
|
_rst2man=rst2man
|
|
if [ -f "$(which rst2man.py)" ] ; then
|
|
_rst2man=rst2man.py
|
|
fi
|
|
|
|
echocheck "whether to build manpages with rst2man"
|
|
if test "$_build_man" = auto ; then
|
|
_build_man=no
|
|
command_check "$_rst2man" --version && _build_man=yes
|
|
else
|
|
_build_man=no
|
|
fi
|
|
echores "$_build_man"
|
|
|
|
_rst2pdf=rst2pdf
|
|
if [ -f "$(which rst2pdf.py)" ] ; then
|
|
_rst2pdf=rst2pdf.py
|
|
fi
|
|
|
|
echocheck "whether to build manual PDFs with rst2pdf"
|
|
pdfcheck() {
|
|
echo test | $_rst2pdf -c --repeat-table-rows -o "$mplayer_tmpdir/test.pdf"
|
|
}
|
|
|
|
if test "$_build_pdf" = auto ; then
|
|
_build_pdf=no
|
|
command_check pdfcheck && _build_pdf=yes
|
|
else
|
|
_build_pdf=no
|
|
fi
|
|
echores "$_build_pdf"
|
|
|
|
echocheck "whether to print binary build date"
|
|
if test "$_build_date" = yes ; then
|
|
_build_yes=yes
|
|
else
|
|
_build_date=no
|
|
extra_cflags="$extra_cflags -DNO_BUILD_TIMESTAMPS"
|
|
fi
|
|
echores "$_build_date"
|
|
|
|
|
|
|
|
TMPC="$mplayer_tmpdir/tmp.c"
|
|
TMPCPP="$mplayer_tmpdir/tmp.cpp"
|
|
TMPEXE="$mplayer_tmpdir/tmp$_exesuf"
|
|
TMPH="$mplayer_tmpdir/tmp.h"
|
|
TMPS="$mplayer_tmpdir/tmp.S"
|
|
|
|
# Checking CC version...
|
|
# Intel C++ Compilers (no autoselect, use CC=/some/binary ./configure)
|
|
if test "$(basename $_cc)" = "icc" || test "$(basename $_cc)" = "ecc"; then
|
|
echocheck "$_cc version"
|
|
cc_vendor=intel
|
|
cc_name=$($_cc -V 2>&1 | head -n 1 | cut -d ',' -f 1)
|
|
cc_version=$($_cc -V 2>&1 | head -n 1 | cut -d ',' -f 2 | cut -d ' ' -f 3)
|
|
_cc_major=$(echo $cc_version | cut -d '.' -f 1)
|
|
_cc_minor=$(echo $cc_version | cut -d '.' -f 2)
|
|
# TODO verify older icc/ecc compatibility
|
|
case $cc_version in
|
|
'')
|
|
cc_version="v. ?.??, bad"
|
|
cc_fail=yes
|
|
;;
|
|
10.1|11.0|11.1)
|
|
cc_version="$cc_version, ok"
|
|
;;
|
|
*)
|
|
cc_version="$cc_version, bad"
|
|
cc_fail=yes
|
|
;;
|
|
esac
|
|
echores "$cc_version"
|
|
else
|
|
for _cc in "$_cc" gcc cc ; do
|
|
cc_name_tmp=$($_cc -v 2>&1 | tail -n 1 | cut -d ' ' -f 1)
|
|
if test "$cc_name_tmp" = "gcc"; then
|
|
cc_name=$cc_name_tmp
|
|
echocheck "$_cc version"
|
|
cc_vendor=gnu
|
|
cc_version=$($_cc -dumpversion 2>&1)
|
|
case $cc_version in
|
|
2.96*)
|
|
cc_fail=yes
|
|
;;
|
|
*)
|
|
_cc_major=$(echo $cc_version | cut -d '.' -f 1)
|
|
_cc_minor=$(echo $cc_version | cut -d '.' -f 2)
|
|
_cc_mini=$(echo $cc_version | cut -d '.' -f 3)
|
|
;;
|
|
esac
|
|
echores "$cc_version"
|
|
break
|
|
fi
|
|
if $_cc -v 2>&1 | grep -q "clang"; then
|
|
echocheck "$_cc version"
|
|
cc_vendor=clang
|
|
cc_version=$($_cc -dumpversion 2>&1)
|
|
res_comment="experimental support only"
|
|
echores "clang $cc_version"
|
|
break
|
|
fi
|
|
cc_name_tmp=$($_cc -V 2>&1 | head -n 1 | cut -d ' ' -f 2,3)
|
|
done
|
|
fi # icc
|
|
test "$cc_fail" = yes && die "unsupported compiler version"
|
|
|
|
echocheck "working compiler"
|
|
cflag_check "" || die "Compiler is not functioning correctly. Check your installation and custom CFLAGS $CFLAGS ."
|
|
echo "yes"
|
|
|
|
echocheck "perl"
|
|
command_check perl -Mv5.8 -e';' || die "Perl is not functioning correctly or is ancient. Install the latest perl available."
|
|
echo yes
|
|
|
|
echo "Detected operating system: $system_name"
|
|
|
|
# ---
|
|
|
|
echocheck "compiler support of -pipe option"
|
|
# -I. helps to detect compilers that just misunderstand -pipe like Sun C
|
|
cflag_check -pipe -I. && _pipe="-pipe" && echores "yes" || echores "no"
|
|
|
|
# Checking for CFLAGS
|
|
if test -z "$CFLAGS" ; then
|
|
if test "$cc_vendor" = "intel" ; then
|
|
CFLAGS="$_opt $_debug $_pipe"
|
|
WARNFLAGS="-wd167 -wd556 -wd144"
|
|
elif test "$cc_vendor" = "clang"; then
|
|
CFLAGS="$_opt $_debug $_pipe"
|
|
WARNFLAGS="-Wall -Wno-switch -Wno-logical-op-parentheses -Wpointer-arith -Wundef -Wno-pointer-sign -Wmissing-prototypes -Wshadow -Wempty-body"
|
|
ERRORFLAGS="-Werror=implicit-function-declaration -Wno-error=deprecated-declarations -Wno-error=unused-function"
|
|
elif test "$cc_vendor" != "gnu" ; then
|
|
CFLAGS="$_opt $_debug $_pipe"
|
|
else
|
|
CFLAGS="$_opt $_debug $_pipe"
|
|
WARNFLAGS="-Wall -Wno-switch -Wno-parentheses -Wpointer-arith -Wredundant-decls"
|
|
ERRORFLAGS="-Werror-implicit-function-declaration -Wno-error=deprecated-declarations -Wno-error=unused-function -Wshadow -Wempty-body"
|
|
extra_ldflags="$extra_ldflags"
|
|
fi
|
|
else
|
|
warn_cflags=yes
|
|
fi
|
|
|
|
if test "$cc_vendor" = "gnu" ; then
|
|
cflag_check -Wundef && WARNFLAGS="-Wundef $WARNFLAGS"
|
|
# -std=c99 is not a warning flag but is placed in WARN_CFLAGS because
|
|
# that's the only variable specific to C now, and this option is not valid
|
|
# for C++.
|
|
cflag_check -std=c99 && WARN_CFLAGS="-std=c99 $WARN_CFLAGS"
|
|
cflag_check -Wno-pointer-sign && WARN_CFLAGS="-Wno-pointer-sign $WARN_CFLAGS"
|
|
cflag_check -Wdisabled-optimization && WARN_CFLAGS="-Wdisabled-optimization $WARN_CFLAGS"
|
|
cflag_check -Wmissing-prototypes && WARN_CFLAGS="-Wmissing-prototypes $WARN_CFLAGS"
|
|
cflag_check -Wstrict-prototypes && WARN_CFLAGS="-Wstrict-prototypes $WARN_CFLAGS"
|
|
else
|
|
CFLAGS="-D_ISOC99_SOURCE -D_BSD_SOURCE $CFLAGS"
|
|
fi
|
|
|
|
cflag_check -MD -MP && DEPFLAGS="-MD -MP"
|
|
|
|
|
|
if test -n "$LDFLAGS" ; then
|
|
extra_ldflags="$extra_ldflags $LDFLAGS"
|
|
warn_cflags=yes
|
|
elif test "$cc_vendor" = "intel" ; then
|
|
extra_ldflags="$extra_ldflags -i-static"
|
|
fi
|
|
if test -n "$CPPFLAGS" ; then
|
|
extra_cflags="$extra_cflags $CPPFLAGS"
|
|
warn_cflags=yes
|
|
fi
|
|
|
|
|
|
######################
|
|
# MAIN TESTS GO HERE #
|
|
######################
|
|
|
|
|
|
echocheck "-lm"
|
|
if cflag_check -lm ; then
|
|
_ld_lm="-lm"
|
|
echores "yes"
|
|
else
|
|
_ld_lm=""
|
|
echores "no"
|
|
fi
|
|
|
|
|
|
echocheck "nanosleep"
|
|
_nanosleep=no
|
|
statement_check time.h 'nanosleep(0, 0)' && _nanosleep=yes
|
|
if test "$_nanosleep" = yes ; then
|
|
def_nanosleep='#define HAVE_NANOSLEEP 1'
|
|
else
|
|
def_nanosleep='#define HAVE_NANOSLEEP 0'
|
|
fi
|
|
echores "$_nanosleep"
|
|
|
|
|
|
echocheck "mman.h"
|
|
_mman=no
|
|
statement_check sys/mman.h 'mmap(0, 0, 0, 0, 0, 0)' && _mman=yes
|
|
if test "$_mman" = yes ; then
|
|
def_mman_h='#define HAVE_SYS_MMAN_H 1'
|
|
else
|
|
def_mman_h='#define HAVE_SYS_MMAN_H 0'
|
|
fi
|
|
echores "$_mman"
|
|
|
|
|
|
echocheck "dynamic loader"
|
|
_dl=no
|
|
for _ld_tmp in "" "-ldl"; do
|
|
statement_check dlfcn.h 'dlopen("", 0)' $_ld_tmp && _ld_dl="$_ld_tmp" && _dl=yes && break
|
|
done
|
|
if test "$_dl" = yes ; then
|
|
def_dl='#define HAVE_LIBDL 1'
|
|
else
|
|
def_dl='#define HAVE_LIBDL 0'
|
|
fi
|
|
echores "$_dl"
|
|
|
|
|
|
echocheck "pthread"
|
|
def_pthreads='#define HAVE_PTHREADS 0'
|
|
if linux ; then
|
|
THREAD_CFLAGS=-D_REENTRANT
|
|
elif freebsd || netbsd || openbsd ; then
|
|
THREAD_CFLAGS=-D_THREAD_SAFE
|
|
fi
|
|
if test "$_pthreads" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <pthread.h>
|
|
static void *func(void *arg) { return arg; }
|
|
int main(void) {
|
|
pthread_t tid;
|
|
#ifdef PTW32_STATIC_LIB
|
|
pthread_win32_process_attach_np();
|
|
pthread_win32_thread_attach_np();
|
|
#endif
|
|
return pthread_create (&tid, 0, func, 0) != 0;
|
|
}
|
|
EOF
|
|
_pthreads=no
|
|
for _ld_tmp in "-lpthreadGC2" "" "-lpthread" "-pthread" ; do
|
|
# for crosscompilation, we cannot execute the program, be happy if we can link statically
|
|
cc_check $THREAD_CFLAGS $_ld_tmp && (tmp_run || test "$_ld_static") && _ld_pthread="$_ld_tmp" && _pthreads=yes && break
|
|
done
|
|
if test "$_pthreads" = no && mingw32 ; then
|
|
_ld_tmp="-lpthreadGC2 -lws2_32"
|
|
cc_check $_ld_tmp -DPTW32_STATIC_LIB && (tmp_run || test "$_ld_static") && _ld_pthread="$_ld_tmp" && _pthreads=yes && CFLAGS="$CFLAGS -DPTW32_STATIC_LIB"
|
|
fi
|
|
fi
|
|
if test "$_pthreads" = yes ; then
|
|
test "$_ld_pthread" && res_comment="using $_ld_pthread"
|
|
def_pthreads='#define HAVE_PTHREADS 1'
|
|
extra_cflags="$extra_cflags $THREAD_CFLAGS"
|
|
else
|
|
res_comment="v4l2 disabled"
|
|
def_pthreads='#define HAVE_PTHREADS 0'
|
|
_tv_v4l2=no
|
|
fi
|
|
echores "$_pthreads"
|
|
|
|
if test "$_pthreads" = no ; then
|
|
die "Unable to find pthreads support."
|
|
fi
|
|
|
|
|
|
echocheck "compiler support for __atomic built-ins"
|
|
_atomic=no
|
|
for _ld_tmp in "" "-latomic" ; do
|
|
statement_check stdint.h 'int64_t test = 0; test = __atomic_add_fetch(&test, 1, __ATOMIC_SEQ_CST)' $_ld_tmp &&
|
|
libs_mplayer="$libs_mplayer $_ld_tmp" && _atomic=yes && break
|
|
done
|
|
if test "$_atomic" = yes ; then
|
|
def_atomic="#define HAVE_ATOMIC_BUILTINS 1"
|
|
else
|
|
def_atomic="#define HAVE_ATOMIC_BUILTINS 0"
|
|
fi
|
|
echores "$_atomic"
|
|
|
|
if test "$_atomic" = no ; then
|
|
echocheck "compiler support for __sync built-ins"
|
|
_sync=no
|
|
statement_check stdint.h 'int64_t test = 0; test = __sync_add_and_fetch(&test, 1)' && _sync=yes
|
|
if test "$_sync" = yes ; then
|
|
def_sync="#define HAVE_SYNC_BUILTINS 1"
|
|
else
|
|
def_sync="#define HAVE_SYNC_BUILTINS 0"
|
|
fi
|
|
echores "$_sync"
|
|
else
|
|
def_sync="#define HAVE_SYNC_BUILTINS 0"
|
|
fi
|
|
|
|
if test "$_atomic" = no && test "$_sync" = no ; then
|
|
die "your compiler must support either __atomic or __sync built-ins."
|
|
fi
|
|
|
|
if test "$_pthreads" = yes ; then
|
|
|
|
# Cargo-cult for -lrt, which is needed on not so recent glibc version for
|
|
# clock_gettime. It's documented as required before before glibc 2.17, which
|
|
# was released in december 2012. On newer glibc versions or on other systems,
|
|
# this will hopefully do nothing.
|
|
echocheck "linking with -lrt"
|
|
_rt=no
|
|
cc_check "$_ld_pthread -lrt" && _rt=yes
|
|
if test "$_rt" = yes ; then
|
|
_ld_pthread="$_ld_pthread -lrt"
|
|
fi
|
|
echores "$_rt"
|
|
|
|
fi
|
|
|
|
echocheck "stream cache"
|
|
_stream_cache="$_pthreads"
|
|
if test "$_stream_cache" = yes ; then
|
|
def_stream_cache='#define HAVE_STREAM_CACHE 1'
|
|
else
|
|
def_stream_cache='#define HAVE_STREAM_CACHE 0'
|
|
fi
|
|
echores "$_stream_cache"
|
|
|
|
echocheck "rpath"
|
|
if test "$_rpath" = yes ; then
|
|
for I in $(echo $extra_ldflags | sed 's/-L//g') ; do
|
|
tmp="$tmp $(echo $I | sed 's/.*/ -L& -Wl,-R&/')"
|
|
done
|
|
extra_ldflags=$tmp
|
|
fi
|
|
echores "$_rpath"
|
|
|
|
echocheck "iconv"
|
|
if test "$_iconv" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <unistd.h>
|
|
#include <iconv.h>
|
|
#define INBUFSIZE 1024
|
|
#define OUTBUFSIZE 4096
|
|
|
|
char inbuffer[INBUFSIZE];
|
|
char outbuffer[OUTBUFSIZE];
|
|
|
|
int main(void) {
|
|
size_t numread;
|
|
iconv_t icdsc;
|
|
char *tocode="UTF-8";
|
|
char *fromcode="cp1250";
|
|
if ((icdsc = iconv_open(tocode, fromcode)) != (iconv_t)(-1)) {
|
|
while ((numread = read(0, inbuffer, INBUFSIZE))) {
|
|
char *iptr=inbuffer;
|
|
char *optr=outbuffer;
|
|
size_t inleft=numread;
|
|
size_t outleft=OUTBUFSIZE;
|
|
if (iconv(icdsc, &iptr, &inleft, &optr, &outleft)
|
|
!= (size_t)(-1)) {
|
|
write(1, outbuffer, OUTBUFSIZE - outleft);
|
|
}
|
|
}
|
|
if (iconv_close(icdsc) == -1)
|
|
;
|
|
}
|
|
return 0;
|
|
}
|
|
EOF
|
|
_iconv=no
|
|
for _ld_tmp in "" "-liconv" "-liconv $_ld_dl" ; do
|
|
cc_check $_ld_lm $_ld_tmp && libs_mplayer="$libs_mplayer $_ld_tmp" &&
|
|
_iconv=yes && break
|
|
done
|
|
if test "$_iconv" != yes ; then
|
|
die "Unable to find iconv which should be part of standard compilation environment. Aborting. If you really mean to compile without iconv support use --disable-iconv."
|
|
fi
|
|
fi
|
|
if test "$_iconv" = yes ; then
|
|
def_iconv='#define HAVE_ICONV 1'
|
|
else
|
|
def_iconv='#define HAVE_ICONV 0'
|
|
fi
|
|
echores "$_iconv"
|
|
|
|
|
|
echocheck "soundcard.h"
|
|
_soundcard_h=no
|
|
def_soundcard_h='#define HAVE_SOUNDCARD_H 0'
|
|
def_sys_soundcard_h='#define HAVE_SYS_SOUNDCARD_H 0'
|
|
for _soundcard_header in "sys/soundcard.h" "soundcard.h"; do
|
|
header_check $_soundcard_header && _soundcard_h=yes &&
|
|
res_comment="$_soundcard_header" && break
|
|
done
|
|
|
|
if test "$_soundcard_h" = yes ; then
|
|
if test $_soundcard_header = "sys/soundcard.h"; then
|
|
def_sys_soundcard_h='#define HAVE_SYS_SOUNDCARD_H 1'
|
|
else
|
|
def_soundcard_h='#define HAVE_SOUNDCARD_H 1'
|
|
fi
|
|
fi
|
|
echores "$_soundcard_h"
|
|
|
|
|
|
echocheck "sys/videoio.h"
|
|
sys_videoio_h=no
|
|
def_sys_videoio_h='#define HAVE_SYS_VIDEOIO_H 0'
|
|
header_check sys/videoio.h && sys_videoio_h=yes &&
|
|
def_sys_videoio_h='#define HAVE_SYS_VIDEOIO_H 1'
|
|
echores "$sys_videoio_h"
|
|
|
|
|
|
echocheck "terminfo"
|
|
if test "$_terminfo" = auto ; then
|
|
_terminfo=no
|
|
for _ld_tmp in "-lncurses" "-lncursesw"; do
|
|
statement_check term.h 'setupterm(0, 1, 0)' $_ld_tmp &&
|
|
libs_mplayer="$libs_mplayer $_ld_tmp" && _terminfo=yes && break
|
|
done
|
|
fi
|
|
if test "$_terminfo" = yes ; then
|
|
def_terminfo='#define HAVE_TERMINFO 1'
|
|
test $_ld_tmp && res_comment="using $_ld_tmp"
|
|
|
|
if test "$_termcap" = auto ; then
|
|
_termcap=yes # terminfo provides termcap
|
|
fi
|
|
else
|
|
def_terminfo='#define HAVE_TERMINFO 0'
|
|
fi
|
|
echores "$_terminfo"
|
|
|
|
|
|
echocheck "termcap"
|
|
if test "$_termcap" = auto ; then
|
|
_termcap=no
|
|
for _ld_tmp in "-lncurses" "-ltinfo" "-ltermcap"; do
|
|
statement_check term.h 'tgetent(0, 0)' $_ld_tmp &&
|
|
libs_mplayer="$libs_mplayer $_ld_tmp" && _termcap=yes && break
|
|
done
|
|
fi
|
|
if test "$_termcap" = yes ; then
|
|
def_termcap='#define HAVE_TERMCAP 1'
|
|
test $_ld_tmp && res_comment="using $_ld_tmp"
|
|
else
|
|
def_termcap='#define HAVE_TERMCAP 0'
|
|
fi
|
|
echores "$_termcap"
|
|
|
|
|
|
echocheck "termios"
|
|
def_termios='#define HAVE_TERMIOS 0'
|
|
def_termios_h='#define HAVE_TERMIOS_H 0'
|
|
def_termios_sys_h='#define HAVE_SYS_TERMIOS_H 0'
|
|
if test "$_termios" = auto ; then
|
|
_termios=no
|
|
for _termios_header in "termios.h" "sys/termios.h"; do
|
|
header_check $_termios_header && _termios=yes &&
|
|
res_comment="using $_termios_header" && break
|
|
done
|
|
fi
|
|
|
|
if test "$_termios" = yes ; then
|
|
def_termios='#define HAVE_TERMIOS 1'
|
|
if test "$_termios_header" = "termios.h" ; then
|
|
def_termios_h='#define HAVE_TERMIOS_H 1'
|
|
else
|
|
def_termios_sys_h='#define HAVE_SYS_TERMIOS_H 1'
|
|
fi
|
|
fi
|
|
echores "$_termios"
|
|
|
|
|
|
echocheck "shm"
|
|
if test "$_shm" = auto ; then
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/ipc.h>
|
|
#include <sys/shm.h>
|
|
int main(void) {
|
|
shmget(0, 0, 0);
|
|
shmat(0, 0, 0);
|
|
shmctl(0, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
_shm=no
|
|
cc_check && _shm=yes
|
|
fi
|
|
if test "$_shm" = yes ; then
|
|
def_shm='#define HAVE_SHM 1'
|
|
else
|
|
def_shm='#define HAVE_SHM 0'
|
|
fi
|
|
echores "$_shm"
|
|
|
|
|
|
echocheck "POSIX select()"
|
|
cat > $TMPC << EOF
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <sys/types.h>
|
|
#include <string.h>
|
|
#include <sys/time.h>
|
|
#include <unistd.h>
|
|
int main(void) {int nfds = 1; fd_set readfds; struct timeval timeout; select(nfds, &readfds, NULL, NULL, &timeout); return 0; }
|
|
EOF
|
|
_posix_select=no
|
|
def_posix_select='#define HAVE_POSIX_SELECT 0'
|
|
cc_check && _posix_select=yes &&
|
|
def_posix_select='#define HAVE_POSIX_SELECT 1'
|
|
echores "$_posix_select"
|
|
|
|
|
|
echocheck "audio select()"
|
|
if test "$_select" = no ; then
|
|
def_select='#define HAVE_AUDIO_SELECT 0'
|
|
elif test "$_select" = yes ; then
|
|
def_select='#define HAVE_AUDIO_SELECT 1'
|
|
fi
|
|
echores "$_select"
|
|
|
|
|
|
echocheck "glob()"
|
|
_glob=no
|
|
statement_check glob.h 'glob("filename", 0, 0, 0)' && _glob=yes
|
|
need_glob=no
|
|
if test "$_glob" = yes ; then
|
|
def_glob='#define HAVE_GLOB 1'
|
|
else
|
|
def_glob='#define HAVE_GLOB 0'
|
|
# HACK! need_glob currently enables compilation of a
|
|
# win32-specific glob()-replacement.
|
|
# Other OS neither need it nor can they use it (mf:// is disabled for them).
|
|
win32 && need_glob=yes
|
|
fi
|
|
echores "$_glob"
|
|
|
|
|
|
echocheck "setmode()"
|
|
_setmode=no
|
|
def_setmode='#define HAVE_SETMODE 0'
|
|
statement_check io.h 'setmode(0, 0)' && _setmode=yes && def_setmode='#define HAVE_SETMODE 1'
|
|
echores "$_setmode"
|
|
|
|
|
|
echocheck "sys/sysinfo.h"
|
|
_sys_sysinfo=no
|
|
statement_check sys/sysinfo.h 'struct sysinfo s_info; s_info.mem_unit=0; sysinfo(&s_info)' && _sys_sysinfo=yes
|
|
if test "$_sys_sysinfo" = yes ; then
|
|
def_sys_sysinfo_h='#define HAVE_SYS_SYSINFO_H 1'
|
|
else
|
|
def_sys_sysinfo_h='#define HAVE_SYS_SYSINFO_H 0'
|
|
fi
|
|
echores "$_sys_sysinfo"
|
|
|
|
|
|
echocheck "pkg-config"
|
|
if $($_pkg_config --version > /dev/null 2>&1); then
|
|
if test "$_ld_static"; then
|
|
_pkg_config="$_pkg_config --static"
|
|
fi
|
|
echores "yes"
|
|
else
|
|
_pkg_config=false
|
|
echores "no"
|
|
fi
|
|
|
|
|
|
echocheck "libguess support"
|
|
if test "$_libguess" = auto ; then
|
|
_libguess=no
|
|
if pkg_config_add 'libguess >= 1.0' ; then
|
|
_libguess=yes
|
|
fi
|
|
fi
|
|
if test "$_libguess" = yes; then
|
|
def_libguess="#define HAVE_LIBGUESS 1"
|
|
else
|
|
def_libguess="#define HAVE_LIBGUESS 0"
|
|
fi
|
|
echores "$_libguess"
|
|
|
|
|
|
echocheck "Samba support (libsmbclient)"
|
|
if test "$_smb" = auto ; then
|
|
_smb=no
|
|
if pkg_config_add 'smbclient >= 0.2.0' ; then
|
|
_smb=yes
|
|
fi
|
|
fi
|
|
if test "$_smb" = yes; then
|
|
def_smb="#define HAVE_LIBSMBCLIENT 1"
|
|
inputmodules="smb $inputmodules"
|
|
else
|
|
def_smb="#define HAVE_LIBSMBCLIENT 0"
|
|
noinputmodules="smb $noinputmodules"
|
|
fi
|
|
echores "$_smb"
|
|
|
|
|
|
echocheck "libquvi 0.4.x support"
|
|
if test "$_libquvi4" = auto ; then
|
|
_libquvi4=no
|
|
if pkg_config_add 'libquvi >= 0.4.1' ; then
|
|
_libquvi4=yes
|
|
fi
|
|
fi
|
|
echores "$_libquvi4"
|
|
|
|
echocheck "libquvi 0.9.x support"
|
|
if test "$_libquvi4" = yes ; then
|
|
_libquvi9=no
|
|
res_comment="using libquvi 0.4.x"
|
|
fi
|
|
if test "$_libquvi9" = auto ; then
|
|
_libquvi9=no
|
|
if pkg_config_add 'libquvi-0.9 >= 0.9.0' ; then
|
|
_libquvi9=yes
|
|
fi
|
|
fi
|
|
echores "$_libquvi9"
|
|
|
|
if test "$_libquvi9" = yes || test "$_libquvi4" = yes; then
|
|
def_libquvi9="#define HAVE_LIBQUVI 1"
|
|
else
|
|
def_libquvi9="#define HAVE_LIBQUVI 0"
|
|
fi
|
|
|
|
#########
|
|
# VIDEO #
|
|
#########
|
|
|
|
|
|
if darwin; then
|
|
|
|
echocheck "Cocoa"
|
|
if test "$_cocoa" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <CoreServices/CoreServices.h>
|
|
#include <OpenGL/OpenGL.h>
|
|
int main(void) {
|
|
NSApplicationLoad();
|
|
}
|
|
EOF
|
|
_cocoa=no
|
|
cc_check -framework IOKit -framework Cocoa -framework OpenGL && _cocoa=yes
|
|
fi
|
|
if test "$_cocoa" = yes ; then
|
|
libs_mplayer="$libs_mplayer -framework IOKit -framework Cocoa -framework OpenGL"
|
|
extra_ldflags="$extra_ldflags -fobjc-arc" # needed for OS X 10.7
|
|
def_cocoa='#define HAVE_COCOA 1'
|
|
else
|
|
def_cocoa='#define HAVE_COCOA 0'
|
|
fi
|
|
echores "$_cocoa"
|
|
|
|
echocheck "CoreVideo"
|
|
if test "$_cocoa" = yes && test "$_corevideo" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <QuartzCore/CoreVideo.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_corevideo=no
|
|
cc_check -framework Cocoa -framework QuartzCore -framework OpenGL && _corevideo=yes
|
|
fi
|
|
if test "$_corevideo" = yes ; then
|
|
vomodules="corevideo $vomodules"
|
|
libs_mplayer="$libs_mplayer -framework QuartzCore"
|
|
def_corevideo='#define HAVE_COREVIDEO 1'
|
|
else
|
|
novomodules="corevideo $novomodules"
|
|
def_corevideo='#define HAVE_COREVIDEO 0'
|
|
fi
|
|
echores "$_corevideo"
|
|
|
|
depends_on_application_services(){
|
|
test "$_corevideo" = yes
|
|
}
|
|
|
|
else
|
|
def_cocoa='#define HAVE_COCOA 0'
|
|
def_corevideo='#define HAVE_COREVIDEO 0'
|
|
fi #if darwin
|
|
|
|
_wlver="1.2.0"
|
|
echocheck "Wayland"
|
|
if test "$_wayland" = yes || test "$_wayland" = auto; then
|
|
_wayland="no"
|
|
pkg_config_add "wayland-client >= $_wlver wayland-cursor >= $_wlver xkbcommon >= 0.3.0" \
|
|
&& _wayland="yes"
|
|
fi
|
|
if test "$_wayland" = yes; then
|
|
res_comment=""
|
|
def_wayland='#define HAVE_WAYLAND 1'
|
|
vomodules="wayland $vomodules"
|
|
else
|
|
res_comment="version >= $_wlver"
|
|
def_wayland='#define HAVE_WAYLAND 0'
|
|
novomodules="wayland $novomodules"
|
|
fi
|
|
echores "$_wayland"
|
|
unset _wlver
|
|
|
|
echocheck "X11"
|
|
if test "$_x11" = auto ; then
|
|
_x11="no"
|
|
pkg_config_add "x11" && _x11="yes"
|
|
fi
|
|
if test "$_x11" = yes ; then
|
|
def_x11='#define HAVE_X11 1'
|
|
vomodules="x11 $vomodules"
|
|
else
|
|
_x11=no
|
|
def_x11='#define HAVE_X11 0'
|
|
novomodules="x11 $novomodules"
|
|
res_comment="check if the dev(el) packages are installed"
|
|
fi
|
|
echores "$_x11"
|
|
|
|
echocheck "Xss screensaver extensions"
|
|
if test "$_xss" = auto ; then
|
|
_xss=no
|
|
statement_check "X11/extensions/scrnsaver.h" 'XScreenSaverSuspend(NULL, True)' -lXss && _xss=yes
|
|
fi
|
|
if test "$_xss" = yes ; then
|
|
def_xss='#define HAVE_XSS 1'
|
|
libs_mplayer="$libs_mplayer -lXss"
|
|
else
|
|
def_xss='#define HAVE_XSS 0'
|
|
fi
|
|
echores "$_xss"
|
|
|
|
echocheck "X extensions"
|
|
_xext=no
|
|
if test "$_x11" = yes ; then
|
|
pkg_config_add "xext" && _xext="yes"
|
|
fi
|
|
if test "$_xext" = yes ; then
|
|
def_xext='#define HAVE_XEXT 1'
|
|
echores "yes"
|
|
else
|
|
def_xext='#define HAVE_XEXT 0'
|
|
echores "no"
|
|
fi
|
|
|
|
echocheck "Xv"
|
|
if test "$_xv" = auto && test "$_x11" = yes ; then
|
|
_xv=no
|
|
statement_check_broken X11/Xlib.h X11/extensions/Xvlib.h 'XvGetPortAttribute(0, 0, 0, 0)' -lXv && _xv=yes
|
|
fi
|
|
|
|
if test "$_xv" = yes ; then
|
|
def_xv='#define HAVE_XV 1'
|
|
libs_mplayer="$libs_mplayer -lXv"
|
|
vomodules="xv $vomodules"
|
|
else
|
|
def_xv='#define HAVE_XV 0'
|
|
novomodules="xv $novomodules"
|
|
fi
|
|
echores "$_xv"
|
|
|
|
|
|
echocheck "VDPAU"
|
|
if test "$_vdpau" = auto && test "$_x11" = yes ; then
|
|
_vdpau=no
|
|
if test "$_dl" = yes ; then
|
|
pkg_config_add 'vdpau >= 0.2' && _vdpau=yes
|
|
fi
|
|
fi
|
|
if test "$_vdpau" = yes ; then
|
|
def_vdpau='#define HAVE_VDPAU 1'
|
|
def_vdpau_hwaccel='#define HAVE_VDPAU_HWACCEL 1'
|
|
vomodules="vdpau $vomodules"
|
|
else
|
|
def_vdpau='#define HAVE_VDPAU 0'
|
|
def_vdpau_hwaccel='#define HAVE_VDPAU_HWACCEL 0'
|
|
novomodules="vdpau $novomodules"
|
|
fi
|
|
echores "$_vdpau"
|
|
|
|
|
|
echocheck "VAAPI"
|
|
_vaapi_vpp=no
|
|
def_vaapi_vpp='#define HAVE_VAAPI_VPP 0'
|
|
_vaapi_glx=no
|
|
def_vaapi_glx='#define HAVE_VAAPI_GLX 0'
|
|
if test "$_vaapi" = auto && test "$_x11" = yes ; then
|
|
_vaapi=no
|
|
if test "$_dl" = yes ; then
|
|
pkg_config_add 'libva >= 0.32.0 libva-x11 >= 0.32.0' && _vaapi=yes
|
|
fi
|
|
fi
|
|
if test "$_vaapi" = yes ; then
|
|
def_vaapi='#define HAVE_VAAPI 1'
|
|
def_vaapi_hwaccel='#define HAVE_VAAPI_HWACCEL 1'
|
|
vomodules="vaapi $vomodules"
|
|
else
|
|
def_vaapi='#define HAVE_VAAPI 0'
|
|
def_vaapi_hwaccel='#define HAVE_VAAPI_HWACCEL 0'
|
|
novomodules="vaapi $novomodules"
|
|
fi
|
|
echores "$_vaapi"
|
|
|
|
if test "$_vaapi" = yes ; then
|
|
echocheck "VAAPI VPP"
|
|
if pkg-config 'libva >= 0.34.0' ; then
|
|
_vaapi_vpp=yes
|
|
def_vaapi_vpp='#define HAVE_VAAPI_VPP 1'
|
|
fi
|
|
echores "$_vaapi_glx"
|
|
echocheck "VAAPI GLX"
|
|
if pkg_config_add 'libva-glx >= 0.32.0' ; then
|
|
_vaapi_glx=yes
|
|
def_vaapi_glx='#define HAVE_VAAPI_GLX 1'
|
|
fi
|
|
echores "$_vaapi_glx"
|
|
fi
|
|
|
|
|
|
echocheck "Xinerama"
|
|
if test "$_xinerama" = auto && test "$_x11" = yes ; then
|
|
_xinerama=no
|
|
statement_check X11/extensions/Xinerama.h 'XineramaIsActive(0)' -lXinerama && _xinerama=yes
|
|
fi
|
|
|
|
if test "$_xinerama" = yes ; then
|
|
def_xinerama='#define HAVE_XINERAMA 1'
|
|
libs_mplayer="$libs_mplayer -lXinerama"
|
|
else
|
|
def_xinerama='#define HAVE_XINERAMA 0'
|
|
fi
|
|
echores "$_xinerama"
|
|
|
|
|
|
# Note: the -lXxf86vm library is the VideoMode extension and though it's not
|
|
# needed for DGA, AFAIK every distribution packages together with DGA stuffs
|
|
# named 'X extensions' or something similar.
|
|
# This check may be useful for future mplayer versions (to change resolution)
|
|
# If you run into problems, remove '-lXxf86vm'.
|
|
echocheck "Xxf86vm"
|
|
if test "$_vm" = auto && test "$_x11" = yes ; then
|
|
_vm=no
|
|
statement_check_broken X11/Xlib.h X11/extensions/xf86vmode.h 'XF86VidModeQueryExtension(0, 0, 0)' -lXxf86vm && _vm=yes
|
|
fi
|
|
if test "$_vm" = yes ; then
|
|
def_vm='#define HAVE_XF86VM 1'
|
|
libs_mplayer="$libs_mplayer -lXxf86vm"
|
|
else
|
|
def_vm='#define HAVE_XF86VM 0'
|
|
fi
|
|
echores "$_vm"
|
|
|
|
# Check for the presence of special keycodes, like audio control buttons
|
|
# that XFree86 might have. Used to be bundled with the xf86vm check, but
|
|
# has nothing to do with xf86vm and XFree 3.x has xf86vm but does NOT
|
|
# have these new keycodes.
|
|
echocheck "XF86keysym"
|
|
if test "$_xf86keysym" = auto && test "$_x11" = yes ; then
|
|
_xf86keysym=no
|
|
return_check X11/XF86keysym.h XF86XK_AudioPause && _xf86keysym=yes
|
|
fi
|
|
if test "$_xf86keysym" = yes ; then
|
|
def_xf86keysym='#define HAVE_XF86XK 1'
|
|
else
|
|
def_xf86keysym='#define HAVE_XF86XK 0'
|
|
fi
|
|
echores "$_xf86keysym"
|
|
|
|
|
|
echocheck "CACA"
|
|
if test "$_caca" = auto ; then
|
|
_caca=no
|
|
pkg_config_add 'caca >= 0.99.beta18' && _caca=yes
|
|
fi
|
|
if test "$_caca" = yes ; then
|
|
def_caca='#define HAVE_CACA 1'
|
|
vomodules="caca $vomodules"
|
|
else
|
|
def_caca='#define HAVE_CACA 0'
|
|
novomodules="caca $novomodules"
|
|
fi
|
|
echores "$_caca"
|
|
|
|
|
|
echocheck "DVB"
|
|
if test "$_dvb" = auto ; then
|
|
_dvb=no
|
|
cat >$TMPC << EOF
|
|
#include <poll.h>
|
|
#include <sys/ioctl.h>
|
|
#include <stdio.h>
|
|
#include <time.h>
|
|
#include <unistd.h>
|
|
#include <linux/dvb/dmx.h>
|
|
#include <linux/dvb/frontend.h>
|
|
#include <linux/dvb/video.h>
|
|
#include <linux/dvb/audio.h>
|
|
int main(void) {return 0;}
|
|
EOF
|
|
for _inc_tmp in "" "-I/usr/src/DVB/include" ; do
|
|
cc_check $_inc_tmp && _dvb=yes &&
|
|
extra_cflags="$extra_cflags $_inc_tmp" && break
|
|
done
|
|
fi
|
|
echores "$_dvb"
|
|
if test "$_dvb" = yes ; then
|
|
_dvbin=yes
|
|
inputmodules="dvb $inputmodules"
|
|
def_dvb='#define HAVE_DVB 1'
|
|
def_dvbin='#define HAVE_DVBIN 1'
|
|
else
|
|
_dvbin=no
|
|
noinputmodules="dvb $noinputmodules"
|
|
def_dvb='#define HAVE_DVB 0'
|
|
def_dvbin='#define HAVE_DVBIN 0 '
|
|
fi
|
|
|
|
|
|
echocheck "JPEG support"
|
|
if test "$_jpeg" = auto ; then
|
|
_jpeg=no
|
|
header_check_broken stdio.h jpeglib.h -ljpeg $_ld_lm && _jpeg=yes
|
|
fi
|
|
echores "$_jpeg"
|
|
|
|
if test "$_jpeg" = yes ; then
|
|
def_jpeg='#define HAVE_JPEG 1'
|
|
libs_mplayer="$libs_mplayer -ljpeg"
|
|
else
|
|
def_jpeg='#define HAVE_JPEG 0'
|
|
fi
|
|
|
|
|
|
#################
|
|
# VIDEO + AUDIO #
|
|
#################
|
|
|
|
|
|
# make sure this stays below CoreVideo to avoid issues due to namespace
|
|
# conflicts between -lGL and -framework OpenGL
|
|
echocheck "OpenGL"
|
|
#Note: this test is run even with --enable-gl since we autodetect linker flags
|
|
if (test "$_x11" = yes || test "$_wayland" = yes || test "$_cocoa" = yes || win32) && test "$_gl" != no ; then
|
|
cat > $TMPC << EOF
|
|
#ifdef GL_WIN32
|
|
#include <windows.h>
|
|
#elif defined(GL_WAYLAND)
|
|
#include <EGL/egl.h>
|
|
#else
|
|
#include <X11/Xlib.h>
|
|
#include <GL/glx.h>
|
|
#endif
|
|
#include <GL/gl.h>
|
|
int main(int argc, char *argv[]) {
|
|
#ifdef GL_WIN32
|
|
HDC dc;
|
|
wglCreateContext(dc);
|
|
#elif defined(GL_WAYLAND)
|
|
eglCreateContext(NULL, NULL, EGL_NO_CONTEXT, NULL);
|
|
#else
|
|
glXCreateContext(NULL, NULL, NULL, True);
|
|
#endif
|
|
glFinish();
|
|
return 0;
|
|
}
|
|
EOF
|
|
_gl=no
|
|
if test "$_x11" = yes ; then
|
|
for _ld_tmp in "" -lGL "-lGL -lXdamage" "-lGL $_ld_pthread" ; do
|
|
if cc_check $_ld_tmp $_ld_lm ; then
|
|
_gl=yes
|
|
_gl_x11=yes
|
|
libs_mplayer="$libs_mplayer $_ld_tmp $_ld_dl"
|
|
break
|
|
fi
|
|
done
|
|
fi
|
|
if test "$_wayland" = yes && cc_check -DGL_WAYLAND -lGL -lEGL &&
|
|
pkg_config_add "wayland-egl >= 9.0.0"; then
|
|
_gl=yes
|
|
_gl_wayland=yes
|
|
libs_mplayer="$libs_mplayer -lGL -lEGL"
|
|
fi
|
|
if win32 && cc_check -DGL_WIN32 -lopengl32 ; then
|
|
_gl=yes
|
|
_gl_win32=yes
|
|
libs_mplayer="$libs_mplayer -lopengl32 -lgdi32"
|
|
fi
|
|
if test "$_cocoa" = yes ; then
|
|
_gl=yes
|
|
_gl_cocoa=yes
|
|
fi
|
|
|
|
cat > $TMPC << EOF
|
|
#ifdef __APPLE__
|
|
#include <OpenGL/gl.h>
|
|
#include <OpenGL/glext.h>
|
|
#else
|
|
#include <GL/gl.h>
|
|
#include <GL/glext.h>
|
|
#endif
|
|
int main(int argc, char *argv[]) {
|
|
return !GL_INVALID_FRAMEBUFFER_OPERATION;
|
|
}
|
|
EOF
|
|
if ! cc_check; then
|
|
_gl=no
|
|
_gl_x11=no
|
|
_gl_wayland=no
|
|
_gl_win32=no
|
|
_gl_cocoa=no
|
|
res_comment="missing glext.h, get from http://www.opengl.org/registry/api/glext.h"
|
|
fi
|
|
else
|
|
_gl=no
|
|
fi
|
|
|
|
def_gl_cocoa='#define HAVE_GL_COCOA 0'
|
|
def_gl_win32='#define HAVE_GL_WIN32 0'
|
|
def_gl_x11='#define HAVE_GL_X11 0'
|
|
def_gl_wayland='#define HAVE_GL_WAYLAND 0'
|
|
|
|
if test "$_gl" = yes ; then
|
|
def_gl='#define HAVE_GL 1'
|
|
res_comment="backends:"
|
|
if test "$_gl_cocoa" = yes ; then
|
|
def_gl_cocoa='#define HAVE_GL_COCOA 1'
|
|
res_comment="$res_comment cocoa"
|
|
fi
|
|
if test "$_gl_win32" = yes ; then
|
|
def_gl_win32='#define HAVE_GL_WIN32 1'
|
|
res_comment="$res_comment win32"
|
|
fi
|
|
if test "$_gl_x11" = yes ; then
|
|
def_gl_x11='#define HAVE_GL_X11 1'
|
|
res_comment="$res_comment x11"
|
|
fi
|
|
if test "$_gl_wayland" = yes ; then
|
|
def_gl_wayland='#define HAVE_GL_WAYLAND 1'
|
|
res_comment="$res_comment wayland"
|
|
fi
|
|
vomodules="opengl $vomodules"
|
|
else
|
|
def_gl='#define HAVE_GL 0'
|
|
novomodules="opengl $novomodules"
|
|
fi
|
|
echores "$_gl"
|
|
|
|
|
|
echocheck "VDPAU with OpenGL/X11"
|
|
if test "$_gl_x11" = yes && test "$_vdpau" = yes ; then
|
|
def_vdpau_gl_x11='#define HAVE_VDPAU_GL_X11 1'
|
|
_vdpau_gl_x11=yes
|
|
else
|
|
def_vdpau_gl_x11='#define HAVE_VDPAU_GL_X11 0'
|
|
_vdpau_gl_x11=no
|
|
fi
|
|
echores "$_vdpau_gl_x11"
|
|
|
|
|
|
if win32; then
|
|
|
|
|
|
echocheck "Direct3D"
|
|
if test "$_direct3d" = auto ; then
|
|
_direct3d=no
|
|
header_check d3d9.h && _direct3d=yes
|
|
fi
|
|
if test "$_direct3d" = yes ; then
|
|
def_direct3d='#define HAVE_DIRECT3D 1'
|
|
vomodules="direct3d $vomodules"
|
|
else
|
|
def_direct3d='#define HAVE_DIRECT3D 0'
|
|
novomodules="direct3d $novomodules"
|
|
fi
|
|
echores "$_direct3d"
|
|
|
|
|
|
echocheck "DirectSound"
|
|
if test "$_dsound" = auto ; then
|
|
_dsound=no
|
|
header_check dsound.h && _dsound=yes
|
|
fi
|
|
if test "$_dsound" = yes ; then
|
|
def_dsound='#define HAVE_DSOUND 1'
|
|
aomodules="dsound $aomodules"
|
|
else
|
|
def_dsound='#define HAVE_DSOUND 0'
|
|
noaomodules="dsound $noaomodules"
|
|
fi
|
|
echores "$_dsound"
|
|
|
|
echocheck "WASAPI"
|
|
if test "$_wasapi" = auto ; then
|
|
_wasapi=no
|
|
|
|
cat > $TMPC << EOF
|
|
#define COBJMACROS 1
|
|
#define _WIN32_WINNT 0x600
|
|
#include <malloc.h>
|
|
#include <stdlib.h>
|
|
#include <process.h>
|
|
#include <initguid.h>
|
|
#include <audioclient.h>
|
|
#include <endpointvolume.h>
|
|
#include <mmdeviceapi.h>
|
|
#include <avrt.h>
|
|
const GUID *check1[] = {
|
|
&IID_IAudioClient,
|
|
&IID_IAudioRenderClient,
|
|
&IID_IAudioClient,
|
|
&IID_IAudioEndpointVolume,
|
|
};
|
|
int main(void) {
|
|
return 0;
|
|
}
|
|
EOF
|
|
|
|
if cc_check "-lole32"; then
|
|
_wasapi="yes"
|
|
fi
|
|
|
|
fi
|
|
if test "$_wasapi" = yes ; then
|
|
def_wasapi='#define HAVE_WASAPI 1'
|
|
aomodules="wasapi $aomodules"
|
|
libs_mplayer="$libs_mplayer -lole32"
|
|
else
|
|
def_wasapi='#define HAVE_WASAPI 0'
|
|
noaomodules="wasapi $noaomodules"
|
|
fi
|
|
echores "$_wasapi"
|
|
|
|
else
|
|
def_direct3d='#define HAVE_DIRECT3D 0'
|
|
def_dsound='#define HAVE_DSOUND 0'
|
|
def_wasapi='#define HAVE_WASAPI 0'
|
|
fi #if win32; then
|
|
|
|
|
|
echocheck "SDL 2.0"
|
|
if test "$_sdl2" = yes ; then
|
|
pkg_config_add 'sdl2' && _sdl2=yes
|
|
fi
|
|
if test "$_sdl2" = yes ; then
|
|
_sdl=yes # sdl2 implies sdl
|
|
def_sdl='#define HAVE_SDL1 1'
|
|
def_sdl2='#define HAVE_SDL2 1'
|
|
vomodules="sdl $vomodules"
|
|
aomodules="sdl $aomodules"
|
|
echores "$_sdl2"
|
|
else
|
|
def_sdl2='#define HAVE_SDL2 0'
|
|
echores "$_sdl2"
|
|
echocheck "SDL"
|
|
if test "$_sdl" = yes ; then
|
|
pkg_config_add 'sdl' && _sdl=yes
|
|
fi
|
|
if test "$_sdl" = yes ; then
|
|
def_sdl='#define HAVE_SDL1 1'
|
|
novomodules="sdl $novomodules"
|
|
aomodules="sdl $aomodules"
|
|
else
|
|
def_sdl='#define HAVE_SDL1 0'
|
|
novomodules="sdl $novomodules"
|
|
noaomodules="sdl $noaomodules"
|
|
fi
|
|
echores "$_sdl"
|
|
fi
|
|
|
|
|
|
|
|
|
|
#########
|
|
# AUDIO #
|
|
#########
|
|
|
|
|
|
echocheck "OSS Audio"
|
|
if test "$_ossaudio" = auto ; then
|
|
_ossaudio=no
|
|
return_check $_soundcard_header SNDCTL_DSP_SETFRAGMENT && _ossaudio=yes
|
|
fi
|
|
if test "$_ossaudio" = yes ; then
|
|
def_ossaudio='#define HAVE_OSS_AUDIO 1'
|
|
aomodules="oss $aomodules"
|
|
cat > $TMPC << EOF
|
|
#include <$_soundcard_header>
|
|
#ifdef OPEN_SOUND_SYSTEM
|
|
int main(void) { return 0; }
|
|
#else
|
|
#error Not the real thing
|
|
#endif
|
|
EOF
|
|
_real_ossaudio=no
|
|
cc_check && _real_ossaudio=yes
|
|
if test "$_real_ossaudio" = yes; then
|
|
def_ossaudio_devdsp='#define PATH_DEV_DSP "/dev/dsp"'
|
|
# Check for OSS4 headers (override default headers)
|
|
# Does not apply to systems where OSS4 is native (e.g. FreeBSD)
|
|
if test -f /etc/oss.conf; then
|
|
. /etc/oss.conf
|
|
_ossinc="$OSSLIBDIR/include"
|
|
if test -f "$_ossinc/sys/soundcard.h"; then
|
|
extra_cflags="-I$_ossinc $extra_cflags"
|
|
fi
|
|
fi
|
|
elif netbsd || openbsd ; then
|
|
def_ossaudio_devdsp='#define PATH_DEV_DSP "/dev/sound"'
|
|
libs_mplayer="$libs_mplayer -lossaudio"
|
|
else
|
|
def_ossaudio_devdsp='#define PATH_DEV_DSP "/dev/dsp"'
|
|
fi
|
|
def_ossaudio_devmixer='#define PATH_DEV_MIXER "/dev/mixer"'
|
|
else
|
|
def_ossaudio='#define HAVE_OSS_AUDIO 0'
|
|
def_ossaudio_devdsp='#define PATH_DEV_DSP ""'
|
|
def_ossaudio_devmixer='#define PATH_DEV_MIXER ""'
|
|
noaomodules="oss $noaomodules"
|
|
fi
|
|
echores "$_ossaudio"
|
|
|
|
|
|
echocheck "RSound"
|
|
if test "$_rsound" = auto ; then
|
|
_rsound=no
|
|
statement_check rsound.h 'rsd_init(NULL);' -lrsound && _rsound=yes
|
|
fi
|
|
echores "$_rsound"
|
|
|
|
if test "$_rsound" = yes ; then
|
|
def_rsound='#define HAVE_RSOUND 1'
|
|
aomodules="rsound $aomodules"
|
|
libs_mplayer="$libs_mplayer -lrsound"
|
|
else
|
|
def_rsound='#define HAVE_RSOUND 0'
|
|
noaomodules="rsound $noaomodules"
|
|
fi
|
|
|
|
|
|
echocheck "sndio"
|
|
if test "$_sndio" = auto ; then
|
|
_sndio=no
|
|
statement_check sndio.h 'struct sio_par par; sio_initpar(&par); const char *s = SIO_DEVANY' -lsndio && _sndio=yes
|
|
fi
|
|
echores "$_sndio"
|
|
|
|
if test "$_sndio" = yes ; then
|
|
def_sndio='#define HAVE_SNDIO 1'
|
|
aomodules="sndio $_aomodules"
|
|
libs_mplayer="$libs_mplayer -lsndio"
|
|
else
|
|
def_sndio='#define HAVE_SNDIO 0'
|
|
noaomodules="sndio $_noaomodules"
|
|
fi
|
|
|
|
|
|
echocheck "pulse"
|
|
if test "$_pulse" = auto ; then
|
|
_pulse=no
|
|
if pkg_config_add 'libpulse >= 0.9' ; then
|
|
_pulse=yes
|
|
fi
|
|
fi
|
|
echores "$_pulse"
|
|
|
|
if test "$_pulse" = yes ; then
|
|
def_pulse='#define HAVE_PULSE 1'
|
|
aomodules="pulse $aomodules"
|
|
else
|
|
def_pulse='#define HAVE_PULSE 0'
|
|
noaomodules="pulse $noaomodules"
|
|
fi
|
|
|
|
|
|
echocheck "PortAudio"
|
|
if test "$_portaudio" = auto && test "$_pthreads" != yes ; then
|
|
_portaudio=no
|
|
res_comment="pthreads not enabled"
|
|
fi
|
|
if test "$_portaudio" = auto ; then
|
|
_portaudio=no
|
|
if pkg_config_add 'portaudio-2.0 >= 19' ; then
|
|
_portaudio=yes
|
|
fi
|
|
fi
|
|
echores "$_portaudio"
|
|
|
|
if test "$_portaudio" = yes ; then
|
|
def_portaudio='#define HAVE_PORTAUDIO 1'
|
|
aomodules="portaudio $aomodules"
|
|
else
|
|
def_portaudio='#define HAVE_PORTAUDIO 0'
|
|
noaomodules="portaudio $noaomodules"
|
|
fi
|
|
|
|
|
|
echocheck "JACK"
|
|
if test "$_jack" = auto ; then
|
|
_jack=no
|
|
if pkg_config_add jack ; then
|
|
_jack=yes
|
|
fi
|
|
fi
|
|
|
|
if test "$_jack" = yes ; then
|
|
def_jack='#define HAVE_JACK 1'
|
|
aomodules="jack $aomodules"
|
|
else
|
|
def_jack='#define HAVE_JACK 0'
|
|
noaomodules="jack $noaomodules"
|
|
fi
|
|
echores "$_jack"
|
|
|
|
|
|
echocheck "OpenAL"
|
|
if test "$_openal" = auto ; then
|
|
_openal=no
|
|
if pkg_config_add 'openal >= 1.13' ; then
|
|
_openal=yes
|
|
fi
|
|
fi
|
|
echores "$_openal"
|
|
|
|
if test "$_openal" = yes ; then
|
|
def_openal='#define HAVE_OPENAL 1'
|
|
aomodules="openal $aomodules"
|
|
else
|
|
def_openal='#define HAVE_OPENAL 0'
|
|
noaomodules="openal $noaomodules"
|
|
fi
|
|
|
|
|
|
echocheck "ALSA audio"
|
|
if test "$_alsa" = auto ; then
|
|
_alsa=no
|
|
if pkg_config_add "alsa >= 1.0.9" ; then
|
|
_alsa=yes
|
|
fi
|
|
fi
|
|
def_alsa='#define HAVE_ALSA 0'
|
|
if test "$_alsa" = yes ; then
|
|
aomodules="alsa $aomodules"
|
|
def_alsa='#define HAVE_ALSA 1'
|
|
else
|
|
noaomodules="alsa $noaomodules"
|
|
fi
|
|
echores "$_alsa"
|
|
|
|
|
|
def_coreaudio='#define HAVE_COREAUDIO 0'
|
|
if darwin; then
|
|
echocheck "CoreAudio"
|
|
if test "$_coreaudio" = auto ; then
|
|
cat > $TMPC <<EOF
|
|
#include <CoreAudio/CoreAudio.h>
|
|
#include <AudioToolbox/AudioToolbox.h>
|
|
#include <AudioUnit/AudioUnit.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
_coreaudio=no
|
|
cc_check -framework CoreAudio -framework AudioUnit -framework AudioToolbox && _coreaudio=yes
|
|
fi
|
|
if test "$_coreaudio" = yes ; then
|
|
libs_mplayer="$libs_mplayer -framework CoreAudio -framework AudioUnit -framework AudioToolbox"
|
|
def_coreaudio='#define HAVE_COREAUDIO 1'
|
|
aomodules="coreaudio $aomodules"
|
|
else
|
|
def_coreaudio='#define HAVE_COREAUDIO 0'
|
|
noaomodules="coreaudio $noaomodules"
|
|
fi
|
|
echores $_coreaudio
|
|
fi #if darwin
|
|
|
|
|
|
# set default CD/DVD devices
|
|
if win32 ; then
|
|
default_cdrom_device="D:"
|
|
elif darwin ; then
|
|
default_cdrom_device="/dev/disk1"
|
|
elif dragonfly ; then
|
|
default_cdrom_device="/dev/cd0"
|
|
elif freebsd ; then
|
|
default_cdrom_device="/dev/cd0"
|
|
elif openbsd ; then
|
|
default_cdrom_device="/dev/rcd0c"
|
|
else
|
|
default_cdrom_device="/dev/cdrom"
|
|
fi
|
|
|
|
if win32 || dragonfly || freebsd || openbsd ; then
|
|
default_dvd_device=$default_cdrom_device
|
|
elif darwin ; then
|
|
default_dvd_device="/dev/rdiskN"
|
|
else
|
|
default_dvd_device="/dev/dvd"
|
|
fi
|
|
|
|
|
|
echocheck "VCD support"
|
|
if test "$_vcd" = auto; then
|
|
_vcd=no
|
|
if linux || freebsd || netbsd || openbsd || dragonfly || darwin ; then
|
|
_vcd=yes
|
|
elif mingw32; then
|
|
header_check_broken windows.h ntddcdrm.h && _vcd=yes
|
|
fi
|
|
fi
|
|
if test "$_vcd" = yes; then
|
|
inputmodules="vcd $inputmodules"
|
|
def_vcd='#define HAVE_VCD 1'
|
|
else
|
|
def_vcd='#define HAVE_VCD 0'
|
|
noinputmodules="vcd $noinputmodules"
|
|
res_comment="not supported on this OS"
|
|
fi
|
|
echores "$_vcd"
|
|
|
|
|
|
|
|
echocheck "Blu-ray support"
|
|
if test "$_bluray" = auto ; then
|
|
_bluray=no
|
|
pkg_config_add 'libbluray >= 0.2.1' && _bluray=yes
|
|
fi
|
|
if test "$_bluray" = yes ; then
|
|
def_bluray='#define HAVE_LIBBLURAY 1'
|
|
inputmodules="bluray $inputmodules"
|
|
else
|
|
def_bluray='#define HAVE_LIBBLURAY 0'
|
|
noinputmodules="bluray $noinputmodules"
|
|
fi
|
|
echores "$_bluray"
|
|
|
|
|
|
echocheck "dvdread"
|
|
if test "$_dvdread" = auto ; then
|
|
_dvdread=no
|
|
pkg_config_add 'dvdread >= 4.1.0' && _dvdread=yes
|
|
fi
|
|
if test "$_dvdread" = yes ; then
|
|
def_dvdread='#define HAVE_DVDREAD 1'
|
|
inputmodules="dvdread $inputmodules"
|
|
else
|
|
def_dvdread='#define HAVE_DVDREAD 0'
|
|
noinputmodules="dvdread $noinputmodules"
|
|
fi
|
|
echores "$_dvdread"
|
|
|
|
|
|
echocheck "dvdnav"
|
|
if test "$_dvdnav" = auto ; then
|
|
_dvdnav=no
|
|
pkg_config_add 'dvdnav >= 4.2.0' && _dvdnav=yes
|
|
fi
|
|
if test "$_dvdnav" = yes ; then
|
|
def_dvdnav='#define HAVE_DVDNAV 1'
|
|
inputmodules="dvdnav $inputmodules"
|
|
else
|
|
def_dvdnav='#define HAVE_DVDNAV 0'
|
|
noinputmodules="dvdnav $noinputmodules"
|
|
fi
|
|
echores "$_dvdnav"
|
|
|
|
|
|
echocheck "libcdio"
|
|
if test "$_libcdio" = auto ; then
|
|
_libcdio=no
|
|
if pkg_config_add 'libcdio_paranoia' ; then
|
|
_libcdio=yes
|
|
fi
|
|
fi
|
|
if test "$_libcdio" = yes ; then
|
|
_cdda='yes'
|
|
def_cdda='#define HAVE_CDDA 1'
|
|
inputmodules="cdda $inputmodules"
|
|
else
|
|
_libcdio=no
|
|
_cdda='no'
|
|
def_cdda='#define HAVE_CDDA 0'
|
|
noinputmodules="cdda $noinputmodules"
|
|
fi
|
|
echores "$_libcdio"
|
|
|
|
|
|
echocheck "SSA/ASS support"
|
|
if test "$_ass" = auto ; then
|
|
if pkg_config_add libass ; then
|
|
_ass=yes
|
|
def_ass='#define HAVE_LIBASS 1'
|
|
else
|
|
die "Unable to find development files for libass. Aborting. If you really mean to compile without libass support use --disable-libass."
|
|
fi
|
|
else
|
|
def_ass='#define HAVE_LIBASS 0'
|
|
fi
|
|
echores "$_ass"
|
|
|
|
|
|
echocheck "libass OSD support"
|
|
_dummy_osd=yes
|
|
if test "$_libass_osd" = auto ; then
|
|
_libass_osd=no
|
|
if test "$_ass" = yes ; then
|
|
_libass_osd=yes
|
|
_dummy_osd=no
|
|
fi
|
|
fi
|
|
echores "$_libass_osd"
|
|
|
|
|
|
echocheck "ENCA"
|
|
if test "$_enca" = auto ; then
|
|
_enca=no
|
|
statement_check enca.h 'enca_get_languages(NULL)' -lenca $_ld_lm && _enca=yes
|
|
fi
|
|
if test "$_enca" = yes ; then
|
|
def_enca='#define HAVE_ENCA 1'
|
|
libs_mplayer="$libs_mplayer -lenca"
|
|
else
|
|
def_enca='#define HAVE_ENCA 0'
|
|
fi
|
|
echores "$_enca"
|
|
|
|
|
|
echocheck "zlib"
|
|
_zlib=no
|
|
statement_check zlib.h 'inflate(0, Z_NO_FLUSH)' -lz && _zlib=yes
|
|
if test "$_zlib" = yes ; then
|
|
def_zlib='#define HAVE_ZLIB 1'
|
|
libs_mplayer="$libs_mplayer -lz"
|
|
else
|
|
die "Unable to find development files for zlib."
|
|
fi
|
|
echores "$_zlib"
|
|
|
|
|
|
echocheck "mpg123 support"
|
|
def_mpg123='#define HAVE_MPG123 0'
|
|
if test "$_mpg123" = auto; then
|
|
_mpg123=no
|
|
pkg_config_add 'libmpg123 >= 1.14.0' && _mpg123=yes
|
|
fi
|
|
if test "$_mpg123" = yes ; then
|
|
def_mpg123='#define HAVE_MPG123 1'
|
|
codecmodules="mpg123 $codecmodules"
|
|
else
|
|
nocodecmodules="mpg123 $nocodecmodules"
|
|
fi
|
|
echores "$_mpg123"
|
|
|
|
|
|
echocheck "LADSPA plugin support"
|
|
if test "$_ladspa" = auto ; then
|
|
_ladspa=no
|
|
if test "$_dl" = yes ; then
|
|
statement_check ladspa.h 'LADSPA_Descriptor ld = {0}' && _ladspa=yes
|
|
fi
|
|
fi
|
|
if test "$_ladspa" = yes; then
|
|
def_ladspa="#define HAVE_LADSPA 1"
|
|
else
|
|
def_ladspa="#define HAVE_LADSPA 0"
|
|
fi
|
|
echores "$_ladspa"
|
|
|
|
|
|
echocheck "libbs2b audio filter support"
|
|
if test "$_libbs2b" = auto ; then
|
|
_libbs2b=no
|
|
if pkg_config_add libbs2b ; then
|
|
_libbs2b=yes
|
|
fi
|
|
fi
|
|
def_libbs2b="#define HAVE_LIBBS2B 0"
|
|
test "$_libbs2b" = yes && def_libbs2b="#define HAVE_LIBBS2B 1"
|
|
echores "$_libbs2b"
|
|
|
|
|
|
echocheck "LCMS2 support"
|
|
if test "$_lcms2" = auto ; then
|
|
_lcms2=no
|
|
if pkg_config_add lcms2 ; then
|
|
_lcms2=yes
|
|
fi
|
|
fi
|
|
if test "$_lcms2" = yes; then
|
|
def_lcms2="#define HAVE_LCMS2 1"
|
|
else
|
|
def_lcms2="#define HAVE_LCMS2 0"
|
|
fi
|
|
echores "$_lcms2"
|
|
|
|
|
|
echocheck "VapurSynth support"
|
|
if test "$_vapoursynth" = auto ; then
|
|
_vapoursynth=no
|
|
if pkg_config_add 'vapoursynth >= 23 vapoursynth-script >= 23' ; then
|
|
_vapoursynth=yes
|
|
fi
|
|
fi
|
|
if test "$_vapoursynth" = yes ; then
|
|
def_vapoursynth="#define HAVE_VAPOURSYNTH 1"
|
|
else
|
|
def_vapoursynth="#define HAVE_VAPOURSYNTH 0"
|
|
fi
|
|
echores "$_vapoursynth"
|
|
|
|
|
|
all_libav_libs="libavutil >= 52.48.101:libavcodec >= 55.34.1:libavformat >= 55.12.0:libswscale >= 2.1.2"
|
|
echocheck "Libav ($all_libav_libs)"
|
|
if test "$ffmpeg" = auto ; then
|
|
IFS=":" # shell should not be used for programming
|
|
if ! pkg_config_add $all_libav_libs ; then
|
|
die "Unable to find development files for some of the required Libav libraries above. Aborting."
|
|
fi
|
|
fi
|
|
echores "yes"
|
|
|
|
|
|
_resampler=no
|
|
_avresample=no
|
|
|
|
|
|
def_libswresample='#define HAVE_LIBSWRESAMPLE 0'
|
|
def_libavresample='#define HAVE_LIBAVRESAMPLE 0'
|
|
|
|
echocheck "libavresample >= 1.1.0"
|
|
if test "$_disable_avresample" = no ; then
|
|
if pkg_config_add "libavresample >= 1.1.0" ; then
|
|
_resampler=yes
|
|
_avresample=yes
|
|
def_libavresample='#define HAVE_LIBAVRESAMPLE 1'
|
|
fi
|
|
fi
|
|
echores "$_resampler"
|
|
|
|
|
|
if test "$_resampler" = no ; then
|
|
echocheck "libswresample >= 0.17.104"
|
|
if pkg_config_add "libswresample >= 0.17.104" ; then
|
|
_resampler=yes
|
|
def_libswresample='#define HAVE_LIBSWRESAMPLE 1'
|
|
fi
|
|
echores "$_resampler"
|
|
fi
|
|
|
|
|
|
if test "$_resampler" = no ; then
|
|
die "No resampler found. Install libavresample or libswresample (FFmpeg)."
|
|
fi
|
|
|
|
|
|
echocheck "libavcodec avcodec_enum_to_chroma_pos API"
|
|
_avcodec_has_chroma_pos_api=no
|
|
statement_check libavcodec/avcodec.h 'int x, y; avcodec_enum_to_chroma_pos(&x, &y, AVCHROMA_LOC_UNSPECIFIED)' && _avcodec_has_chroma_pos_api=yes
|
|
if test "$_avcodec_has_chroma_pos_api" = yes ; then
|
|
def_avcodec_has_chroma_pos_api='#define HAVE_AVCODEC_CHROMA_POS_API 1'
|
|
else
|
|
def_avcodec_has_chroma_pos_api='#define HAVE_AVCODEC_CHROMA_POS_API 0'
|
|
fi
|
|
echores "$_avcodec_has_chroma_pos_api"
|
|
|
|
|
|
echocheck "libavcodec metadata update side data"
|
|
_avcodec_has_metadata_update_side_data=no
|
|
statement_check libavcodec/avcodec.h 'enum AVPacketSideDataType type = AV_PKT_DATA_METADATA_UPDATE' && _avcodec_has_metadata_update_side_data=yes
|
|
if test "$_avcodec_has_metadata_update_side_data" = yes ; then
|
|
def_avcodec_has_metadata_update_side_data='#define HAVE_AVCODEC_METADATA_UPDATE_SIDE_DATA 1'
|
|
else
|
|
def_avcodec_has_metadata_update_side_data='#define HAVE_AVCODEC_METADATA_UPDATE_SIDE_DATA 0'
|
|
fi
|
|
echores "$_avcodec_has_metadata_update_side_data"
|
|
|
|
|
|
echocheck "libavcodec replaygain side data"
|
|
_avcodec_has_replaygain_side_data=no
|
|
statement_check libavcodec/avcodec.h 'enum AVPacketSideDataType type = AV_PKT_DATA_REPLAYGAIN' && _avcodec_has_replaygain_side_data=yes
|
|
if test "$_avcodec_has_replaygain_side_data" = yes ; then
|
|
def_avcodec_has_replaygain_side_data='#define HAVE_AVCODEC_REPLAYGAIN_SIDE_DATA 1'
|
|
else
|
|
def_avcodec_has_replaygain_side_data='#define HAVE_AVCODEC_REPLAYGAIN_SIDE_DATA 0'
|
|
fi
|
|
echores "$_avcodec_has_replaygain_side_data"
|
|
|
|
|
|
echocheck "libavutil AVFrame metadata"
|
|
_avutil_has_avframe_metadata=no
|
|
statement_check libavutil/frame.h 'av_frame_get_metadata(NULL)' && _avutil_has_avframe_metadata=yes
|
|
if test "$_avutil_has_avframe_metadata" = yes ; then
|
|
def_avutil_has_avframe_metadata='#define HAVE_AVFRAME_METADATA 1'
|
|
else
|
|
def_avutil_has_avframe_metadata='#define HAVE_AVFRAME_METADATA 0'
|
|
fi
|
|
echores "$_avutil_has_avframe_metadata"
|
|
|
|
|
|
echocheck "libavutil QP API"
|
|
_avutil_has_qp_api=no
|
|
statement_check libavutil/frame.h 'av_frame_get_qp_table(NULL, NULL, NULL)' && _avutil_has_qp_api=yes
|
|
if test "$_avutil_has_qp_api" = yes ; then
|
|
def_avutil_has_qp_api='#define HAVE_AVUTIL_QP_API 1'
|
|
else
|
|
def_avutil_has_qp_api='#define HAVE_AVUTIL_QP_API 0'
|
|
fi
|
|
echores "$_avutil_has_qp_api"
|
|
|
|
|
|
echocheck "libavfilter"
|
|
if test "$libavfilter" = auto ; then
|
|
libavfilter=no
|
|
if pkg_config_add "libavfilter >= 3.90.100" ; then
|
|
libavfilter=yes
|
|
fi
|
|
fi
|
|
if test "$libavfilter" = yes ; then
|
|
def_libavfilter='#define HAVE_LIBAVFILTER 1'
|
|
else
|
|
def_libavfilter='#define HAVE_LIBAVFILTER 0'
|
|
fi
|
|
echores "$libavfilter"
|
|
|
|
|
|
echocheck "libavdevice >= 54.0.0"
|
|
if test "$libavdevice" = auto ; then
|
|
libavdevice=no
|
|
if pkg_config_add "libavdevice >= 54.0.0" ; then
|
|
libavdevice=yes
|
|
fi
|
|
fi
|
|
if test "$libavdevice" = yes ; then
|
|
def_libavdevice='#define HAVE_LIBAVDEVICE 1'
|
|
else
|
|
def_libavdevice='#define HAVE_LIBAVDEVICE 0'
|
|
fi
|
|
echores "$libavdevice"
|
|
|
|
|
|
echocheck "libpostproc >= 52.0.0"
|
|
if test "$libpostproc" = auto ; then
|
|
libpostproc=no
|
|
if pkg_config_add "libpostproc >= 52.0.0" ; then
|
|
libpostproc=yes
|
|
fi
|
|
fi
|
|
if test "$libpostproc" = yes ; then
|
|
def_libpostproc='#define HAVE_LIBPOSTPROC 1'
|
|
else
|
|
def_libpostproc='#define HAVE_LIBPOSTPROC 0'
|
|
fi
|
|
echores "$libpostproc"
|
|
|
|
if darwin ; then
|
|
|
|
echocheck "VDA"
|
|
if test "$_vda" = auto ; then
|
|
_vda=no
|
|
header_check VideoDecodeAcceleration/VDADecoder.h &&
|
|
statement_check libavcodec/vda.h 'ff_vda_create_decoder(NULL, NULL, NULL)' &&
|
|
_vda=yes
|
|
fi
|
|
if test "$_vda" = yes ; then
|
|
def_vda='#define HAVE_VDA_HWACCEL 1'
|
|
libs_mplayer="$libs_mplayer -framework VideoDecodeAcceleration -framework QuartzCore -framework IOSurface"
|
|
else
|
|
def_vda='#define HAVE_VDA_HWACCEL 0'
|
|
fi
|
|
echores "$_vda"
|
|
|
|
echocheck "VDA libavcodec refcounting"
|
|
_vda_refcounting=no
|
|
if test "$_vda" = yes ; then
|
|
statement_check libavcodec/vda.h 'struct vda_context a = (struct vda_context) { .use_ref_buffer = 1 }' &&
|
|
_vda_refcounting=yes
|
|
fi
|
|
if test "$_vda_refcounting" = "yes" ; then
|
|
def_vda_refcounting='#define HAVE_VDA_LIBAVCODEC_REFCOUNTING 1'
|
|
else
|
|
def_vda_refcounting='#define HAVE_VDA_LIBAVCODEC_REFCOUNTING 0'
|
|
fi
|
|
echores "$_vda_refcounting"
|
|
|
|
echocheck "VDA with OpenGL"
|
|
if test "$_gl_cocoa" = yes && test "$_vda" = yes ; then
|
|
def_vda_gl='#define HAVE_VDA_GL 1'
|
|
_vda_gl=yes
|
|
else
|
|
def_vda_gl='#define HAVE_VDA_GL 0'
|
|
_vda_gl=no
|
|
fi
|
|
echores "$_vda_gl"
|
|
|
|
else
|
|
def_vda='#define HAVE_VDA_HWACCEL 0'
|
|
def_vda_refcounting='#define HAVE_VDA_LIBAVCODEC_REFCOUNTING 0'
|
|
def_vda_gl='#define HAVE_VDA_GL 0'
|
|
fi
|
|
|
|
|
|
echocheck "TV interface"
|
|
if test "$_tv" = yes ; then
|
|
def_tv='#define HAVE_TV 1'
|
|
inputmodules="tv $inputmodules"
|
|
else
|
|
noinputmodules="tv $noinputmodules"
|
|
def_tv='#define HAVE_TV 0'
|
|
fi
|
|
echores "$_tv"
|
|
|
|
|
|
echocheck "Video 4 Linux 2 TV interface"
|
|
if test "$_tv_v4l2" = auto ; then
|
|
_tv_v4l2=no
|
|
if test "$_tv" = yes && linux ; then
|
|
header_check_broken sys/time.h linux/videodev2.h && _tv_v4l2=yes
|
|
elif test "$_tv" = yes && freebsd ; then
|
|
header_check linux/videodev2.h && _tv_v4l2=yes
|
|
elif test "$_tv" = yes && test "$sys_videoio_h" = "yes" ; then
|
|
_tv_v4l2=yes
|
|
fi
|
|
fi
|
|
if test "$_tv_v4l2" = yes ; then
|
|
_audio_input=yes
|
|
def_tv_v4l2='#define HAVE_TV_V4L2 1'
|
|
inputmodules="tv-v4l2 $inputmodules"
|
|
else
|
|
noinputmodules="tv-v4l2 $noinputmodules"
|
|
def_tv_v4l2='#define HAVE_TV_V4L2 0'
|
|
fi
|
|
echores "$_tv_v4l2"
|
|
|
|
echocheck "libv4l2 support"
|
|
if test "$_libv4l2" = auto ; then
|
|
_libv4l2=no
|
|
if pkg_config_add "libv4l2" ; then
|
|
_libv4l2=yes
|
|
fi
|
|
fi
|
|
if test "$_libv4l2" = yes; then
|
|
def_libv4l2="#define HAVE_LIBV4L2 1"
|
|
else
|
|
def_libv4l2="#define HAVE_LIBV4L2 0"
|
|
fi
|
|
echores "$_libv4l2"
|
|
|
|
|
|
echocheck "Video 4 Linux 2 MPEG PVR interface"
|
|
if test "$_pvr" = auto ; then
|
|
_pvr=no
|
|
if test "$_tv_v4l2" = yes ; then
|
|
cat > $TMPC <<EOF
|
|
#include <sys/time.h>
|
|
#include <linux/videodev2.h>
|
|
int main(void) { struct v4l2_ext_controls ext; return ext.controls->value; }
|
|
EOF
|
|
cc_check && _pvr=yes
|
|
fi
|
|
fi
|
|
if test "$_pvr" = yes ; then
|
|
def_pvr='#define HAVE_PVR 1'
|
|
inputmodules="pvr $inputmodules"
|
|
else
|
|
noinputmodules="pvr $noinputmodules"
|
|
def_pvr='#define HAVE_PVR 0'
|
|
fi
|
|
echores "$_pvr"
|
|
|
|
|
|
# Note: Lua has no official .pc file, so there are different OS-specific ones.
|
|
# Also, we support luajit, which is compatible to 5.1.
|
|
# The situation is further complicated by distros supporting multiple Lua
|
|
# versions, without ensuring libraries linking to conflicting Lua libs don't
|
|
# cause issues. This is a real problem with libquvi.
|
|
|
|
cat > $TMPC << EOF
|
|
#include <stdlib.h>
|
|
#include <lua.h>
|
|
#include <lualib.h>
|
|
#include <lauxlib.h>
|
|
EOF
|
|
# abuse $TMPH file as second temp file
|
|
cat > $TMPH << EOF
|
|
void test_lua(void) {
|
|
lua_State *L = luaL_newstate();
|
|
lua_pushstring(L, "test");
|
|
lua_setglobal(L, "test");
|
|
}
|
|
void test_other(void) {
|
|
EOF
|
|
|
|
# test all other Lua using packages (hack that gives us time)
|
|
if test "$_libquvi4" = yes ; then
|
|
|
|
echo "#include <quvi/quvi.h>" >> $TMPC
|
|
cat >> $TMPH << EOF
|
|
quvi_t q;
|
|
if (quvi_init(&q) == QUVI_OK)
|
|
quvi_supported(q, "http://nope");
|
|
EOF
|
|
|
|
fi
|
|
|
|
if test "$_libquvi9" = yes ; then
|
|
|
|
echo "#include <quvi.h>" >> $TMPC
|
|
cat >> $TMPH << EOF
|
|
quvi_t q = quvi_new();
|
|
if (quvi_ok(q))
|
|
quvi_supports(q, "http://nope", QUVI_SUPPORTS_MODE_OFFLINE, QUVI_SUPPORTS_TYPE_MEDIA);
|
|
EOF
|
|
|
|
fi
|
|
|
|
cat >> $TMPH << EOF
|
|
}
|
|
int main(void) {
|
|
test_lua();
|
|
test_other();
|
|
return 0;
|
|
}
|
|
EOF
|
|
|
|
cat $TMPH >> $TMPC
|
|
|
|
test_lua() {
|
|
# changed by pkg_config_add
|
|
old_extra_cflags="$extra_cflags"
|
|
old_libs_mplayer="$libs_mplayer"
|
|
echocheck "Lua $2 ($1)"
|
|
if test "$lua" = yes ; then
|
|
echores "no"
|
|
return 1
|
|
fi
|
|
if test "x$lua_pkg" != "x" && test "$lua_pkg" != "$1" ; then
|
|
echores "no"
|
|
return 1
|
|
fi
|
|
if pkg_config_add "$2" ; then
|
|
if test $_cross_compile = no ; then
|
|
if cc_check && tmp_run ; then
|
|
echo > /dev/null # idiot NOP
|
|
else
|
|
extra_cflags="$old_extra_cflags"
|
|
libs_mplayer="$old_libs_mplayer"
|
|
echores "no - does not run"
|
|
return 1
|
|
fi
|
|
fi
|
|
lua=yes
|
|
fi
|
|
echores "$lua"
|
|
test "$lua" = yes
|
|
return $?
|
|
}
|
|
|
|
if test "$lua" = auto ; then
|
|
|
|
lua=no
|
|
test_lua 51 "lua >= 5.1.0 lua < 5.2.0"
|
|
test_lua 51deb "lua5.1 >= 5.1.0" # debian
|
|
test_lua luajit "luajit >= 2.0.0"
|
|
# assume all our dependencies (libquvi in particular) link with 5.1
|
|
test_lua 52 "lua >= 5.2.0"
|
|
test_lua 52deb "lua5.2 >= 5.2.0" # debian
|
|
|
|
fi
|
|
|
|
if test "$lua" = yes ; then
|
|
def_lua='#define HAVE_LUA 1'
|
|
else
|
|
def_lua='#define HAVE_LUA 0'
|
|
fi
|
|
|
|
echocheck "encoding"
|
|
if test "$_encoding" = yes ; then
|
|
def_encoding="#define HAVE_ENCODING 1"
|
|
else
|
|
def_encoding="#define HAVE_ENCODING 0"
|
|
fi
|
|
echores "$_encoding"
|
|
|
|
|
|
# needs dlopen on unix, uses winapi on windows
|
|
_dlopen="$_dl"
|
|
if win32 ; then
|
|
_dlopen=yes
|
|
fi
|
|
|
|
if test "$_dlopen" = yes ; then
|
|
def_dlopen='#define HAVE_DLOPEN 1'
|
|
else
|
|
def_dlopen='#define HAVE_DLOPEN 0'
|
|
fi
|
|
|
|
#############################################################################
|
|
|
|
echocheck "compiler support for noexecstack"
|
|
if cflag_check -Wl,-z,noexecstack ; then
|
|
extra_ldflags="-Wl,-z,noexecstack $extra_ldflags"
|
|
echores "yes"
|
|
else
|
|
echores "no"
|
|
fi
|
|
|
|
echocheck "linker support for --nxcompat --no-seh --dynamicbase"
|
|
if cflag_check "-Wl,--nxcompat -Wl,--no-seh -Wl,--dynamicbase" ; then
|
|
extra_ldflags="-Wl,--nxcompat -Wl,--no-seh -Wl,--dynamicbase $extra_ldflags"
|
|
echores "yes"
|
|
else
|
|
echores "no"
|
|
fi
|
|
|
|
|
|
extra_ldflags="$extra_ldflags $_ld_pthread"
|
|
libs_mplayer="$libs_mplayer $_ld_dl"
|
|
|
|
|
|
echocheck "joystick"
|
|
def_joystick='#define HAVE_JOYSTICK 0'
|
|
if test "$_joystick" = yes ; then
|
|
if linux || freebsd ; then
|
|
# TODO add some check
|
|
def_joystick='#define HAVE_JOYSTICK 1'
|
|
else
|
|
_joystick="no"
|
|
res_comment="unsupported under $system_name"
|
|
fi
|
|
fi
|
|
echores "$_joystick"
|
|
|
|
echocheck "lirc"
|
|
if test "$_lirc" = auto ; then
|
|
_lirc=no
|
|
header_check lirc/lirc_client.h -llirc_client && _lirc=yes
|
|
fi
|
|
if test "$_lirc" = yes ; then
|
|
def_lirc='#define HAVE_LIRC 1'
|
|
libs_mplayer="$libs_mplayer -llirc_client"
|
|
else
|
|
def_lirc='#define HAVE_LIRC 0'
|
|
fi
|
|
echores "$_lirc"
|
|
|
|
#############################################################################
|
|
|
|
if mingw32 ; then
|
|
# add this last, so that --libs from pkg-config can't override it
|
|
end_ldflags="$end_ldflags -mconsole"
|
|
fi
|
|
|
|
CFLAGS="$CFLAGS -D_LARGEFILE_SOURCE -D_FILE_OFFSET_BITS=64 -D_LARGEFILE64_SOURCE"
|
|
|
|
CXXFLAGS=" $CFLAGS -D__STDC_CONSTANT_MACROS -D__STDC_FORMAT_MACROS -D__STDC_LIMIT_MACROS"
|
|
|
|
# DO NOT ADD ANY TESTS THAT USE LINKER FLAGS HERE (like cc_check).
|
|
|
|
# This is done so waf builds won't conflict with this. In fact, waf and old
|
|
# build system can coexist in parallel, at the same time. This is because
|
|
# waf always does out-of-tree builds, while this build system does always
|
|
# in-tree builds.
|
|
|
|
if test ! -f Makefile ; then
|
|
ln -s old-makefile Makefile
|
|
fi
|
|
rm -rf old_build
|
|
mkdir old_build
|
|
|
|
BUILDCFLAGS="-Iold_build"
|
|
|
|
#############################################################################
|
|
echo "Creating config.mak"
|
|
cat > old_build/config.mak << EOF
|
|
# -------- Generated by configure -----------
|
|
|
|
# Ensure that locale settings do not interfere with shell commands.
|
|
export LC_ALL = C
|
|
|
|
CONFIGURATION = $configuration
|
|
|
|
prefix = \$(DESTDIR)$_prefix
|
|
BINDIR = \$(DESTDIR)$_bindir
|
|
MANDIR = \$(DESTDIR)$_mandir
|
|
DOCDIR = \$(DESTDIR)$_docdir
|
|
CONFDIR = \$(DESTDIR)$_confdir
|
|
|
|
CC = $_cc
|
|
CXX = $_cc
|
|
INSTALL = $_install
|
|
WINDRES = $_windres
|
|
|
|
CFLAGS = $BUILDCFLAGS $WARNFLAGS $ERRORFLAGS $WARN_CFLAGS $CFLAGS $extra_cflags
|
|
CXXFLAGS = $BUILDCFLAGS $WARNFLAGS $ERRORFLAGS $CXXFLAGS $extra_cflags $extra_cxxflags
|
|
DEPFLAGS = $DEPFLAGS
|
|
|
|
EXTRALIBS = $extra_ldflags $_ld_static $_ld_lm $extra_libs $libs_mplayer $end_ldflags
|
|
|
|
GETCH = $_getch
|
|
TIMER = $_timer
|
|
RST2MAN = $_rst2man
|
|
BUILD_MAN = $_build_man
|
|
RST2PDF = $_rst2pdf
|
|
BUILD_PDF = $_build_pdf
|
|
|
|
EXESUF = $_exesuf
|
|
EXESUFS_ALL = .exe
|
|
|
|
NEED_GLOB = $need_glob
|
|
|
|
# features
|
|
ALSA = $_alsa
|
|
AUDIO_INPUT = $_audio_input
|
|
CACA = $_caca
|
|
CDDA = $_cdda
|
|
COCOA = $_cocoa
|
|
COREAUDIO = $_coreaudio
|
|
COREVIDEO = $_corevideo
|
|
DIRECT3D = $_direct3d
|
|
DL = $_dl
|
|
DLOPEN = $_dlopen
|
|
SDL = $_sdl
|
|
SDL2 = $_sdl2
|
|
DSOUND = $_dsound
|
|
WASAPI = $_wasapi
|
|
DVBIN = $_dvbin
|
|
DVDREAD = $_dvdread
|
|
DVDNAV = $_dvdnav
|
|
GL = $_gl
|
|
GL_COCOA = $_gl_cocoa
|
|
GL_WIN32 = $_gl_win32
|
|
GL_X11 = $_gl_x11
|
|
GL_WAYLAND = $_gl_wayland
|
|
HAVE_POSIX_SELECT = $_posix_select
|
|
HAVE_SYS_MMAN_H = $_mman
|
|
JACK = $_jack
|
|
JOYSTICK = $_joystick
|
|
JPEG = $_jpeg
|
|
LADSPA = $_ladspa
|
|
LIBASS = $_ass
|
|
LIBASS_OSD = $_libass_osd
|
|
DUMMY_OSD = $_dummy_osd
|
|
LIBBLURAY = $_bluray
|
|
LIBBS2B = $_libbs2b
|
|
LCMS2 = $_lcms2
|
|
VAPOURSYNTH = $_vapoursynth
|
|
LUA = $lua
|
|
LIBPOSTPROC = $libpostproc
|
|
LIBAVDEVICE = $libavdevice
|
|
LIBAVFILTER = $libavfilter
|
|
LIBSMBCLIENT = $_smb
|
|
LIBQUVI = $_libquvi4
|
|
LIBQUVI9 = $_libquvi9
|
|
LIBGUESS = $_libguess
|
|
LIRC = $_lirc
|
|
MPG123 = $_mpg123
|
|
OPENAL = $_openal
|
|
OSS = $_ossaudio
|
|
PE_EXECUTABLE = $_pe_executable
|
|
PRIORITY = $_priority
|
|
PULSE = $_pulse
|
|
PORTAUDIO = $_portaudio
|
|
PVR = $_pvr
|
|
RSOUND = $_rsound
|
|
SNDIO = $_sndio
|
|
STREAM_CACHE = $_stream_cache
|
|
TV = $_tv
|
|
TV_V4L2 = $_tv_v4l2
|
|
LIBV4L2 = $_libv4l2
|
|
VCD = $_vcd
|
|
VDPAU = $_vdpau
|
|
VDPAU_GL_X11 = $_vdpau_gl_x11
|
|
VDA = $_vda
|
|
VDA_REFCOUNTING = $_vda_refcounting
|
|
VDA_GL = $_vda_gl
|
|
VAAPI = $_vaapi
|
|
VAAPI_VPP = $_vaapi_vpp
|
|
VAAPI_GLX = $_vaapi_glx
|
|
WIN32 = $_win32
|
|
X11 = $_x11
|
|
WAYLAND = $_wayland
|
|
XV = $_xv
|
|
|
|
# FFmpeg
|
|
ENCODING = $_encoding
|
|
|
|
CONFIG_VDPAU = $_vdpau
|
|
CONFIG_VDA = $_vda
|
|
CONFIG_VAAPI = $_vaapi
|
|
CONFIG_ZLIB = $_zlib
|
|
|
|
HAVE_PTHREADS = $_pthreads
|
|
HAVE_SHM = $_shm
|
|
|
|
EOF
|
|
|
|
#############################################################################
|
|
|
|
echo "Creating config.h"
|
|
cat > $TMPH << EOF
|
|
/*----------------------------------------------------------------------------
|
|
** This file has been automatically generated by configure any changes in it
|
|
** will be lost when you run configure again.
|
|
** Instead of modifying definitions here, use the --enable/--disable options
|
|
** of the configure script! See ./configure --help for details.
|
|
*---------------------------------------------------------------------------*/
|
|
|
|
#ifndef MPLAYER_CONFIG_H
|
|
#define MPLAYER_CONFIG_H
|
|
|
|
#define CONFIGURATION "$configuration"
|
|
|
|
#define MPLAYER_CONFDIR "$_confdir"
|
|
|
|
/* we didn't bother to add actual config checks for this */
|
|
#define HAVE_BSD_FSTATFS 0
|
|
#define HAVE_LINUX_FSTATFS 0
|
|
|
|
/* system headers */
|
|
$def_mman_h
|
|
$def_mman_has_map_failed
|
|
$def_soundcard_h
|
|
$def_sys_soundcard_h
|
|
$def_sys_sysinfo_h
|
|
$def_sys_videoio_h
|
|
$def_termios_h
|
|
$def_termios_sys_h
|
|
$def_winsock2_h
|
|
|
|
|
|
/* system functions */
|
|
$def_glob
|
|
$def_nanosleep
|
|
$def_posix_select
|
|
$def_select
|
|
$def_setmode
|
|
$def_shm
|
|
$def_terminfo
|
|
$def_termcap
|
|
$def_termios
|
|
|
|
|
|
/* system-specific features */
|
|
$def_dl
|
|
$def_dos_paths
|
|
$def_iconv
|
|
$def_priority
|
|
|
|
|
|
/* configurable options */
|
|
$def_stream_cache
|
|
|
|
/* Blu-ray/DVD/VCD/CD */
|
|
#define DEFAULT_CDROM_DEVICE "$default_cdrom_device"
|
|
#define DEFAULT_DVD_DEVICE "$default_dvd_device"
|
|
$def_bluray
|
|
$def_cdda
|
|
$def_dvdread
|
|
$def_dvdnav
|
|
$def_vcd
|
|
|
|
|
|
/* codec libraries */
|
|
$def_mpg123
|
|
$def_zlib
|
|
|
|
$def_avutil_has_qp_api
|
|
$def_avcodec_has_chroma_pos_api
|
|
$def_avcodec_has_metadata_update_side_data
|
|
$def_avcodec_has_replaygain_side_data
|
|
$def_avutil_has_avframe_metadata
|
|
$def_libpostproc
|
|
$def_libavdevice
|
|
$def_libavfilter
|
|
|
|
$def_dlopen
|
|
|
|
/* Audio output drivers */
|
|
$def_alsa
|
|
$def_coreaudio
|
|
$def_jack
|
|
$def_openal
|
|
$def_openal_h
|
|
$def_ossaudio
|
|
$def_ossaudio_devdsp
|
|
$def_ossaudio_devmixer
|
|
$def_pulse
|
|
$def_portaudio
|
|
$def_rsound
|
|
$def_sndio
|
|
|
|
$def_ladspa
|
|
$def_libbs2b
|
|
|
|
|
|
/* input */
|
|
$def_joystick
|
|
$def_lirc
|
|
$def_pvr
|
|
$def_tv
|
|
$def_tv_v4l2
|
|
$def_libv4l2
|
|
|
|
|
|
/* font stuff */
|
|
$def_ass
|
|
$def_enca
|
|
|
|
/* networking */
|
|
$def_smb
|
|
$def_libquvi4
|
|
$def_libquvi9
|
|
$def_libguess
|
|
|
|
$def_lcms2
|
|
$def_vapoursynth
|
|
$def_lua
|
|
|
|
|
|
/* libvo options */
|
|
$def_caca
|
|
$def_corevideo
|
|
$def_cocoa
|
|
$def_direct3d
|
|
$def_sdl
|
|
$def_sdl2
|
|
$def_dsound
|
|
$def_wasapi
|
|
$def_dvb
|
|
$def_dvbin
|
|
$def_gl
|
|
$def_gl_cocoa
|
|
$def_gl_win32
|
|
$def_gl_x11
|
|
$def_gl_wayland
|
|
$def_jpeg
|
|
$def_v4l2
|
|
$def_vdpau
|
|
$def_vdpau_gl_x11
|
|
$def_vdpau_hwaccel
|
|
$def_vda
|
|
$def_vda_gl
|
|
$def_vda_refcounting
|
|
$def_vaapi
|
|
$def_vaapi_vpp
|
|
$def_vaapi_glx
|
|
$def_vaapi_hwaccel
|
|
$def_vm
|
|
$def_x11
|
|
$def_wayland
|
|
$def_xext
|
|
$def_xf86keysym
|
|
$def_xinerama
|
|
$def_xss
|
|
$def_xv
|
|
|
|
|
|
/* FFmpeg */
|
|
$def_encoding
|
|
$def_libavresample
|
|
$def_libswresample
|
|
|
|
$def_fast_64bit
|
|
$def_pthreads
|
|
$def_atomic
|
|
$def_sync
|
|
|
|
#endif /* MPLAYER_CONFIG_H */
|
|
EOF
|
|
|
|
# Do not overwrite an unchanged config.h to avoid superfluous rebuilds.
|
|
cmp -s "$TMPH" old_build/config.h || mv -f "$TMPH" old_build/config.h
|
|
|
|
# Kill old config.h, as it might interfere with the build. Also get rid of
|
|
# old config.mak, which might be confusing.
|
|
rm -f config.h config.mak
|
|
|
|
#############################################################################
|
|
|
|
cat << EOF
|
|
|
|
Config files successfully generated by ./configure $configuration !
|
|
|
|
Install prefix: $_prefix
|
|
Config direct.: $_confdir
|
|
|
|
Enabled optional drivers:
|
|
Input: $inputmodules
|
|
Codecs: libavcodecs $codecmodules
|
|
Audio output: $aomodules
|
|
Video output: $vomodules
|
|
|
|
Disabled optional drivers:
|
|
Input: $noinputmodules
|
|
Codecs: $nocodecmodules
|
|
Audio output: $noaomodules
|
|
Video output: $novomodules
|
|
|
|
'config.h' and 'config.mak' contain your configuration options.
|
|
Note: If you alter theses files (for instance CFLAGS) mpv may no longer
|
|
compile *** DO NOT REPORT BUGS if you tweak these files ***
|
|
|
|
'make' will now compile mpv and 'make install' will install it.
|
|
Note: On non-Linux systems you might need to use 'gmake' instead of 'make'.
|
|
|
|
EOF
|
|
|
|
|
|
cat <<EOF
|
|
Check $TMPLOG if you wonder why an autodetection failed (make sure
|
|
development headers/packages are installed).
|
|
|
|
NOTE: The --enable-* parameters unconditionally force options on, completely
|
|
skipping autodetection. This behavior is unlike what you may be used to from
|
|
autoconf-based configure scripts that can decide to override you. This greater
|
|
level of control comes at a price. You may have to provide the correct compiler
|
|
and linker flags yourself.
|
|
If you used one of these options and experience a compilation or
|
|
linking failure, make sure you have passed the necessary compiler/linker flags
|
|
to configure.
|
|
|
|
WARNING: The ./old-configure + make build system you are using is deprecated in
|
|
favour of waf and will be removed in a future version of mpv. Check the
|
|
README for instructions on how to build mpv with the new build system.
|
|
|
|
EOF
|
|
|
|
if test "$warn_cflags" = yes; then
|
|
cat <<EOF
|
|
|
|
mpv compilation will use the CPPFLAGS/CFLAGS/LDFLAGS set by you, but:
|
|
|
|
*** *** DO NOT REPORT BUGS IF IT DOES NOT COMPILE/WORK! *** ***
|
|
|
|
It is strongly recommended to let mpv choose the correct CFLAGS!
|
|
To do so, execute 'CFLAGS= ./configure <options>'
|
|
|
|
EOF
|
|
fi
|
|
|
|
# Last move:
|
|
rm -rf "$mplayer_tmpdir"
|