mirror of
https://github.com/home-assistant/core
synced 2024-10-01 05:30:36 +02:00
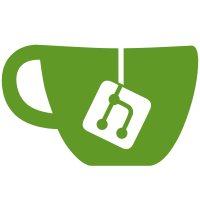
* Adds support for config entries and device registry * Fixing string formatting for logger * Add unit test for abode config flow * Fix for lights, only allow one config, add ability to unload entry * Fix for subscribing to hass_events on adding abode component * Several fixes from code review * Several fixes from second code review * Several fixes from third code review * Update documentation url to fix branch conflict * Fixes config flow and removes unused constants * Fix for switches, polling entry option, improved tests * Update .coveragerc, disable pylint W0611, remove polling from UI * Multiple fixes and edits to adhere to style guidelines * Removed unique_id * Minor correction for formatting error in rebase * Resolves issue causing CI to fail * Bump abodepy version * Add remove device callback and minor clean up * Fix incorrect method name * Docstring edits * Fix duplicate import issues from rebase * Add logout_listener attribute to AbodeSystem * Add additional test for complete coverage
69 lines
1.8 KiB
Python
69 lines
1.8 KiB
Python
"""Support for Abode Security System switches."""
|
|
import logging
|
|
|
|
import abodepy.helpers.constants as CONST
|
|
import abodepy.helpers.timeline as TIMELINE
|
|
|
|
from homeassistant.components.switch import SwitchDevice
|
|
|
|
from . import AbodeAutomation, AbodeDevice
|
|
from .const import DOMAIN
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
|
|
async def async_setup_platform(hass, config, async_add_entities, discovery_info=None):
|
|
"""Platform uses config entry setup."""
|
|
pass
|
|
|
|
|
|
async def async_setup_entry(hass, config_entry, async_add_entities):
|
|
"""Set up Abode switch devices."""
|
|
data = hass.data[DOMAIN]
|
|
|
|
devices = []
|
|
|
|
for device in data.abode.get_devices(generic_type=CONST.TYPE_SWITCH):
|
|
devices.append(AbodeSwitch(data, device))
|
|
|
|
for automation in data.abode.get_automations(generic_type=CONST.TYPE_AUTOMATION):
|
|
devices.append(
|
|
AbodeAutomationSwitch(data, automation, TIMELINE.AUTOMATION_EDIT_GROUP)
|
|
)
|
|
|
|
async_add_entities(devices)
|
|
|
|
|
|
class AbodeSwitch(AbodeDevice, SwitchDevice):
|
|
"""Representation of an Abode switch."""
|
|
|
|
def turn_on(self, **kwargs):
|
|
"""Turn on the device."""
|
|
self._device.switch_on()
|
|
|
|
def turn_off(self, **kwargs):
|
|
"""Turn off the device."""
|
|
self._device.switch_off()
|
|
|
|
@property
|
|
def is_on(self):
|
|
"""Return true if device is on."""
|
|
return self._device.is_on
|
|
|
|
|
|
class AbodeAutomationSwitch(AbodeAutomation, SwitchDevice):
|
|
"""A switch implementation for Abode automations."""
|
|
|
|
def turn_on(self, **kwargs):
|
|
"""Turn on the device."""
|
|
self._automation.set_active(True)
|
|
|
|
def turn_off(self, **kwargs):
|
|
"""Turn off the device."""
|
|
self._automation.set_active(False)
|
|
|
|
@property
|
|
def is_on(self):
|
|
"""Return True if the binary sensor is on."""
|
|
return self._automation.is_active
|