mirror of
https://github.com/rapid7/metasploit-payloads
synced 2025-04-06 01:16:37 +02:00
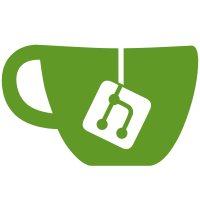
POSIX was out of whack with Windows as a result of the changes made around channels. The schedular in posix was very different, and this commit brings it into line. Other than the obvious issues, a non-obvious issue with the changes was that the channel was being freed up on close prior to the thread terminating. This doesn't appear to be an issue on Windows, but was causing crashes on close in POSIX. The changes go quite deep. This changeset requires a lot of testing.
98 lines
2.4 KiB
C
98 lines
2.4 KiB
C
/*!
|
|
* @file common.c
|
|
* @brief Definitions for various common components used across the Meterpreter suite.
|
|
*/
|
|
#include "common.h"
|
|
|
|
|
|
#ifdef _WIN32
|
|
/*!
|
|
* @brief Returns a unix timestamp in UTC.
|
|
* @return Integer value representing the UTC Unix timestamp of the current time.
|
|
*/
|
|
int current_unix_timestamp(void) {
|
|
SYSTEMTIME system_time;
|
|
FILETIME file_time;
|
|
ULARGE_INTEGER ularge;
|
|
|
|
GetSystemTime(&system_time);
|
|
SystemTimeToFileTime(&system_time, &file_time);
|
|
|
|
ularge.LowPart = file_time.dwLowDateTime;
|
|
ularge.HighPart = file_time.dwHighDateTime;
|
|
return (long)((ularge.QuadPart - 116444736000000000) / 10000000L);
|
|
}
|
|
#else
|
|
|
|
#include <sys/time.h>
|
|
|
|
/*!
|
|
* @brief Returns a unix timestamp in UTC.
|
|
* @return Integer value representing the UTC Unix timestamp of the current time.
|
|
*/
|
|
int current_unix_timestamp(void) {
|
|
struct timeval tv;
|
|
struct timezone tz;
|
|
|
|
memset(&tv, 0, sizeof(tv));
|
|
memset(&tz, 0, sizeof(tz));
|
|
|
|
gettimeofday(&tv, &tz);
|
|
return (long) tv.tv_usec;
|
|
}
|
|
#endif
|
|
|
|
#ifndef _WIN32
|
|
|
|
int debugging_enabled = 1;
|
|
|
|
/*
|
|
*/
|
|
|
|
/*!
|
|
* @brief Output a debug string to the debug console.
|
|
* @details The function emits debug strings via `OutputDebugStringA`, hence all messages can be viewed
|
|
* using Visual Studio's _Output_ window, _DebugView_ from _SysInternals_, or _Windbg_.
|
|
* @remark If we supply real_dprintf in the common.h, each .o file will have a private copy of that symbol.
|
|
* This leads to bloat. Defining it here means that there will only be a single implementation of it.
|
|
*/
|
|
void real_dprintf(char *filename, int line, const char *function, char *format, ...)
|
|
{
|
|
va_list args;
|
|
char buffer[2048];
|
|
int size;
|
|
static int fd;
|
|
int retried = 0;
|
|
|
|
filename = basename(filename);
|
|
size = snprintf(buffer, sizeof(buffer), "[%s:%d (%s)] ", filename, line, function);
|
|
|
|
va_start(args, format);
|
|
vsnprintf(buffer + size, sizeof(buffer) - size, format, args);
|
|
strcat(buffer, "\n");
|
|
va_end(args);
|
|
|
|
retry_log:
|
|
if(fd <= 0) {
|
|
char filename[128];
|
|
sprintf(filename, "/tmp/meterpreter.log.%d%s", getpid(), retried ? ".retry" : "" );
|
|
|
|
fd = open(filename, O_RDWR|O_TRUNC|O_CREAT|O_SYNC, 0644);
|
|
|
|
if(fd <= 0) return;
|
|
}
|
|
|
|
if(write(fd, buffer, strlen(buffer)) == -1 && (errno == EBADF)) {
|
|
fd = -1;
|
|
retried++;
|
|
goto retry_log;
|
|
}
|
|
}
|
|
|
|
void enable_debugging()
|
|
{
|
|
debugging_enabled = 1;
|
|
}
|
|
|
|
#endif
|