mirror of
https://github.com/rapid7/metasploit-payloads
synced 2025-04-18 07:11:12 +02:00
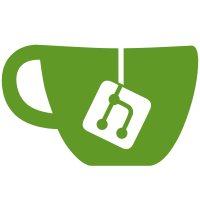
The 'common' library has been removed. The only project that actually used it was metsrv, so the code that metsrv required from common is now directly compiled in as part of that project. The common folder now contains files that are importanta cross all of the projects, with a primary focus on the new "API" style function. What this means is that MetSrv has an API that it exposes through a function pointer that is passed to the extension when it's initialised. This pointer references a structure with all the API functions wired in. This means that: * Extensions don't need to know anything about metsrv at compile time. * The delay loading code can be removed, which was one of the last instances of "metsrv.dll" as a string. * Metsrv.dll no longer exports any functions. More to come.
112 lines
3.7 KiB
C
Executable File
112 lines
3.7 KiB
C
Executable File
/*!
|
|
* @file common.h
|
|
* @brief Declarations for various common components used across the Meterpreter suite.
|
|
*/
|
|
#ifndef _METERPRETER_SOURCE_COMMON_COMMON_H
|
|
#define _METERPRETER_SOURCE_COMMON_COMMON_H
|
|
|
|
/*! @brief Set to 0 for "normal", and 1 to "verbose", comment out to disable completely. */
|
|
//#define DEBUGTRACE 0
|
|
|
|
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
#include <time.h>
|
|
|
|
#define SAFE_FREE(x) if(x){free(x);x=NULL;}
|
|
|
|
#include <winsock2.h>
|
|
#include <windows.h>
|
|
|
|
// Simple trick to get the current meterpreters arch
|
|
#define PROCESS_ARCH_UNKNOWN 0
|
|
#define PROCESS_ARCH_X86 1
|
|
#define PROCESS_ARCH_X64 2
|
|
#define PROCESS_ARCH_IA64 3
|
|
|
|
#ifdef _WIN64
|
|
#define dwMeterpreterArch PROCESS_ARCH_X64
|
|
#else
|
|
#define dwMeterpreterArch PROCESS_ARCH_X86
|
|
#endif
|
|
|
|
typedef struct __UNICODE_STRING
|
|
{
|
|
USHORT Length;
|
|
USHORT MaximumLength;
|
|
PWSTR Buffer;
|
|
} _UNICODE_STRING, * _PUNICODE_STRING;
|
|
|
|
typedef DWORD __u32;
|
|
typedef struct ___u128 {
|
|
__u32 a1;
|
|
__u32 a2;
|
|
__u32 a3;
|
|
__u32 a4;
|
|
}__u128;
|
|
|
|
/*
|
|
* Avoid conflicts with Windows crypto API defines
|
|
*/
|
|
#undef OCSP_RESPONSE
|
|
#undef PKCS7_SIGNER_INFO
|
|
#undef X509_EXTENSIONS
|
|
#undef X509_CERT_PAIR
|
|
#undef X509_NAME
|
|
|
|
#ifdef DEBUGTRACE
|
|
#define dprintf(...) real_dprintf(__VA_ARGS__)
|
|
#if DEBUGTRACE == 1
|
|
#define vdprintf dprintf
|
|
#else
|
|
#define vdprintf(...) do{}while(0);
|
|
#endif
|
|
#else
|
|
#define dprintf(...) do{}while(0);
|
|
#define vdprintf(...) do{}while(0);
|
|
#endif
|
|
|
|
/*! @brief Sets `dwResult` to the return value of `GetLastError()`, prints debug output, then does `break;` */
|
|
#define BREAK_ON_ERROR( str ) { dwResult = GetLastError(); dprintf( "%s. error=%d (0x%x)", str, dwResult, (ULONG_PTR)dwResult ); break; }
|
|
/*! @brief Sets `dwResult` to `error`, prints debug output, then `break;` */
|
|
#define BREAK_WITH_ERROR( str, err ) { dwResult = err; dprintf( "%s. error=%d (0x%x)", str, dwResult, (ULONG_PTR)dwResult ); break; }
|
|
/*! @brief Sets `dwResult` to the return value of `WASGetLastError()`, prints debug output, then does `break;` */
|
|
#define BREAK_ON_WSAERROR( str ) { dwResult = WSAGetLastError(); dprintf( "%s. error=%d (0x%x)", str, dwResult, (ULONG_PTR)dwResult ); break; }
|
|
/*! @brief Sets `dwResult` to the return value of `GetLastError()`, prints debug output, then does `continue;` */
|
|
#define CONTINUE_ON_ERROR( str ) { dwResult = GetLastError(); dprintf( "%s. error=%d (0x%x)", str, dwResult, (ULONG_PTR)dwResult ); continue; }
|
|
|
|
/*! @brief Close a service handle if not already closed and set the handle to NULL. */
|
|
#define CLOSE_SERVICE_HANDLE( h ) if( h ) { CloseServiceHandle( h ); h = NULL; }
|
|
/*! @brief Close a handle if not already closed and set the handle to NULL. */
|
|
#define CLOSE_HANDLE( h ) if( h ) { DWORD dwHandleFlags; if(GetHandleInformation( h , &dwHandleFlags)) CloseHandle( h ); h = NULL; }
|
|
|
|
/*!
|
|
* @brief Output a debug string to the debug console.
|
|
* @details The function emits debug strings via `OutputDebugStringA`, hence all messages can be viewed
|
|
* using Visual Studio's _Output_ window, _DebugView_ from _SysInternals_, or _Windbg_.
|
|
*/
|
|
static _inline void real_dprintf(char *format, ...)
|
|
{
|
|
va_list args;
|
|
char buffer[1024];
|
|
size_t len;
|
|
_snprintf_s(buffer, sizeof(buffer), sizeof(buffer)-1, "[%x] ", GetCurrentThreadId());
|
|
len = strlen(buffer);
|
|
va_start(args, format);
|
|
vsnprintf_s(buffer + len, sizeof(buffer)-len, sizeof(buffer)-len - 3, format, args);
|
|
strcat_s(buffer, sizeof(buffer), "\r\n");
|
|
OutputDebugStringA(buffer);
|
|
va_end(args);
|
|
}
|
|
|
|
#include "common_base.h"
|
|
#include "common_core.h"
|
|
#include "common_remote.h"
|
|
#include "common_channel.h"
|
|
#include "common_list.h"
|
|
#include "common_config.h"
|
|
#include "common_pivot_tree.h"
|
|
#include "common_thread.h"
|
|
#include "common_scheduler.h"
|
|
|
|
#endif
|