mirror of
https://github.com/home-assistant/core
synced 2024-10-07 10:13:38 +02:00
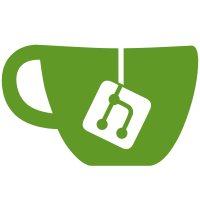
* Add support of Z-Wave.Me Z-Way and RaZberry server (#61182) Co-authored-by: Paulus Schoutsen <paulus@home-assistant.io> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> Co-authored-by: LawfulChaos <kerbalspacema@gmail.com> * Add switch platform to Z-Wave.Me integration (#64957) Co-authored-by: Martin Hjelmare <marhje52@gmail.com> Co-authored-by: Dmitry Vlasov <kerbalspacema@gmail.com> * Add button platform to Z-Wave.Me integration (#65109) Co-authored-by: epenet <6771947+epenet@users.noreply.github.com> Co-authored-by: Dmitry Vlasov <kerbalspacema@gmail.com> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Fix button controller access (#65117) * Add lock platform to Z-Wave.Me integration #65109 (#65114) Co-authored-by: epenet <6771947+epenet@users.noreply.github.com> Co-authored-by: Dmitry Vlasov <kerbalspacema@gmail.com> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Add sensor platform to Z-Wave.Me integration (#65132) * Sensor Entity * Sensor fixes * Apply suggestions from code review Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Inline descriotion according to review proposal * State Classes for sensor * Generic sensor * Generic sensor Co-authored-by: Dmitry Vlasov <kerbalspacema@gmail.com> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Add binary sensor platform to Z-Wave.Me integration (#65306) * Binary Sensor Entity * Update docstring Co-authored-by: Dmitry Vlasov <kerbalspacema@gmail.com> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Add Light Entity platform to Z-Wave.Me integration (#65331) * Light Entity * mypy fix * Fixes, ZWaveMePlatforms enum * Apply suggestions from code review Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Fixes * Fixes * Fixes Co-authored-by: Dmitry Vlasov <kerbalspacema@gmail.com> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Add Thermostat platform to Z-Wave.Me integration #65331 (#65371) * Climate entity * Climate entity * Apply suggestions from code review Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Climate entity fix * Clean up * cleanup * Import order fix * Correct naming Co-authored-by: Dmitry Vlasov <kerbalspacema@gmail.com> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Correct zwave_me .coveragerc (#65491) Co-authored-by: Martin Hjelmare <marhje52@gmail.com> Co-authored-by: Paulus Schoutsen <paulus@home-assistant.io> Co-authored-by: Martin Hjelmare <marhje52@gmail.com> Co-authored-by: LawfulChaos <kerbalspacema@gmail.com> Co-authored-by: epenet <6771947+epenet@users.noreply.github.com>
61 lines
1.7 KiB
Python
61 lines
1.7 KiB
Python
"""Representation of a doorlock."""
|
|
from __future__ import annotations
|
|
|
|
from typing import Any
|
|
|
|
from zwave_me_ws import ZWaveMeData
|
|
|
|
from homeassistant.components.lock import LockEntity
|
|
from homeassistant.config_entries import ConfigEntry
|
|
from homeassistant.core import HomeAssistant, callback
|
|
from homeassistant.helpers.dispatcher import async_dispatcher_connect
|
|
from homeassistant.helpers.entity_platform import AddEntitiesCallback
|
|
|
|
from . import ZWaveMeEntity
|
|
from .const import DOMAIN, ZWaveMePlatform
|
|
|
|
DEVICE_NAME = ZWaveMePlatform.LOCK
|
|
|
|
|
|
async def async_setup_entry(
|
|
hass: HomeAssistant,
|
|
config_entry: ConfigEntry,
|
|
async_add_entities: AddEntitiesCallback,
|
|
) -> None:
|
|
"""Set up the lock platform."""
|
|
|
|
@callback
|
|
def add_new_device(new_device: ZWaveMeData) -> None:
|
|
"""Add a new device."""
|
|
controller = hass.data[DOMAIN][config_entry.entry_id]
|
|
lock = ZWaveMeLock(controller, new_device)
|
|
|
|
async_add_entities(
|
|
[
|
|
lock,
|
|
]
|
|
)
|
|
|
|
config_entry.async_on_unload(
|
|
async_dispatcher_connect(
|
|
hass, f"ZWAVE_ME_NEW_{DEVICE_NAME.upper()}", add_new_device
|
|
)
|
|
)
|
|
|
|
|
|
class ZWaveMeLock(ZWaveMeEntity, LockEntity):
|
|
"""Representation of a ZWaveMe lock."""
|
|
|
|
@property
|
|
def is_locked(self) -> bool:
|
|
"""Return the state of the lock."""
|
|
return self.device.level == "close"
|
|
|
|
def unlock(self, **kwargs: Any) -> None:
|
|
"""Send command to unlock the lock."""
|
|
self.controller.zwave_api.send_command(self.device.id, "open")
|
|
|
|
def lock(self, **kwargs: Any) -> None:
|
|
"""Send command to lock the lock."""
|
|
self.controller.zwave_api.send_command(self.device.id, "close")
|