mirror of
https://github.com/home-assistant/core
synced 2024-09-03 08:14:07 +02:00
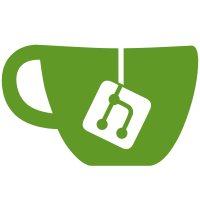
* Extracting group and extra info from ihc products * Make suggested area not optional * Revert back to assignment expression := * Make auto setup show device info for all platforms * Change code comment to # * Add return typing * Remove device_info key without value * get_manual_configuration typings for everything * Adding IHCController typings * Remove "ihc" from unique id * Remove device_info * Separator in unique id * Return typing on ihc_setup Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Update homeassistant/components/ihc/ihcdevice.py Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Update homeassistant/components/ihc/ihcdevice.py Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Raise ValueError instead of logging an error * Update homeassistant/components/ihc/service_functions.py Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Catch up with dev Co-authored-by: Martin Hjelmare <marhje52@gmail.com>
86 lines
2.6 KiB
Python
86 lines
2.6 KiB
Python
"""Support for IHC binary sensors."""
|
|
from __future__ import annotations
|
|
|
|
from ihcsdk.ihccontroller import IHCController
|
|
|
|
from homeassistant.components.binary_sensor import BinarySensorEntity
|
|
from homeassistant.const import CONF_TYPE
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.helpers.entity_platform import AddEntitiesCallback
|
|
from homeassistant.helpers.typing import ConfigType, DiscoveryInfoType
|
|
|
|
from .const import CONF_INVERTING, DOMAIN, IHC_CONTROLLER
|
|
from .ihcdevice import IHCDevice
|
|
|
|
|
|
def setup_platform(
|
|
hass: HomeAssistant,
|
|
config: ConfigType,
|
|
add_entities: AddEntitiesCallback,
|
|
discovery_info: DiscoveryInfoType | None = None,
|
|
) -> None:
|
|
"""Set up the IHC binary sensor platform."""
|
|
if discovery_info is None:
|
|
return
|
|
devices = []
|
|
for name, device in discovery_info.items():
|
|
ihc_id = device["ihc_id"]
|
|
product_cfg = device["product_cfg"]
|
|
product = device["product"]
|
|
# Find controller that corresponds with device id
|
|
controller_id = device["ctrl_id"]
|
|
ihc_controller: IHCController = hass.data[DOMAIN][controller_id][IHC_CONTROLLER]
|
|
sensor = IHCBinarySensor(
|
|
ihc_controller,
|
|
controller_id,
|
|
name,
|
|
ihc_id,
|
|
product_cfg.get(CONF_TYPE),
|
|
product_cfg[CONF_INVERTING],
|
|
product,
|
|
)
|
|
devices.append(sensor)
|
|
add_entities(devices)
|
|
|
|
|
|
class IHCBinarySensor(IHCDevice, BinarySensorEntity):
|
|
"""IHC Binary Sensor.
|
|
|
|
The associated IHC resource can be any in or output from a IHC product
|
|
or function block, but it must be a boolean ON/OFF resources.
|
|
"""
|
|
|
|
def __init__(
|
|
self,
|
|
ihc_controller: IHCController,
|
|
controller_id: str,
|
|
name: str,
|
|
ihc_id: int,
|
|
sensor_type: str,
|
|
inverting: bool,
|
|
product=None,
|
|
) -> None:
|
|
"""Initialize the IHC binary sensor."""
|
|
super().__init__(ihc_controller, controller_id, name, ihc_id, product)
|
|
self._state = None
|
|
self._sensor_type = sensor_type
|
|
self.inverting = inverting
|
|
|
|
@property
|
|
def device_class(self):
|
|
"""Return the class of this sensor."""
|
|
return self._sensor_type
|
|
|
|
@property
|
|
def is_on(self):
|
|
"""Return true if the binary sensor is on/open."""
|
|
return self._state
|
|
|
|
def on_ihc_change(self, ihc_id, value):
|
|
"""IHC resource has changed."""
|
|
if self.inverting:
|
|
self._state = not value
|
|
else:
|
|
self._state = value
|
|
self.schedule_update_ha_state()
|