mirror of
https://github.com/mpv-player/mpv
synced 2024-10-30 04:46:41 +01:00
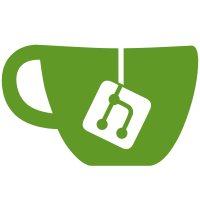
git-svn-id: svn://svn.mplayerhq.hu/mplayer/trunk@3560 b3059339-0415-0410-9bf9-f77b7e298cf2
59 lines
1.3 KiB
C
59 lines
1.3 KiB
C
/*
|
|
* command line and config file parser
|
|
*/
|
|
|
|
#ifndef __CONFIG_H
|
|
#define __CONFIG_H
|
|
|
|
#define CONF_TYPE_FLAG 0
|
|
#define CONF_TYPE_INT 1
|
|
#define CONF_TYPE_FLOAT 2
|
|
#define CONF_TYPE_STRING 3
|
|
#define CONF_TYPE_FUNC 4
|
|
#define CONF_TYPE_FUNC_PARAM 5
|
|
#define CONF_TYPE_PRINT 6
|
|
#define CONF_TYPE_FUNC_FULL 7
|
|
#define CONF_TYPE_SUBCONFIG 8
|
|
|
|
|
|
#define ERR_NOT_AN_OPTION -1
|
|
#define ERR_MISSING_PARAM -2
|
|
#define ERR_OUT_OF_RANGE -3
|
|
#define ERR_FUNC_ERR -4
|
|
|
|
|
|
|
|
#define CONF_MIN (1<<0)
|
|
#define CONF_MAX (1<<1)
|
|
#define CONF_RANGE (CONF_MIN|CONF_MAX)
|
|
#define CONF_NOCFG (1<<2)
|
|
#define CONF_NOCMD (1<<3)
|
|
|
|
struct config {
|
|
char *name;
|
|
void *p;
|
|
unsigned int type;
|
|
unsigned int flags;
|
|
float min,max;
|
|
};
|
|
|
|
typedef int (*cfg_func_arg_param_t)(struct config *, char *, char *);
|
|
typedef int (*cfg_func_param_t)(struct config *, char *);
|
|
typedef int (*cfg_func_t)(struct config *);
|
|
|
|
/* parse_config_file returns:
|
|
* -1 on error (can't malloc, invalid option...)
|
|
* 0 if can't open configfile
|
|
* 1 on success
|
|
*/
|
|
int parse_config_file(struct config *conf, char *conffile);
|
|
|
|
/* parse_command_line returns:
|
|
* -1 on error (invalid option...)
|
|
* 0 if there was no filename on command line
|
|
* >=1 if there were filenames
|
|
*/
|
|
int parse_command_line(struct config *conf, int argc, char **argv, char **envp, char ***filenames);
|
|
|
|
#endif /* __CONFIG_H */
|