mirror of
https://github.com/mpv-player/mpv
synced 2024-07-31 16:29:58 +02:00
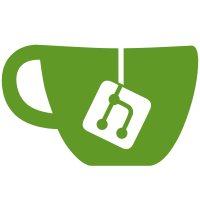
Current volume was always queried from the the audio output driver (or filter in case of --softvol). The only case where it was stored on mixer level was that when turning off mute, volume was set to the value it had before mute was activated. Change the mixer code to always store the current target volume internally. It still checks for significant changes from external sources and resets the internal value in that case. The main functionality changes are: Volume will now be kept separately from mute status. Increasing or decreasing volume will now change it relative to the original value before mute, even if mute is implemented by setting AO level volume to 0. Volume changes no longer automatically disable mute. The exception is relative changes up (like the volume increase key in default keybindings); that's the only case which still disables mute. Keeping the value internally avoids problems with granularity of possible volume values supported by AO. Increase/decrease keys could work unsymmetrically, or when specifying a smaller than default --volstep, even fail completely. In one case occurring in practice, if the AO only supports changing volume in steps of about 2 and rounds down the requested volume, then volume down key would decrease by 4 but volume up would increase by 2 (previous volume plus or minus the default change of 3, rounded down to a multiple of 2). Now, the internal value will keep full precision.
48 lines
1.5 KiB
C
48 lines
1.5 KiB
C
/*
|
|
* This file is part of MPlayer.
|
|
*
|
|
* MPlayer is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* MPlayer is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with MPlayer; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#ifndef MPLAYER_MIXER_H
|
|
#define MPLAYER_MIXER_H
|
|
|
|
#include <stdbool.h>
|
|
|
|
#include "libaf/af.h"
|
|
#include "libao2/audio_out.h"
|
|
|
|
typedef struct mixer {
|
|
struct ao *ao;
|
|
af_stream_t *afilter;
|
|
int volstep;
|
|
bool softvol;
|
|
float softvol_max;
|
|
bool muted;
|
|
float vol_l, vol_r;
|
|
} mixer_t;
|
|
|
|
void mixer_getvolume(mixer_t *mixer, float *l, float *r);
|
|
void mixer_setvolume(mixer_t *mixer, float l, float r);
|
|
void mixer_incvolume(mixer_t *mixer);
|
|
void mixer_decvolume(mixer_t *mixer);
|
|
void mixer_getbothvolume(mixer_t *mixer, float *b);
|
|
void mixer_setmute(mixer_t *mixer, bool mute);
|
|
bool mixer_getmute(mixer_t *mixer);
|
|
void mixer_getbalance(mixer_t *mixer, float *bal);
|
|
void mixer_setbalance(mixer_t *mixer, float bal);
|
|
|
|
#endif /* MPLAYER_MIXER_H */
|