mirror of
https://github.com/home-assistant/core
synced 2024-10-07 10:13:38 +02:00
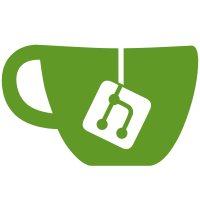
* Initial implementation of Vilfo router integration. This commit is a combination of several commits, with commit messages in bullet form below. * Added additional files to Vilfo integration. * Added generated files. * Fixed alphabetic order in generated config_flows. * Continued implementation of config flow for Vilfo integration. * Continued work on config_flow for Vilfo. * Updated requirements in manifest for Vilfo Router integration. * Some strings added to Vilfo Router integration. * Vilfo Router integration updated with sensor support. * Code style cleanup. * Additional cleanup of config flow. * Added additional UI strings for Vilfo Router * Updated tests of config flow and fixed formatting * Updated requirement upon vilfo-api-client. * Sensor refactoring including support for icons * Code style changes for Vilfo Router integration * Code cleanup * Fixed linting issues in Vilfo Router integration * Fixed import order in test for Vilfo integration. * Updates to Vilfo Router integration based on feedback Based on the feedback received, updates have been made to the Vilfo Router integration. A couple of the points mentioned have not been addressed yet, since the appropriate action has not yet been determined. These are: * https://github.com/home-assistant/home-assistant/pull/31177#discussion_r371124477 * https://github.com/home-assistant/home-assistant/pull/31177#discussion_r371202896 This commit consists of: * Removed unused folder/submodule * Fixes to __init__ * Fixes to config_flow * Fixes to const * Refactored sensors and applied fixes * Fix issue with wrong exception type in config flow * Updated tests for Vilfo integration config_flow * Updated dependency upon vilfo-api-client to improve testability * Import order fixes in test * Use constants instead of strings in tests * Updated the VilfoRouterData class to only use the hostname as unique_id when it is the default one (admin.vilfo.com). * Refactored based on feedback during review. * Changes to constant names, * Blocking IO separated to executor job, * Data for uptime sensor changed from being computed to being a timestamp, * Started refactoring uptime sensor in terms of naming and unit. * Updated constants for boot time (previously uptime) sensor. * Refactored test of Vilfo config flow to avoid patching code under test. * UI naming fixes and better exception handling. * Removed unused exception class. * Various changes to Vilfo Router integration. * Removed unit of measurement for boot time sensor, * Added support for a sensor not having a unit, * Updated the config_flow to handle when the integration is already configured, * Updated tests to avoid mocking the code under test and also to cover the aforementioned changes. * Exception handling in Vilfo Router config flow refactored to be more readable. * Refactored constant usage, fixed sensor availability and fix API client library doing I/O in async context. * Updated signature with hass first * Update call to constructor with changed order of arguments
126 lines
3.7 KiB
Python
126 lines
3.7 KiB
Python
"""The Vilfo Router integration."""
|
|
import asyncio
|
|
from datetime import timedelta
|
|
import logging
|
|
|
|
from vilfo import Client as VilfoClient
|
|
from vilfo.exceptions import VilfoException
|
|
|
|
from homeassistant.config_entries import ConfigEntry
|
|
from homeassistant.const import CONF_ACCESS_TOKEN, CONF_HOST
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.exceptions import ConfigEntryNotReady
|
|
from homeassistant.helpers.typing import ConfigType, HomeAssistantType
|
|
from homeassistant.util import Throttle
|
|
|
|
from .const import ATTR_BOOT_TIME, ATTR_LOAD, DOMAIN, ROUTER_DEFAULT_HOST
|
|
|
|
PLATFORMS = ["sensor"]
|
|
|
|
DEFAULT_SCAN_INTERVAL = timedelta(seconds=30)
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
|
|
async def async_setup(hass: HomeAssistantType, config: ConfigType):
|
|
"""Set up the Vilfo Router component."""
|
|
hass.data.setdefault(DOMAIN, {})
|
|
return True
|
|
|
|
|
|
async def async_setup_entry(hass: HomeAssistant, entry: ConfigEntry):
|
|
"""Set up Vilfo Router from a config entry."""
|
|
host = entry.data[CONF_HOST]
|
|
access_token = entry.data[CONF_ACCESS_TOKEN]
|
|
|
|
vilfo_router = VilfoRouterData(hass, host, access_token)
|
|
|
|
await vilfo_router.async_update()
|
|
|
|
if not vilfo_router.available:
|
|
raise ConfigEntryNotReady
|
|
|
|
hass.data[DOMAIN][entry.entry_id] = vilfo_router
|
|
|
|
for platform in PLATFORMS:
|
|
hass.async_create_task(
|
|
hass.config_entries.async_forward_entry_setup(entry, platform)
|
|
)
|
|
|
|
return True
|
|
|
|
|
|
async def async_unload_entry(hass: HomeAssistant, entry: ConfigEntry):
|
|
"""Unload a config entry."""
|
|
unload_ok = all(
|
|
await asyncio.gather(
|
|
*[
|
|
hass.config_entries.async_forward_entry_unload(entry, platform)
|
|
for platform in PLATFORMS
|
|
]
|
|
)
|
|
)
|
|
if unload_ok:
|
|
hass.data[DOMAIN].pop(entry.entry_id)
|
|
|
|
return unload_ok
|
|
|
|
|
|
class VilfoRouterData:
|
|
"""Define an object to hold sensor data."""
|
|
|
|
def __init__(self, hass, host, access_token):
|
|
"""Initialize."""
|
|
self._vilfo = VilfoClient(host, access_token)
|
|
self.hass = hass
|
|
self.host = host
|
|
self.available = False
|
|
self.firmware_version = None
|
|
self.mac_address = self._vilfo.mac
|
|
self.data = {}
|
|
self._unavailable_logged = False
|
|
|
|
@property
|
|
def unique_id(self):
|
|
"""Get the unique_id for the Vilfo Router."""
|
|
if self.mac_address:
|
|
return self.mac_address
|
|
|
|
if self.host == ROUTER_DEFAULT_HOST:
|
|
return self.host
|
|
|
|
return self.host
|
|
|
|
def _fetch_data(self):
|
|
board_information = self._vilfo.get_board_information()
|
|
load = self._vilfo.get_load()
|
|
|
|
return {
|
|
"board_information": board_information,
|
|
"load": load,
|
|
}
|
|
|
|
@Throttle(DEFAULT_SCAN_INTERVAL)
|
|
async def async_update(self):
|
|
"""Update data using calls to VilfoClient library."""
|
|
try:
|
|
data = await self.hass.async_add_executor_job(self._fetch_data)
|
|
|
|
self.firmware_version = data["board_information"]["version"]
|
|
self.data[ATTR_BOOT_TIME] = data["board_information"]["bootTime"]
|
|
self.data[ATTR_LOAD] = data["load"]
|
|
|
|
self.available = True
|
|
except VilfoException as error:
|
|
if not self._unavailable_logged:
|
|
_LOGGER.error(
|
|
"Could not fetch data from %s, error: %s", self.host, error
|
|
)
|
|
self._unavailable_logged = True
|
|
self.available = False
|
|
return
|
|
|
|
if self.available and self._unavailable_logged:
|
|
_LOGGER.info("Vilfo Router %s is available again", self.host)
|
|
self._unavailable_logged = False
|