mirror of
https://github.com/home-assistant/core
synced 2024-08-02 23:40:32 +02:00
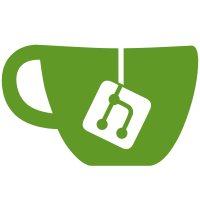
* Add config_flow to Environment Canada * Add unique_id * Remove erroneous directory. * Tests working!! * Add back setup. * First cut of import. * Temp * Tweak names. * Import config.yaml. * Clean up imports. * Import working! Some refactor to clean it up. * Add import test. * Small optimization. * Fix comments from code review. * Remove CONF_NAME and config_flow for it. * Fixup strings to match new config_flow. * Fixes for comments from last review. * Update tests to match new import code. * Clean up use of CONF_TITLE; fix lint error on push. * Phew. More cleanup on import. Really streamlined now! * Update tests. * Fix lint error. * Fix lint error, try 2. * Revert unique_id to use location as part of ID. * Fix code review comments. * Fix review comments.
80 lines
2.3 KiB
Python
80 lines
2.3 KiB
Python
"""The Environment Canada (EC) component."""
|
|
from functools import partial
|
|
import logging
|
|
|
|
from env_canada import ECData, ECRadar
|
|
|
|
from homeassistant.config_entries import SOURCE_IMPORT
|
|
from homeassistant.const import CONF_LATITUDE, CONF_LONGITUDE
|
|
|
|
from .const import CONF_LANGUAGE, CONF_STATION, DOMAIN
|
|
|
|
PLATFORMS = ["camera", "sensor", "weather"]
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
|
|
async def async_setup_entry(hass, config_entry):
|
|
"""Set up EC as config entry."""
|
|
lat = config_entry.data.get(CONF_LATITUDE)
|
|
lon = config_entry.data.get(CONF_LONGITUDE)
|
|
station = config_entry.data.get(CONF_STATION)
|
|
lang = config_entry.data.get(CONF_LANGUAGE, "English")
|
|
|
|
weather_api = {}
|
|
|
|
weather_init = partial(
|
|
ECData, station_id=station, coordinates=(lat, lon), language=lang.lower()
|
|
)
|
|
weather_data = await hass.async_add_executor_job(weather_init)
|
|
weather_api["weather_data"] = weather_data
|
|
|
|
radar_init = partial(ECRadar, coordinates=(lat, lon))
|
|
radar_data = await hass.async_add_executor_job(radar_init)
|
|
weather_api["radar_data"] = radar_data
|
|
await hass.async_add_executor_job(radar_data.get_loop)
|
|
|
|
hass.data.setdefault(DOMAIN, {})
|
|
hass.data[DOMAIN][config_entry.entry_id] = weather_api
|
|
|
|
hass.config_entries.async_setup_platforms(config_entry, PLATFORMS)
|
|
|
|
return True
|
|
|
|
|
|
async def async_unload_entry(hass, config_entry):
|
|
"""Unload a config entry."""
|
|
unload_ok = await hass.config_entries.async_unload_platforms(
|
|
config_entry, PLATFORMS
|
|
)
|
|
|
|
hass.data[DOMAIN].pop(config_entry.entry_id)
|
|
|
|
return unload_ok
|
|
|
|
|
|
def trigger_import(hass, config):
|
|
"""Trigger a import of YAML config into a config_entry."""
|
|
_LOGGER.warning(
|
|
"Environment Canada YAML configuration is deprecated; your YAML configuration "
|
|
"has been imported into the UI and can be safely removed"
|
|
)
|
|
if not config.get(CONF_LANGUAGE):
|
|
config[CONF_LANGUAGE] = "English"
|
|
|
|
data = {}
|
|
for key in (
|
|
CONF_STATION,
|
|
CONF_LATITUDE,
|
|
CONF_LONGITUDE,
|
|
CONF_LANGUAGE,
|
|
): # pylint: disable=consider-using-tuple
|
|
if config.get(key):
|
|
data[key] = config[key]
|
|
|
|
hass.async_create_task(
|
|
hass.config_entries.flow.async_init(
|
|
DOMAIN, context={"source": SOURCE_IMPORT}, data=data
|
|
)
|
|
)
|