mirror of
https://github.com/home-assistant/core
synced 2024-08-02 23:40:32 +02:00
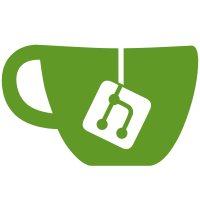
* Strict typing * Rebase * Tweak import * Cleanup * Rebase + typing hub * Flake8 * Update homeassistant/components/neato/config_flow.py Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Update homeassistant/components/neato/vacuum.py Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Update homeassistant/components/neato/camera.py Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Address review comments * Black * Update homeassistant/components/neato/config_flow.py Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Specific dict definition * Annotations Co-authored-by: Martin Hjelmare <marhje52@gmail.com>
67 lines
2.4 KiB
Python
67 lines
2.4 KiB
Python
"""Config flow for Neato Botvac."""
|
|
from __future__ import annotations
|
|
|
|
import logging
|
|
from types import MappingProxyType
|
|
from typing import Any
|
|
|
|
import voluptuous as vol
|
|
|
|
from homeassistant.config_entries import SOURCE_REAUTH
|
|
from homeassistant.data_entry_flow import FlowResult
|
|
from homeassistant.helpers import config_entry_oauth2_flow
|
|
|
|
from .const import NEATO_DOMAIN
|
|
|
|
|
|
class OAuth2FlowHandler(
|
|
config_entry_oauth2_flow.AbstractOAuth2FlowHandler, domain=NEATO_DOMAIN
|
|
):
|
|
"""Config flow to handle Neato Botvac OAuth2 authentication."""
|
|
|
|
DOMAIN = NEATO_DOMAIN
|
|
|
|
@property
|
|
def logger(self) -> logging.Logger:
|
|
"""Return logger."""
|
|
return logging.getLogger(__name__)
|
|
|
|
async def async_step_user(
|
|
self, user_input: dict[str, Any] | None = None
|
|
) -> FlowResult:
|
|
"""Create an entry for the flow."""
|
|
current_entries = self._async_current_entries()
|
|
if self.source != SOURCE_REAUTH and current_entries:
|
|
# Already configured
|
|
return self.async_abort(reason="already_configured")
|
|
|
|
return await super().async_step_user(user_input=user_input)
|
|
|
|
async def async_step_reauth(self, data: MappingProxyType[str, Any]) -> FlowResult:
|
|
"""Perform reauth upon migration of old entries."""
|
|
return await self.async_step_reauth_confirm()
|
|
|
|
async def async_step_reauth_confirm(
|
|
self, user_input: dict[str, Any] | None = None
|
|
) -> FlowResult:
|
|
"""Confirm reauth upon migration of old entries."""
|
|
if user_input is None:
|
|
return self.async_show_form(
|
|
step_id="reauth_confirm", data_schema=vol.Schema({})
|
|
)
|
|
return await self.async_step_user()
|
|
|
|
async def async_oauth_create_entry(self, data: dict[str, Any]) -> FlowResult:
|
|
"""Create an entry for the flow. Update an entry if one already exist."""
|
|
current_entries = self._async_current_entries()
|
|
if self.source == SOURCE_REAUTH and current_entries:
|
|
# Update entry
|
|
self.hass.config_entries.async_update_entry(
|
|
current_entries[0], title=self.flow_impl.name, data=data
|
|
)
|
|
self.hass.async_create_task(
|
|
self.hass.config_entries.async_reload(current_entries[0].entry_id)
|
|
)
|
|
return self.async_abort(reason="reauth_successful")
|
|
return self.async_create_entry(title=self.flow_impl.name, data=data)
|