mirror of
https://github.com/home-assistant/core
synced 2024-08-31 05:57:13 +02:00
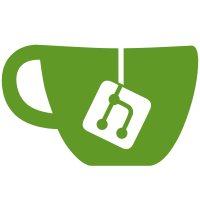
* A platform is not a component * Fix dynalite * SUPPORTED_PLATFORMS --> PLATFORMS * In tests * In tests 2 * Fix SmartThings * Fix ZHA test * Fix Z-Wave * Revert Z-Wave * Use PLATFORMS const in ambient_station * Fix ihc comment
78 lines
2.3 KiB
Python
78 lines
2.3 KiB
Python
"""Support for VELUX KLF 200 devices."""
|
|
import logging
|
|
|
|
from pyvlx import PyVLX, PyVLXException
|
|
import voluptuous as vol
|
|
|
|
from homeassistant.const import CONF_HOST, CONF_PASSWORD, EVENT_HOMEASSISTANT_STOP
|
|
from homeassistant.helpers import discovery
|
|
import homeassistant.helpers.config_validation as cv
|
|
|
|
DOMAIN = "velux"
|
|
DATA_VELUX = "data_velux"
|
|
PLATFORMS = ["cover", "scene"]
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
CONFIG_SCHEMA = vol.Schema(
|
|
{
|
|
DOMAIN: vol.Schema(
|
|
{vol.Required(CONF_HOST): cv.string, vol.Required(CONF_PASSWORD): cv.string}
|
|
)
|
|
},
|
|
extra=vol.ALLOW_EXTRA,
|
|
)
|
|
|
|
|
|
async def async_setup(hass, config):
|
|
"""Set up the velux component."""
|
|
try:
|
|
hass.data[DATA_VELUX] = VeluxModule(hass, config[DOMAIN])
|
|
hass.data[DATA_VELUX].setup()
|
|
await hass.data[DATA_VELUX].async_start()
|
|
|
|
except PyVLXException as ex:
|
|
_LOGGER.exception("Can't connect to velux interface: %s", ex)
|
|
return False
|
|
|
|
for platform in PLATFORMS:
|
|
hass.async_create_task(
|
|
discovery.async_load_platform(hass, platform, DOMAIN, {}, config)
|
|
)
|
|
return True
|
|
|
|
|
|
class VeluxModule:
|
|
"""Abstraction for velux component."""
|
|
|
|
def __init__(self, hass, domain_config):
|
|
"""Initialize for velux component."""
|
|
self.pyvlx = None
|
|
self._hass = hass
|
|
self._domain_config = domain_config
|
|
|
|
def setup(self):
|
|
"""Velux component setup."""
|
|
|
|
async def on_hass_stop(event):
|
|
"""Close connection when hass stops."""
|
|
_LOGGER.debug("Velux interface terminated")
|
|
await self.pyvlx.disconnect()
|
|
|
|
async def async_reboot_gateway(service_call):
|
|
await self.pyvlx.reboot_gateway()
|
|
|
|
self._hass.bus.async_listen_once(EVENT_HOMEASSISTANT_STOP, on_hass_stop)
|
|
host = self._domain_config.get(CONF_HOST)
|
|
password = self._domain_config.get(CONF_PASSWORD)
|
|
self.pyvlx = PyVLX(host=host, password=password)
|
|
|
|
self._hass.services.async_register(
|
|
DOMAIN, "reboot_gateway", async_reboot_gateway
|
|
)
|
|
|
|
async def async_start(self):
|
|
"""Start velux component."""
|
|
_LOGGER.debug("Velux interface started")
|
|
await self.pyvlx.load_scenes()
|
|
await self.pyvlx.load_nodes()
|