mirror of
https://github.com/home-assistant/core
synced 2024-08-31 05:57:13 +02:00
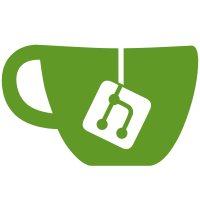
* Add mysensors notify platform * Make add_devices optional in platform callback function. * Use new argument structure for all existing mysensors platforms. * Add notify platform. * Update mysensors gateway. * Refactor notify setup * Enable discovery of notify platforms. * Update and add tests for notify component and some platforms. * Continue setup of notify platforms if a platform fails setup. * Remove notify tests that check platform config. These tests are not needed when config validation is used. * Add config validation to APNS notify platform. * Use discovery to set up mysensors notify platform. * Add discovery_info to get_service and update tests * Add discovery_info as keyword argument to the get_service function signature and update all notify platforms. * Update existing notify tests to check config validation using test helper. * Add removed tests back in that checked config in apns, command_line and file platforms, but use config validation test helper to verify config. * Add a test for notify file to increase coverage. * Fix some PEP issues. * Fix comments and use more constants * Move apns notify service under notify domain
59 lines
1.9 KiB
Python
59 lines
1.9 KiB
Python
"""
|
|
Support for file notification.
|
|
|
|
For more details about this platform, please refer to the documentation at
|
|
https://home-assistant.io/components/notify.file/
|
|
"""
|
|
import logging
|
|
import os
|
|
|
|
import voluptuous as vol
|
|
|
|
import homeassistant.util.dt as dt_util
|
|
from homeassistant.components.notify import (
|
|
ATTR_TITLE, ATTR_TITLE_DEFAULT, PLATFORM_SCHEMA, BaseNotificationService)
|
|
from homeassistant.const import CONF_FILENAME
|
|
import homeassistant.helpers.config_validation as cv
|
|
|
|
CONF_TIMESTAMP = 'timestamp'
|
|
|
|
PLATFORM_SCHEMA = PLATFORM_SCHEMA.extend({
|
|
vol.Required(CONF_FILENAME): cv.string,
|
|
vol.Optional(CONF_TIMESTAMP, default=False): cv.boolean,
|
|
})
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
|
|
def get_service(hass, config, discovery_info=None):
|
|
"""Get the file notification service."""
|
|
filename = config[CONF_FILENAME]
|
|
timestamp = config[CONF_TIMESTAMP]
|
|
|
|
return FileNotificationService(hass, filename, timestamp)
|
|
|
|
|
|
class FileNotificationService(BaseNotificationService):
|
|
"""Implement the notification service for the File service."""
|
|
|
|
def __init__(self, hass, filename, add_timestamp):
|
|
"""Initialize the service."""
|
|
self.filepath = os.path.join(hass.config.config_dir, filename)
|
|
self.add_timestamp = add_timestamp
|
|
|
|
def send_message(self, message="", **kwargs):
|
|
"""Send a message to a file."""
|
|
with open(self.filepath, 'a') as file:
|
|
if os.stat(self.filepath).st_size == 0:
|
|
title = '{} notifications (Log started: {})\n{}\n'.format(
|
|
kwargs.get(ATTR_TITLE, ATTR_TITLE_DEFAULT),
|
|
dt_util.utcnow().isoformat(),
|
|
'-' * 80)
|
|
file.write(title)
|
|
|
|
if self.add_timestamp:
|
|
text = '{} {}\n'.format(dt_util.utcnow().isoformat(), message)
|
|
else:
|
|
text = '{}\n'.format(message)
|
|
file.write(text)
|