mirror of
https://github.com/home-assistant/core
synced 2024-08-28 03:36:46 +02:00
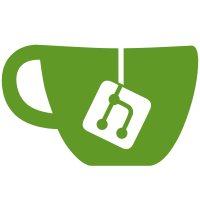
* Add foscam coordinator * Code cleanup * Coordinator cleanup * Coordinator cleanup * Replace async_timeout with asyncio.timeout * Ignore coordinator (requires external device) --------- Co-authored-by: G Johansson <goran.johansson@shiftit.se>
97 lines
2.8 KiB
Python
97 lines
2.8 KiB
Python
"""The foscam component."""
|
|
|
|
from libpyfoscam import FoscamCamera
|
|
|
|
from homeassistant.config_entries import ConfigEntry
|
|
from homeassistant.const import (
|
|
CONF_HOST,
|
|
CONF_PASSWORD,
|
|
CONF_PORT,
|
|
CONF_USERNAME,
|
|
Platform,
|
|
)
|
|
from homeassistant.core import HomeAssistant, callback
|
|
from homeassistant.helpers.entity_registry import async_migrate_entries
|
|
|
|
from .config_flow import DEFAULT_RTSP_PORT
|
|
from .const import CONF_RTSP_PORT, DOMAIN, LOGGER, SERVICE_PTZ, SERVICE_PTZ_PRESET
|
|
from .coordinator import FoscamCoordinator
|
|
|
|
PLATFORMS = [Platform.CAMERA]
|
|
|
|
|
|
async def async_setup_entry(hass: HomeAssistant, entry: ConfigEntry) -> bool:
|
|
"""Set up foscam from a config entry."""
|
|
|
|
session = FoscamCamera(
|
|
entry.data[CONF_HOST],
|
|
entry.data[CONF_PORT],
|
|
entry.data[CONF_USERNAME],
|
|
entry.data[CONF_PASSWORD],
|
|
verbose=False,
|
|
)
|
|
coordinator = FoscamCoordinator(hass, session)
|
|
|
|
await coordinator.async_config_entry_first_refresh()
|
|
|
|
hass.data.setdefault(DOMAIN, {})[entry.entry_id] = coordinator
|
|
|
|
await hass.config_entries.async_forward_entry_setups(entry, PLATFORMS)
|
|
|
|
return True
|
|
|
|
|
|
async def async_unload_entry(hass: HomeAssistant, entry: ConfigEntry) -> bool:
|
|
"""Unload a config entry."""
|
|
unload_ok = await hass.config_entries.async_unload_platforms(entry, PLATFORMS)
|
|
if unload_ok:
|
|
hass.data[DOMAIN].pop(entry.entry_id)
|
|
|
|
if not hass.data[DOMAIN]:
|
|
hass.services.async_remove(domain=DOMAIN, service=SERVICE_PTZ)
|
|
hass.services.async_remove(domain=DOMAIN, service=SERVICE_PTZ_PRESET)
|
|
|
|
return unload_ok
|
|
|
|
|
|
async def async_migrate_entry(hass: HomeAssistant, entry: ConfigEntry) -> bool:
|
|
"""Migrate old entry."""
|
|
LOGGER.debug("Migrating from version %s", entry.version)
|
|
|
|
if entry.version == 1:
|
|
# Change unique id
|
|
@callback
|
|
def update_unique_id(entry):
|
|
return {"new_unique_id": entry.entry_id}
|
|
|
|
await async_migrate_entries(hass, entry.entry_id, update_unique_id)
|
|
|
|
entry.unique_id = None
|
|
|
|
# Get RTSP port from the camera or use the fallback one and store it in data
|
|
camera = FoscamCamera(
|
|
entry.data[CONF_HOST],
|
|
entry.data[CONF_PORT],
|
|
entry.data[CONF_USERNAME],
|
|
entry.data[CONF_PASSWORD],
|
|
verbose=False,
|
|
)
|
|
|
|
ret, response = await hass.async_add_executor_job(camera.get_port_info)
|
|
|
|
rtsp_port = DEFAULT_RTSP_PORT
|
|
|
|
if ret != 0:
|
|
rtsp_port = response.get("rtspPort") or response.get("mediaPort")
|
|
|
|
hass.config_entries.async_update_entry(
|
|
entry, data={**entry.data, CONF_RTSP_PORT: rtsp_port}
|
|
)
|
|
|
|
# Change entry version
|
|
entry.version = 2
|
|
|
|
LOGGER.info("Migration to version %s successful", entry.version)
|
|
|
|
return True
|