mirror of
https://github.com/home-assistant/core
synced 2024-08-02 23:40:32 +02:00
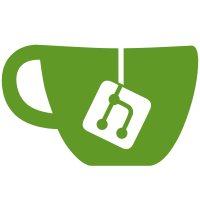
Co-authored-by: J. Nick Koston <nick@koston.org> Co-authored-by: Teemu R. <tpr@iki.fi> Co-authored-by: Martin Hjelmare <marhje52@gmail.com>
58 lines
2.0 KiB
Python
58 lines
2.0 KiB
Python
"""Component to embed TP-Link smart home devices."""
|
|
from __future__ import annotations
|
|
|
|
from datetime import timedelta
|
|
import logging
|
|
|
|
from kasa import SmartDevice, SmartDeviceException
|
|
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.helpers.debounce import Debouncer
|
|
from homeassistant.helpers.update_coordinator import DataUpdateCoordinator, UpdateFailed
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
REQUEST_REFRESH_DELAY = 0.35
|
|
|
|
|
|
class TPLinkDataUpdateCoordinator(DataUpdateCoordinator):
|
|
"""DataUpdateCoordinator to gather data for a specific TPLink device."""
|
|
|
|
def __init__(
|
|
self,
|
|
hass: HomeAssistant,
|
|
device: SmartDevice,
|
|
) -> None:
|
|
"""Initialize DataUpdateCoordinator to gather data for specific SmartPlug."""
|
|
self.device = device
|
|
self.update_children = True
|
|
update_interval = timedelta(seconds=10)
|
|
super().__init__(
|
|
hass,
|
|
_LOGGER,
|
|
name=device.host,
|
|
update_interval=update_interval,
|
|
# We don't want an immediate refresh since the device
|
|
# takes a moment to reflect the state change
|
|
request_refresh_debouncer=Debouncer(
|
|
hass, _LOGGER, cooldown=REQUEST_REFRESH_DELAY, immediate=False
|
|
),
|
|
)
|
|
|
|
async def async_request_refresh_without_children(self) -> None:
|
|
"""Request a refresh without the children."""
|
|
# If the children do get updated this is ok as this is an
|
|
# optimization to reduce the number of requests on the device
|
|
# when we do not need it.
|
|
self.update_children = False
|
|
await self.async_request_refresh()
|
|
|
|
async def _async_update_data(self) -> None:
|
|
"""Fetch all device and sensor data from api."""
|
|
try:
|
|
await self.device.update(update_children=self.update_children)
|
|
except SmartDeviceException as ex:
|
|
raise UpdateFailed(ex) from ex
|
|
finally:
|
|
self.update_children = True
|