mirror of
https://github.com/home-assistant/core
synced 2024-08-02 23:40:32 +02:00
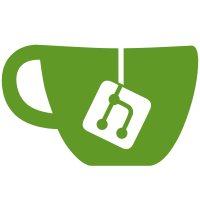
* add CONF_STATE_CLASS to const.py
* move to `sensor/__init__.py`
* move to sensor/const.py
* Revert "move to sensor/const.py"
This reverts commit 604d0d066b
.
* move it to `sensor/const.py` but import it from `sensor/__init__.py`
* add Modbus
88 lines
2.9 KiB
Python
88 lines
2.9 KiB
Python
"""Support for KNX/IP sensors."""
|
|
from __future__ import annotations
|
|
|
|
from typing import Any
|
|
|
|
from xknx import XKNX
|
|
from xknx.devices import Sensor as XknxSensor
|
|
|
|
from homeassistant.components.sensor import (
|
|
CONF_STATE_CLASS,
|
|
DEVICE_CLASSES,
|
|
SensorEntity,
|
|
)
|
|
from homeassistant.const import CONF_NAME, CONF_TYPE
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.helpers.entity_platform import AddEntitiesCallback
|
|
from homeassistant.helpers.typing import ConfigType, DiscoveryInfoType, StateType
|
|
from homeassistant.util import dt
|
|
|
|
from .const import ATTR_LAST_KNX_UPDATE, ATTR_SOURCE, DOMAIN
|
|
from .knx_entity import KnxEntity
|
|
from .schema import SensorSchema
|
|
|
|
|
|
async def async_setup_platform(
|
|
hass: HomeAssistant,
|
|
config: ConfigType,
|
|
async_add_entities: AddEntitiesCallback,
|
|
discovery_info: DiscoveryInfoType | None = None,
|
|
) -> None:
|
|
"""Set up sensor(s) for KNX platform."""
|
|
if not discovery_info or not discovery_info["platform_config"]:
|
|
return
|
|
platform_config = discovery_info["platform_config"]
|
|
xknx: XKNX = hass.data[DOMAIN].xknx
|
|
|
|
async_add_entities(
|
|
KNXSensor(xknx, entity_config) for entity_config in platform_config
|
|
)
|
|
|
|
|
|
def _create_sensor(xknx: XKNX, config: ConfigType) -> XknxSensor:
|
|
"""Return a KNX sensor to be used within XKNX."""
|
|
return XknxSensor(
|
|
xknx,
|
|
name=config[CONF_NAME],
|
|
group_address_state=config[SensorSchema.CONF_STATE_ADDRESS],
|
|
sync_state=config[SensorSchema.CONF_SYNC_STATE],
|
|
always_callback=config[SensorSchema.CONF_ALWAYS_CALLBACK],
|
|
value_type=config[CONF_TYPE],
|
|
)
|
|
|
|
|
|
class KNXSensor(KnxEntity, SensorEntity):
|
|
"""Representation of a KNX sensor."""
|
|
|
|
_device: XknxSensor
|
|
|
|
def __init__(self, xknx: XKNX, config: ConfigType) -> None:
|
|
"""Initialize of a KNX sensor."""
|
|
super().__init__(_create_sensor(xknx, config))
|
|
self._attr_device_class = (
|
|
self._device.ha_device_class()
|
|
if self._device.ha_device_class() in DEVICE_CLASSES
|
|
else None
|
|
)
|
|
self._attr_force_update = self._device.always_callback
|
|
self._attr_unique_id = str(self._device.sensor_value.group_address_state)
|
|
self._attr_native_unit_of_measurement = self._device.unit_of_measurement()
|
|
self._attr_state_class = config.get(CONF_STATE_CLASS)
|
|
|
|
@property
|
|
def native_value(self) -> StateType:
|
|
"""Return the state of the sensor."""
|
|
return self._device.resolve_state()
|
|
|
|
@property
|
|
def extra_state_attributes(self) -> dict[str, Any] | None:
|
|
"""Return device specific state attributes."""
|
|
attr: dict[str, Any] = {}
|
|
|
|
if self._device.last_telegram is not None:
|
|
attr[ATTR_SOURCE] = str(self._device.last_telegram.source_address)
|
|
attr[ATTR_LAST_KNX_UPDATE] = str(
|
|
dt.as_utc(self._device.last_telegram.timestamp)
|
|
)
|
|
return attr
|