mirror of
https://github.com/rapid7/metasploit-framework
synced 2024-11-05 14:57:30 +01:00
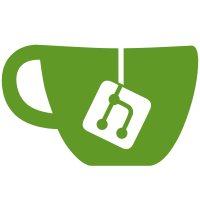
MSP-11368 MSP-11143 Remove fastlib as it slows down the code loading process. From the previous commit, the mean loading for `METASPLOIT_FRAMEWORK_PROFILE=true msfconsole -q -x exit` was 27.9530±0.3485 seconds (N=10). The mean after removal of fastlib was 17.9820±0.6497 seconds (N=10). This means an average 35.67% reduction in boot time.
69 lines
1.8 KiB
Ruby
Executable File
69 lines
1.8 KiB
Ruby
Executable File
#!/usr/bin/env ruby
|
|
#
|
|
# $Id$
|
|
#
|
|
# This script lists all modules with their mixins. Handy for finding different "kinds" of modules.
|
|
#
|
|
# $Revision$
|
|
#
|
|
|
|
msfbase = __FILE__
|
|
while File.symlink?(msfbase)
|
|
msfbase = File.expand_path(File.readlink(msfbase), File.dirname(msfbase))
|
|
end
|
|
|
|
$:.unshift(File.expand_path(File.join(File.dirname(msfbase), '..', 'lib')))
|
|
require 'msfenv'
|
|
|
|
$:.unshift(ENV['MSF_LOCAL_LIB']) if ENV['MSF_LOCAL_LIB']
|
|
|
|
require 'rex'
|
|
require 'msf/ui'
|
|
require 'msf/base'
|
|
|
|
def do_want(klass)
|
|
return false if klass.class != Module
|
|
return false if [ Kernel, ERB::Util, SNMP::BER].include?(klass)
|
|
return false if klass.to_s.match(/^Rex::Ui::Subscriber/)
|
|
|
|
return true
|
|
end
|
|
|
|
# Initialize the simplified framework instance.
|
|
$framework = Msf::Simple::Framework.create('DisableDatabase' => true)
|
|
|
|
all_modules = $framework.exploits
|
|
|
|
# If you give an argument (any argument will do), you really want a sorted
|
|
# list of mixins, regardles of the module they're in.
|
|
if ARGV[0]
|
|
mod_hash = {}
|
|
longest_name = 0
|
|
all_modules.each_module do |name, mod|
|
|
x = mod.new
|
|
mixins = x.class.ancestors.select {|y| do_want(y) }
|
|
mixins.each do |m|
|
|
mod_hash[m] ||= 0
|
|
mod_hash[m] += 1
|
|
longest_name = m.to_s.size unless m.to_s.size < longest_name
|
|
end
|
|
end
|
|
mod_hash.sort_by {|a| a[1]}.reverse.each do |arr|
|
|
puts "%-#{longest_name}s | %d" % arr
|
|
end
|
|
else
|
|
# Tables kind of suck for this.
|
|
results = []
|
|
longest_name = 0
|
|
all_modules.each_module do |name, mod|
|
|
x = mod.new
|
|
mixins = x.class.ancestors.select {|y| do_want(y) }
|
|
results << [x.fullname, mixins.sort {|a,b| a.to_s <=> b.to_s}.join(", ")]
|
|
longest_name = x.fullname.size if longest_name < x.fullname.size
|
|
end
|
|
# name | module1, module1, etc.
|
|
results.each do |r|
|
|
puts "%-#{longest_name}s | %s" % r
|
|
end
|
|
end
|