mirror of
https://github.com/rapid7/metasploit-payloads
synced 2025-03-18 15:14:10 +01:00
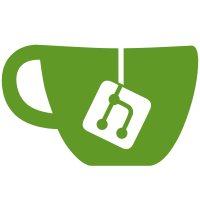
By making this a static _inline, it is not necessary to guard it, since an inline is only instantiated if it is used. This also allows adding one-off debug message for use during debugging sessions, without turning on DEBUGTRACE all over the place. Convert a few of the extensions to also do this as well, making them perhaps slightly smaller. I am curious why Windows builds define debug this way, vs posix that just includes it in common.c. Could I just do that instead, assuming there's no historical reason. Finally, correct the docs in the posix version of real_dprintf.
91 lines
2.0 KiB
C
91 lines
2.0 KiB
C
/*!
|
|
* @file common.c
|
|
* @brief Definitions for various common components used across the Meterpreter suite.
|
|
*/
|
|
#include "common.h"
|
|
|
|
|
|
#ifdef _WIN32
|
|
/*!
|
|
* @brief Returns a unix timestamp in UTC.
|
|
* @return Integer value representing the UTC Unix timestamp of the current time.
|
|
*/
|
|
int current_unix_timestamp(void) {
|
|
SYSTEMTIME system_time;
|
|
FILETIME file_time;
|
|
ULARGE_INTEGER ularge;
|
|
|
|
GetSystemTime(&system_time);
|
|
SystemTimeToFileTime(&system_time, &file_time);
|
|
|
|
ularge.LowPart = file_time.dwLowDateTime;
|
|
ularge.HighPart = file_time.dwHighDateTime;
|
|
return (long)((ularge.QuadPart - 116444736000000000) / 10000000L);
|
|
}
|
|
#else
|
|
|
|
#include <sys/time.h>
|
|
|
|
/*!
|
|
* @brief Returns a unix timestamp in UTC.
|
|
* @return Integer value representing the UTC Unix timestamp of the current time.
|
|
*/
|
|
int current_unix_timestamp(void) {
|
|
struct timeval tv;
|
|
struct timezone tz;
|
|
|
|
memset(&tv, 0, sizeof(tv));
|
|
memset(&tz, 0, sizeof(tz));
|
|
|
|
gettimeofday(&tv, &tz);
|
|
return (long) tv.tv_usec;
|
|
}
|
|
#endif
|
|
|
|
#ifndef _WIN32
|
|
|
|
int debugging_enabled;
|
|
|
|
/*!
|
|
* @brief Writes debug to a temporary file based on the current PID.
|
|
*/
|
|
void real_dprintf(char *filename, int line, const char *function, char *format, ...)
|
|
{
|
|
va_list args;
|
|
char buffer[2048];
|
|
int size;
|
|
static int fd;
|
|
int retried = 0;
|
|
|
|
filename = basename(filename);
|
|
size = snprintf(buffer, sizeof(buffer), "[%s:%d (%s)] ", filename, line, function);
|
|
|
|
va_start(args, format);
|
|
vsnprintf(buffer + size, sizeof(buffer) - size, format, args);
|
|
strcat(buffer, "\n");
|
|
va_end(args);
|
|
|
|
retry_log:
|
|
if(fd <= 0) {
|
|
char filename[128];
|
|
sprintf(filename, "/tmp/meterpreter.log.%d%s", getpid(), retried ? ".retry" : "" );
|
|
|
|
fd = open(filename, O_RDWR|O_TRUNC|O_CREAT|O_SYNC, 0644);
|
|
|
|
if(fd <= 0) return;
|
|
}
|
|
|
|
if(write(fd, buffer, strlen(buffer)) == -1 && (errno == EBADF)) {
|
|
fd = -1;
|
|
retried++;
|
|
goto retry_log;
|
|
}
|
|
}
|
|
|
|
void enable_debugging()
|
|
{
|
|
debugging_enabled = 1;
|
|
}
|
|
|
|
#endif
|