mirror of
https://github.com/home-assistant/core
synced 2024-10-04 07:58:43 +02:00
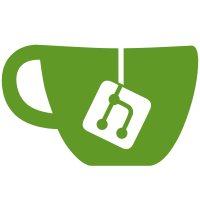
* Adding basic Plum Lightpad support - https://plumlife.com/ * Used Const values is_on is a bool * Added LightpadPowerMeter Sensor to the plum_lightpad platform * Moved to async setup, Introduced a PlumManager, events, subscription, Light and Power meter working * Added PlumMotionSensor * Added Glow Ring support * Updated plum library and re-normalized * set the glow-ring's icon * Naming the glow ring * Formatting and linting * Cleaned up a number of linting issues. Left a number of documentation warnings * setup_platform migrated to async_setup_platform Plum discovery run as a job * bumped plumlightpad version * On shutdown disconnect the telnet session from each plum lightpad * Cleanup & formatting. Worked on parallell cloud update * Moved setup from async to non-async * Utilize async_call_later from the helpers * Cleanedup and linted, down to documentation & one #TODO * Remove commented out debug lines * Fixed Linting issues * Remove TODO * Updated comments & fixed Linting issues * Added plumlightpad to requirements_all.txt * Fixing imports with isort * Added components to .coveragerc * Added PLUM_DATA constant for accessing hass.data[PLUM_DATA] * used dictionary syntax vs get(...) for config * Linting needed an additonal line * Fully async_setup now. removed @callback utilize bus events for detecting new devices found. * Upgraded to plumlightpad 0.0.10 * Removed extra unused PLATFORM_SCHEMA declarations * Moved listener attachment to `async_added_to_hass` and removed unused properties & device_state_attributes * Utilized Discovery when devices were located * Linting and cleanup * used `hass.async_create_task` instead of `hass.async_add_job` per Martin * Removed redundant criteria in if block * Without discovery info, there is no need to setup * Better state management and async on/off for Glow Ring * renamed async_set_config back to set_config, fixed cleanup callback and Plum Initialization * Fixed flake8 linting issues * plumlightpad package update * Add 'motion' device_class to Motion Sensor * Fixed last known Linting issue * let homeassistant handle setting the brightness state * String formatting vs concatenation * use shared aiohttp session from homeassistant * Updating to use new formatting style * looks like @cleanup isn't neccesary * ditch the serial awaits * Ensure async_add_entities is only called once per async_setup_platform * Creating tasks to wait for vs coroutines * Remove unused white component in the GlowRing * Used local variables for GlowRing colors & added a setter for the hs_color property to keep the values in sync * Linted and added docstring * Update the documentation path to point to the component page * Removed the extra sensor and binary_sensor platforms as requested. (To be added in later PRs) * Update plum_lightpad.py * Update plum_lightpad.py
77 lines
2.2 KiB
Python
77 lines
2.2 KiB
Python
"""
|
|
Support for Plum Lightpad switches.
|
|
|
|
For more details about this component, please refer to the documentation at
|
|
https://home-assistant.io/components/plum_lightpad
|
|
"""
|
|
import asyncio
|
|
import logging
|
|
|
|
import voluptuous as vol
|
|
|
|
from homeassistant.const import (
|
|
CONF_PASSWORD, CONF_USERNAME, EVENT_HOMEASSISTANT_STOP)
|
|
from homeassistant.helpers import discovery
|
|
from homeassistant.helpers.aiohttp_client import async_get_clientsession
|
|
import homeassistant.helpers.config_validation as cv
|
|
|
|
REQUIREMENTS = ['plumlightpad==0.0.11']
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
DOMAIN = 'plum_lightpad'
|
|
|
|
CONFIG_SCHEMA = vol.Schema({
|
|
DOMAIN: vol.Schema({
|
|
vol.Required(CONF_USERNAME): cv.string,
|
|
vol.Required(CONF_PASSWORD): cv.string,
|
|
}),
|
|
}, extra=vol.ALLOW_EXTRA)
|
|
|
|
PLUM_DATA = 'plum'
|
|
|
|
|
|
async def async_setup(hass, config):
|
|
"""Plum Lightpad Platform initialization."""
|
|
from plumlightpad import Plum
|
|
|
|
conf = config[DOMAIN]
|
|
plum = Plum(conf[CONF_USERNAME], conf[CONF_PASSWORD])
|
|
|
|
hass.data[PLUM_DATA] = plum
|
|
|
|
def cleanup(event):
|
|
"""Clean up resources."""
|
|
plum.cleanup()
|
|
|
|
hass.bus.async_listen_once(EVENT_HOMEASSISTANT_STOP, cleanup)
|
|
|
|
cloud_web_sesison = async_get_clientsession(hass, verify_ssl=True)
|
|
await plum.loadCloudData(cloud_web_sesison)
|
|
|
|
async def new_load(device):
|
|
"""Load light and sensor platforms when LogicalLoad is detected."""
|
|
await asyncio.wait([
|
|
hass.async_create_task(
|
|
discovery.async_load_platform(
|
|
hass, 'light', DOMAIN,
|
|
discovered=device, hass_config=conf))
|
|
])
|
|
|
|
async def new_lightpad(device):
|
|
"""Load light and binary sensor platforms when Lightpad detected."""
|
|
await asyncio.wait([
|
|
hass.async_create_task(
|
|
discovery.async_load_platform(
|
|
hass, 'light', DOMAIN,
|
|
discovered=device, hass_config=conf))
|
|
])
|
|
|
|
device_web_session = async_get_clientsession(hass, verify_ssl=False)
|
|
hass.async_create_task(
|
|
plum.discover(hass.loop,
|
|
loadListener=new_load, lightpadListener=new_lightpad,
|
|
websession=device_web_session))
|
|
|
|
return True
|