mirror of
https://github.com/home-assistant/core
synced 2024-08-02 23:40:32 +02:00
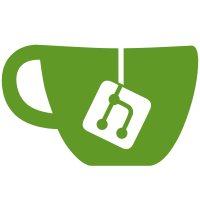
* Add config flow to eight_sleep * simplify tests * Remove extra file * remove unused import * fix redundant code * Update homeassistant/components/eight_sleep/__init__.py Co-authored-by: J. Nick Koston <nick@koston.org> * incorporate feedback * Review comments * remove typing from tests * Fix based on changes * Fix requirements * Remove stale comment * Fix tests * Reverse the flow and force the config entry to reconnect * Review comments * Abort if import flow fails * Split import and user logic * Fix error Co-authored-by: J. Nick Koston <nick@koston.org>
66 lines
2.0 KiB
Python
66 lines
2.0 KiB
Python
"""Support for Eight Sleep binary sensors."""
|
|
from __future__ import annotations
|
|
|
|
import logging
|
|
|
|
from pyeight.eight import EightSleep
|
|
|
|
from homeassistant.components.binary_sensor import (
|
|
BinarySensorDeviceClass,
|
|
BinarySensorEntity,
|
|
)
|
|
from homeassistant.config_entries import ConfigEntry
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.helpers.entity_platform import AddEntitiesCallback
|
|
from homeassistant.helpers.update_coordinator import DataUpdateCoordinator
|
|
|
|
from . import EightSleepBaseEntity, EightSleepConfigEntryData
|
|
from .const import DOMAIN
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
BINARY_SENSORS = ["bed_presence"]
|
|
|
|
|
|
async def async_setup_entry(
|
|
hass: HomeAssistant, entry: ConfigEntry, async_add_entities: AddEntitiesCallback
|
|
) -> None:
|
|
"""Set up the eight sleep binary sensor."""
|
|
config_entry_data: EightSleepConfigEntryData = hass.data[DOMAIN][entry.entry_id]
|
|
eight = config_entry_data.api
|
|
heat_coordinator = config_entry_data.heat_coordinator
|
|
async_add_entities(
|
|
EightHeatSensor(entry, heat_coordinator, eight, user.user_id, binary_sensor)
|
|
for user in eight.users.values()
|
|
for binary_sensor in BINARY_SENSORS
|
|
)
|
|
|
|
|
|
class EightHeatSensor(EightSleepBaseEntity, BinarySensorEntity):
|
|
"""Representation of a Eight Sleep heat-based sensor."""
|
|
|
|
_attr_device_class = BinarySensorDeviceClass.OCCUPANCY
|
|
|
|
def __init__(
|
|
self,
|
|
entry: ConfigEntry,
|
|
coordinator: DataUpdateCoordinator,
|
|
eight: EightSleep,
|
|
user_id: str | None,
|
|
sensor: str,
|
|
) -> None:
|
|
"""Initialize the sensor."""
|
|
super().__init__(entry, coordinator, eight, user_id, sensor)
|
|
assert self._user_obj
|
|
_LOGGER.debug(
|
|
"Presence Sensor: %s, Side: %s, User: %s",
|
|
sensor,
|
|
self._user_obj.side,
|
|
user_id,
|
|
)
|
|
|
|
@property
|
|
def is_on(self) -> bool:
|
|
"""Return true if the binary sensor is on."""
|
|
assert self._user_obj
|
|
return bool(self._user_obj.bed_presence)
|