mirror of
https://github.com/thepeacockproject/Peacock
synced 2024-11-22 22:12:45 +01:00
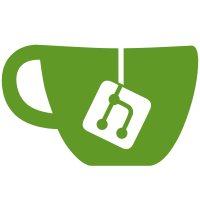
* Add multi-version mastery files * Add pro1 unlocks to legacy allunlockables * Add 47's suit to scpc all unlockables * Add and remove various configs * Remove some useless promises * Fix scpc hub * Fix issue with user profile saving * Fix scpc issues for hub * Add singleplayer/multiplayer sniper * A great many things - Add multi-version mastery - Improve sniper mastery support - Improve general H2016 support * Fix some warnings * Fix pro1 mastery on destination screens * Remove entP from createInventory, lock/unlock pro1 accordingly * Remove JSDoc entP parameter from createInventory * Remove difficultyunlocks from safehouse pages * Add versioned user profiles * Prettier run * Remove false point from user profiles docs * Add comment about profile versioning to types * Fix default profile links * Remove remaining lowercase * Fix sniper showing XP as XP * Add game versions to the unlockable map * Update getMasteryForUnlockable call in planning * Fix missing locations when updating profiles * Update versions to v7 * Fix ICA Facility destination mastery * Fix sniper challenge unlockables showing in inventory * Sniper Scoring (#273) * Initial sniper scoring * Fix linting errors * Update require table * Calculate and display final sniper score on end screen * Bump SMP version to v5.7.0 * Update since version for scoring * Fix create inventory call for sniper scoring * Support sniper unlockables in the inventory * Update versions to v7 * Reflect changes to createInventory in scoreHandler * Get unlockable name in completion data * It was not okay. * Thanks webstorm * Add support for /profiles/page/GetMasteryCompletionDataForUnlockable * Support sniper play next * Remove sniper gamemodes template from overrides * Remove debug prints from scoring event handler * Fix challenge multiplier * Exclude sniper unlockables from stashpoint * Start fixing up the missionEnd response for sniper * Update misleading comment * Use existing global challenge to check for SA on sniper contracts * Re-add removed global challenges * Proper support for the mission end screen on sniper contracts * Remove redundant label --------- Signed-off-by: Anthony Fuller <24512050+AnthonyFuller@users.noreply.github.com> Co-authored-by: Govert de Gans <grappigegovert@hotmail.com> * Add co-op sniper scoring defs * Update MasteryUnlockable template * Bump SMP version to v5.9.3 * Re-add deepmerge * Fix SMP checksum * Fix linting errors caused by merge * Fix score handler imports * Move load flags * Remove unnecessary game version arg * Whoopsies Co-authored-by: Reece Dunham <me@rdil.rocks> Signed-off-by: Anthony Fuller <24512050+AnthonyFuller@users.noreply.github.com> --------- Signed-off-by: Anthony Fuller <24512050+AnthonyFuller@users.noreply.github.com> Co-authored-by: Govert de Gans <grappigegovert@hotmail.com> Co-authored-by: Reece Dunham <me@rdil.rocks>
60 lines
2.0 KiB
TypeScript
60 lines
2.0 KiB
TypeScript
/*
|
|
* The Peacock Project - a HITMAN server replacement.
|
|
* Copyright (C) 2021-2023 The Peacock Project Team
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU Affero General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Affero General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Affero General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
import { getUserData, writeUserData } from "./databaseHandler"
|
|
import { getConfig } from "./configSwizzleManager"
|
|
import { ContractProgressionData } from "./types/types"
|
|
import { getFlag } from "./flags"
|
|
import { EVERGREEN_LEVEL_INFO } from "./utils"
|
|
|
|
export function setCpd(
|
|
data: ContractProgressionData,
|
|
uID: string,
|
|
cpdID: string,
|
|
) {
|
|
const userData = getUserData(uID, "h3")
|
|
|
|
userData.Extensions.CPD[cpdID] = {
|
|
...userData.Extensions.CPD[cpdID],
|
|
...data,
|
|
}
|
|
|
|
writeUserData(uID, "h3")
|
|
}
|
|
|
|
export function getCpd(uID: string, cpdID: string): ContractProgressionData {
|
|
const userData = getUserData(uID, "h3")
|
|
|
|
if (!Object.keys(userData.Extensions.CPD).includes(cpdID)) {
|
|
const defaultCPD = getConfig(
|
|
"DefaultCpdConfig",
|
|
false,
|
|
) as ContractProgressionData
|
|
|
|
setCpd(defaultCPD, uID, cpdID)
|
|
}
|
|
|
|
// NOTE: Override the EvergreenLevel with the latest Mastery Level
|
|
if (getFlag("gameplayUnlockAllFreelancerMasteries")) {
|
|
userData.Extensions.CPD[cpdID]["EvergreenLevel"] =
|
|
EVERGREEN_LEVEL_INFO.length
|
|
}
|
|
|
|
return userData.Extensions.CPD[cpdID]
|
|
}
|